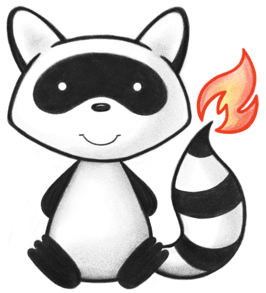
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Duration43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.DataRequirement43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.Expression43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.RelatedArtifact43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.TriggerDefinition43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Id43_50; 019import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 020import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 021import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 022import org.hl7.fhir.exceptions.FHIRException; 023import org.hl7.fhir.r5.model.DataRequirement; 024import org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionInputComponent; 025import org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionOutputComponent; 026 027/* 028 Copyright (c) 2011+, HL7, Inc. 029 All rights reserved. 030 031 Redistribution and use in source and binary forms, with or without modification, 032 are permitted provided that the following conditions are met: 033 034 * Redistributions of source code must retain the above copyright notice, this 035 list of conditions and the following disclaimer. 036 * Redistributions in binary form must reproduce the above copyright notice, 037 this list of conditions and the following disclaimer in the documentation 038 and/or other materials provided with the distribution. 039 * Neither the name of HL7 nor the names of its contributors may be used to 040 endorse or promote products derived from this software without specific 041 prior written permission. 042 043 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 044 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 045 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 046 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 047 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 048 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 049 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 050 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 051 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 052 POSSIBILITY OF SUCH DAMAGE. 053 054*/ 055// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 056public class PlanDefinition43_50 { 057 058 public static org.hl7.fhir.r5.model.PlanDefinition convertPlanDefinition(org.hl7.fhir.r4b.model.PlanDefinition src) throws FHIRException { 059 if (src == null) 060 return null; 061 org.hl7.fhir.r5.model.PlanDefinition tgt = new org.hl7.fhir.r5.model.PlanDefinition(); 062 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 063 if (src.hasUrl()) 064 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 065 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 066 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 067 if (src.hasVersion()) 068 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 069 if (src.hasName()) 070 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 071 if (src.hasTitle()) 072 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 073 if (src.hasSubtitle()) 074 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 075 if (src.hasType()) 076 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 077 if (src.hasStatus()) 078 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 079 if (src.hasExperimental()) 080 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 081 if (src.hasSubject()) 082 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 083 if (src.hasDate()) 084 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 085 if (src.hasPublisher()) 086 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 087 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 088 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 089 if (src.hasDescription()) 090 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 091 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 092 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 093 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 094 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 095 if (src.hasPurpose()) 096 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 097 if (src.hasUsage()) 098 tgt.setUsageElement(String43_50.convertStringToMarkdown(src.getUsageElement())); 099 if (src.hasCopyright()) 100 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 101 if (src.hasApprovalDate()) 102 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 103 if (src.hasLastReviewDate()) 104 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 105 if (src.hasEffectivePeriod()) 106 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 107 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getTopic()) 108 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 109 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getAuthor()) 110 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 111 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEditor()) 112 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 113 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getReviewer()) 114 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 115 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEndorser()) 116 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 117 for (org.hl7.fhir.r4b.model.RelatedArtifact t : src.getRelatedArtifact()) 118 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 119 