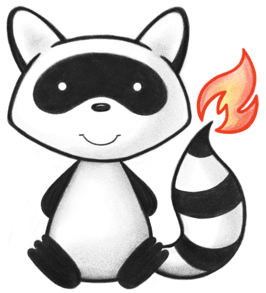
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Duration43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.UnsignedInt43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Dosage43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.Enumerations; 018import org.hl7.fhir.r5.model.MedicationRequest; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class MedicationRequest43_50 { 050 051 public static org.hl7.fhir.r5.model.MedicationRequest convertMedicationRequest(org.hl7.fhir.r4b.model.MedicationRequest src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r5.model.MedicationRequest tgt = new org.hl7.fhir.r5.model.MedicationRequest(); 055 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 058 if (src.hasStatus()) 059 tgt.setStatusElement(convertMedicationRequestStatus(src.getStatusElement())); 060 if (src.hasStatusReason()) 061 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 062 if (src.hasIntent()) 063 tgt.setIntentElement(convertMedicationRequestIntent(src.getIntentElement())); 064 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 065 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 066 if (src.hasPriority()) 067 tgt.setPriorityElement(convertMedicationRequestPriority(src.getPriorityElement())); 068 if (src.hasDoNotPerform()) 069 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 070 if (src.hasReportedBooleanType()) 071 tgt.setReportedElement(Boolean43_50.convertBoolean(src.getReportedBooleanType())); 072 if (src.hasReportedReference()) 073 tgt.addInformationSource(Reference43_50.convertReference(src.getReportedReference())); 074 if (src.hasMedicationCodeableConcept()) 075 tgt.getMedication().setConcept(CodeableConcept43_50.convertCodeableConcept(src.getMedicationCodeableConcept())); 076 if (src.hasMedicationReference()) 077 tgt.getMedication().setReference(Reference43_50.convertReference(src.getMedicationReference())); 078 if (src.hasSubject()) 079 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 080 if (src.hasEncounter()) 081 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 082 for (org.hl7.fhir.r4b.model.Reference t : src.getSupportingInformation()) 083 tgt.addSupportingInformation(Reference43_50.convertReference(t)); 084 if (src.hasAuthoredOn()) 085 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 086 if (src.hasRequester()) 087 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 088 if (src.hasPerformer()) 089 tgt.addPerformer(Reference43_50.convertReference(src.getPerformer())); 090 if (src.hasPerformerType()) 091 tgt.setPerformerType(CodeableConcept43_50.convertCodeableConcept(src.getPerformerType())); 092 if (src.hasRecorder()) 093 tgt.setRecorder(Reference43_50.convertReference(src.getRecorder())); 094 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 095 tgt.addReason().setConcept(CodeableConcept43_50.convertCodeableConcept(t)); 096 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 097 tgt.addReason().setReference(Reference43_50.convertReference(t)); 098// for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 099// tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 100// for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 101// tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 102 for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 103 if (src.hasGroupIdentifier()) 104 tgt.setGroupIdentifier(Identifier43_50.convertIdentifier(src.getGroupIdentifier())); 105 if (src.hasCourseOfTherapyType()) 106 tgt.setCourseOfTherapyType(CodeableConcept43_50.convertCodeableConcept(src.getCourseOfTherapyType())); 107 for (org.hl7.fhir.r4b.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 108 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 109 for (org.hl7.fhir.r4b.model.Dosage t : src.getDosageInstruction()) 110 tgt.addDosageInstruction(Dosage43_50.convertDosage(t)); 111 if (src.hasDispenseRequest()) 112 tgt.setDispenseRequest(convertMedicationRequestDispenseRequestComponent(src.getDispenseRequest())); 113 if (src.hasSubstitution()) 114 tgt.setSubstitution(convertMedicationRequestSubstitutionComponent(src.getSubstitution())); 115 if (src.hasPriorPrescription()) 116 tgt.setPriorPrescription(Reference43_50.convertReference(src.getPriorPrescription())); 117 for (org.hl7.fhir.r4b.model.Reference t : src.getDetectedIssue()) 118// tgt.addDetectedIssue(Reference43_50.convertReference(t)); 119// for (org.hl7.fhir.r4b.model.Reference t : src.getEventHistory()) 120 tgt.addEventHistory(Reference43_50.convertReference(t)); 121 return tgt; 122 } 123 124 public static org.hl7.fhir.r4b.model.MedicationRequest convertMedicationRequest(org.hl7.fhir.r5.model.MedicationRequest src) throws FHIRException { 125 if (src == null) 126 return null; 127 org.hl7.fhir.r4b.model.MedicationRequest tgt = new org.hl7.fhir.r4b.model.MedicationRequest(); 128 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 129 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 130 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 131 if (src.hasStatus()) 132 tgt.setStatusElement(convertMedicationRequestStatus(src.getStatusElement())); 133 if (src.hasStatusReason()) 134 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 135 if (src.hasIntent()) 136 tgt.setIntentElement(convertMedicationRequestIntent(src.getIntentElement())); 137 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 138 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 139 if (src.hasPriority()) 140 tgt.setPriorityElement(convertMedicationRequestPriority(src.getPriorityElement())); 141 if (src.hasDoNotPerform()) 142 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 143 if (src.hasReported()) 144 tgt.setReported(Boolean43_50.convertBoolean(src.getReportedElement())); 145 if (src.hasInformationSource()) 146 tgt.setReported(Reference43_50.convertReference(src.getInformationSourceFirstRep())); 147 if (src.getMedication().hasReference()) 148 tgt.setMedication(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getMedication().getReference())); 149 if (src.getMedication().hasConcept()) 150 tgt.setMedication(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getMedication().getConcept())); 151 if (src.hasSubject()) 152 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 153 if (src.hasEncounter()) 154 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 155 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInformation()) 156 tgt.addSupportingInformation(Reference43_50.convertReference(t)); 157 if (src.hasAuthoredOn()) 158 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 159 if (src.hasRequester()) 160 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 161 if (src.hasPerformer()) 162 tgt.setPerformer(Reference43_50.convertReference(src.getPerformerFirstRep())); 163 if (src.hasPerformerType()) 164 tgt.setPerformerType(CodeableConcept43_50.convertCodeableConcept(src.getPerformerType())); 165 if (src.hasRecorder()) 166 tgt.setRecorder(Reference43_50.convertReference(src.getRecorder())); 167 for (org.hl7.fhir.r5.model.CodeableReference t : src.getReason()) { 168 if (t.hasConcept()) 169 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 170 if (t.hasReference()) 171 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 172 } 173// for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 174// tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 175// for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 176// tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 177 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 178 if (src.hasGroupIdentifier()) 179 tgt.setGroupIdentifier(Identifier43_50.convertIdentifier(src.getGroupIdentifier())); 180 if (src.hasCourseOfTherapyType()) 181 tgt.setCourseOfTherapyType(CodeableConcept43_50.convertCodeableConcept(src.getCourseOfTherapyType())); 182 for (org.hl7.fhir.r5.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 183 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 184 for (org.hl7.fhir.r5.model.Dosage t : src.getDosageInstruction()) 185 tgt.addDosageInstruction(Dosage43_50.convertDosage(t)); 186 if (src.hasDispenseRequest()) 187 tgt.setDispenseRequest(convertMedicationRequestDispenseRequestComponent(src.getDispenseRequest())); 188 if (src.hasSubstitution()) 189 tgt.setSubstitution(convertMedicationRequestSubstitutionComponent(src.getSubstitution())); 190 if (src.hasPriorPrescription()) 191 tgt.setPriorPrescription(Reference43_50.convertReference(src.getPriorPrescription())); 192// for (org.hl7.fhir.r5.model.Reference t : src.getDetectedIssue()) 193// tgt.addDetectedIssue(Reference43_50.convertReference(t)); 194 for (org.hl7.fhir.r5.model.Reference t : src.getEventHistory()) 195 tgt.addEventHistory(Reference43_50.convertReference(t)); 196 return tgt; 197 } 198 199 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationrequestStatus> convertMedicationRequestStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus> src) throws FHIRException { 200 if (src == null || src.isEmpty()) 201 return null; 202 Enumeration<MedicationRequest.MedicationrequestStatus> tgt = new Enumeration<>(new MedicationRequest.MedicationrequestStatusEnumFactory()); 203 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 204 if (src.getValue() == null) { 205 tgt.setValue(null); 206 } else { 207 switch (src.getValue()) { 208 case ACTIVE: 209 tgt.setValue(MedicationRequest.MedicationrequestStatus.ACTIVE); 210 break; 211 case ONHOLD: 212 tgt.setValue(MedicationRequest.MedicationrequestStatus.ONHOLD); 213 break; 214 case CANCELLED: 215 tgt.setValue(MedicationRequest.MedicationrequestStatus.CANCELLED); 216 break; 217 case COMPLETED: 218 tgt.setValue(MedicationRequest.MedicationrequestStatus.COMPLETED); 219 break; 220 case ENTEREDINERROR: 221 tgt.setValue(MedicationRequest.MedicationrequestStatus.ENTEREDINERROR); 222 break; 223 case STOPPED: 224 tgt.setValue(MedicationRequest.MedicationrequestStatus.STOPPED); 225 break; 226 case DRAFT: 227 tgt.setValue(MedicationRequest.MedicationrequestStatus.DRAFT); 228 break; 229 case UNKNOWN: 230 tgt.setValue(MedicationRequest.MedicationrequestStatus.UNKNOWN); 231 break; 232 default: 233 tgt.setValue(MedicationRequest.MedicationrequestStatus.NULL); 234 break; 235 } 236 } 237 return tgt; 238 } 239 240 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus> convertMedicationRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationrequestStatus> src) throws FHIRException { 241 if (src == null || src.isEmpty()) 242 return null; 243 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatusEnumFactory()); 244 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 245 if (src.getValue() == null) { 246 tgt.setValue(null); 247 } else { 248 switch (src.getValue()) { 249 case ACTIVE: 250 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.ACTIVE); 251 break; 252 case ONHOLD: 253 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.ONHOLD); 254 break; 