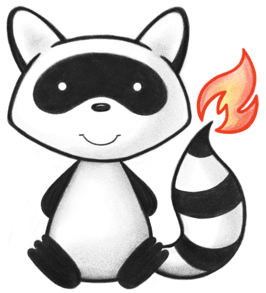
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Duration43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Enumeration; 014import org.hl7.fhir.r5.model.Specimen; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class Specimen43_50 { 046 047 public static org.hl7.fhir.r5.model.Specimen convertSpecimen(org.hl7.fhir.r4b.model.Specimen src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.Specimen tgt = new org.hl7.fhir.r5.model.Specimen(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 if (src.hasAccessionIdentifier()) 055 tgt.setAccessionIdentifier(Identifier43_50.convertIdentifier(src.getAccessionIdentifier())); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertSpecimenStatus(src.getStatusElement())); 058 if (src.hasType()) 059 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 060 if (src.hasSubject()) 061 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 062 if (src.hasReceivedTime()) 063 tgt.setReceivedTimeElement(DateTime43_50.convertDateTime(src.getReceivedTimeElement())); 064 for (org.hl7.fhir.r4b.model.Reference t : src.getParent()) tgt.addParent(Reference43_50.convertReference(t)); 065 for (org.hl7.fhir.r4b.model.Reference t : src.getRequest()) tgt.addRequest(Reference43_50.convertReference(t)); 066 if (src.hasCollection()) 067 tgt.setCollection(convertSpecimenCollectionComponent(src.getCollection())); 068 for (org.hl7.fhir.r4b.model.Specimen.SpecimenProcessingComponent t : src.getProcessing()) 069 tgt.addProcessing(convertSpecimenProcessingComponent(t)); 070 for (org.hl7.fhir.r4b.model.Specimen.SpecimenContainerComponent t : src.getContainer()) 071 tgt.addContainer(convertSpecimenContainerComponent(t)); 072 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCondition()) 073 tgt.addCondition(CodeableConcept43_50.convertCodeableConcept(t)); 074 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4b.model.Specimen convertSpecimen(org.hl7.fhir.r5.model.Specimen src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4b.model.Specimen tgt = new org.hl7.fhir.r4b.model.Specimen(); 082 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 083 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 084 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 085 if (src.hasAccessionIdentifier()) 086 tgt.setAccessionIdentifier(Identifier43_50.convertIdentifier(src.getAccessionIdentifier())); 087 if (src.hasStatus()) 088 tgt.setStatusElement(convertSpecimenStatus(src.getStatusElement())); 089 if (src.hasType()) 090 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 091 if (src.hasSubject()) 092 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 093 if (src.hasReceivedTime()) 094 tgt.setReceivedTimeElement(DateTime43_50.convertDateTime(src.getReceivedTimeElement())); 095 for (org.hl7.fhir.r5.model.Reference t : src.getParent()) tgt.addParent(Reference43_50.convertReference(t)); 096 for (org.hl7.fhir.r5.model.Reference t : src.getRequest()) tgt.addRequest(Reference43_50.convertReference(t)); 097 if (src.hasCollection()) 098 tgt.setCollection(convertSpecimenCollectionComponent(src.getCollection())); 099 for (org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent t : src.getProcessing()) 100 tgt.addProcessing(convertSpecimenProcessingComponent(t)); 101 for (org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent t : src.getContainer()) 102 tgt.addContainer(convertSpecimenContainerComponent(t)); 103 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCondition()) 104 tgt.addCondition(CodeableConcept43_50.convertCodeableConcept(t)); 105 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Specimen.SpecimenStatus> convertSpecimenStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Specimen.SpecimenStatus> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 Enumeration<Specimen.SpecimenStatus> tgt = new Enumeration<>(new Specimen.SpecimenStatusEnumFactory()); 113 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 114 if (src.getValue() == null) { 115 tgt.setValue(null); 116 } else { 117 switch (src.getValue()) { 118 case AVAILABLE: 119 tgt.setValue(Specimen.SpecimenStatus.AVAILABLE); 120 break; 121 case UNAVAILABLE: 122 tgt.setValue(Specimen.SpecimenStatus.UNAVAILABLE); 123 break; 124 case UNSATISFACTORY: 125 tgt.setValue(Specimen.SpecimenStatus.UNSATISFACTORY); 126 break; 127 case ENTEREDINERROR: 128 tgt.setValue(Specimen.SpecimenStatus.ENTEREDINERROR); 129 break; 130 default: 131 tgt.setValue(Specimen.SpecimenStatus.NULL); 132 break; 133 } 134 } 135 return tgt; 136 } 137 138 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Specimen.SpecimenStatus> convertSpecimenStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Specimen.SpecimenStatus> src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Specimen.SpecimenStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Specimen.SpecimenStatusEnumFactory()); 142 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 143 if (src.getValue() == null) { 144 tgt.setValue(null); 145 } else { 146 switch (src.getValue()) { 147 case AVAILABLE: 148 tgt.setValue(org.hl7.fhir.r4b.model.Specimen.SpecimenStatus.AVAILABLE); 149 break; 150 case UNAVAILABLE: 151 tgt.setValue(org.hl7.fhir.r4b.model.Specimen.SpecimenStatus.UNAVAILABLE); 152 break; 153 case UNSATISFACTORY: 154 tgt.setValue(org.hl7.fhir.r4b.model.Specimen.SpecimenStatus.UNSATISFACTORY); 155 break; 156 case ENTEREDINERROR: 157 tgt.setValue(org.hl7.fhir.r4b.model.Specimen.SpecimenStatus.ENTEREDINERROR); 158 break; 159 default: 160 tgt.setValue(org.hl7.fhir.r4b.model.Specimen.SpecimenStatus.NULL); 161 break; 162 } 163 } 164 return tgt; 165 } 166 167 public static org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent convertSpecimenCollectionComponent(org.hl7.fhir.r4b.model.Specimen.SpecimenCollectionComponent src) throws FHIRException { 168 if (src == null) 169 return null; 170 org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent(); 171 