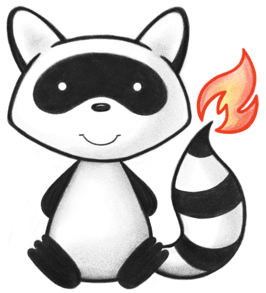
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableReference; 015import org.hl7.fhir.r5.model.Enumeration; 016import org.hl7.fhir.r5.model.Enumerations; 017import org.hl7.fhir.r5.model.ServiceRequest.ServiceRequestOrderDetailComponent; 018import org.hl7.fhir.r5.model.ServiceRequest.ServiceRequestOrderDetailParameterComponent; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class ServiceRequest43_50 { 050 051 public static org.hl7.fhir.r5.model.ServiceRequest convertServiceRequest(org.hl7.fhir.r4b.model.ServiceRequest src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r5.model.ServiceRequest tgt = new org.hl7.fhir.r5.model.ServiceRequest(); 055 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 058 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 059 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 060 for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 061 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 062 for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 063 for (org.hl7.fhir.r4b.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference43_50.convertReference(t)); 064 if (src.hasRequisition()) 065 tgt.setRequisition(Identifier43_50.convertIdentifier(src.getRequisition())); 066 if (src.hasStatus()) 067 tgt.setStatusElement(convertServiceRequestStatus(src.getStatusElement())); 068 if (src.hasIntent()) 069 tgt.setIntentElement(convertServiceRequestIntent(src.getIntentElement())); 070 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 071 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 072 if (src.hasPriority()) 073 tgt.setPriorityElement(convertServiceRequestPriority(src.getPriorityElement())); 074 if (src.hasDoNotPerform()) 075 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 076 if (src.hasCode()) 077 tgt.setCode(CodeableConcept43_50.convertCodeableConceptToCodeableReference(src.getCode())); 078 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getOrderDetail()) 079 tgt.addOrderDetail().addParameter().setValue(CodeableConcept43_50.convertCodeableConcept(t)); 080 if (src.hasQuantity()) 081 tgt.setQuantity(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getQuantity())); 082 if (src.hasSubject()) 083 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 084 if (src.hasEncounter()) 085 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 086 if (src.hasOccurrence()) 087 tgt.setOccurrence(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOccurrence())); 088 if (src.hasAsNeeded()) 089 tgt.setAsNeeded(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAsNeeded())); 090 if (src.hasAuthoredOn()) 091 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 092 if (src.hasRequester()) 093 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 094 if (src.hasPerformerType()) 095 tgt.setPerformerType(CodeableConcept43_50.convertCodeableConcept(src.getPerformerType())); 096 for (org.hl7.fhir.r4b.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference43_50.convertReference(t)); 097 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getLocationCode()) 098 tgt.addLocation(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 099 for (org.hl7.fhir.r4b.model.Reference t : src.getLocationReference()) 100 tgt.addLocation(Reference43_50.convertReferenceToCodeableReference(t)); 101 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 102 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 103 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 104 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 105 for (org.hl7.fhir.r4b.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 106 for (org.hl7.fhir.r4b.model.Reference t : src.getSupportingInfo()) 107 tgt.addSupportingInfo(Reference43_50.convertReferenceToCodeableReference(t)); 108 for (org.hl7.fhir.r4b.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference43_50.convertReference(t)); 109 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getBodySite()) 110 tgt.addBodySite(CodeableConcept43_50.convertCodeableConcept(t)); 111 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 112 if (src.hasPatientInstruction()) 113 tgt.addPatientInstruction().setInstruction(String43_50.convertStringToMarkdown(src.getPatientInstructionElement())); 114 for (org.hl7.fhir.r4b.model.Reference t : src.getRelevantHistory()) 115 tgt.addRelevantHistory(Reference43_50.convertReference(t)); 116 return tgt; 117 } 118 119 public static org.hl7.fhir.r4b.model.ServiceRequest convertServiceRequest(org.hl7.fhir.r5.model.ServiceRequest src) throws FHIRException { 120 if (src == null) 121 return null; 122 org.hl7.fhir.r4b.model.ServiceRequest tgt = new org.hl7.fhir.r4b.model.ServiceRequest(); 123 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 124 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 125 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 126 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 127 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 128 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 129 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 130 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 131 for (org.hl7.fhir.r5.