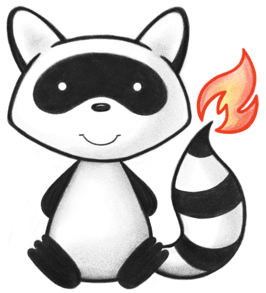
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableReference; 015import org.hl7.fhir.r5.model.Enumeration; 016import org.hl7.fhir.r5.model.Immunization; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class Immunization43_50 { 048 049 public static org.hl7.fhir.r5.model.Immunization convertImmunization(org.hl7.fhir.r4b.model.Immunization src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.Immunization tgt = new org.hl7.fhir.r5.model.Immunization(); 053 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertImmunizationStatus(src.getStatusElement())); 058 if (src.hasStatusReason()) 059 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 060 if (src.hasVaccineCode()) 061 tgt.setVaccineCode(CodeableConcept43_50.convertCodeableConcept(src.getVaccineCode())); 062 if (src.hasPatient()) 063 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 064 if (src.hasEncounter()) 065 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 066 if (src.hasOccurrence()) 067 tgt.setOccurrence(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOccurrence())); 068// if (src.hasRecorded()) 069// tgt.setRecordedElement(DateTime43_50.convertDateTime(src.getRecordedElement())); 070 if (src.hasPrimarySource()) 071 tgt.setPrimarySourceElement(Boolean43_50.convertBoolean(src.getPrimarySourceElement())); 072 if (src.hasReportOrigin()) 073 tgt.setInformationSource(new CodeableReference(CodeableConcept43_50.convertCodeableConcept(src.getReportOrigin()))); 074 if (src.hasLocation()) 075 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 076 if (src.hasManufacturer()) 077 tgt.setManufacturer(Reference43_50.convertReferenceToCodeableReference(src.getManufacturer())); 078 if (src.hasLotNumber()) 079 tgt.setLotNumberElement(String43_50.convertString(src.getLotNumberElement())); 080 if (src.hasExpirationDate()) 081 tgt.setExpirationDateElement(Date43_50.convertDate(src.getExpirationDateElement())); 082 if (src.hasSite()) 083 tgt.setSite(CodeableConcept43_50.convertCodeableConcept(src.getSite())); 084 if (src.hasRoute()) 085 tgt.setRoute(CodeableConcept43_50.convertCodeableConcept(src.getRoute())); 086 if (src.hasDoseQuantity()) 087 tgt.setDoseQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getDoseQuantity())); 088 for (org.hl7.fhir.r4b.model.Immunization.ImmunizationPerformerComponent t : src.getPerformer()) 089 tgt.addPerformer(convertImmunizationPerformerComponent(t)); 090 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 091 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 092 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 093 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 094 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 095 if (src.hasIsSubpotent()) 096 tgt.setIsSubpotentElement(Boolean43_50.convertBoolean(src.getIsSubpotentElement())); 097 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getSubpotentReason()) 098 tgt.addSubpotentReason(CodeableConcept43_50.convertCodeableConcept(t)); 099// for (org.hl7.fhir.r4b.model.Immunization.ImmunizationEducationComponent t : src.getEducation()) 100// tgt.addEducation(convertImmunizationEducationComponent(t)); 101// for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getProgramEligibility()) 102// tgt.addProgramEligibility(CodeableConcept43_50.convertCodeableConcept(t)); 103 if (src.hasFundingSource()) 104 tgt.setFundingSource(CodeableConcept43_50.convertCodeableConcept(src.getFundingSource())); 105 for (org.hl7.fhir.r4b.model.Immunization.ImmunizationReactionComponent t : src.getReaction()) 106 tgt.addReaction(convertImmunizationReactionComponent(t)); 107 for (org.hl7.fhir.r4b.model.Immunization.ImmunizationProtocolAppliedComponent t : src.getProtocolApplied()) 108 tgt.addProtocolApplied(convertImmunizationProtocolAppliedComponent(t)); 109 return tgt; 110 } 111 112 public static org.hl7.fhir.r4b.model.Immunization convertImmunization(org.hl7.fhir.r5.model.Immunization src) throws FHIRException { 113 if (src == null) 114 return null; 115 org.hl7.fhir.r4b.model.Immunization tgt = new org.hl7.fhir.r4b.model.Immunization(); 116 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 117 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 