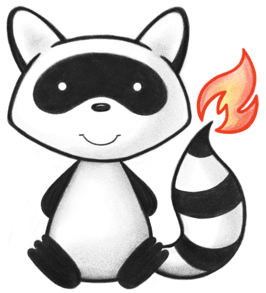
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.Expression43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.RelatedArtifact43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r5.model.Measure.MeasureTermComponent; 020 021/* 022 Copyright (c) 2011+, HL7, Inc. 023 All rights reserved. 024 025 Redistribution and use in source and binary forms, with or without modification, 026 are permitted provided that the following conditions are met: 027 028 * Redistributions of source code must retain the above copyright notice, this 029 list of conditions and the following disclaimer. 030 * Redistributions in binary form must reproduce the above copyright notice, 031 this list of conditions and the following disclaimer in the documentation 032 and/or other materials provided with the distribution. 033 * Neither the name of HL7 nor the names of its contributors may be used to 034 endorse or promote products derived from this software without specific 035 prior written permission. 036 037 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 038 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 039 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 040 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 041 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 042 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 043 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 044 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 045 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 046 POSSIBILITY OF SUCH DAMAGE. 047 048*/ 049// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 050public class Measure43_50 { 051 052 public static org.hl7.fhir.r5.model.Measure convertMeasure(org.hl7.fhir.r4b.model.Measure src) throws FHIRException { 053 if (src == null) 054 return null; 055 org.hl7.fhir.r5.model.Measure tgt = new org.hl7.fhir.r5.model.Measure(); 056 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 057 if (src.hasUrl()) 058 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 059 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 060 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 061 if (src.hasVersion()) 062 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 063 if (src.hasName()) 064 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 065 if (src.hasTitle()) 066 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 067 if (src.hasSubtitle()) 068 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 069 if (src.hasStatus()) 070 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 071 if (src.hasExperimental()) 072 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 073 if (src.hasSubject()) 074 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 075 if (src.hasDate()) 076 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 077 if (src.hasPublisher()) 078 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 079 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 080 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 081 if (src.hasDescription()) 082 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 083 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 084 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 085 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 086 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 087 if (src.hasPurpose()) 088 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 089 if (src.hasUsage()) 090 tgt.setUsageElement(String43_50.convertStringToMarkdown(src.getUsageElement())); 091 if (src.hasCopyright()) 092 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 093 if (src.hasApprovalDate()) 094 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 095 if (src.hasLastReviewDate()) 096 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 097 if (src.hasEffectivePeriod()) 098 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 099 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getTopic()) 100 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 101 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getAuthor()) 102 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 103 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEditor()) 104 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 105 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getReviewer()) 106 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 107 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEndorser()) 108 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 109 for (org.hl7.fhir.r4b.model.RelatedArtifact t : src.getRelatedArtifact()) 110 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 111 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getLibrary()) 112 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 113 if (src.hasDisclaimer()) 114 tgt.setDisclaimerElement(MarkDown43_50.convertMarkdown(src.getDisclaimerElement())); 115 if (src.hasScoring()) 116 tgt.setScoring(CodeableConcept43_50.convertCodeableConcept(src.getScoring())); 117 if (src.hasCompositeScoring()) 118 tgt.setCompositeScoring(CodeableConcept43_50.convertCodeableConcept(src.getCompositeScoring())); 119 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getType()) 120 tgt.addType(CodeableConcept43_50.convertCodeableConcept(t)); 121 if (src.hasRiskAdjustment()) 122 tgt.setRiskAdjustmentElement(String43_50.convertStringToMarkdown(src.getRiskAdjustmentElement())); 123 if (src.hasRateAggregation()) 124 tgt.setRateAggregationElement(String43_50.convertStringToMarkdown(src.getRateAggregationElement())); 125 if (src.hasRationale()) 126 tgt.setRationaleElement(MarkDown43_50.convertMarkdown(src.getRationaleElement())); 127 if (src.hasClinicalRecommendationStatement()) 128 tgt.setClinicalRecommendationStatementElement(MarkDown43_50.convertMarkdown(src.getClinicalRecommendationStatementElement())); 129 if (src.hasImprovementNotation()) 130 tgt.setImprovementNotation(CodeableConcept43_50.convertCodeableConcept(src.getImprovementNotation())); 131 for (org.hl7.fhir.r4b.model.MarkdownType t : src.getDefinition()) 132 tgt.addTerm().setDefinitionElement(MarkDown43_50.convertMarkdown(t)); 133 if (src.hasGuidance()) 134 tgt.setGuidanceElement(MarkDown43_50.convertMarkdown(src.getGuidanceElement())); 135 for (org.hl7.fhir.r4b.model.Measure.MeasureGroupComponent t : src.getGroup()) 136 tgt.addGroup(convertMeasureGroupComponent(t)); 137 for (org.hl7.fhir.r4b.model.Measure.MeasureSupplementalDataComponent t : src.getSupplementalData()) 138 tgt.addSupplementalData(convertMeasureSupplementalDataComponent(t)); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.r4b.model.Measure convertMeasure(org.hl7.fhir.r5.model.Measure src) throws FHIRException { 143 if (src == null) 144 return null; 145 org.hl7.fhir.r4b.model.Measure tgt = new org.hl7.fhir.r4b.model.Measure(); 146 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 147 if (src.hasUrl()) 148 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 149 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 150 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 151 if (src.hasVersion()) 152 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 153 if (src.hasName()) 154 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 155 if (src.hasTitle()) 156 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 157 if (src.hasSubtitle()) 158 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 159 if (src.hasStatus()) 160 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 161 if (src.hasExperimental()) 162 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 163 if (src.hasSubject()) 164 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 165 if (src.hasDate()) 166 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 167 if (src.hasPublisher()) 168 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 169 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 170 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 171 if (src.hasDescription()) 172 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 173 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 174 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 175 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 176 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 177 if (src.hasPurpose()) 178 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 179 if (src.hasUsage()) 180 tgt.setUsageElement(String43_50.convertString(src.getUsageElement())); 181 if (src.hasCopyright()) 182 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 183 if (src.hasApprovalDate()) 184 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 185 if (src.hasLastReviewDate()) 186 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 187 if (src.hasEffectivePeriod()) 188 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 189 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getTopic()) 190 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 191 for (org.hl7.fhir.r5.model.ContactDetail t : src.getAuthor()) 192 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 193 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEditor()) 194 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 195 for (org.hl7.fhir.r5.model.ContactDetail t : src.getReviewer()) 196 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 197 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEndorser()) 198 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 199 for (org.hl7.fhir.r5.model.RelatedArtifact t : src.getRelatedArtifact()) 200 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 201 for (org.hl7.fhir.r5.model.CanonicalType t : src.getLibrary()) 202 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 203 if (src.hasDisclaimer()) 204 tgt.setDisclaimerElement(MarkDown43_50.convertMarkdown(src.getDisclaimerElement())); 205 if (src.hasScoring()) 206 