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 172 if (src.hasCollector()) 173 tgt.setCollector(Reference43_50.convertReference(src.getCollector())); 174 if (src.hasCollected()) 175 tgt.setCollected(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getCollected())); 176 if (src.hasDuration()) 177 tgt.setDuration(Duration43_50.convertDuration(src.getDuration())); 178 if (src.hasQuantity()) 179 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 180 if (src.hasMethod()) 181 tgt.setMethod(CodeableConcept43_50.convertCodeableConcept(src.getMethod())); 182 if (src.hasBodySite()) 183 tgt.getBodySite().setConcept(CodeableConcept43_50.convertCodeableConcept(src.getBodySite())); 184 if (src.hasFastingStatus()) 185 tgt.setFastingStatus(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getFastingStatus())); 186 return tgt; 187 } 188 189 public static org.hl7.fhir.r4b.model.Specimen.SpecimenCollectionComponent convertSpecimenCollectionComponent(org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent src) throws FHIRException { 190 if (src == null) 191 return null; 192 org.hl7.fhir.r4b.model.Specimen.SpecimenCollectionComponent tgt = new org.hl7.fhir.r4b.model.Specimen.SpecimenCollectionComponent(); 193 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 194 if (src.hasCollector()) 195 tgt.setCollector(Reference43_50.convertReference(src.getCollector())); 196 if (src.hasCollected()) 197 tgt.setCollected(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getCollected())); 198 if (src.hasDuration()) 199 tgt.setDuration(Duration43_50.convertDuration(src.getDuration())); 200 if (src.hasQuantity()) 201 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 202 if (src.hasMethod()) 203 tgt.setMethod(CodeableConcept43_50.convertCodeableConcept(src.getMethod())); 204 if (src.getBodySite().hasConcept()) 205 tgt.setBodySite(CodeableConcept43_50.convertCodeableConcept(src.getBodySite().getConcept())); 206 if (src.hasFastingStatus()) 207 tgt.setFastingStatus(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getFastingStatus())); 208 return tgt; 209 } 210 211 public static org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent convertSpecimenProcessingComponent(org.hl7.fhir.r4b.model.Specimen.SpecimenProcessingComponent src) throws FHIRException { 212 if (src == null) 213 return null; 214 org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent(); 215 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 216 if (src.hasDescription()) 217 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 218// if (src.hasProcedure()) 219// tgt.setProcedure(CodeableConcept43_50.convertCodeableConcept(src.getProcedure())); 220 for (org.hl7.fhir.r4b.model.Reference t : src.getAdditive()) tgt.addAdditive(Reference43_50.convertReference(t)); 221 if (src.hasTime()) 222 tgt.setTime(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTime())); 223 return tgt; 224 } 225 226 public static org.hl7.fhir.r4b.model.Specimen.SpecimenProcessingComponent convertSpecimenProcessingComponent(org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent src) throws FHIRException { 227 if (src == null) 228 return null; 229 org.hl7.fhir.r4b.model.Specimen.SpecimenProcessingComponent tgt = new org.hl7.fhir.r4b.model.Specimen.SpecimenProcessingComponent(); 230 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 231 if (src.hasDescription()) 232 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 233// if (src.hasProcedure()) 234// tgt.setProcedure(CodeableConcept43_50.convertCodeableConcept(src.getProcedure())); 235 for (org.hl7.fhir.r5.model.Reference t : src.getAdditive()) tgt.addAdditive(Reference43_50.convertReference(t)); 236 if (src.hasTime()) 237 tgt.setTime(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTime())); 238 return tgt; 239 } 240 241 public static org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent convertSpecimenContainerComponent(org.hl7.fhir.r4b.model.Specimen.SpecimenContainerComponent src) throws FHIRException { 242 if (src == null) 243 return null; 244 org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent(); 245 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 246// for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 247// tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 248// if (src.hasDescription()) 249// tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 250// if (src.hasType()) 251// tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 252// if (src.hasCapacity()) 253// tgt.setCapacity(SimpleQuantity43_50.convertSimpleQuantity(src.getCapacity())); 254// if (src.hasSpecimenQuantity()) 255// tgt.setSpecimenQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getSpecimenQuantity())); 256// if (src.hasAdditive()) 257// tgt.setAdditive(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAdditive())); 258 return tgt; 259 } 260 261 public static org.hl7.fhir.r4b.model.Specimen.SpecimenContainerComponent convertSpecimenContainerComponent(org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent src) throws FHIRException { 262 if (src == null) 263 return null; 264 org.hl7.fhir.r4b.model.Specimen.SpecimenContainerComponent tgt = new org.hl7.fhir.r4b.model.Specimen.SpecimenContainerComponent(); 265 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 266// for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 267// tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 268// if (src.hasDescription()) 269// tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 270// if (src.hasType()) 271// tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 272// if (src.hasCapacity()) 273// tgt.setCapacity(SimpleQuantity43_50.convertSimpleQuantity(src.getCapacity())); 274// if (src.hasSpecimenQuantity()) 275// tgt.setSpecimenQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getSpecimenQuantity())); 276// if (src.hasAdditive()) 277// tgt.setAdditive(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getAdditive())); 278 return tgt; 279 } 280}