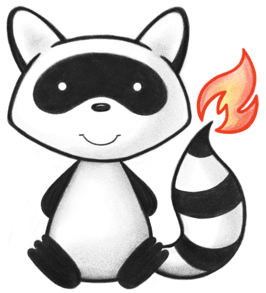
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Address43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.ContactPoint43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.HumanName43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.Practitioner.PractitionerCommunicationComponent; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class Practitioner43_50 { 047 048 public static org.hl7.fhir.r5.model.Practitioner convertPractitioner(org.hl7.fhir.r4b.model.Practitioner src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.Practitioner tgt = new org.hl7.fhir.r5.model.Practitioner(); 052 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 055 if (src.hasActive()) 056 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 057 for (org.hl7.fhir.r4b.model.HumanName t : src.getName()) tgt.addName(HumanName43_50.convertHumanName(t)); 058 for (org.hl7.fhir.r4b.model.ContactPoint t : src.getTelecom()) 059 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 060 for (org.hl7.fhir.r4b.model.Address t : src.getAddress()) tgt.addAddress(Address43_50.convertAddress(t)); 061 if (src.hasGender()) 062 tgt.setGenderElement(Enumerations43_50.convertAdministrativeGender(src.getGenderElement())); 063 if (src.hasBirthDate()) 064 tgt.setBirthDateElement(Date43_50.convertDate(src.getBirthDateElement())); 065 for (org.hl7.fhir.r4b.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment43_50.convertAttachment(t)); 066 for (org.hl7.fhir.r4b.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 067 tgt.addQualification(convertPractitionerQualificationComponent(t)); 068 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCommunication()) 069 tgt.addCommunication().setLanguage(CodeableConcept43_50.convertCodeableConcept(t)); 070 return tgt; 071 } 072 073 public static org.hl7.fhir.r4b.model.Practitioner convertPractitioner(org.hl7.fhir.r5.model.Practitioner src) throws FHIRException { 074 if (src == null) 075 return null; 076 org.hl7.fhir.r4b.model.Practitioner tgt = new org.hl7.fhir.r4b.model.Practitioner(); 077 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 078 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 079 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 080 if (src.hasActive()) 081 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 082 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.addName(HumanName43_50.convertHumanName(t)); 083 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 084 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 085 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address43_50.convertAddress(t)); 086 if (src.hasGender()) 087 tgt.setGenderElement(Enumerations43_50.convertAdministrativeGender(src.getGenderElement())); 088 if (src.hasBirthDate()) 089 tgt.setBirthDateElement(Date43_50.convertDate(src.getBirthDateElement())); 090 for (org.hl7.fhir.r5.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment43_50.convertAttachment(t)); 091 for (org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 092 tgt.addQualification(convertPractitionerQualificationComponent(t)); 093 for (PractitionerCommunicationComponent t : src.getCommunication()) 094 tgt.addCommunication(CodeableConcept43_50.convertCodeableConcept(t.getLanguage())); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.r4b.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 099 if (src == null) 100 return null; 101 org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent(); 102 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 103 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 104 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 105 if (src.hasCode()) 106 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 107 if (src.hasPeriod()) 108 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 109 if (src.hasIssuer()) 110 tgt.setIssuer(Reference43_50.convertReference(src.getIssuer())); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.r4b.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 115 if (src == null) 116 return null; 117 org.hl7.fhir.r4b.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.r4b.model.Practitioner.PractitionerQualificationComponent(); 118 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 119 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 120 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 121 if (src.hasCode()) 122 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 123 if (src.hasPeriod()) 124 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 125 if (src.hasIssuer()) 126 tgt.setIssuer(Reference43_50.convertReference(src.getIssuer())); 127 return tgt; 128 } 129}