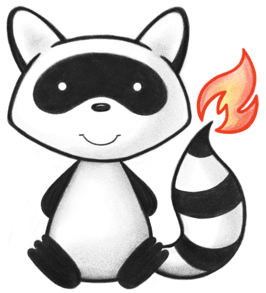
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.conv43_50.datatypes43_50.BackboneElement43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class MarketingStatus43_50 extends BackboneElement43_50 { 010 public static org.hl7.fhir.r5.model.MarketingStatus convertMarketingStatus(org.hl7.fhir.r4b.model.MarketingStatus src) throws FHIRException { 011 if (src == null) return null; 012 org.hl7.fhir.r5.model.MarketingStatus tgt = new org.hl7.fhir.r5.model.MarketingStatus(); 013 copyBackboneElement(src, tgt); 014 if (src.hasCountry()) tgt.setCountry(CodeableConcept43_50.convertCodeableConcept(src.getCountry())); 015 if (src.hasJurisdiction()) tgt.setJurisdiction(CodeableConcept43_50.convertCodeableConcept(src.getJurisdiction())); 016 if (src.hasStatus()) tgt.setStatus(CodeableConcept43_50.convertCodeableConcept(src.getStatus())); 017 if (src.hasDateRange()) tgt.setDateRange(Period43_50.convertPeriod(src.getDateRange())); 018 if (src.hasRestoreDate()) tgt.setRestoreDateElement(DateTime43_50.convertDateTime(src.getRestoreDateElement())); 019 return tgt; 020 } 021 022 public static org.hl7.fhir.r4b.model.MarketingStatus convertMarketingStatus(org.hl7.fhir.r5.model.MarketingStatus src) throws FHIRException { 023 if (src == null) return null; 024 org.hl7.fhir.r4b.model.MarketingStatus tgt = new org.hl7.fhir.r4b.model.MarketingStatus(); 025 copyBackboneElement(src, tgt); 026 if (src.hasCountry()) tgt.setCountry(CodeableConcept43_50.convertCodeableConcept(src.getCountry())); 027 if (src.hasJurisdiction()) tgt.setJurisdiction(CodeableConcept43_50.convertCodeableConcept(src.getJurisdiction())); 028 if (src.hasStatus()) tgt.setStatus(CodeableConcept43_50.convertCodeableConcept(src.getStatus())); 029 if (src.hasDateRange()) tgt.setDateRange(Period43_50.convertPeriod(src.getDateRange())); 030 if (src.hasRestoreDate()) tgt.setRestoreDateElement(DateTime43_50.convertDateTime(src.getRestoreDateElement())); 031 return tgt; 032 } 033}