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getLibrary()) 120 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 121 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalComponent t : src.getGoal()) 122 tgt.addGoal(convertPlanDefinitionGoalComponent(t)); 123 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent t : src.getAction()) 124 tgt.addAction(convertPlanDefinitionActionComponent(t)); 125 return tgt; 126 } 127 128 public static org.hl7.fhir.r4b.model.PlanDefinition convertPlanDefinition(org.hl7.fhir.r5.model.PlanDefinition src) throws FHIRException { 129 if (src == null) 130 return null; 131 org.hl7.fhir.r4b.model.PlanDefinition tgt = new org.hl7.fhir.r4b.model.PlanDefinition(); 132 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 133 if (src.hasUrl()) 134 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 135 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 136 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 137 if (src.hasVersion()) 138 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 139 if (src.hasName()) 140 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 141 if (src.hasTitle()) 142 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 143 if (src.hasSubtitle()) 144 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 145 if (src.hasType()) 146 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 147 if (src.hasStatus()) 148 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 149 if (src.hasExperimental()) 150 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 151 if (src.hasSubject()) 152 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 153 if (src.hasDate()) 154 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 155 if (src.hasPublisher()) 156 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 157 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 158 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 159 if (src.hasDescription()) 160 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 161 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 162 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 163 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 164 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 165 if (src.hasPurpose()) 166 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 167 if (src.hasUsage()) 168 tgt.setUsageElement(String43_50.convertString(src.getUsageElement())); 169 if (src.hasCopyright()) 170 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 171 if (src.hasApprovalDate()) 172 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 173 if (src.hasLastReviewDate()) 174 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 175 if (src.hasEffectivePeriod()) 176 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 177 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getTopic()) 178 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 179 for (org.hl7.fhir.r5.model.ContactDetail t : src.getAuthor()) 180 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 181 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEditor()) 182 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 183 for (org.hl7.fhir.r5.model.ContactDetail t : src.getReviewer()) 184 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 185 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEndorser()) 186 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 187 for (org.hl7.fhir.r5.model.RelatedArtifact t : src.getRelatedArtifact()) 188 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 189 for (org.hl7.fhir.r5.model.CanonicalType t : src.getLibrary()) 190 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 191 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalComponent t : src.getGoal()) 192 tgt.addGoal(convertPlanDefinitionGoalComponent(t)); 193 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent t : src.getAction()) 194 tgt.addAction(convertPlanDefinitionActionComponent(t)); 195 return tgt; 196 } 197 198 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalComponent convertPlanDefinitionGoalComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalComponent src) throws FHIRException { 199 if (src == null) 200 return null; 201 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalComponent(); 202 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 203 if (src.hasCategory()) 204 tgt.setCategory(CodeableConcept43_50.convertCodeableConcept(src.getCategory())); 205 if (src.hasDescription()) 206 tgt.setDescription(CodeableConcept43_50.convertCodeableConcept(src.getDescription())); 207 if (src.hasPriority()) 208 tgt.setPriority(CodeableConcept43_50.convertCodeableConcept(src.getPriority())); 209 if (src.hasStart()) 210 tgt.setStart(CodeableConcept43_50.convertCodeableConcept(src.getStart())); 211 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getAddresses()) 212 tgt.addAddresses(CodeableConcept43_50.convertCodeableConcept(t)); 213 for (org.hl7.fhir.r4b.model.RelatedArtifact t : src.getDocumentation()) 214 tgt.addDocumentation(RelatedArtifact43_50.convertRelatedArtifact(t)); 215 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalTargetComponent t : src.getTarget()) 216 tgt.addTarget(convertPlanDefinitionGoalTargetComponent(t)); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalComponent convertPlanDefinitionGoalComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalComponent src) throws FHIRException { 221 if (src == null) 222 return null; 223 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalComponent(); 