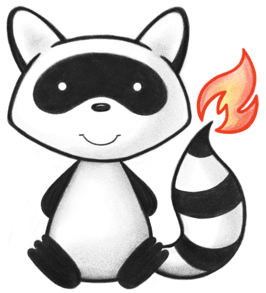
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import java.util.List; 004import java.util.stream.Collectors; 005import java.util.stream.Stream; 006 007import org.hl7.fhir.convertors.context.ConversionContext43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 019import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 020import org.hl7.fhir.r4b.model.SubscriptionTopic; 021 022public class SubscriptionTopic43_50 { 023 024 025 public static final String NAME_EXTENSION_URL = "http://hl7.org/fhir/5.0/StructureDefinition/extension-SubscriptionTopic.name"; 026 public static final String COPYRIGHT_LABEL_EXTENSION_URL = "http://hl7.org/fhir/5.0/StructureDefinition/extension-SubscriptionTopic.copyrightLabel"; 027 028 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 029 NAME_EXTENSION_URL, 030 COPYRIGHT_LABEL_EXTENSION_URL 031 }; 032 033 public static org.hl7.fhir.r4b.model.SubscriptionTopic convertSubscriptionTopic(org.hl7.fhir.r5.model.SubscriptionTopic src) { 034 if (src == null) 035 return null; 036 org.hl7.fhir.r4b.model.SubscriptionTopic tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic(); 037 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 038 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 039 if (src.hasUrl()) 040 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 041 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 042 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 043 if (src.hasTitle()) 044 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 045 for (org.hl7.fhir.r5.model.CanonicalType t : src.getDerivedFrom()) 046 tgt.getDerivedFrom().add(Canonical43_50.convertCanonical(t)); 047 if (src.hasStatus()) 048 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 049 if (src.hasVersion()) 050 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 051 if (src.hasTitle()) 052 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 053 if (src.hasStatus()) 054 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 055 if (src.hasExperimental()) 056 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 057 if (src.hasDate()) 058 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 059 if (src.hasPublisher()) 060 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 061 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 062 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 063 if (src.hasDescription()) 064 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 065 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 066 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 067 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 068 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 069 if (src.hasPurpose()) 070 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 071 if (src.hasCopyright()) 072 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 073 if (src.hasCopyrightLabel()) { 074 tgt.addExtension(COPYRIGHT_LABEL_EXTENSION_URL, String43_50.convertString(src.getCopyrightLabelElement())); 075 } 076 if (src.hasName()) { 077 tgt.addExtension(NAME_EXTENSION_URL, String43_50.convertString(src.getNameElement())); 078 } 079 if (src.hasApprovalDate()) 080 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 081 if (src.hasLastReviewDate()) 082 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 083 if (src.hasEffectivePeriod()) 084 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 085 for(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent triggerComponent : src.getResourceTrigger()) { 086 tgt.addResourceTrigger(convertResourceTrigger(triggerComponent));} 087 for (org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent canFilterByComponent : src.getCanFilterBy()) { 088 tgt.addCanFilterBy(convertCanFilterBy(canFilterByComponent)); 089 } 090 for (org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent eventTrigger : src.getEventTrigger()) { 091 tgt.addEventTrigger(convertEventTrigger(eventTrigger)); 092 } 093 for (org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent notificationShape : src.getNotificationShape()) { 094 tgt.addNotificationShape(convertNotificationShape(notificationShape)); 095 } 096 return tgt; 097 } 098 099 private static SubscriptionTopic.SubscriptionTopicEventTriggerComponent convertEventTrigger(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent src) { 100 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent(); 101 if (src.hasDescription()) { 102 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 103 } 104 if (src.hasEvent()) { 105 tgt.setEvent(CodeableConcept43_50.convertCodeableConcept(src.getEvent())); 106 } 107 if (src.hasResource()) { 108 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 109 } 110 return tgt; 111 } 112 113 private static org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent convertNotificationShape(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent src) { 114 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent(); 115 if (src.hasResource()) { 116 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 117 } 118 if (src.hasInclude()) { 119 tgt.setInclude(src.getInclude().stream().map(String43_50::convertString).collect(Collectors.toList())); 120 } 121 if (src.hasRevInclude()) { 122 tgt.setRevInclude(src.getRevInclude().stream().map(String43_50::convertString).collect(Collectors.toList())); 123 } 124 return tgt; 125 } 126 127 private static org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent convertCanFilterBy(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent src) { 128 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent(); 129 if (src.hasDescription()) { 130 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 131 } 132 if (src.hasResource()) { 133 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 134 } 135 if (src.hasFilterParameter()) { 136 tgt.setFilterParameterElement(String43_50.convertString(src.getFilterParameterElement())); 137 } 138 if (src.hasFilterDefinition()) { 139 // TODO r4b spec has this, but the Java model does not 140 } 141 if (src.hasModifier() || src.hasComparator()) { 142 List<org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> tgtModifiers = convertR5ModifierToR4BModifier(src.getModifier()); 143 144 