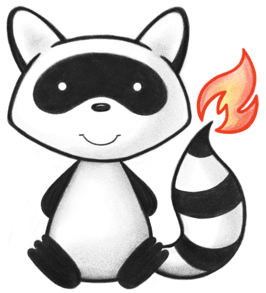
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Address43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.ContactPoint43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.HumanName43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 012import org.hl7.fhir.exceptions.FHIRException; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041*/ 042// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 043public class Person43_50 { 044 045 public static org.hl7.fhir.r5.model.Person convertPerson(org.hl7.fhir.r4b.model.Person src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r5.model.Person tgt = new org.hl7.fhir.r5.model.Person(); 049 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 052 for (org.hl7.fhir.r4b.model.HumanName t : src.getName()) tgt.addName(HumanName43_50.convertHumanName(t)); 053 for (org.hl7.fhir.r4b.model.ContactPoint t : src.getTelecom()) 054 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 055 if (src.hasGender()) 056 tgt.setGenderElement(Enumerations43_50.convertAdministrativeGender(src.getGenderElement())); 057 if (src.hasBirthDate()) 058 tgt.setBirthDateElement(Date43_50.convertDate(src.getBirthDateElement())); 059 for (org.hl7.fhir.r4b.model.Address t : src.getAddress()) tgt.addAddress(Address43_50.convertAddress(t)); 060 if (src.hasPhoto()) 061 tgt.addPhoto(Attachment43_50.convertAttachment(src.getPhoto())); 062 if (src.hasManagingOrganization()) 063 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 064 if (src.hasActive()) 065 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 066 for (org.hl7.fhir.r4b.model.Person.PersonLinkComponent t : src.getLink()) tgt.addLink(convertPersonLinkComponent(t)); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.r4b.model.Person convertPerson(org.hl7.fhir.r5.model.Person src) throws FHIRException { 071 if (src == null) 072 return null; 073 org.hl7.fhir.r4b.model.Person tgt = new org.hl7.fhir.r4b.model.Person(); 074 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 075 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 076 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 077 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.addName(HumanName43_50.convertHumanName(t)); 078 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 079 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 080 if (src.hasGender()) 081 tgt.setGenderElement(Enumerations43_50.convertAdministrativeGender(src.getGenderElement())); 082 if (src.hasBirthDate()) 083 tgt.setBirthDateElement(Date43_50.convertDate(src.getBirthDateElement())); 084 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address43_50.convertAddress(t)); 085 if (src.hasPhoto()) 086 tgt.setPhoto(Attachment43_50.convertAttachment(src.getPhotoFirstRep())); 087 if (src.hasManagingOrganization()) 088 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 089 if (src.hasActive()) 090 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 091 for (org.hl7.fhir.r5.model.Person.PersonLinkComponent t : src.getLink()) tgt.addLink(convertPersonLinkComponent(t)); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.r5.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.r4b.model.Person.PersonLinkComponent src) throws FHIRException { 096 if (src == null) 097 return null; 098 org.hl7.fhir.r5.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.r5.model.Person.PersonLinkComponent(); 099 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 100 if (src.hasTarget()) 101 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 102 if (src.hasAssurance()) 103 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 104 return tgt; 105 } 106 107 public static org.hl7.fhir.r4b.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.r5.model.Person.PersonLinkComponent src) throws FHIRException { 108 if (src == null) 109 return null; 110 org.hl7.fhir.r4b.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.r4b.model.Person.PersonLinkComponent(); 111 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 112 if (src.hasTarget()) 113 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 114 if (src.hasAssurance()) 115 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 116 return tgt; 117 } 118 119 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 120 if (src == null || src.isEmpty()) 121 return null; 122 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Person.IdentityAssuranceLevelEnumFactory()); 123 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 124 switch (src.getValue()) { 125 case LEVEL1: 126 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL1); 127 break; 128 case LEVEL2: 129 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL2); 130 break; 131 case LEVEL3: 132 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL3); 133 break; 134 case LEVEL4: 135 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL4); 136 break; 137 default: 138 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.NULL); 139 break; 140 } 141 return tgt; 142 } 143 144 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevelEnumFactory()); 148 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 149 switch (src.getValue()) { 150 case LEVEL1: 151 tgt.setValue(org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel.LEVEL1); 152 break; 153 case LEVEL2: 154 tgt.setValue(org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel.LEVEL2); 155 break; 156 case LEVEL3: 157 tgt.setValue(org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel.LEVEL3); 158 break; 159 case LEVEL4: 160 tgt.setValue(org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel.LEVEL4); 161 break; 162 default: 163 tgt.setValue(org.hl7.fhir.r4b.model.Person.IdentityAssuranceLevel.NULL); 164 break; 165 } 166 return tgt; 167 } 168}