224 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 225 if (src.hasCategory()) 226 tgt.setCategory(CodeableConcept43_50.convertCodeableConcept(src.getCategory())); 227 if (src.hasDescription()) 228 tgt.setDescription(CodeableConcept43_50.convertCodeableConcept(src.getDescription())); 229 if (src.hasPriority()) 230 tgt.setPriority(CodeableConcept43_50.convertCodeableConcept(src.getPriority())); 231 if (src.hasStart()) 232 tgt.setStart(CodeableConcept43_50.convertCodeableConcept(src.getStart())); 233 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAddresses()) 234 tgt.addAddresses(CodeableConcept43_50.convertCodeableConcept(t)); 235 for (org.hl7.fhir.r5.model.RelatedArtifact t : src.getDocumentation()) 236 tgt.addDocumentation(RelatedArtifact43_50.convertRelatedArtifact(t)); 237 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalTargetComponent t : src.getTarget()) 238 tgt.addTarget(convertPlanDefinitionGoalTargetComponent(t)); 239 return tgt; 240 } 241 242 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalTargetComponent convertPlanDefinitionGoalTargetComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalTargetComponent src) throws FHIRException { 243 if (src == null) 244 return null; 245 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalTargetComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalTargetComponent(); 246 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 247 if (src.hasMeasure()) 248 tgt.setMeasure(CodeableConcept43_50.convertCodeableConcept(src.getMeasure())); 249 if (src.hasDetail()) 250 tgt.setDetail(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDetail())); 251 if (src.hasDue()) 252 tgt.setDue(Duration43_50.convertDuration(src.getDue())); 253 return tgt; 254 } 255 256 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalTargetComponent convertPlanDefinitionGoalTargetComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionGoalTargetComponent src) throws FHIRException { 257 if (src == null) 258 return null; 259 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalTargetComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionGoalTargetComponent(); 260 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 261 if (src.hasMeasure()) 262 tgt.setMeasure(CodeableConcept43_50.convertCodeableConcept(src.getMeasure())); 263 if (src.hasDetail()) 264 tgt.setDetail(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDetail())); 265 if (src.hasDue()) 266 tgt.setDue(Duration43_50.convertDuration(src.getDue())); 267 return tgt; 268 } 269 270 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent convertPlanDefinitionActionComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent src) throws FHIRException { 271 if (src == null) 272 return null; 273 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent(); 274 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 275 if (src.hasPrefix()) 276 tgt.setPrefixElement(String43_50.convertString(src.getPrefixElement())); 277 if (src.hasTitle()) 278 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 279 if (src.hasDescription()) 280 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 281 if (src.hasTextEquivalent()) 282 tgt.setTextEquivalentElement(String43_50.convertStringToMarkdown(src.getTextEquivalentElement())); 283 if (src.hasPriority()) 284 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 285 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCode()) 286 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(t)); 287 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReason()) 288 tgt.addReason(CodeableConcept43_50.convertCodeableConcept(t)); 289 for (org.hl7.fhir.r4b.model.RelatedArtifact t : src.getDocumentation()) 290 tgt.addDocumentation(RelatedArtifact43_50.convertRelatedArtifact(t)); 291 for (org.hl7.fhir.r4b.model.IdType t : src.getGoalId()) tgt.getGoalId().add(Id43_50.convertId(t)); 292 if (src.hasSubject()) 293 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 294 for (org.hl7.fhir.r4b.model.TriggerDefinition t : src.getTrigger()) 295 tgt.addTrigger(TriggerDefinition43_50.convertTriggerDefinition(t)); 296 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionConditionComponent t : src.getCondition()) 297 tgt.addCondition(convertPlanDefinitionActionConditionComponent(t)); 298 for (org.hl7.fhir.r4b.model.DataRequirement t : src.getInput()) 299 tgt.addInput(wrapInput(DataRequirement43_50.convertDataRequirement(t))); 300 for (org.hl7.fhir.r4b.model.DataRequirement t : src.getOutput()) 301 tgt.addOutput(wrapOutput(DataRequirement43_50.convertDataRequirement(t))); 302 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent t : src.getRelatedAction()) 303 tgt.addRelatedAction(convertPlanDefinitionActionRelatedActionComponent(t)); 304 if (src.hasTiming()) 305 tgt.setTiming(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTiming())); 306 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionParticipantComponent t : src.getParticipant()) 307 tgt.addParticipant(convertPlanDefinitionActionParticipantComponent(t)); 308 if (src.hasType()) 309 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 310 if (src.hasGroupingBehavior()) 311 tgt.setGroupingBehaviorElement(convertActionGroupingBehavior(src.getGroupingBehaviorElement())); 312 if (src.hasSelectionBehavior()) 313 tgt.setSelectionBehaviorElement(convertActionSelectionBehavior(src.getSelectionBehaviorElement())); 314 if (src.hasRequiredBehavior()) 315 tgt.setRequiredBehaviorElement(convertActionRequiredBehavior(src.getRequiredBehaviorElement())); 