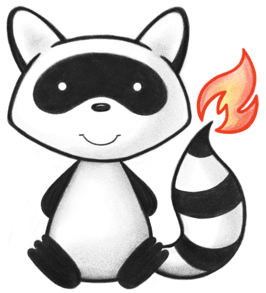
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.ContactPoint43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Url43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Enumeration; 012import org.hl7.fhir.r5.model.MessageHeader; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041*/ 042// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 043public class MessageHeader43_50 { 044 045 public static org.hl7.fhir.r5.model.MessageHeader convertMessageHeader(org.hl7.fhir.r4b.model.MessageHeader src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r5.model.MessageHeader tgt = new org.hl7.fhir.r5.model.MessageHeader(); 049 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 050 if (src.hasEvent()) 051 tgt.setEvent(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEvent())); 052 for (org.hl7.fhir.r4b.model.MessageHeader.MessageDestinationComponent t : src.getDestination()) 053 tgt.addDestination(convertMessageDestinationComponent(t)); 054 if (src.hasSender()) 055 tgt.setSender(Reference43_50.convertReference(src.getSender())); 056// if (src.hasEnterer()) 057// tgt.setEnterer(Reference43_50.convertReference(src.getEnterer())); 058 if (src.hasAuthor()) 059 tgt.setAuthor(Reference43_50.convertReference(src.getAuthor())); 060 if (src.hasSource()) 061 tgt.setSource(convertMessageSourceComponent(src.getSource())); 062 if (src.hasResponsible()) 063 tgt.setResponsible(Reference43_50.convertReference(src.getResponsible())); 064 if (src.hasReason()) 065 tgt.setReason(CodeableConcept43_50.convertCodeableConcept(src.getReason())); 066 if (src.hasResponse()) 067 tgt.setResponse(convertMessageHeaderResponseComponent(src.getResponse())); 068 for (org.hl7.fhir.r4b.model.Reference t : src.getFocus()) tgt.addFocus(Reference43_50.convertReference(t)); 069 if (src.hasDefinition()) 070 tgt.setDefinitionElement(Canonical43_50.convertCanonical(src.getDefinitionElement())); 071 return tgt; 072 } 073 074 public static org.hl7.fhir.r4b.model.MessageHeader convertMessageHeader(org.hl7.fhir.r5.model.MessageHeader src) throws FHIRException { 075 if (src == null) 076 return null; 077 org.hl7.fhir.r4b.model.MessageHeader tgt = new org.hl7.fhir.r4b.model.MessageHeader(); 078 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 079 if (src.hasEvent()) 080 tgt.setEvent(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEvent())); 081 for (org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent t : src.getDestination()) 082 tgt.addDestination(convertMessageDestinationComponent(t)); 083 if (src.hasSender()) 084 tgt.setSender(Reference43_50.convertReference(src.getSender())); 085// if (src.hasEnterer()) 086// tgt.setEnterer(Reference43_50.convertReference(src.getEnterer())); 087 if (src.hasAuthor()) 088 tgt.setAuthor(Reference43_50.convertReference(src.getAuthor())); 089 if (src.hasSource()) 090 tgt.setSource(convertMessageSourceComponent(src.getSource())); 091 if (src.hasResponsible()) 092 tgt.setResponsible(Reference43_50.convertReference(src.getResponsible())); 093 if (src.hasReason()) 094 tgt.setReason(CodeableConcept43_50.convertCodeableConcept(src.getReason())); 095 if (src.hasResponse()) 096 tgt.setResponse(convertMessageHeaderResponseComponent(src.getResponse())); 097 for (org.hl7.fhir.r5.model.Reference t : src.getFocus()) tgt.addFocus(Reference43_50.convertReference(t)); 098 if (src.hasDefinition()) 099 tgt.setDefinitionElement(Canonical43_50.convertCanonical(src.getDefinitionElement())); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent convertMessageDestinationComponent(org.hl7.fhir.r4b.model.MessageHeader.MessageDestinationComponent src) throws FHIRException { 104 if (src == null) 105 return null; 106 org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent(); 107 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 108 if (src.hasName()) 109 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 110 if (src.hasTarget()) 111 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 112 if (src.hasEndpoint()) 113 tgt.setEndpoint(Url43_50.convertUrl(src.getEndpointElement())); 114 if (src.hasReceiver()) 115 tgt.setReceiver(Reference43_50.convertReference(src.getReceiver())); 116 return tgt; 117 } 118 119 public static org.hl7.fhir.r4b.model.MessageHeader.MessageDestinationComponent convertMessageDestinationComponent(org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent src) throws FHIRException { 120 if (src == null) 121 return null; 122 org.hl7.fhir.r4b.model.MessageHeader.MessageDestinationComponent tgt = new org.hl7.fhir.r4b.model.MessageHeader.MessageDestinationComponent(); 123 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 124 if (src.hasName()) 125 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 126 if (src.hasTarget()) 127 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 128 if (src.hasEndpointUrlType()) 129 tgt.setEndpointElement(Url43_50.convertUrl(src.getEndpointUrlType())); 130 if (src.hasReceiver()) 131 tgt.setReceiver(Reference43_50.convertReference(src.getReceiver())); 132 return tgt; 133 } 134 135 public static org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent convertMessageSourceComponent(org.hl7.fhir.r4b.model.MessageHeader.MessageSourceComponent src) throws FHIRException { 136 if (src == null) 137 return null; 138 org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent(); 139 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 140 if (src.hasName()) 141 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 142 if (src.hasSoftware()) 143 tgt.setSoftwareElement(String43_50.convertString(src.getSoftwareElement())); 144 if (src.hasVersion()) 145 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 146 if (src.hasContact()) 147 tgt.setContact(ContactPoint43_50.convertContactPoint(src.getContact())); 148 if (src.hasEndpoint()) 149 tgt.setEndpoint(Url43_50.convertUrl(src.getEndpointElement())); 150 return tgt; 151 } 152 153 public static org.hl7.fhir.r4b.model.MessageHeader.MessageSourceComponent convertMessageSourceComponent(org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent src) throws FHIRException { 154 if (src == null) 155 return null; 156 org.hl7.fhir.r4b.model.MessageHeader.MessageSourceComponent tgt = new org.hl7.fhir.r4b.model.MessageHeader.MessageSourceComponent(); 157 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 158 if (src.hasName()) 159 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 160 if (src.hasSoftware()) 161 tgt.setSoftwareElement(String43_50.convertString(src.getSoftwareElement())); 162 if (src.hasVersion()) 163 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 164 if (src.hasContact()) 165 tgt.setContact(ContactPoint43_50.convertContactPoint(src.getContact())); 166 if (src.hasEndpointUrlType()) 167 tgt.setEndpointElement(Url43_50.convertUrl(src.getEndpointUrlType())); 168 return tgt; 169 } 170 171 public static org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent convertMessageHeaderResponseComponent(org.hl7.fhir.r4b.model.MessageHeader.MessageHeaderResponseComponent src) throws FHIRException { 172 if (src == null) 173 return null; 174 org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent(); 175 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 176 if (src.hasIdentifier()) 177 tgt.setIdentifier(new org.hl7.fhir.r5.model.Identifier().setValue(src.getIdentifier())); 178 if (src.hasCode()) 179 tgt.setCodeElement(convertResponseType(src.getCodeElement())); 180 if (src.hasDetails()) 181 tgt.setDetails(Reference43_50.convertReference(src.getDetails())); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.r4b.model.MessageHeader.MessageHeaderResponseComponent convertMessageHeaderResponseComponent(org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent src) throws FHIRException { 186 if (src == null) 187 return null; 188 org.hl7.fhir.r4b.model.MessageHeader.MessageHeaderResponseComponent tgt = new org.hl7.fhir.r4b.model.MessageHeader.MessageHeaderResponseComponent(); 189 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 190 if (src.hasIdentifier()) 191 tgt.setIdentifierElement(new org.hl7.fhir.r4b.model.IdType(src.getIdentifier().getValue())); 192 if (src.hasCode()) 193 tgt.setCodeElement(convertResponseType(src.getCodeElement())); 194 if (src.hasDetails()) 195 tgt.setDetails(Reference43_50.convertReference(src.getDetails())); 196 return tgt; 197 } 198 199 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MessageHeader.ResponseType> convertResponseType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MessageHeader.ResponseType> src) throws FHIRException { 200 if (src == null || src.isEmpty()) 201 return null; 202 Enumeration<MessageHeader.ResponseType> tgt = new Enumeration<>(new MessageHeader.ResponseTypeEnumFactory()); 203 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 204 if (src.getValue() == null) { 205 tgt.setValue(null); 206 } else { 207 switch (src.getValue()) { 208 case OK: 209 tgt.setValue(MessageHeader.ResponseType.OK); 210 break; 211 case TRANSIENTERROR: 212 tgt.setValue(MessageHeader.ResponseType.TRANSIENTERROR); 213 break; 214 case FATALERROR: 215 tgt.setValue(MessageHeader.ResponseType.FATALERROR); 216 break; 217 default: 218 tgt.setValue(MessageHeader.ResponseType.NULL); 219 break; 220 } 221 } 222 return tgt; 223 } 224 225 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MessageHeader.ResponseType> convertResponseType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MessageHeader.ResponseType> src) throws FHIRException { 226 if (src == null || src.isEmpty()) 227 return null; 228 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.MessageHeader.ResponseType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.MessageHeader.ResponseTypeEnumFactory()); 229 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 230 if (src.getValue() == null) { 231 tgt.setValue(null); 232 } else { 233 switch (src.getValue()) { 234 case OK: 235 tgt.setValue(org.hl7.fhir.r4b.model.MessageHeader.ResponseType.OK); 236 break; 237 case TRANSIENTERROR: 238 tgt.setValue(org.hl7.fhir.r4b.model.MessageHeader.ResponseType.TRANSIENTERROR); 239 break; 240 case FATALERROR: 241 tgt.setValue(org.hl7.fhir.r4b.model.MessageHeader.ResponseType.FATALERROR); 242 break; 243 default: 244 tgt.setValue(org.hl7.fhir.r4b.model.MessageHeader.ResponseType.NULL); 245 break; 246 } 247 } 248 return tgt; 249 } 250}