tgt.setScoring(CodeableConcept43_50.convertCodeableConcept(src.getScoring())); 207 if (src.hasCompositeScoring()) 208 tgt.setCompositeScoring(CodeableConcept43_50.convertCodeableConcept(src.getCompositeScoring())); 209 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 210 tgt.addType(CodeableConcept43_50.convertCodeableConcept(t)); 211 if (src.hasRiskAdjustment()) 212 tgt.setRiskAdjustmentElement(String43_50.convertString(src.getRiskAdjustmentElement())); 213 if (src.hasRateAggregation()) 214 tgt.setRateAggregationElement(String43_50.convertString(src.getRateAggregationElement())); 215 if (src.hasRationale()) 216 tgt.setRationaleElement(MarkDown43_50.convertMarkdown(src.getRationaleElement())); 217 if (src.hasClinicalRecommendationStatement()) 218 tgt.setClinicalRecommendationStatementElement(MarkDown43_50.convertMarkdown(src.getClinicalRecommendationStatementElement())); 219 if (src.hasImprovementNotation()) 220 tgt.setImprovementNotation(CodeableConcept43_50.convertCodeableConcept(src.getImprovementNotation())); 221 for (MeasureTermComponent t : src.getTerm()) 222 tgt.getDefinition().add(MarkDown43_50.convertMarkdown(t.getDefinitionElement())); 223 if (src.hasGuidance()) 224 tgt.setGuidanceElement(MarkDown43_50.convertMarkdown(src.getGuidanceElement())); 225 for (org.hl7.fhir.r5.model.Measure.MeasureGroupComponent t : src.getGroup()) 226 tgt.addGroup(convertMeasureGroupComponent(t)); 227 for (org.hl7.fhir.r5.model.Measure.MeasureSupplementalDataComponent t : src.getSupplementalData()) 228 tgt.addSupplementalData(convertMeasureSupplementalDataComponent(t)); 229 return tgt; 230 } 231 232 public static org.hl7.fhir.r5.model.Measure.MeasureGroupComponent convertMeasureGroupComponent(org.hl7.fhir.r4b.model.Measure.MeasureGroupComponent src) throws FHIRException { 233 if (src == null) 234 return null; 235 org.hl7.fhir.r5.model.Measure.MeasureGroupComponent tgt = new org.hl7.fhir.r5.model.Measure.MeasureGroupComponent(); 236 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 237 if (src.hasCode()) 238 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 239 if (src.hasDescription()) 240 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 241 for (org.hl7.fhir.r4b.model.Measure.MeasureGroupPopulationComponent t : src.getPopulation()) 242 tgt.addPopulation(convertMeasureGroupPopulationComponent(t)); 243 for (org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponent t : src.getStratifier()) 244 tgt.addStratifier(convertMeasureGroupStratifierComponent(t)); 245 return tgt; 246 } 247 248 public static org.hl7.fhir.r4b.model.Measure.MeasureGroupComponent convertMeasureGroupComponent(org.hl7.fhir.r5.model.Measure.MeasureGroupComponent src) throws FHIRException { 249 if (src == null) 250 return null; 251 org.hl7.fhir.r4b.model.Measure.MeasureGroupComponent tgt = new org.hl7.fhir.r4b.model.Measure.MeasureGroupComponent(); 252 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 253 if (src.hasCode()) 254 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 255 if (src.hasDescription()) 256 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 257 for (org.hl7.fhir.r5.model.Measure.MeasureGroupPopulationComponent t : src.getPopulation()) 258 tgt.addPopulation(convertMeasureGroupPopulationComponent(t)); 259 for (org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponent t : src.getStratifier()) 260 tgt.addStratifier(convertMeasureGroupStratifierComponent(t)); 261 return tgt; 262 } 263 264 public static org.hl7.fhir.r5.model.Measure.MeasureGroupPopulationComponent convertMeasureGroupPopulationComponent(org.hl7.fhir.r4b.model.Measure.MeasureGroupPopulationComponent src) throws FHIRException { 265 if (src == null) 266 return null; 267 org.hl7.fhir.r5.model.Measure.MeasureGroupPopulationComponent tgt = new org.hl7.fhir.r5.model.Measure.MeasureGroupPopulationComponent(); 268 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 269 if (src.hasCode()) 270 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 271 if (src.hasDescription()) 272 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 273 if (src.hasCriteria()) 274 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.r4b.model.Measure.MeasureGroupPopulationComponent convertMeasureGroupPopulationComponent(org.hl7.fhir.r5.model.Measure.MeasureGroupPopulationComponent src) throws FHIRException { 279 if (src == null) 280 return null; 281 org.hl7.fhir.r4b.model.Measure.MeasureGroupPopulationComponent tgt = new org.hl7.fhir.r4b.model.Measure.MeasureGroupPopulationComponent(); 282 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 283 if (src.hasCode()) 284 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 285 if (src.hasDescription()) 286 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 287 if (src.hasCriteria()) 288 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 289 return tgt; 290 } 291 292 public static org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponent convertMeasureGroupStratifierComponent(org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponent src) throws FHIRException { 293 if (src == null) 294 return null; 295 org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponent tgt = new org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponent(); 296 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 297 if (src.hasCode()) 298 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 299 if (src.hasDescription()) 300 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 301 if (src.hasCriteria()) 302 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 303 for (org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponentComponent t : src.getComponent()) 304 tgt.addComponent(convertMeasureGroupStratifierComponentComponent(t)); 305 return tgt; 306 } 307 308 public static org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponent convertMeasureGroupStratifierComponent(org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponent src) throws FHIRException { 309 if (src == null) 310 return null; 311 org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponent tgt = new org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponent(); 312 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 313 if (src.hasCode()) 314 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 315 if (src.hasDescription()) 316 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 317 if (src.hasCriteria()) 318 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 319 for (org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponentComponent t : src.getComponent()) 320 tgt.addComponent(convertMeasureGroupStratifierComponentComponent(t)); 321 return tgt; 322 } 323 324 public static org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponentComponent convertMeasureGroupStratifierComponentComponent(org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponentComponent src) throws FHIRException { 325 if (src == null) 326 return null; 327 org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponentComponent tgt = new org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponentComponent(); 328 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 329 if (src.hasCode()) 330 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 331 if (src.hasDescription()) 332 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 333 if (src.hasCriteria()) 334 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 335 return tgt; 336 } 337 338 public static org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponentComponent convertMeasureGroupStratifierComponentComponent(org.hl7.fhir.r5.model.Measure.MeasureGroupStratifierComponentComponent src) throws FHIRException { 339 if (src == null) 340 return null; 341 org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponentComponent tgt = new org.hl7.fhir.r4b.model.Measure.MeasureGroupStratifierComponentComponent(); 342 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 343 if (src.hasCode()) 344 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 345 if (src.hasDescription()) 346 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 347 if (src.hasCriteria()) 348 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 349 return tgt; 350 } 351 352 public static org.hl7.fhir.r5.model.Measure.MeasureSupplementalDataComponent convertMeasureSupplementalDataComponent(org.hl7.fhir.r4b.model.Measure.MeasureSupplementalDataComponent src) throws FHIRException { 353 if (src == null) 354 return null; 355 org.hl7.fhir.r5.model.Measure.MeasureSupplementalDataComponent tgt = new org.hl7.fhir.r5.model.Measure.MeasureSupplementalDataComponent(); 356 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 357 if (src.hasCode()) 358 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 359 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getUsage()) 360 tgt.addUsage(CodeableConcept43_50.convertCodeableConcept(t)); 361 if (src.hasDescription()) 362 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 363 if (src.hasCriteria()) 364 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 365 return tgt; 366 } 367 368 public static org.hl7.fhir.r4b.model.Measure.MeasureSupplementalDataComponent convertMeasureSupplementalDataComponent(org.hl7.fhir.r5.model.Measure.MeasureSupplementalDataComponent src) throws FHIRException { 369 if (src == null) 370 return null; 371 org.hl7.fhir.r4b.model.Measure.MeasureSupplementalDataComponent tgt = new org.hl7.fhir.r4b.model.Measure.MeasureSupplementalDataComponent(); 372 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 373 if (src.hasCode()) 374 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 375 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getUsage()) 376 tgt.addUsage(CodeableConcept43_50.convertCodeableConcept(t)); 377 if (src.hasDescription()) 378 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 379 if (src.hasCriteria()) 380 tgt.setCriteria(Expression43_50.convertExpression(src.getCriteria())); 381 return tgt; 382 } 383}