316 if (src.hasPrecheckBehavior()) 317 tgt.setPrecheckBehaviorElement(convertActionPrecheckBehavior(src.getPrecheckBehaviorElement())); 318 if (src.hasCardinalityBehavior()) 319 tgt.setCardinalityBehaviorElement(convertActionCardinalityBehavior(src.getCardinalityBehaviorElement())); 320 if (src.hasDefinition()) 321 tgt.setDefinition(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDefinition())); 322 if (src.hasTransform()) 323 tgt.setTransformElement(Canonical43_50.convertCanonical(src.getTransformElement())); 324 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent t : src.getDynamicValue()) 325 tgt.addDynamicValue(convertPlanDefinitionActionDynamicValueComponent(t)); 326 for (org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent t : src.getAction()) 327 tgt.addAction(convertPlanDefinitionActionComponent(t)); 328 return tgt; 329 } 330 331 private static PlanDefinitionActionOutputComponent wrapOutput(DataRequirement dr) { 332 return new PlanDefinitionActionOutputComponent().setRequirement(dr); 333 } 334 335 private static PlanDefinitionActionInputComponent wrapInput(DataRequirement dr) { 336 return new PlanDefinitionActionInputComponent().setRequirement(dr); 337 } 338 339 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent convertPlanDefinitionActionComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent src) throws FHIRException { 340 if (src == null) 341 return null; 342 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionComponent(); 343 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 344 if (src.hasPrefix()) 345 tgt.setPrefixElement(String43_50.convertString(src.getPrefixElement())); 346 if (src.hasTitle()) 347 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 348 if (src.hasDescription()) 349 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 350 if (src.hasTextEquivalent()) 351 tgt.setTextEquivalentElement(String43_50.convertString(src.getTextEquivalentElement())); 352 if (src.hasPriority()) 353 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 354 if (src.hasCode()) tgt.addCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 355 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getReason()) 356 tgt.addReason(CodeableConcept43_50.convertCodeableConcept(t)); 357 for (org.hl7.fhir.r5.model.RelatedArtifact t : src.getDocumentation()) 358 tgt.addDocumentation(RelatedArtifact43_50.convertRelatedArtifact(t)); 359 for (org.hl7.fhir.r5.model.IdType t : src.getGoalId()) tgt.getGoalId().add(Id43_50.convertId(t)); 360 if (src.hasSubject()) 361 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 362 for (org.hl7.fhir.r5.model.TriggerDefinition t : src.getTrigger()) 363 tgt.addTrigger(TriggerDefinition43_50.convertTriggerDefinition(t)); 364 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionConditionComponent t : src.getCondition()) 365 tgt.addCondition(convertPlanDefinitionActionConditionComponent(t)); 366 for (PlanDefinitionActionInputComponent t : src.getInput()) 367 tgt.addInput(DataRequirement43_50.convertDataRequirement(t.getRequirement())); 368 for (PlanDefinitionActionOutputComponent t : src.getOutput()) 369 tgt.addOutput(DataRequirement43_50.convertDataRequirement(t.getRequirement())); 370 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent t : src.getRelatedAction()) 371 tgt.addRelatedAction(convertPlanDefinitionActionRelatedActionComponent(t)); 372 if (src.hasTiming()) 373 tgt.setTiming(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTiming())); 374 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionParticipantComponent t : src.getParticipant()) 375 tgt.addParticipant(convertPlanDefinitionActionParticipantComponent(t)); 376 if (src.hasType()) 377 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 378 if (src.hasGroupingBehavior()) 379 tgt.setGroupingBehaviorElement(convertActionGroupingBehavior(src.getGroupingBehaviorElement())); 380 if (src.hasSelectionBehavior()) 381 tgt.setSelectionBehaviorElement(convertActionSelectionBehavior(src.getSelectionBehaviorElement())); 382 if (src.hasRequiredBehavior()) 383 tgt.setRequiredBehaviorElement(convertActionRequiredBehavior(src.getRequiredBehaviorElement())); 384 if (src.hasPrecheckBehavior()) 385 tgt.setPrecheckBehaviorElement(convertActionPrecheckBehavior(src.getPrecheckBehaviorElement())); 386 if (src.hasCardinalityBehavior()) 387 tgt.setCardinalityBehaviorElement(convertActionCardinalityBehavior(src.getCardinalityBehaviorElement())); 388 if (src.hasDefinition()) 389 tgt.setDefinition(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getDefinition())); 390 if (src.hasTransform()) 391 tgt.setTransformElement(Canonical43_50.convertCanonical(src.getTransformElement())); 392 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent t : src.getDynamicValue()) 393 tgt.addDynamicValue(convertPlanDefinitionActionDynamicValueComponent(t)); 394 for (org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionComponent t : src.getAction()) 395 tgt.addAction(convertPlanDefinitionActionComponent(t)); 396 return tgt; 397 } 398 399 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 400 if (src == null || src.isEmpty()) 401 return null; 402 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.RequestPriorityEnumFactory()); 403 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 404 switch (src.getValue()) { 405 case ROUTINE: 406 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.ROUTINE); 407 break; 408 case URGENT: 409 