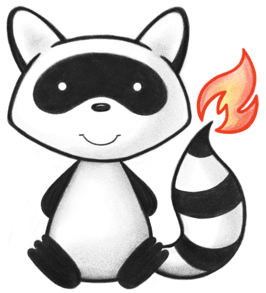
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableReference; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class FamilyMemberHistory43_50 { 046 047 public static org.hl7.fhir.r5.model.FamilyMemberHistory convertFamilyMemberHistory(org.hl7.fhir.r4b.model.FamilyMemberHistory src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.FamilyMemberHistory tgt = new org.hl7.fhir.r5.model.FamilyMemberHistory(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 055 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 056 for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 057 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 058 if (src.hasStatus()) 059 tgt.setStatusElement(convertFamilyHistoryStatus(src.getStatusElement())); 060 if (src.hasDataAbsentReason()) 061 tgt.setDataAbsentReason(CodeableConcept43_50.convertCodeableConcept(src.getDataAbsentReason())); 062 if (src.hasPatient()) 063 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 064 if (src.hasDate()) 065 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 066 if (src.hasName()) 067 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 068 if (src.hasRelationship()) 069 tgt.setRelationship(CodeableConcept43_50.convertCodeableConcept(src.getRelationship())); 070 if (src.hasSex()) 071 tgt.setSex(CodeableConcept43_50.convertCodeableConcept(src.getSex())); 072 if (src.hasBorn()) 073 tgt.setBorn(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getBorn())); 074 if (src.hasAge()) 075 tgt.setAge(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAge())); 076 if (src.hasEstimatedAge()) 077 tgt.setEstimatedAgeElement(Boolean43_50.convertBoolean(src.getEstimatedAgeElement())); 078 if (src.hasDeceased()) 079 tgt.setDeceased(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDeceased())); 080 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 081 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 082 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 083 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 084 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 085 for (org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent t : src.getCondition()) 086 tgt.addCondition(convertFamilyMemberHistoryConditionComponent(t)); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.r4b.model.FamilyMemberHistory convertFamilyMemberHistory(org.hl7.fhir.r5.model.FamilyMemberHistory src) throws FHIRException { 091 if (src == null) 092 return null; 093 org.hl7.fhir.r4b.model.FamilyMemberHistory tgt = new org.hl7.fhir.r4b.model.FamilyMemberHistory(); 094 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 095 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 096 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 097 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 098 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 099 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 100 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 101 if (src.hasStatus()) 102 tgt.setStatusElement(convertFamilyHistoryStatus(src.getStatusElement())); 103 if (src.hasDataAbsentReason()) 104 tgt.setDataAbsentReason(CodeableConcept43_50.convertCodeableConcept(src.getDataAbsentReason())); 105 if (src.hasPatient()) 106 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 107 if (src.hasDate()) 108 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 109 if (src.hasName()) 110 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 111 if (src.hasRelationship()) 112 tgt.setRelationship(CodeableConcept43_50.convertCodeableConcept(src.getRelationship())); 113 if (src.hasSex()) 114 tgt.setSex(CodeableConcept43_50.convertCodeableConcept(src.getSex())); 115 if (src.hasBorn()) 116 tgt.setBorn(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getBorn())); 117 if (src.hasAge()) 118 tgt.setAge(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAge())); 119 if (src.hasEstimatedAge()) 120 tgt.setEstimatedAgeElement(Boolean43_50.convertBoolean(src.getEstimatedAgeElement())); 121 if (src.hasDeceased()) 122 tgt.setDeceased(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDeceased())); 123 for (CodeableReference t : src.getReason()) 124 if (t.hasConcept()) 125 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 126 for (CodeableReference t : src.getReason()) 127 if (t.hasReference()) 128 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 129 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 130 for (org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent t : src.getCondition()) 131 tgt.addCondition(convertFamilyMemberHistoryConditionComponent(t)); 132 return tgt; 133 } 134 135 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus> convertFamilyHistoryStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus> src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatusEnumFactory()); 139 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 140 switch (src.getValue()) { 141 case PARTIAL: 142 tgt.setValue(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus.PARTIAL); 143 break; 144 case COMPLETED: 145 tgt.setValue(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus.COMPLETED); 146 break; 147 case ENTEREDINERROR: 148 tgt.setValue(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus.ENTEREDINERROR); 149 break; 150 case HEALTHUNKNOWN: 151 tgt.setValue(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus.HEALTHUNKNOWN); 152 break; 153 default: 154 tgt.setValue(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus.NULL); 155 break; 156 } 157 return tgt; 158 } 159 160 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus> convertFamilyHistoryStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyHistoryStatus> src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatusEnumFactory()); 164 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 165 switch (src.getValue()) { 166 case PARTIAL: 167 tgt.setValue(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus.PARTIAL); 168 break; 169 case COMPLETED: 170 tgt.setValue(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus.COMPLETED); 171 break; 172 case ENTEREDINERROR: 173 tgt.setValue(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus.ENTEREDINERROR); 174 break; 175 case HEALTHUNKNOWN: 176 tgt.setValue(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus.HEALTHUNKNOWN); 177 break; 178 default: 179 tgt.setValue(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyHistoryStatus.NULL); 180 break; 181 } 182 return tgt; 183 } 184 185 public static org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent convertFamilyMemberHistoryConditionComponent(org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent src) throws FHIRException { 186 if (src == null) 187 return null; 188 org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent tgt = new org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 189 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 190 if (src.hasCode()) 191 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 192 if (src.hasOutcome()) 193 tgt.setOutcome(CodeableConcept43_50.convertCodeableConcept(src.getOutcome())); 194 if (src.hasContributedToDeath()) 195 tgt.setContributedToDeathElement(Boolean43_50.convertBoolean(src.getContributedToDeathElement())); 196 if (src.hasOnset()) 197 tgt.setOnset(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOnset())); 198 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 199 return tgt; 200 } 201 202 public static org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent convertFamilyMemberHistoryConditionComponent(org.hl7.fhir.r5.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent src) throws FHIRException { 203 if (src == null) 204 return null; 205 org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent tgt = new org.hl7.fhir.r4b.model.FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 206 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 207 if (src.hasCode()) 208 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 209 if (src.hasOutcome()) 210 tgt.setOutcome(CodeableConcept43_50.convertCodeableConcept(src.getOutcome())); 211 if (src.hasContributedToDeath()) 212 tgt.setContributedToDeathElement(Boolean43_50.convertBoolean(src.getContributedToDeathElement())); 213 if (src.hasOnset()) 214 tgt.setOnset(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOnset())); 215 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 216 return tgt; 217 } 218}