List<org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> tgtComparatorModifiers = convertR5ComparatorToR4BModifier(src.getComparator()); 145 146 tgt.setModifier(Stream.concat(tgtModifiers.stream(), tgtComparatorModifiers.stream()) 147 .collect(Collectors.toList())); 148 } 149 150 return tgt; 151 } 152 153 private static List<org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> convertR5ComparatorToR4BModifier(List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator>> srcList) { 154 return srcList.stream().map(SubscriptionTopic43_50::convertR5ComparatorToR4BModifier).filter(x -> x != null).collect(Collectors.toList()); 155 } 156 157 private static org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier> convertR5ComparatorToR4BModifier(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> src){ 158 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifierEnumFactory enumFactory = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifierEnumFactory(); 159 switch(src.getValue()) { 160 case NULL: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.NULL); 161 case EQ: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.EQ); 162 case NE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.NE); 163 case GT: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.GT); 164 case LT: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.LT); 165 case GE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.GE); 166 case LE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.LE); 167 case SA: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.SA); 168 case EB: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.EB); 169 case AP: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.AP); 170 } 171 return null; 172 } 173 174 private static List<org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> convertR5ModifierToR4BModifier(List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode>> srcList) { 175 return srcList.stream().map(SubscriptionTopic43_50::convertR5ModifierToR4BModifier).filter(x -> x != null).collect(Collectors.toList()); 176 } 177 178 private static org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier> convertR5ModifierToR4BModifier(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> src){ 179 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifierEnumFactory enumFactory = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifierEnumFactory(); 180 switch(src.getValue()) { 181 case NULL: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.NULL); 182 case IN: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.IN); 183 case NOTIN: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.NOTIN); 184 case BELOW: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.BELOW); 185 case ABOVE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.ABOVE); 186 case OFTYPE: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier.OFTYPE); 187 } 188 return null; 189 } 190 private static org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent convertResourceTrigger(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent src) { 191 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent(); 192 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 193 if (src.hasDescription()) { 194 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 195 } 196 if (src.hasResource()) { 197 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 198 } 199 if (src.hasSupportedInteraction()) { 200 for (org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger> srcItem : src.getSupportedInteraction()) { 201 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger> newElement = tgt.addSupportedInteractionElement(); 202 newElement.setValue(convertInteractionTrigger(srcItem.getValue())); 203 } 204 } 205 if (src.hasQueryCriteria()) { 206 tgt.setQueryCriteria(convertResourceTriggerQueryCriteriaComponent(src.getQueryCriteria())); 207 } 208 if (src.hasFhirPathCriteria()) { 209 tgt.setFhirPathCriteriaElement(String43_50.convertString(src.getFhirPathCriteriaElement())); 210 } 211 return tgt; 212 } 213 214 private static org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent convertResourceTriggerQueryCriteriaComponent(org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent src) { 215 org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent tgt = new org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 216 if (src.hasPrevious()) { 217 tgt.setPreviousElement(String43_50.convertString(src.getPreviousElement())); 218 } 219 if (src.hasResultForCreate()) { 220 tgt.setResultForCreateElement(convertCriteriaNotExistsBehavior(src.getResultForCreateElement())); 221 } 222 if (src.hasCurrent()) { 223 tgt.setCurrentElement(String43_50.convertString(src.getCurrentElement())); 224 } 225 if (src.hasResultForDelete()) { 226 tgt.setResultForDeleteElement(convertCriteriaNotExistsBehavior(src.getResultForDeleteElement())); 227 } 228 if (src.hasRequireBoth()) { 229 tgt.setRequireBothElement(Boolean43_50.convertBoolean(src.getRequireBothElement())); 230 } 231 232 return tgt; 233 } 234 235 private static org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehavior> convertCriteriaNotExistsBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehavior> src) { 236 org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehaviorEnumFactory enumFactory = new org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehaviorEnumFactory(); 237 switch(src.getValue()) { 238 case TESTFAILS: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehavior.TESTFAILS); 239 case TESTPASSES: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehavior.TESTPASSES); 240 case NULL: return new org.hl7.fhir.r4b.model.Enumeration<>(enumFactory, org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehavior.NULL); 241 } 242 return null; 243 } 244 245 private static org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger convertInteractionTrigger(org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger value) { 246 switch(value) { 247 case CREATE : return org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger.CREATE; 248 case UPDATE: return org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger.UPDATE; 249 case DELETE: return