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.URGENT); 410 break; 411 case ASAP: 412 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.ASAP); 413 break; 414 case STAT: 415 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.STAT); 416 break; 417 default: 418 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.NULL); 419 break; 420 } 421 return tgt; 422 } 423 424 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 425 if (src == null || src.isEmpty()) 426 return null; 427 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 428 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 429 switch (src.getValue()) { 430 case ROUTINE: 431 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 432 break; 433 case URGENT: 434 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 435 break; 436 case ASAP: 437 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 438 break; 439 case STAT: 440 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 441 break; 442 default: 443 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 444 break; 445 } 446 return tgt; 447 } 448 449 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior> convertActionGroupingBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior> src) throws FHIRException { 450 if (src == null || src.isEmpty()) 451 return null; 452 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehaviorEnumFactory()); 453 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 454 switch (src.getValue()) { 455 case VISUALGROUP: 456 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior.VISUALGROUP); 457 break; 458 case LOGICALGROUP: 459 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior.LOGICALGROUP); 460 break; 461 case SENTENCEGROUP: 462 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior.SENTENCEGROUP); 463 break; 464 default: 465 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior.NULL); 466 break; 467 } 468 return tgt; 469 } 470 471 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior> convertActionGroupingBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionGroupingBehavior> src) throws FHIRException { 472 if (src == null || src.isEmpty()) 473 return null; 474 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehaviorEnumFactory()); 475 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 476 switch (src.getValue()) { 477 case VISUALGROUP: 478 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior.VISUALGROUP); 479 break; 480 case LOGICALGROUP: 481 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior.LOGICALGROUP); 482 break; 483 case SENTENCEGROUP: 484 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior.SENTENCEGROUP); 485 break; 486 default: 487 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionGroupingBehavior.NULL); 488 break; 489 } 490 return tgt; 491 } 492 493 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior> convertActionSelectionBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior> src) throws FHIRException { 494 if (src == null || src.isEmpty()) 495 return null; 496 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehaviorEnumFactory()); 497 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 498 switch (src.getValue()) { 499 case ANY: 500 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.ANY); 501 break; 502 case ALL: 503 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.ALL); 504 break; 505 case ALLORNONE: 506 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.ALLORNONE); 507 break; 508 case EXACTLYONE: 509 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.EXACTLYONE); 510 break; 511 case ATMOSTONE: 512 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.ATMOSTONE); 513 break; 514 case ONEORMORE: 515 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.ONEORMORE); 516 break; 517 default: 518 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior.NULL); 519 break; 520 } 521 return tgt; 522 } 523 524 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior> convertActionSelectionBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionSelectionBehavior> src) throws FHIRException { 525 if (src == null || src.isEmpty()) 526 return null; 527 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehaviorEnumFactory()); 528 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 529 switch (src.getValue()) { 530 case ANY: 531 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.ANY); 532 break; 533 case ALL: 534 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.ALL); 535 break; 536 case ALLORNONE: 537 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.ALLORNONE); 538 break; 539 case EXACTLYONE: 540 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.EXACTLYONE); 541 break; 542 case ATMOSTONE: 543 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.ATMOSTONE); 544 break; 545 case ONEORMORE: 546 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.ONEORMORE); 547 break; 548 default: 549 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionSelectionBehavior.NULL); 550 break; 551 } 552 return tgt; 553 } 554 555 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior> convertActionRequiredBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior> src) throws FHIRException { 556 if (src == null || src.isEmpty()) 557 return null; 558 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehaviorEnumFactory()); 559 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 560 switch (src.getValue()) { 561 case MUST: 562 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior.MUST); 