118 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 119 if (src.hasStatus()) 120 tgt.setStatusElement(convertImmunizationStatus(src.getStatusElement())); 121 if (src.hasStatusReason()) 122 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 123 if (src.hasVaccineCode()) 124 tgt.setVaccineCode(CodeableConcept43_50.convertCodeableConcept(src.getVaccineCode())); 125 if (src.hasPatient()) 126 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 127 if (src.hasEncounter()) 128 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 129 if (src.hasOccurrence()) 130 tgt.setOccurrence(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOccurrence())); 131// if (src.hasRecorded()) 132// tgt.setRecordedElement(DateTime43_50.convertDateTime(src.getRecordedElement())); 133 if (src.hasPrimarySource()) 134 tgt.setPrimarySourceElement(Boolean43_50.convertBoolean(src.getPrimarySourceElement())); 135 if (src.getInformationSource().hasConcept()) 136 tgt.setReportOrigin(CodeableConcept43_50.convertCodeableConcept(src.getInformationSource().getConcept())); 137 if (src.hasLocation()) 138 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 139 if (src.hasManufacturer()) 140 tgt.setManufacturer(Reference43_50.convertCodeableReferenceToReference(src.getManufacturer())); 141 if (src.hasLotNumber()) 142 tgt.setLotNumberElement(String43_50.convertString(src.getLotNumberElement())); 143 if (src.hasExpirationDate()) 144 tgt.setExpirationDateElement(Date43_50.convertDate(src.getExpirationDateElement())); 145 if (src.hasSite()) 146 tgt.setSite(CodeableConcept43_50.convertCodeableConcept(src.getSite())); 147 if (src.hasRoute()) 148 tgt.setRoute(CodeableConcept43_50.convertCodeableConcept(src.getRoute())); 149 if (src.hasDoseQuantity()) 150 tgt.setDoseQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getDoseQuantity())); 151 for (org.hl7.fhir.r5.model.Immunization.ImmunizationPerformerComponent t : src.getPerformer()) 152 tgt.addPerformer(convertImmunizationPerformerComponent(t)); 153 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 154 for (CodeableReference t : src.getReason()) 155 if (t.hasConcept()) 156 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 157 for (CodeableReference t : src.getReason()) 158 if (t.hasReference()) 159 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 160 if (src.hasIsSubpotent()) 161 tgt.setIsSubpotentElement(Boolean43_50.convertBoolean(src.getIsSubpotentElement())); 162 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSubpotentReason()) 163 tgt.addSubpotentReason(CodeableConcept43_50.convertCodeableConcept(t)); 164// for (org.hl7.fhir.r5.model.Immunization.ImmunizationEducationComponent t : src.getEducation()) 165// tgt.addEducation(convertImmunizationEducationComponent(t)); 166// for (org.hl7.fhir.r5.model.CodeableConcept t : src.getProgramEligibility()) 167// tgt.addProgramEligibility(CodeableConcept43_50.convertCodeableConcept(t)); 168 if (src.hasFundingSource()) 169 tgt.setFundingSource(CodeableConcept43_50.convertCodeableConcept(src.getFundingSource())); 170 for (org.hl7.fhir.r5.model.Immunization.ImmunizationReactionComponent t : src.getReaction()) 171 tgt.addReaction(convertImmunizationReactionComponent(t)); 172 for (org.hl7.fhir.r5.model.Immunization.ImmunizationProtocolAppliedComponent t : src.getProtocolApplied()) 173 tgt.addProtocolApplied(convertImmunizationProtocolAppliedComponent(t)); 174 return tgt; 175 } 176 177 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Immunization.ImmunizationStatusCodes> convertImmunizationStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes> src) throws FHIRException { 178 if (src == null || src.isEmpty()) 179 return null; 180 Enumeration<Immunization.ImmunizationStatusCodes> tgt = new Enumeration<>(new Immunization.ImmunizationStatusCodesEnumFactory()); 181 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 182 if (src.getValue() == null) { 183 tgt.setValue(null); 184 } else { 185 switch (src.getValue()) { 186 case COMPLETED: 187 tgt.setValue(Immunization.ImmunizationStatusCodes.COMPLETED); 188 break; 189 case ENTEREDINERROR: 190 tgt.setValue(Immunization.ImmunizationStatusCodes.ENTEREDINERROR); 191 break; 192 case