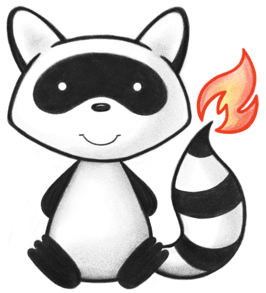
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.conv43_50.datatypes43_50.BackboneElement43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Quantity43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class ProdCharacteristic43_50 { 011 public static org.hl7.fhir.r5.model.ProdCharacteristic convertProdCharacteristic(org.hl7.fhir.r4b.model.ProdCharacteristic src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r5.model.ProdCharacteristic tgt = new org.hl7.fhir.r5.model.ProdCharacteristic(); 014 BackboneElement43_50.copyBackboneElement(src, tgt); 015 if (src.hasHeight()) tgt.setHeight(Quantity43_50.convertQuantity(src.getHeight())); 016 if (src.hasWidth()) tgt.setWidth(Quantity43_50.convertQuantity(src.getWidth())); 017 if (src.hasDepth()) tgt.setDepth(Quantity43_50.convertQuantity(src.getDepth())); 018 if (src.hasWeight()) tgt.setWeight(Quantity43_50.convertQuantity(src.getWeight())); 019 if (src.hasNominalVolume()) tgt.setNominalVolume(Quantity43_50.convertQuantity(src.getNominalVolume())); 020 if (src.hasExternalDiameter()) tgt.setExternalDiameter(Quantity43_50.convertQuantity(src.getExternalDiameter())); 021 if (src.hasShape()) tgt.setShapeElement(String43_50.convertString(src.getShapeElement())); 022 for (org.hl7.fhir.r4b.model.StringType t : src.getColor()) tgt.getColor().add(String43_50.convertString(t)); 023 for (org.hl7.fhir.r4b.model.StringType t : src.getImprint()) tgt.getImprint().add(String43_50.convertString(t)); 024 for (org.hl7.fhir.r4b.model.Attachment t : src.getImage()) tgt.addImage(Attachment43_50.convertAttachment(t)); 025 if (src.hasScoring()) tgt.setScoring(CodeableConcept43_50.convertCodeableConcept(src.getScoring())); 026 return tgt; 027 } 028 029 public static org.hl7.fhir.r4b.model.ProdCharacteristic convertProdCharacteristic(org.hl7.fhir.r5.model.ProdCharacteristic src) throws FHIRException { 030 if (src == null) return null; 031 org.hl7.fhir.r4b.model.ProdCharacteristic tgt = new org.hl7.fhir.r4b.model.ProdCharacteristic(); 032 BackboneElement43_50.copyBackboneElement(src, tgt); 033 if (src.hasHeight()) tgt.setHeight(Quantity43_50.convertQuantity(src.getHeight())); 034 if (src.hasWidth()) tgt.setWidth(Quantity43_50.convertQuantity(src.getWidth())); 035 if (src.hasDepth()) tgt.setDepth(Quantity43_50.convertQuantity(src.getDepth())); 036 if (src.hasWeight()) tgt.setWeight(Quantity43_50.convertQuantity(src.getWeight())); 037 if (src.hasNominalVolume()) tgt.setNominalVolume(Quantity43_50.convertQuantity(src.getNominalVolume())); 038 if (src.hasExternalDiameter()) tgt.setExternalDiameter(Quantity43_50.convertQuantity(src.getExternalDiameter())); 039 if (src.hasShape()) tgt.setShapeElement(String43_50.convertString(src.getShapeElement())); 040 for (org.hl7.fhir.r5.model.StringType t : src.getColor()) tgt.getColor().add(String43_50.convertString(t)); 041 for (org.hl7.fhir.r5.model.StringType t : src.getImprint()) tgt.getImprint().add(String43_50.convertString(t)); 042 for (org.hl7.fhir.r5.model.Attachment t : src.getImage()) tgt.addImage(Attachment43_50.convertAttachment(t)); 043 if (src.hasScoring()) tgt.setScoring(CodeableConcept43_50.convertCodeableConcept(src.getScoring())); 044 return tgt; 045 } 046}