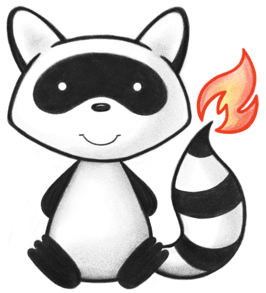
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Dosage43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012 013/* 014 Copyright (c) 2011+, HL7, Inc. 015 All rights reserved. 016 017 Redistribution and use in source and binary forms, with or without modification, 018 are permitted provided that the following conditions are met: 019 020 * Redistributions of source code must retain the above copyright notice, this 021 list of conditions and the following disclaimer. 022 * Redistributions in binary form must reproduce the above copyright notice, 023 this list of conditions and the following disclaimer in the documentation 024 and/or other materials provided with the distribution. 025 * Neither the name of HL7 nor the names of its contributors may be used to 026 endorse or promote products derived from this software without specific 027 prior written permission. 028 029 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 030 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 031 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 032 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 033 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 034 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 035 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 036 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 037 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 038 POSSIBILITY OF SUCH DAMAGE. 039 040*/ 041// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 042public class MedicationStatement43_50 { 043 044 public static org.hl7.fhir.r5.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r4b.model.MedicationStatement src) throws FHIRException { 045 if (src == null) 046 return null; 047 org.hl7.fhir.r5.model.MedicationStatement tgt = new org.hl7.fhir.r5.model.MedicationStatement(); 048 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 049 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 050 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 051// for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 052// for (org.hl7.fhir.r4b.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 055// for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getStatusReason()) 056// tgt.addStatusReason(CodeableConcept43_50.convertCodeableConcept(t)); 057 if (src.hasCategory()) 058 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(src.getCategory())); 059 if (src.hasMedicationCodeableConcept()) { 060 tgt.getMedication().setConcept(CodeableConcept43_50.convertCodeableConcept(src.getMedicationCodeableConcept())); 061 } 062 if (src.hasMedicationReference()) { 063 tgt.getMedication().setReference(Reference43_50.convertReference(src.getMedicationReference())); 064 } 065 if (src.hasSubject()) 066 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 067 if (src.hasContext()) 068 tgt.setEncounter(Reference43_50.convertReference(src.getContext())); 069 if (src.hasEffective()) 070 tgt.setEffective(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEffective())); 071 if (src.hasDateAsserted()) 072 tgt.setDateAssertedElement(DateTime43_50.convertDateTime(src.getDateAssertedElement())); 073 if (src.hasInformationSource()) 074 tgt.addInformationSource(Reference43_50.convertReference(src.getInformationSource())); 075 for (org.hl7.fhir.r4b.model.Reference t : src.getDerivedFrom()) 076 tgt.addDerivedFrom(Reference43_50.convertReference(t)); 077 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 078 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 079 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 080 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 081 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 082 for (org.hl7.fhir.r4b.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage43_50.convertDosage(t)); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.r4b.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r5.model.MedicationStatement src) throws FHIRException { 087 if (src == null) 088 return null; 089 org.hl7.fhir.r4b.model.MedicationStatement tgt = new org.hl7.fhir.r4b.model.MedicationStatement(); 090 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 091 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 092 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 093// for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 094// for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 095 if (src.hasStatus()) 096 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 097// for (org.hl7.fhir.r5.model.CodeableConcept t : src.getStatusReason()) 098// tgt.addStatusReason(CodeableConcept43_50.convertCodeableConcept(t)); 099 if (src.hasCategory()) 100 tgt.setCategory(CodeableConcept43_50.convertCodeableConcept(src.getCategoryFirstRep())); 101 if (src.getMedication().hasConcept()) { 102 tgt.setMedication(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getMedication().getConcept())); 103 } 104 if (src.getMedication().hasReference()) { 105 tgt.setMedication(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getMedication().getReference())); 106 } 107 if (src.hasSubject()) 108 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 109 if (src.hasEncounter()) 110 tgt.setContext(Reference43_50.convertReference(src.getEncounter())); 111 if (src.hasEffective()) 112 tgt.setEffective(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEffective())); 113 if (src.hasDateAsserted()) 114 tgt.setDateAssertedElement(DateTime43_50.convertDateTime(src.getDateAssertedElement())); 115 if (src.hasInformationSource()) 116 tgt.setInformationSource(Reference43_50.convertReference(src.getInformationSourceFirstRep())); 117 for (org.hl7.fhir.r5.model.Reference t : src.getDerivedFrom()) 118 tgt.addDerivedFrom(Reference43_50.convertReference(t)); 119 for (CodeableReference t : src.getReason()) 120 if (t.hasConcept()) 121 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 122 for (CodeableReference t : src.getReason()) 123 if (t.hasReference()) 124 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 125 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 126 for (org.hl7.fhir.r5.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage43_50.convertDosage(t)); 127 return tgt; 128 } 129 130 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> convertMedicationStatementStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes> src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodesEnumFactory()); 134 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 135 switch (src.getValue()) { 136 case ACTIVE: 137 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 138 break; 139 case COMPLETED: 140 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 141 break; 142 case ENTEREDINERROR: 143 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.ENTEREDINERROR); 144 break; 145 case INTENDED: 146 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 147 break; 148 case STOPPED: 149 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 150 break; 151 case ONHOLD: 152 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 153 break; 154 case UNKNOWN: 155 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 156 break; 157 case NOTTAKEN: 158 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.RECORDED); 159 break; 160 default: 161 tgt.setValue(org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes.NULL); 162 break; 163 } 164 return tgt; 165 } 166 167 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes> convertMedicationStatementStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> src) throws FHIRException { 168 if (src == null || src.isEmpty()) 169 return null; 170 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodesEnumFactory()); 171 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 172 switch (src.getValue()) { 173// case ACTIVE: 174// tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.ACTIVE); 175// break; 176 case RECORDED: 177 tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.COMPLETED); 178 break; 179 case ENTEREDINERROR: 180 tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.ENTEREDINERROR); 181 break; 182// case UNKNOWN: 183// tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.INTENDED); 184// break; 185// case STOPPED: 186// tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.STOPPED); 187// break; 188// case ONHOLD: 189// tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.ONHOLD); 190// break; 191 case DRAFT: 192 tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.UNKNOWN); 193 break; 194// case NOTTAKEN: 195// tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.NOTTAKEN); 196// break; 197 default: 198 tgt.setValue(org.hl7.fhir.r4b.model.MedicationStatement.MedicationStatusCodes.NULL); 199 break; 200 } 201 return tgt; 202 } 203}