NOTDONE: 193 tgt.setValue(Immunization.ImmunizationStatusCodes.NOTDONE); 194 break; 195 default: 196 tgt.setValue(Immunization.ImmunizationStatusCodes.NULL); 197 break; 198 } 199 } 200 return tgt; 201 } 202 203 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes> convertImmunizationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Immunization.ImmunizationStatusCodes> src) throws FHIRException { 204 if (src == null || src.isEmpty()) 205 return null; 206 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodesEnumFactory()); 207 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 208 if (src.getValue() == null) { 209 tgt.setValue(null); 210 } else { 211 switch (src.getValue()) { 212 case COMPLETED: 213 tgt.setValue(org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes.COMPLETED); 214 break; 215 case ENTEREDINERROR: 216 tgt.setValue(org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes.ENTEREDINERROR); 217 break; 218 case NOTDONE: 219 tgt.setValue(org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes.NOTDONE); 220 break; 221 default: 222 tgt.setValue(org.hl7.fhir.r4b.model.Immunization.ImmunizationStatusCodes.NULL); 223 break; 224 } 225 } 226 return tgt; 227 } 228 229 public static org.hl7.fhir.r5.model.Immunization.ImmunizationPerformerComponent convertImmunizationPerformerComponent(org.hl7.fhir.r4b.model.Immunization.ImmunizationPerformerComponent src) throws FHIRException { 230 if (src == null) 231 return null; 232 org.hl7.fhir.r5.model.Immunization.ImmunizationPerformerComponent tgt = new org.hl7.fhir.r5.model.Immunization.ImmunizationPerformerComponent(); 233 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 234 if (src.hasFunction()) 235 tgt.setFunction(CodeableConcept43_50.convertCodeableConcept(src.getFunction())); 236 if (src.hasActor()) 237 tgt.setActor(Reference43_50.convertReference(src.getActor())); 238 return tgt; 239 } 240 241 public static org.hl7.fhir.r4b.model.Immunization.ImmunizationPerformerComponent convertImmunizationPerformerComponent(org.hl7.fhir.r5.model.Immunization.ImmunizationPerformerComponent src) throws FHIRException { 242 if (src == null) 243 return null; 244 org.hl7.fhir.r4b.model.Immunization.ImmunizationPerformerComponent tgt = new org.hl7.fhir.r4b.model.Immunization.ImmunizationPerformerComponent(); 245 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 246 if (src.hasFunction()) 247 tgt.setFunction(CodeableConcept43_50.convertCodeableConcept(src.getFunction())); 248 if (src.hasActor()) 249 tgt.setActor(Reference43_50.convertReference(src.getActor())); 250 return tgt; 251 } 252 253// public static org.hl7.fhir.r5.model.Immunization.ImmunizationEducationComponent convertImmunizationEducationComponent(org.hl7.fhir.r4b.model.Immunization.ImmunizationEducationComponent src) throws FHIRException { 254// if (src == null) 255// return null; 256// org.hl7.fhir.r5.model.Immunization.ImmunizationEducationComponent tgt = new org.hl7.fhir.r5.model.Immunization.ImmunizationEducationComponent(); 257// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 258// if (src.hasDocumentType()) 259// tgt.setDocumentTypeElement(String43_50.convertString(src.getDocumentTypeElement())); 260// if (src.hasReference()) 261// tgt.setReferenceElement(Uri43_50.convertUri(src.getReferenceElement())); 262// if (src.hasPublicationDate()) 263// tgt.setPublicationDateElement(DateTime43_50.convertDateTime(src.getPublicationDateElement())); 264// if (src.hasPresentationDate()) 265// tgt.setPresentationDateElement(DateTime43_50.convertDateTime(src.getPresentationDateElement())); 266// return tgt; 267// } 268// 269// public static org.hl7.fhir.r4b.model.Immunization.ImmunizationEducationComponent convertImmunizationEducationComponent(org.hl7.fhir.r5.model.Immunization.ImmunizationEducationComponent src) throws FHIRException { 270// if (src == null) 271// return null; 272// org.hl7.fhir.r4b.model.Immunization.ImmunizationEducationComponent tgt = new org.hl7.fhir.r4b.model.Immunization.ImmunizationEducationComponent(); 273// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 274// if (src.hasDocumentType()) 275// tgt.setDocumentTypeElement(String43_50.convertString(src.getDocumentTypeElement())); 