org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger.DELETE; 250 case NULL: return org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger.NULL; 251 } 252 return null; 253 } 254 255 256 257 public static org.hl7.fhir.r5.model.SubscriptionTopic convertSubscriptionTopic(org.hl7.fhir.r4b.model.SubscriptionTopic src) { 258 if (src == null) 259 return null; 260 org.hl7.fhir.r5.model.SubscriptionTopic tgt = new org.hl7.fhir.r5.model.SubscriptionTopic(); 261 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 262 263 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 264 265 if (src.hasExtension(NAME_EXTENSION_URL)) { 266 tgt.setNameElement(String43_50.convertString((org.hl7.fhir.r4b.model.StringType) src.getExtensionByUrl(NAME_EXTENSION_URL).getValue())); 267 } 268 if (src.hasExtension(COPYRIGHT_LABEL_EXTENSION_URL)) { 269 tgt.setCopyrightLabelElement(String43_50.convertString((org.hl7.fhir.r4b.model.StringType) src.getExtensionByUrl(COPYRIGHT_LABEL_EXTENSION_URL).getValue())); 270 } 271 if (src.hasUrl()) 272 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 273 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 274 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 275 if (src.hasTitle()) 276 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 277 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getDerivedFrom()) 278 tgt.getDerivedFrom().add(Canonical43_50.convertCanonical(t)); 279 if (src.hasStatus()) 280 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 281 if (src.hasVersion()) 282 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 283 if (src.hasTitle()) 284 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 285 if (src.hasStatus()) 286 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 287 if (src.hasExperimental()) 288 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 289 if (src.hasDate()) 290 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 291 if (src.hasPublisher()) 292 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 293 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 294 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 295 if (src.hasDescription()) 296 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 297 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 298 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 299 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 300 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 301 if (src.hasPurpose()) 302 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 303 if (src.hasCopyright()) 304 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 305 if (src.hasApprovalDate()) 306 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 307 if (src.hasLastReviewDate()) 308 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 309 if (src.hasEffectivePeriod()) 310 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 311 for(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent triggerComponent : src.getResourceTrigger()) { 312 tgt.addResourceTrigger(convertResourceTrigger(triggerComponent)); 313 } 314 for (org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent eventTrigger : src.getEventTrigger()) { 315 tgt.addEventTrigger(convertEventTrigger(eventTrigger)); 316 } 317 for (org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent canFilterByComponent : src.getCanFilterBy()) { 318 tgt.addCanFilterBy(convertCanFilterBy(canFilterByComponent)); 319 } 320 for (org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent notificationShape : src.getNotificationShape()) { 321 tgt.addNotificationShape(convertNotificationShape(notificationShape)); 322 } 323 return tgt; 324 } 325 326 private static org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent convertNotificationShape(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent src) { 327 org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent tgt = new org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicNotificationShapeComponent(); 328 if (src.hasResource()) { 329 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 330 } 331 if (src.hasInclude()) { 332 tgt.setInclude(src.getInclude().stream().map(String43_50::convertString).collect(Collectors.toList())); 333 } 334 if (src.hasRevInclude()) { 335 tgt.setRevInclude(src.getRevInclude().stream().map(String43_50::convertString).collect(Collectors.toList())); 336 } 337 return tgt; 338 339 } 340 341 private static org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent convertEventTrigger(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent src) { 342 org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent tgt = new org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicEventTriggerComponent(); 343 if (src.hasDescription()) { 344 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 345 } 346 if (src.hasEvent()) { 347 tgt.setEvent(CodeableConcept43_50.convertCodeableConcept(src.getEvent())); 348 } 349 if (src.hasResource()) { 350 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 351 } 352 return tgt; 353 } 354 355 private static org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent convertCanFilterBy(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent src) { 356 org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent tgt = new org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicCanFilterByComponent(); 357 if (src.hasDescription()) { 358 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 359 } 360 if (src.hasResource()) { 361 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 362 } 363 if (src.hasFilterParameter()) { 364 tgt.setFilterParameterElement(String43_50.convertString(src.getFilterParameterElement())); 365 } 366 367 if (src.hasModifier()) { 368 List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode>> tgtModifiers = convertR4BModifierToR5Modifier(src.getModifier()); 369 tgt.setModifier(tgtModifiers); 370 371 List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator>> tgtComparators = covertR4BModifierToR5Comparator(src.getModifier()); 372 tgt.setComparator(tgtComparators); 373 } 374 375 return tgt; 376 } 377 378 private static List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator>> covertR4BModifierToR5Comparator(List<org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> srcList) { 