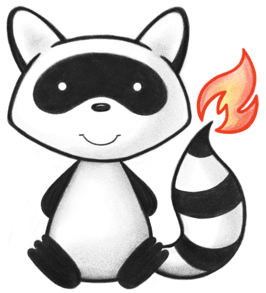
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.exceptions.FHIRException; 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 035public class Media43_50 { 036 037 public static org.hl7.fhir.r5.model.DocumentReference convertMedia(org.hl7.fhir.r4b.model.Media src) throws FHIRException { 038 if (src == null) 039 return null; 040 org.hl7.fhir.r5.model.DocumentReference tgt = new org.hl7.fhir.r5.model.DocumentReference(); 041 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 042 // for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 043 // tgt.addIdentifier(convertIdentifier(t)); 044 // for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) 045 // tgt.addBasedOn(convertReference(t)); 046 // for (org.hl7.fhir.r4b.model.Reference t : src.getPartOf()) 047 // tgt.addPartOf(convertReference(t)); 048 // if (src.hasStatus()) 049 // tgt.setStatus(convertMediaStatus(src.getStatus())); 050 // if (src.hasType()) 051 // tgt.setType(convertCodeableConcept(src.getType())); 052 // if (src.hasModality()) 053 // tgt.setModality(convertCodeableConcept(src.getModality())); 054 // if (src.hasView()) 055 // tgt.setView(convertCodeableConcept(src.getView())); 056 // if (src.hasSubject()) 057 // tgt.setSubject(convertReference(src.getSubject())); 058 // if (src.hasEncounter()) 059 // tgt.setEncounter(convertReference(src.getEncounter())); 060 // if (src.hasCreated()) 061 // tgt.setCreated(convertType(src.getCreated())); 062 // if (src.hasIssued()) 063 // tgt.setIssuedElement(convertInstant(src.getIssuedElement())); 064 // if (src.hasOperator()) 065 // tgt.setOperator(convertReference(src.getOperator())); 066 // for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 067 // tgt.addReasonCode(convertCodeableConcept(t)); 068 // if (src.hasBodySite()) 069 // tgt.setBodySite(convertCodeableConcept(src.getBodySite())); 070 // if (src.hasDeviceName()) 071 // tgt.setDeviceNameElement(convertString(src.getDeviceNameElement())); 072 // if (src.hasDevice()) 073 // tgt.setDevice(convertReference(src.getDevice())); 074 // if (src.hasHeight()) 075 // tgt.setHeightElement(convertPositiveInt(src.getHeightElement())); 076 // if (src.hasWidth()) 077 // tgt.setWidthElement(convertPositiveInt(src.getWidthElement())); 078 // if (src.hasFrames()) 079 // tgt.setFramesElement(convertPositiveInt(src.getFramesElement())); 080 // if (src.hasDuration()) 081 // tgt.setDurationElement(convertDecimal(src.getDurationElement())); 082 // if (src.hasContent()) 083 // tgt.setContent(convertAttachment(src.getContent())); 084 // for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) 085 // tgt.addNote(convertAnnotation(t)); 086 return tgt; 087 } 088 089 /** 090 * Todo: how do you decide that it maps to a media? 091 * 092 * @param src 093 * @return 094 * @throws FHIRException 095 */ 096 public static org.hl7.fhir.r4b.model.Media convertMedia(org.hl7.fhir.r5.model.DocumentReference src) throws FHIRException { 097 if (src == null) 098 return null; 099 org.hl7.fhir.r4b.model.Media tgt = new org.hl7.fhir.r4b.model.Media(); 100 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 101 // for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 102 // tgt.addIdentifier(convertIdentifier(t)); 103 // for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) 104 // tgt.addBasedOn(convertReference(t)); 105 // for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) 106 // tgt.addPartOf(convertReference(t)); 107 // if (src.hasStatus()) 108 // tgt.setStatus(convertMediaStatus(src.getStatus())); 109 // if (src.hasType()) 110 // tgt.setType(convertCodeableConcept(src.getType())); 