563 break; 564 case COULD: 565 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior.COULD); 566 break; 567 case MUSTUNLESSDOCUMENTED: 568 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior.MUSTUNLESSDOCUMENTED); 569 break; 570 default: 571 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior.NULL); 572 break; 573 } 574 return tgt; 575 } 576 577 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior> convertActionRequiredBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRequiredBehavior> src) throws FHIRException { 578 if (src == null || src.isEmpty()) 579 return null; 580 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehaviorEnumFactory()); 581 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 582 switch (src.getValue()) { 583 case MUST: 584 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior.MUST); 585 break; 586 case COULD: 587 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior.COULD); 588 break; 589 case MUSTUNLESSDOCUMENTED: 590 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior.MUSTUNLESSDOCUMENTED); 591 break; 592 default: 593 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRequiredBehavior.NULL); 594 break; 595 } 596 return tgt; 597 } 598 599 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior> convertActionPrecheckBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior> src) throws FHIRException { 600 if (src == null || src.isEmpty()) 601 return null; 602 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehaviorEnumFactory()); 603 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 604 switch (src.getValue()) { 605 case YES: 606 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior.YES); 607 break; 608 case NO: 609 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior.NO); 610 break; 611 default: 612 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior.NULL); 613 break; 614 } 615 return tgt; 616 } 617 618 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior> convertActionPrecheckBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionPrecheckBehavior> src) throws FHIRException { 619 if (src == null || src.isEmpty()) 620 return null; 621 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehaviorEnumFactory()); 622 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 623 switch (src.getValue()) { 624 case YES: 625 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior.YES); 626 break; 627 case NO: 628 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior.NO); 629 break; 630 default: 631 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionPrecheckBehavior.NULL); 632 break; 633 } 634 return tgt; 635 } 636 637 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior> convertActionCardinalityBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior> src) throws FHIRException { 638 if (src == null || src.isEmpty()) 639 return null; 640 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehaviorEnumFactory()); 641 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 642 switch (src.getValue()) { 643 case SINGLE: 644 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior.SINGLE); 645 break; 646 case MULTIPLE: 647 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior.MULTIPLE); 648 break; 649 default: 650 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior.NULL); 651 break; 652 } 653 return tgt; 654 } 655 656 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior> convertActionCardinalityBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionCardinalityBehavior> src) throws FHIRException { 657 if (src == null || src.isEmpty()) 658 return null; 659 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehaviorEnumFactory()); 660 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 661 switch (src.getValue()) { 662 case SINGLE: 663 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior.SINGLE); 664 break; 665 case MULTIPLE: 666 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior.MULTIPLE); 667 break; 668 default: 669 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionCardinalityBehavior.NULL); 670 break; 671 } 672 return tgt; 673 } 674 675 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionConditionComponent convertPlanDefinitionActionConditionComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionConditionComponent src) throws FHIRException { 676 if (src == null) 677 return null; 678 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionConditionComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionConditionComponent(); 679 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 680 if (src.hasKind()) 681 tgt.setKindElement(convertActionConditionKind(src.getKindElement())); 682 if (src.hasExpression()) 683 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 684 return tgt; 685 } 686 687 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionConditionComponent convertPlanDefinitionActionConditionComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionConditionComponent src) throws FHIRException { 688 if (src == null) 689 return null; 690 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionConditionComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionConditionComponent(); 691 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 692 if (src.hasKind()) 693 tgt.setKindElement(convertActionConditionKind(src.getKindElement())); 694 if (src.hasExpression()) 695 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 696 return tgt; 