255 case CANCELLED: 256 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.CANCELLED); 257 break; 258 case COMPLETED: 259 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.COMPLETED); 260 break; 261 case ENTEREDINERROR: 262 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.ENTEREDINERROR); 263 break; 264 case STOPPED: 265 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.STOPPED); 266 break; 267 case DRAFT: 268 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.DRAFT); 269 break; 270 case UNKNOWN: 271 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.UNKNOWN); 272 break; 273 default: 274 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationrequestStatus.NULL); 275 break; 276 } 277 } 278 return tgt; 279 } 280 281 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestIntent> convertMedicationRequestIntent(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent> src) throws FHIRException { 282 if (src == null || src.isEmpty()) 283 return null; 284 Enumeration<MedicationRequest.MedicationRequestIntent> tgt = new Enumeration<>(new MedicationRequest.MedicationRequestIntentEnumFactory()); 285 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 286 if (src.getValue() == null) { 287 tgt.setValue(null); 288 } else { 289 switch (src.getValue()) { 290 case PROPOSAL: 291 tgt.setValue(MedicationRequest.MedicationRequestIntent.PROPOSAL); 292 break; 293 case PLAN: 294 tgt.setValue(MedicationRequest.MedicationRequestIntent.PLAN); 295 break; 296 case ORDER: 297 tgt.setValue(MedicationRequest.MedicationRequestIntent.ORDER); 298 break; 299 case ORIGINALORDER: 300 tgt.setValue(MedicationRequest.MedicationRequestIntent.ORIGINALORDER); 301 break; 302 case REFLEXORDER: 303 tgt.setValue(MedicationRequest.MedicationRequestIntent.REFLEXORDER); 304 break; 305 case FILLERORDER: 306 tgt.setValue(MedicationRequest.MedicationRequestIntent.FILLERORDER); 307 break; 308 case INSTANCEORDER: 309 tgt.setValue(MedicationRequest.MedicationRequestIntent.INSTANCEORDER); 310 break; 311 case OPTION: 312 tgt.setValue(MedicationRequest.MedicationRequestIntent.OPTION); 313 break; 314 default: 315 tgt.setValue(MedicationRequest.MedicationRequestIntent.NULL); 316 break; 317 } 318 } 319 return tgt; 320 } 321 322 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent> convertMedicationRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestIntent> src) throws FHIRException { 323 if (src == null || src.isEmpty()) 324 return null; 325 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntentEnumFactory()); 326 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 327 if (src.getValue() == null) { 328 tgt.setValue(null); 329 } else { 330 switch (src.getValue()) { 331 case PROPOSAL: 332 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.PROPOSAL); 333 break; 334 case PLAN: 335 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.PLAN); 336 break; 337 case ORDER: 338 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.ORDER); 339 break; 340 case ORIGINALORDER: 341 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.ORIGINALORDER); 342 break; 343 case REFLEXORDER: 344 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.REFLEXORDER); 345 break; 346 case FILLERORDER: 347 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.FILLERORDER); 348 break; 349 case INSTANCEORDER: 350 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.INSTANCEORDER); 351 break; 352 case OPTION: 353 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.OPTION); 354 break; 355 default: 356 tgt.setValue(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestIntent.NULL); 357 break; 358 } 359 } 360 return tgt; 361 } 362 363 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertMedicationRequestPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 364 if (src == null || src.isEmpty()) 365 return null; 366 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 367 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 368 if (src.getValue() == null) { 369 tgt.setValue(null); 370 } else { 371 switch (src.getValue()) { 372 case ROUTINE: 373 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 374 break; 375 case URGENT: 376 tgt.setValue(Enumerations.RequestPriority.URGENT); 377 break; 378 case ASAP: 379 tgt.setValue(Enumerations.RequestPriority.ASAP); 380 break; 381 case STAT: 382 tgt.setValue(Enumerations.RequestPriority.STAT); 383 break; 384 default: 385 tgt.setValue(Enumerations.RequestPriority.NULL); 386 break; 387 } 388 } 389 return tgt; 390 } 391 392 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertMedicationRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 393 if (src == null || src.isEmpty()) 394 return null; 395 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 396 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 397 if (src.getValue() == null) { 398 tgt.setValue(null); 399 } else { 400 switch (src.getValue()) { 401 case ROUTINE: 402 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 403 break; 404 case URGENT: 405 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 406 break; 407 case ASAP: 408 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 409 break; 410 case STAT: 411 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 412 break; 413 default: 414 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 415 break; 416 } 417 } 418 return tgt; 419 } 420 421 public static org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent convertMedicationRequestDispenseRequestComponent(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestComponent src) throws FHIRException { 422 if (src == null) 423 return null; 424 org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent tgt = new org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent(); 425 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 426 if (src.hasInitialFill()) 427 tgt.setInitialFill(convertMedicationRequestDispenseRequestInitialFillComponent(src.getInitialFill())); 428 if (src.hasDispenseInterval()) 429 tgt.setDispenseInterval(Duration43_50.convertDuration(src.getDispenseInterval())); 430 if (src.hasValidityPeriod()) 431 tgt.setValidityPeriod(Period43_50.convertPeriod(src.getValidityPeriod())); 432 if (src.hasNumberOfRepeatsAllowed()) 433 tgt.setNumberOfRepeatsAllowedElement(UnsignedInt43_50.convertUnsignedInt(src.getNumberOfRepeatsAllowedElement())); 434 if (src.hasQuantity()) 435 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 436 if (src.hasExpectedSupplyDuration()) 437 tgt.setExpectedSupplyDuration(Duration43_50.convertDuration(src.getExpectedSupplyDuration())); 438 if (src.hasPerformer()) 439 tgt.setDispenser(Reference43_50.convertReference(src.getPerformer())); 440 return tgt; 441 } 442 443 public static org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestComponent convertMedicationRequestDispenseRequestComponent(org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent src) throws FHIRException { 444 if (src == null) 445 return null; 446 org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestComponent tgt = new org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestComponent(); 447 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 448 if (src.hasInitialFill()) 449 tgt.setInitialFill(convertMedicationRequestDispenseRequestInitialFillComponent(src.getInitialFill())); 450 if (src.hasDispenseInterval()) 451 tgt.setDispenseInterval(Duration43_50.convertDuration(src.getDispenseInterval())); 452 if (src.hasValidityPeriod()) 453 tgt.setValidityPeriod(Period43_50.convertPeriod(src.getValidityPeriod())); 454 if (src.hasNumberOfRepeatsAllowed()) 455 tgt.setNumberOfRepeatsAllowedElement(UnsignedInt43_50.convertUnsignedInt(src.getNumberOfRepeatsAllowedElement())); 456 if (src.hasQuantity()) 457 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 458 if (src.hasExpectedSupplyDuration()) 459 tgt.setExpectedSupplyDuration(Duration43_50.convertDuration(src.getExpectedSupplyDuration())); 460 if (src.hasDispenser()) 461 tgt.setPerformer(Reference43_50.convertReference(src.getDispenser())); 462 return tgt; 463 } 464 465 public static org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent convertMedicationRequestDispenseRequestInitialFillComponent(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent src) throws FHIRException { 466 if (src == null) 467 return null; 468 org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent tgt = new org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent(); 469 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 470 if (src.hasQuantity()) 471 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 472 if (src.hasDuration()) 473 tgt.setDuration(Duration43_50.convertDuration(src.getDuration())); 474 return tgt; 475 } 476 477 public static org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent convertMedicationRequestDispenseRequestInitialFillComponent(org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent src) throws FHIRException { 478 if (src == null) 479 return null; 480 org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent tgt = new org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestDispenseRequestInitialFillComponent(); 481 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 482 if (src.hasQuantity()) 483 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 484 if (src.hasDuration()) 485 tgt.setDuration(Duration43_50.convertDuration(src.getDuration())); 486 return tgt; 487 } 488 489 public static org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent convertMedicationRequestSubstitutionComponent(org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestSubstitutionComponent src) throws FHIRException { 490 if (src == null) 491 return null; 492 org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent tgt = new org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent(); 493 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 494 if (src.hasAllowed()) 495 tgt.setAllowed(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAllowed())); 496 if (src.hasReason()) 497 tgt.setReason(CodeableConcept43_50.convertCodeableConcept(src.getReason())); 498 return tgt; 499 } 500 501 public static org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestSubstitutionComponent convertMedicationRequestSubstitutionComponent(org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent src) throws FHIRException { 502 if (src == null) 503 return null; 504 org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestSubstitutionComponent tgt = new org.hl7.fhir.r4b.model.MedicationRequest.MedicationRequestSubstitutionComponent(); 505 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 506 if (src.hasAllowed()) 507 tgt.setAllowed(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAllowed())); 508 if (src.hasReason()) 509 tgt.setReason(CodeableConcept43_50.convertCodeableConcept(src.getReason())); 510 return tgt; 511 } 512}