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference43_50.convertReference(t)); 132 if (src.hasRequisition()) 133 tgt.setRequisition(Identifier43_50.convertIdentifier(src.getRequisition())); 134 if (src.hasStatus()) 135 tgt.setStatusElement(convertServiceRequestStatus(src.getStatusElement())); 136 if (src.hasIntent()) 137 tgt.setIntentElement(convertServiceRequestIntent(src.getIntentElement())); 138 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 139 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 140 if (src.hasPriority()) 141 tgt.setPriorityElement(convertServiceRequestPriority(src.getPriorityElement())); 142 if (src.hasDoNotPerform()) 143 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 144 if (src.hasCode()) 145 tgt.setCode(CodeableConcept43_50.convertCodeableReferenceToCodeableConcept(src.getCode())); 146 for (ServiceRequestOrderDetailComponent t : src.getOrderDetail()) { 147 for (ServiceRequestOrderDetailParameterComponent t1 : t.getParameter()) { 148 if (t1.hasValueCodeableConcept()) { 149 tgt.addOrderDetail(CodeableConcept43_50.convertCodeableConcept(t1.getValueCodeableConcept())); 150 } 151 } 152 } 153 if (src.hasQuantity()) 154 tgt.setQuantity(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getQuantity())); 155 if (src.hasSubject()) 156 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 157 if (src.hasEncounter()) 158 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 159 if (src.hasOccurrence()) 160 tgt.setOccurrence(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getOccurrence())); 161 if (src.hasAsNeeded()) 162 tgt.setAsNeeded(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAsNeeded())); 163 if (src.hasAuthoredOn()) 164 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 165 if (src.hasRequester()) 166 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 167 if (src.hasPerformerType()) 168 tgt.setPerformerType(CodeableConcept43_50.convertCodeableConcept(src.getPerformerType())); 169 for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference43_50.convertReference(t)); 170 for (CodeableReference t : src.getLocation()) 171 if (t.hasConcept()) 172 tgt.addLocationCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 173 for (CodeableReference t : src.getLocation()) 174 if (t.hasReference()) 175 tgt.addLocationReference(Reference43_50.convertReference(t.getReference())); 176 for (CodeableReference t : src.getReason()) 177 if (t.hasConcept()) 178 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 179 for (CodeableReference t : src.getReason()) 180 if (t.hasReference()) 181 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 182 for (org.hl7.fhir.r5.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 183 for (CodeableReference t : src.getSupportingInfo()) 184 if (t.hasReference()) 185 tgt.addSupportingInfo(Reference43_50.convertReference(t.getReference())); 186 for (org.hl7.fhir.r5.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference43_50.convertReference(t)); 187 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getBodySite()) 188 tgt.addBodySite(CodeableConcept43_50.convertCodeableConcept(t)); 189 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 190 if (src.getPatientInstructionFirstRep().hasInstructionMarkdownType()) 191 tgt.setPatientInstructionElement(String43_50.convertString(src.getPatientInstructionFirstRep().getInstructionMarkdownType())); 192 for (org.hl7.fhir.r5.model.Reference t : src.getRelevantHistory()) 193 tgt.addRelevantHistory(Reference43_50.convertReference(t)); 194 return tgt; 195 } 196 197 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertServiceRequestStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 Enumeration<Enumerations.RequestStatus> tgt = new Enumeration<>(new Enumerations.RequestStatusEnumFactory()); 201 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 202 if (src.getValue() == null) { 203 tgt.setValue(null); 204 } else { 205 switch (src.getValue()) { 206 case DRAFT: 207 tgt.setValue(Enumerations.RequestStatus.DRAFT); 208 break; 209 case ACTIVE: 210 tgt.setValue(Enumerations.RequestStatus.ACTIVE); 211 break; 212 case ONHOLD: 213 tgt.setValue(Enumerations.RequestStatus.ONHOLD); 214 break; 215 case REVOKED: 216 tgt.setValue(Enumerations.RequestStatus.REVOKED); 217 break; 218 case COMPLETED: 219 tgt.setValue(Enumerations.RequestStatus.COMPLETED); 220 break; 221 case ENTEREDINERROR: 222 tgt.setValue(Enumerations.RequestStatus.ENTEREDINERROR); 223 break; 224 case UNKNOWN: 225 tgt.setValue(Enumerations.RequestStatus.UNKNOWN); 226 break; 227 default: 228 tgt.setValue(Enumerations.RequestStatus.NULL); 229 break; 230 } 231 } 232 return tgt; 233 } 234 235 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> convertServiceRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 236 if (src == null || src.isEmpty()) 237 return null; 238 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestStatusEnumFactory()); 239 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 240 if (src.getValue() == null) { 241 tgt.setValue(null); 242 } else { 243 switch (src.getValue()) { 244 case DRAFT: 245 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.DRAFT); 246 break; 247 case ACTIVE: 248 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ACTIVE); 