697 } 698 699 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionConditionKind> convertActionConditionKind(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind> src) throws FHIRException { 700 if (src == null || src.isEmpty()) 701 return null; 702 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionConditionKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionConditionKindEnumFactory()); 703 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 704 switch (src.getValue()) { 705 case APPLICABILITY: 706 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionConditionKind.APPLICABILITY); 707 break; 708 case START: 709 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionConditionKind.START); 710 break; 711 case STOP: 712 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionConditionKind.STOP); 713 break; 714 default: 715 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionConditionKind.NULL); 716 break; 717 } 718 return tgt; 719 } 720 721 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind> convertActionConditionKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionConditionKind> src) throws FHIRException { 722 if (src == null || src.isEmpty()) 723 return null; 724 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionConditionKindEnumFactory()); 725 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 726 switch (src.getValue()) { 727 case APPLICABILITY: 728 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind.APPLICABILITY); 729 break; 730 case START: 731 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind.START); 732 break; 733 case STOP: 734 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind.STOP); 735 break; 736 default: 737 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionConditionKind.NULL); 738 break; 739 } 740 return tgt; 741 } 742 743 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent convertPlanDefinitionActionRelatedActionComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent src) throws FHIRException { 744 if (src == null) 745 return null; 746 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent(); 747 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 748 if (src.hasActionId()) 749 tgt.setTargetIdElement(Id43_50.convertId(src.getActionIdElement())); 750 if (src.hasRelationship()) 751 tgt.setRelationshipElement(convertActionRelationshipType(src.getRelationshipElement())); 752 if (src.hasOffset()) 753 tgt.setOffset(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOffset())); 754 return tgt; 755 } 756 757 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent convertPlanDefinitionActionRelatedActionComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent src) throws FHIRException { 758 if (src == null) 759 return null; 760 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionRelatedActionComponent(); 761 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 762 if (src.hasTargetId()) 763 tgt.setActionIdElement(Id43_50.convertId(src.getTargetIdElement())); 764 if (src.hasRelationship()) 765 tgt.setRelationshipElement(convertActionRelationshipType(src.getRelationshipElement())); 766 if (src.hasOffset()) 767 tgt.setOffset(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOffset())); 768 return tgt; 769 } 770 771 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType> convertActionRelationshipType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType> src) throws FHIRException { 772 if (src == null || src.isEmpty()) 773 return null; 774 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionRelationshipTypeEnumFactory()); 775 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 776 switch (src.getValue()) { 777 case BEFORESTART: 778 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.BEFORESTART); 779 break; 780 case BEFORE: 781 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.BEFORE); 782 break; 783 case BEFOREEND: 784 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.BEFOREEND); 785 break; 786 case CONCURRENTWITHSTART: 787 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.CONCURRENTWITHSTART); 788 break; 789 case CONCURRENT: 790 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.CONCURRENT); 791 break; 792 case CONCURRENTWITHEND: 793 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.CONCURRENTWITHEND); 794 break; 795 case AFTERSTART: 796 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.AFTERSTART); 797 break; 798 case AFTER: 799 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.AFTER); 800 break; 801 case AFTEREND: 802 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.AFTEREND); 803 break; 804 default: 805 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType.NULL); 806 break; 807 } 808 return tgt; 809 } 810 811 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType> convertActionRelationshipType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionRelationshipType> src) throws FHIRException { 812 if (src == null || src.isEmpty()) 813 return null; 814 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipTypeEnumFactory()); 815 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 816 switch (src.getValue()) { 817 case BEFORESTART: 818 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.BEFORESTART); 819 break; 820 case BEFORE: 821 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.BEFORE); 822 break; 823 case BEFOREEND: 824 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.BEFOREEND); 825 break; 826 case CONCURRENTWITHSTART: 827 