379 return srcList.stream().map(SubscriptionTopic43_50::convertR4BModifierToR5Comparator).filter(x -> x != null).collect(Collectors.toList()); 380 } 381 382 383 private static org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> convertR4BModifierToR5Comparator(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier> src) { 384 org.hl7.fhir.r5.model.Enumerations.SearchComparatorEnumFactory enumFactory = new org.hl7.fhir.r5.model.Enumerations.SearchComparatorEnumFactory(); 385 switch(src.getValue()) { 386 case NULL: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.NULL); 387 case EQ: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.EQ); 388 case NE: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.NE); 389 case GT: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.GT); 390 case LT: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.LT); 391 case GE: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.GE); 392 case LE: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.LE); 393 case SA: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.SA); 394 case EB: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.EB); 395 case AP: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchComparator.AP); 396 } 397 return null; 398 } 399 400 private static List<org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode>> convertR4BModifierToR5Modifier( 401 List 402 <org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier>> srcList) { 403 return srcList.stream().map(SubscriptionTopic43_50::convertR4BModifierToR5Modifier).filter(x -> x != null).collect(Collectors.toList()); 404 } 405 private static org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> convertR4BModifierToR5Modifier(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionSearchModifier> src) { 406 org.hl7.fhir.r5.model.Enumerations.SearchModifierCodeEnumFactory enumFactory = new org.hl7.fhir.r5.model.Enumerations.SearchModifierCodeEnumFactory(); 407 switch(src.getValue()) { 408 case NULL: 409 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.NULL); 410 case IN: 411 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.IN); 412 case NOTIN: 413 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.NOTIN); 414 case BELOW: 415 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.BELOW); 416 case ABOVE: 417 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.ABOVE); 418 case OFTYPE: 419 return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.Enumerations.SearchModifierCode.OFTYPE); 420 } 421 return null; 422 } 423 424 private static org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent convertResourceTrigger(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent src) { 425 org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent tgt = new org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerComponent(); 426 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 427 if (src.hasDescription()) { 428 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 429 } 430 if (src.hasResource()) { 431 tgt.setResourceElement(Uri43_50.convertUri(src.getResourceElement())); 432 } 433 if (src.hasSupportedInteraction()) { 434 for (org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger> srcItem : src.getSupportedInteraction()) { 435 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger> tgtElement = tgt.addSupportedInteractionElement(); 436 tgtElement.setValue(convertInteractionTrigger(srcItem.getValue())); 437 } 438 } 439 if (src.hasQueryCriteria()) { 440 tgt.setQueryCriteria(convertResourceTriggerQueryCriteriaComponent(src.getQueryCriteria())); 441 } 442 if (src.hasFhirPathCriteria()) { 443 tgt.setFhirPathCriteriaElement(String43_50.convertString(src.getFhirPathCriteriaElement())); 444 } 445 return tgt; 446 447 } 448 449 private static org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent convertResourceTriggerQueryCriteriaComponent(org.hl7.fhir.r4b.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent src) { 450 org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent tgt = new org.hl7.fhir.r5.model.SubscriptionTopic.SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 451 if (src.hasPrevious()) { 452 tgt.setPreviousElement(String43_50.convertString(src.getPreviousElement())); 453 } 454 if (src.hasResultForCreate()) { 455 tgt.setResultForCreateElement(convertCriteriaNotExistsBehavior(src.getResultForCreateElement())); 456 } 457 if (src.hasCurrent()) { 458 tgt.setCurrentElement(String43_50.convertString(src.getCurrentElement())); 459 } 460 if (src.hasResultForDelete()) { 461 tgt.setResultForDeleteElement(convertCriteriaNotExistsBehavior(src.getResultForDeleteElement())); 462 } 463 if (src.hasRequireBoth()) { 464 tgt.setRequireBothElement(Boolean43_50.convertBoolean(src.getRequireBothElement())); 465 } 466 return tgt; 467 } 468 469 private static org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehavior> convertCriteriaNotExistsBehavior(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.SubscriptionTopic.CriteriaNotExistsBehavior> src) { 470 org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehaviorEnumFactory enumFactory = new org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehaviorEnumFactory(); 471 switch(src.getValue()) { 472 473 case TESTFAILS: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehavior.TESTFAILS); 474 case TESTPASSES: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehavior.TESTPASSES); 475 case NULL: return new org.hl7.fhir.r5.model.Enumeration<>(enumFactory, org.hl7.fhir.r5.model.SubscriptionTopic.CriteriaNotExistsBehavior.NULL); 476 } 477 return null; 478 } 479 480 private static org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger convertInteractionTrigger(org.hl7.fhir.r4b.model.SubscriptionTopic.InteractionTrigger value) { 481 switch(value) { 482 case CREATE : return org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger.CREATE; 483 case UPDATE: return org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger.UPDATE; 484 case DELETE: return org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger.DELETE; 485 case NULL: return org.hl7.fhir.r5.model.SubscriptionTopic.InteractionTrigger.NULL; 486 } 487 return null; 488 489 } 490}