276// if (src.hasReference()) 277// tgt.setReferenceElement(Uri43_50.convertUri(src.getReferenceElement())); 278// if (src.hasPublicationDate()) 279// tgt.setPublicationDateElement(DateTime43_50.convertDateTime(src.getPublicationDateElement())); 280// if (src.hasPresentationDate()) 281// tgt.setPresentationDateElement(DateTime43_50.convertDateTime(src.getPresentationDateElement())); 282// return tgt; 283// } 284 285 public static org.hl7.fhir.r5.model.Immunization.ImmunizationReactionComponent convertImmunizationReactionComponent(org.hl7.fhir.r4b.model.Immunization.ImmunizationReactionComponent src) throws FHIRException { 286 if (src == null) 287 return null; 288 org.hl7.fhir.r5.model.Immunization.ImmunizationReactionComponent tgt = new org.hl7.fhir.r5.model.Immunization.ImmunizationReactionComponent(); 289 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 290 if (src.hasDate()) 291 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 292 if (src.hasDetail()) 293 tgt.setManifestation(new CodeableReference(Reference43_50.convertReference(src.getDetail()))); 294 if (src.hasReported()) 295 tgt.setReportedElement(Boolean43_50.convertBoolean(src.getReportedElement())); 296 return tgt; 297 } 298 299 public static org.hl7.fhir.r4b.model.Immunization.ImmunizationReactionComponent convertImmunizationReactionComponent(org.hl7.fhir.r5.model.Immunization.ImmunizationReactionComponent src) throws FHIRException { 300 if (src == null) 301 return null; 302 org.hl7.fhir.r4b.model.Immunization.ImmunizationReactionComponent tgt = new org.hl7.fhir.r4b.model.Immunization.ImmunizationReactionComponent(); 303 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 304 if (src.hasDate()) 305 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 306 if (src.hasManifestation()) 307 tgt.setDetail(Reference43_50.convertReference(src.getManifestation().getReference())); 308 if (src.hasReported()) 309 tgt.setReportedElement(Boolean43_50.convertBoolean(src.getReportedElement())); 310 return tgt; 311 } 312 313 public static org.hl7.fhir.r5.model.Immunization.ImmunizationProtocolAppliedComponent convertImmunizationProtocolAppliedComponent(org.hl7.fhir.r4b.model.Immunization.ImmunizationProtocolAppliedComponent src) throws FHIRException { 314 if (src == null) 315 return null; 316 org.hl7.fhir.r5.model.Immunization.ImmunizationProtocolAppliedComponent tgt = new org.hl7.fhir.r5.model.Immunization.ImmunizationProtocolAppliedComponent(); 317 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 318 if (src.hasSeries()) 319 tgt.setSeriesElement(String43_50.convertString(src.getSeriesElement())); 320 if (src.hasAuthority()) 321 tgt.setAuthority(Reference43_50.convertReference(src.getAuthority())); 322 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getTargetDisease()) 323 tgt.addTargetDisease(CodeableConcept43_50.convertCodeableConcept(t)); 324 if (src.hasDoseNumber()) 325 tgt.setDoseNumber(src.getDoseNumber().primitiveValue()); 326 if (src.hasSeriesDoses()) 327 tgt.setSeriesDoses(src.getSeriesDoses().primitiveValue()); 328 return tgt; 329 } 330 331 public static org.hl7.fhir.r4b.model.Immunization.ImmunizationProtocolAppliedComponent convertImmunizationProtocolAppliedComponent(org.hl7.fhir.r5.model.Immunization.ImmunizationProtocolAppliedComponent src) throws FHIRException { 332 if (src == null) 333 return null; 334 org.hl7.fhir.r4b.model.Immunization.ImmunizationProtocolAppliedComponent tgt = new org.hl7.fhir.r4b.model.Immunization.ImmunizationProtocolAppliedComponent(); 335 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 336 if (src.hasSeries()) 337 tgt.setSeriesElement(String43_50.convertString(src.getSeriesElement())); 338 if (src.hasAuthority()) 339 tgt.setAuthority(Reference43_50.convertReference(src.getAuthority())); 340 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getTargetDisease()) 341 tgt.addTargetDisease(CodeableConcept43_50.convertCodeableConcept(t)); 342 if (src.hasDoseNumber()) 343 tgt.setDoseNumber(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDoseNumberElement())); 344 if (src.hasSeriesDoses()) 345 tgt.setSeriesDoses(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSeriesDosesElement())); 346 return tgt; 347 } 348}