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.CONCURRENTWITHSTART); 828 break; 829 case CONCURRENT: 830 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.CONCURRENT); 831 break; 832 case CONCURRENTWITHEND: 833 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.CONCURRENTWITHEND); 834 break; 835 case AFTERSTART: 836 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.AFTERSTART); 837 break; 838 case AFTER: 839 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.AFTER); 840 break; 841 case AFTEREND: 842 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.AFTEREND); 843 break; 844 default: 845 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionRelationshipType.NULL); 846 break; 847 } 848 return tgt; 849 } 850 851 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionParticipantComponent convertPlanDefinitionActionParticipantComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionParticipantComponent src) throws FHIRException { 852 if (src == null) 853 return null; 854 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionParticipantComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionParticipantComponent(); 855 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 856 if (src.hasType()) 857 tgt.setTypeElement(convertActionParticipantType(src.getTypeElement())); 858 if (src.hasRole()) 859 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 860 return tgt; 861 } 862 863 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionParticipantComponent convertPlanDefinitionActionParticipantComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionParticipantComponent src) throws FHIRException { 864 if (src == null) 865 return null; 866 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionParticipantComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionParticipantComponent(); 867 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 868 if (src.hasType()) 869 tgt.setTypeElement(convertActionParticipantType(src.getTypeElement())); 870 if (src.hasRole()) 871 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 872 return tgt; 873 } 874 875 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionParticipantType> convertActionParticipantType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> src) throws FHIRException { 876 if (src == null || src.isEmpty()) 877 return null; 878 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionParticipantType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ActionParticipantTypeEnumFactory()); 879 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 880 switch (src.getValue()) { 881 case PATIENT: 882 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionParticipantType.PATIENT); 883 break; 884 case PRACTITIONER: 885 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionParticipantType.PRACTITIONER); 886 break; 887 case RELATEDPERSON: 888 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionParticipantType.RELATEDPERSON); 889 break; 890 case DEVICE: 891 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionParticipantType.DEVICE); 892 break; 893 default: 894 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ActionParticipantType.NULL); 895 break; 896 } 897 return tgt; 898 } 899 900 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> convertActionParticipantType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionParticipantType> src) throws FHIRException { 901 if (src == null || src.isEmpty()) 902 return null; 903 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionParticipantTypeEnumFactory()); 904 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 905 switch (src.getValue()) { 906 case PATIENT: 907 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.PATIENT); 908 break; 909 case PRACTITIONER: 910 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.PRACTITIONER); 911 break; 912 case RELATEDPERSON: 913 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.RELATEDPERSON); 914 break; 915 case DEVICE: 916 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.DEVICE); 917 break; 918 default: 919 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.NULL); 920 break; 921 } 922 return tgt; 923 } 924 925 public static org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent convertPlanDefinitionActionDynamicValueComponent(org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent src) throws FHIRException { 926 if (src == null) 927 return null; 928 org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent tgt = new org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent(); 929 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 930 if (src.hasPath()) 931 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 932 if (src.hasExpression()) 933 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 934 return tgt; 935 } 936 937 public static org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent convertPlanDefinitionActionDynamicValueComponent(org.hl7.fhir.r5.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent src) throws FHIRException { 938 if (src == null) 939 return null; 940 org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent tgt = new org.hl7.fhir.r4b.model.PlanDefinition.PlanDefinitionActionDynamicValueComponent(); 941 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 942 if (src.hasPath()) 943 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 944 if (src.hasExpression()) 945 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 946 return tgt; 947 } 948}