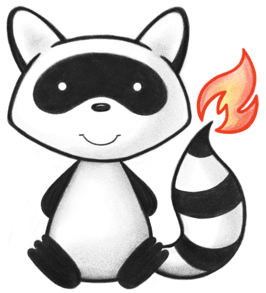
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4b.model.Extension; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.NamingSystem; 018 019/* 020 Copyright (c) 2011+, HL7, Inc. 021 All rights reserved. 022 023 Redistribution and use in source and binary forms, with or without modification, 024 are permitted provided that the following conditions are met: 025 026 * Redistributions of source code must retain the above copyright notice, this 027 list of conditions and the following disclaimer. 028 * Redistributions in binary form must reproduce the above copyright notice, 029 this list of conditions and the following disclaimer in the documentation 030 and/or other materials provided with the distribution. 031 * Neither the name of HL7 nor the names of its contributors may be used to 032 endorse or promote products derived from this software without specific 033 prior written permission. 034 035 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 036 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 037 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 038 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 039 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 040 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 041 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 042 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 043 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 044 POSSIBILITY OF SUCH DAMAGE. 045 046*/ 047// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 048public class NamingSystem43_50 { 049 050 public static org.hl7.fhir.r5.model.NamingSystem convertNamingSystem(org.hl7.fhir.r4b.model.NamingSystem src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r5.model.NamingSystem tgt = new org.hl7.fhir.r5.model.NamingSystem(); 054 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt, VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE); 055 056 if (src.hasExtension(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL)) { 057 tgt.setUrlElement(Uri43_50.convertToUri(src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL).getValue())); 058 } 059 if (src.hasExtension(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION)) { 060 tgt.setVersionElement(String43_50.convertString(src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION).getValueStringType())); 061 } 062 if (src.hasName()) 063 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 064 if (src.hasExtension(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE)) { 065 tgt.setTitleElement(String43_50.convertString(src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE).getValueStringType())); 066 } 067 if (src.hasStatus()) 068 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 069 if (src.hasKind()) 070 tgt.setKindElement(convertNamingSystemType(src.getKindElement())); 071 if (src.hasDate()) 072 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 073 if (src.hasPublisher()) 074 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 075 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 076 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 077 if (src.hasResponsible()) 078 tgt.setResponsibleElement(String43_50.convertString(src.getResponsibleElement())); 079 if (src.hasType()) 080 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 081 if (src.hasDescription()) 082 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 083 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 084 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 085 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 086 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 087 if (src.hasUsage()) 088 tgt.setUsageElement(String43_50.convertString(src.getUsageElement())); 089 for (org.hl7.fhir.r4b.model.NamingSystem.NamingSystemUniqueIdComponent t : src.getUniqueId()) 090 tgt.addUniqueId(convertNamingSystemUniqueIdComponent(t)); 091 return tgt; 092 } 093 094 public static org.hl7.fhir.r4b.model.NamingSystem convertNamingSystem(org.hl7.fhir.r5.model.NamingSystem src) throws FHIRException { 095 if (src == null) 096 return null; 097 org.hl7.fhir.r4b.model.NamingSystem tgt = new org.hl7.fhir.r4b.model.NamingSystem(); 098 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 099 100 if (src.hasUrlElement()) 101 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL).setValue(Uri43_50.convertUri(src.getUrlElement()))); 102 if (src.hasVersionElement()) 103 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION).setValue(String43_50.convertString(src.getVersionElement()))); 104 if (src.hasName()) 105 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 106 if (src.hasTitleElement()) 107 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE).setValue(String43_50.convertString(src.getTitleElement()))); 108 109 if (src.hasStatus()) 110 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 111 if (src.hasKind()) 112 tgt.setKindElement(convertNamingSystemType(src.getKindElement())); 113 if (src.hasDate()) 114 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 115 if (src.hasPublisher()) 116 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 117 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 118 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 119 if (src.hasResponsible()) 120 tgt.setResponsibleElement(String43_50.convertString(src.getResponsibleElement())); 121 if (src.hasType()) 122 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 123 if (src.hasDescription()) 124 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 125 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 126 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 127 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 128 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 129 if (src.hasUsage()) 130 tgt.setUsageElement(String43_50.convertString(src.getUsageElement())); 131 for (org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent t : src.getUniqueId()) 132 tgt.addUniqueId(convertNamingSystemUniqueIdComponent(t)); 133 return tgt; 134 } 135 136 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemType> convertNamingSystemType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType> src) throws FHIRException { 137 if (src == null || src.isEmpty()) 138 return null; 139 Enumeration<NamingSystem.NamingSystemType> tgt = new Enumeration<>(new NamingSystem.NamingSystemTypeEnumFactory()); 140 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 141 if (src.getValue() == null) { 142 tgt.setValue(null); 143 } else { 144 switch (src.getValue()) { 145 case CODESYSTEM: 146 tgt.setValue(NamingSystem.NamingSystemType.CODESYSTEM); 147 break; 148 case IDENTIFIER: 149 tgt.setValue(NamingSystem.NamingSystemType.IDENTIFIER); 150 break; 151 case ROOT: 152 tgt.setValue(NamingSystem.NamingSystemType.ROOT); 153 break; 154 default: 155 tgt.setValue(NamingSystem.NamingSystemType.NULL); 156 break; 157 } 158 } 159 return tgt; 160 } 161 162 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType> convertNamingSystemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemType> src) throws FHIRException { 163 if (src == null || src.isEmpty()) 164 return null; 165 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.NamingSystem.NamingSystemTypeEnumFactory()); 166 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 167 if (src.getValue() == null) { 168 tgt.setValue(null); 169 } else { 170 switch (src.getValue()) { 171 case CODESYSTEM: 172 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType.CODESYSTEM); 173 break; 174 case IDENTIFIER: 175 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType.IDENTIFIER); 176 break; 177 case ROOT: 178 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType.ROOT); 179 break; 180 default: 181 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemType.NULL); 182 break; 183 } 184 } 185 return tgt; 186 } 187 188 public static org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent convertNamingSystemUniqueIdComponent(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemUniqueIdComponent src) throws FHIRException { 189 if (src == null) 190 return null; 191 org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent tgt = new org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent(); 192 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 193 if (src.hasType()) 194 tgt.setTypeElement(convertNamingSystemIdentifierType(src.getTypeElement())); 195 if (src.hasValue()) 196 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 197 if (src.hasPreferred()) 198 tgt.setPreferredElement(Boolean43_50.convertBoolean(src.getPreferredElement())); 199 if (src.hasComment()) 200 tgt.setCommentElement(String43_50.convertString(src.getCommentElement())); 201 if (src.hasPeriod()) 202 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 203 return tgt; 204 } 205 206 public static org.hl7.fhir.r4b.model.NamingSystem.NamingSystemUniqueIdComponent convertNamingSystemUniqueIdComponent(org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent src) throws FHIRException { 207 if (src == null) 208 return null; 209 org.hl7.fhir.r4b.model.NamingSystem.NamingSystemUniqueIdComponent tgt = new org.hl7.fhir.r4b.model.NamingSystem.NamingSystemUniqueIdComponent(); 210 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 211 if (src.hasType()) 212 tgt.setTypeElement(convertNamingSystemIdentifierType(src.getTypeElement())); 213 if (src.hasValue()) 214 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 215 if (src.hasPreferred()) 216 tgt.setPreferredElement(Boolean43_50.convertBoolean(src.getPreferredElement())); 217 if (src.hasComment()) 218 tgt.setCommentElement(String43_50.convertString(src.getCommentElement())); 219 if (src.hasPeriod()) 220 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 221 return tgt; 222 } 223 224 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemIdentifierType> convertNamingSystemIdentifierType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType> src) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 Enumeration<NamingSystem.NamingSystemIdentifierType> tgt = new Enumeration<>(new NamingSystem.NamingSystemIdentifierTypeEnumFactory()); 228 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 229 if (src.getValue() == null) { 230 tgt.setValue(null); 231 } else { 232 switch (src.getValue()) { 233 case OID: 234 tgt.setValue(NamingSystem.NamingSystemIdentifierType.OID); 235 break; 236 case UUID: 237 tgt.setValue(NamingSystem.NamingSystemIdentifierType.UUID); 238 break; 239 case URI: 240 tgt.setValue(NamingSystem.NamingSystemIdentifierType.URI); 241 break; 242 case OTHER: 243 tgt.setValue(NamingSystem.NamingSystemIdentifierType.OTHER); 244 break; 245 default: 246 tgt.setValue(NamingSystem.NamingSystemIdentifierType.NULL); 247 break; 248 } 249 } 250 return tgt; 251 } 252 253 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType> convertNamingSystemIdentifierType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemIdentifierType> src) throws FHIRException { 254 if (src == null || src.isEmpty()) 255 return null; 256 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierTypeEnumFactory()); 257 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 258 if (src.getValue() == null) { 259 tgt.setValue(null); 260 } else { 261 switch (src.getValue()) { 262 case OID: 263 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType.OID); 264 break; 265 case UUID: 266 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType.UUID); 267 break; 268 case URI: 269 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType.URI); 270 break; 271 case OTHER: 272 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType.OTHER); 273 break; 274 default: 275 tgt.setValue(org.hl7.fhir.r4b.model.NamingSystem.NamingSystemIdentifierType.NULL); 276 break; 277 } 278 } 279 return tgt; 280 } 281}