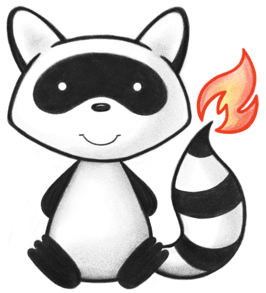
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.conv43_50.datatypes43_50.BackboneElement43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Quantity43_50; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class ProductShelfLife43_50 { 009 public static org.hl7.fhir.r5.model.ProductShelfLife convertProductShelfLife(org.hl7.fhir.r4b.model.ProductShelfLife src) throws FHIRException { 010 if (src == null) return null; 011 org.hl7.fhir.r5.model.ProductShelfLife tgt = new org.hl7.fhir.r5.model.ProductShelfLife(); 012 BackboneElement43_50.copyBackboneElement(src, tgt); 013 if (src.hasType()) tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 014 if (src.hasPeriodDuration()) tgt.setPeriod(Quantity43_50.convertQuantity(src.getPeriodDuration())); 015 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getSpecialPrecautionsForStorage()) 016 tgt.addSpecialPrecautionsForStorage(CodeableConcept43_50.convertCodeableConcept(t)); 017 return tgt; 018 } 019 020 public static org.hl7.fhir.r4b.model.ProductShelfLife convertProductShelfLife(org.hl7.fhir.r5.model.ProductShelfLife src) throws FHIRException { 021 if (src == null) return null; 022 org.hl7.fhir.r4b.model.ProductShelfLife tgt = new org.hl7.fhir.r4b.model.ProductShelfLife(); 023 BackboneElement43_50.copyBackboneElement(src, tgt); 024 if (src.hasType()) tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 025 if (src.hasPeriodDuration()) tgt.setPeriod(Quantity43_50.convertQuantity(src.getPeriodDuration())); 026 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialPrecautionsForStorage()) 027 tgt.addSpecialPrecautionsForStorage(CodeableConcept43_50.convertCodeableConcept(t)); 028 return tgt; 029 } 030}