111 // if (src.hasModality()) 112 // tgt.setModality(convertCodeableConcept(src.getModality())); 113 // if (src.hasView()) 114 // tgt.setView(convertCodeableConcept(src.getView())); 115 // if (src.hasSubject()) 116 // tgt.setSubject(convertReference(src.getSubject())); 117 // if (src.hasEncounter()) 118 // tgt.setEncounter(convertReference(src.getEncounter())); 119 // if (src.hasCreated()) 120 // tgt.setCreated(convertType(src.getCreated())); 121 // if (src.hasIssued()) 122 // tgt.setIssuedElement(convertInstant(src.getIssuedElement())); 123 // if (src.hasOperator()) 124 // tgt.setOperator(convertReference(src.getOperator())); 125 // for (org.hl7.fhir.r5.model.CodeableConcept t : src.getReasonCode()) 126 // tgt.addReasonCode(convertCodeableConcept(t)); 127 // if (src.hasBodySite()) 128 // tgt.setBodySite(convertCodeableConcept(src.getBodySite())); 129 // if (src.hasDeviceName()) 130 // tgt.setDeviceNameElement(convertString(src.getDeviceNameElement())); 131 // if (src.hasDevice()) 132 // tgt.setDevice(convertReference(src.getDevice())); 133 // if (src.hasHeight()) 134 // tgt.setHeightElement(convertPositiveInt(src.getHeightElement())); 135 // if (src.hasWidth()) 136 // tgt.setWidthElement(convertPositiveInt(src.getWidthElement())); 137 // if (src.hasFrames()) 138 // tgt.setFramesElement(convertPositiveInt(src.getFramesElement())); 139 // if (src.hasDuration()) 140 // tgt.setDurationElement(convertDecimal(src.getDurationElement())); 141 // if (src.hasContent()) 142 // tgt.setContent(convertAttachment(src.getContent())); 143 // for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) 144 // tgt.addNote(convertAnnotation(t)); 145 return tgt; 146 } 147 // public static org.hl7.fhir.r5.model.Media.MediaStatus convertMediaStatus(org.hl7.fhir.r4b.model.Media.MediaStatus src) throws FHIRException { 148 // if (src == null) 149 // return null; 150 // switch (src) { 151 // case PREPARATION: return org.hl7.fhir.r5.model.Media.MediaStatus.PREPARATION; 152 // case INPROGRESS: return org.hl7.fhir.r5.model.Media.MediaStatus.INPROGRESS; 153 // case NOTDONE: return org.hl7.fhir.r5.model.Media.MediaStatus.NOTDONE; 154 // case ONHOLD: return org.hl7.fhir.r5.model.Media.MediaStatus.ONHOLD; 155 // case STOPPED: return org.hl7.fhir.r5.model.Media.MediaStatus.STOPPED; 156 // case COMPLETED: return org.hl7.fhir.r5.model.Media.MediaStatus.COMPLETED; 157 // case ENTEREDINERROR: return org.hl7.fhir.r5.model.Media.MediaStatus.ENTEREDINERROR; 158 // case UNKNOWN: return org.hl7.fhir.r5.model.Media.MediaStatus.UNKNOWN; 159 // default: return org.hl7.fhir.r5.model.Media.MediaStatus.NULL; 160 // } 161 // } 162 // 163 // public static org.hl7.fhir.r4b.model.Media.MediaStatus convertMediaStatus(org.hl7.fhir.r5.model.Media.MediaStatus src) throws FHIRException { 164 // if (src == null) 165 // return null; 166 // switch (src) { 167 // case PREPARATION: return org.hl7.fhir.r4b.model.Media.MediaStatus.PREPARATION; 168 // case INPROGRESS: return org.hl7.fhir.r4b.model.Media.MediaStatus.INPROGRESS; 169 // case NOTDONE: return org.hl7.fhir.r4b.model.Media.MediaStatus.NOTDONE; 170 // case ONHOLD: return org.hl7.fhir.r4b.model.Media.MediaStatus.ONHOLD; 171 // case STOPPED: return org.hl7.fhir.r4b.model.Media.MediaStatus.STOPPED; 172 // case COMPLETED: return org.hl7.fhir.r4b.model.Media.MediaStatus.COMPLETED; 173 // case ENTEREDINERROR: return org.hl7.fhir.r4b.model.Media.MediaStatus.ENTEREDINERROR; 174 // case UNKNOWN: return org.hl7.fhir.r4b.model.Media.MediaStatus.UNKNOWN; 175 // default: return org.hl7.fhir.r4b.model.Media.MediaStatus.NULL; 176 // } 177 // } 178}