249 break; 250 case ONHOLD: 251 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ONHOLD); 252 break; 253 case REVOKED: 254 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.REVOKED); 255 break; 256 case COMPLETED: 257 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.COMPLETED); 258 break; 259 case ENTEREDINERROR: 260 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ENTEREDINERROR); 261 break; 262 case UNKNOWN: 263 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.UNKNOWN); 264 break; 265 default: 266 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.NULL); 267 break; 268 } 269 } 270 return tgt; 271 } 272 273 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> convertServiceRequestIntent(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> src) throws FHIRException { 274 if (src == null || src.isEmpty()) 275 return null; 276 Enumeration<Enumerations.RequestIntent> tgt = new Enumeration<>(new Enumerations.RequestIntentEnumFactory()); 277 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 278 if (src.getValue() == null) { 279 tgt.setValue(null); 280 } else { 281 switch (src.getValue()) { 282 case PROPOSAL: 283 tgt.setValue(Enumerations.RequestIntent.PROPOSAL); 284 break; 285 case PLAN: 286 tgt.setValue(Enumerations.RequestIntent.PLAN); 287 break; 288 case DIRECTIVE: 289 tgt.setValue(Enumerations.RequestIntent.DIRECTIVE); 290 break; 291 case ORDER: 292 tgt.setValue(Enumerations.RequestIntent.ORDER); 293 break; 294 case ORIGINALORDER: 295 tgt.setValue(Enumerations.RequestIntent.ORIGINALORDER); 296 break; 297 case REFLEXORDER: 298 tgt.setValue(Enumerations.RequestIntent.REFLEXORDER); 299 break; 300 case FILLERORDER: 301 tgt.setValue(Enumerations.RequestIntent.FILLERORDER); 302 break; 303 case INSTANCEORDER: 304 tgt.setValue(Enumerations.RequestIntent.INSTANCEORDER); 305 break; 306 case OPTION: 307 tgt.setValue(Enumerations.RequestIntent.OPTION); 308 break; 309 default: 310 tgt.setValue(Enumerations.RequestIntent.NULL); 311 break; 312 } 313 } 314 return tgt; 315 } 316 317 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> convertServiceRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> src) throws FHIRException { 318 if (src == null || src.isEmpty()) 319 return null; 320 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestIntentEnumFactory()); 321 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 322 if (src.getValue() == null) { 323 tgt.setValue(null); 324 } else { 325 switch (src.getValue()) { 326 case PROPOSAL: 327 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.PROPOSAL); 328 break; 329 case PLAN: 330 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.PLAN); 331 break; 332 case DIRECTIVE: 333 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.DIRECTIVE); 334 break; 335 case ORDER: 336 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.ORDER); 337 break; 338 case ORIGINALORDER: 339 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.ORIGINALORDER); 340 break; 341 case REFLEXORDER: 342 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.REFLEXORDER); 343 break; 344 case FILLERORDER: 345 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.FILLERORDER); 346 break; 347 case INSTANCEORDER: 348 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.INSTANCEORDER); 349 break; 350 case OPTION: 351 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.OPTION); 352 break; 353 default: 354 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.NULL); 355 break; 356 } 357 } 358 return tgt; 359 } 360 361 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertServiceRequestPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 362 if (src == null || src.isEmpty()) 363 return null; 364 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 365 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 366 if (src.getValue() == null) { 367 tgt.setValue(null); 368 } else { 369 switch (src.getValue()) { 370 case ROUTINE: 371 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 372 break; 373 case URGENT: 374 tgt.setValue(Enumerations.RequestPriority.URGENT); 375 break; 376 case ASAP: 377 tgt.setValue(Enumerations.RequestPriority.ASAP); 378 break; 379 case STAT: 380 tgt.setValue(Enumerations.RequestPriority.STAT); 381 break; 382 default: 383 tgt.setValue(Enumerations.RequestPriority.NULL); 384 break; 385 } 386 } 387 return tgt; 388 } 389 390 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertServiceRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 391 if (src == null || src.isEmpty()) 392 return null; 393 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 394 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 395 if (src.getValue() == null) { 396 tgt.setValue(null); 397 } else { 398 switch (src.getValue()) { 399 case ROUTINE: 400 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 401 break; 402 case URGENT: 403 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 404 break; 405 case ASAP: 406 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 407 break; 408 case STAT: 409 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 410 break; 411 default: 412 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 413 break; 414 } 415 } 416 return tgt; 417 } 418}