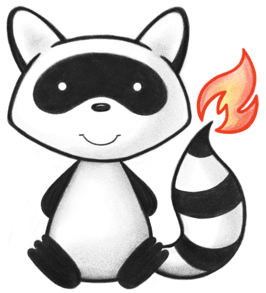
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Ratio43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Enumeration; 012import org.hl7.fhir.r5.model.Identifier; 013import org.hl7.fhir.r5.model.Substance; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Substance43_50 { 045 046 public static org.hl7.fhir.r5.model.Substance convertSubstance(org.hl7.fhir.r4b.model.Substance src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Substance tgt = new org.hl7.fhir.r5.model.Substance(); 050 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 055 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 056 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 057 if (src.hasCode()) 058 tgt.getCode().setConcept(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 059 if (src.hasDescription()) 060 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 061 for (org.hl7.fhir.r4b.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 062 convertSubstanceInstanceComponent(t, tgt); 063 for (org.hl7.fhir.r4b.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 064 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 065 return tgt; 066 } 067 068 public static org.hl7.fhir.r4b.model.Substance convertSubstance(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 069 if (src == null) 070 return null; 071 org.hl7.fhir.r4b.model.Substance tgt = new org.hl7.fhir.r4b.model.Substance(); 072 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 073 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 074 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 075 if (src.hasStatus()) 076 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 077 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 078 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 079 if (src.getCode().hasConcept()) 080 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode().getConcept())); 081 if (src.hasDescription()) 082 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 083 if (src.getInstance()) { 084 tgt.addInstance(convertSubstanceInstanceComponent(src)); 085 } 086 for (org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 087 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 088 return tgt; 089 } 090 091 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 Enumeration<Substance.FHIRSubstanceStatus> tgt = new Enumeration<>(new Substance.FHIRSubstanceStatusEnumFactory()); 095 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 096 if (src.getValue() == null) { 097 tgt.setValue(null); 098 } else { 099 switch (src.getValue()) { 100 case ACTIVE: 101 tgt.setValue(Substance.FHIRSubstanceStatus.ACTIVE); 102 break; 103 case INACTIVE: 104 tgt.setValue(Substance.FHIRSubstanceStatus.INACTIVE); 105 break; 106 case ENTEREDINERROR: 107 tgt.setValue(Substance.FHIRSubstanceStatus.ENTEREDINERROR); 108 break; 109 default: 110 tgt.setValue(Substance.FHIRSubstanceStatus.NULL); 111 break; 112 } 113 } 114 return tgt; 115 } 116 117 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 118 if (src == null || src.isEmpty()) 119 return null; 120 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatusEnumFactory()); 121 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 122 if (src.getValue() == null) { 123 tgt.setValue(null); 124 } else { 125 switch (src.getValue()) { 126 case ACTIVE: 127 tgt.setValue(org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus.ACTIVE); 128 break; 129 case INACTIVE: 130 tgt.setValue(org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus.INACTIVE); 131 break; 132 case ENTEREDINERROR: 133 tgt.setValue(org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 134 break; 135 default: 136 tgt.setValue(org.hl7.fhir.r4b.model.Substance.FHIRSubstanceStatus.NULL); 137 break; 138 } 139 } 140 return tgt; 141 } 142 143 public static void convertSubstanceInstanceComponent(org.hl7.fhir.r4b.model.Substance.SubstanceInstanceComponent src, org.hl7.fhir.r5.model.Substance tgt) throws FHIRException { 144 tgt.setInstance(true); 145 if (src.hasIdentifier()) 146 tgt.addIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 147 if (src.hasExpiry()) 148 tgt.setExpiryElement(DateTime43_50.convertDateTime(src.getExpiryElement())); 149 if (src.hasQuantity()) 150 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 151 } 152 153 public static org.hl7.fhir.r4b.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 154 org.hl7.fhir.r4b.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.r4b.model.Substance.SubstanceInstanceComponent(); 155 for (Identifier t : src.getIdentifier()) { 156 tgt.setIdentifier(Identifier43_50.convertIdentifier(t)); 157 } 158 if (src.hasExpiry()) 159 tgt.setExpiryElement(DateTime43_50.convertDateTime(src.getExpiryElement())); 160 if (src.hasQuantity()) 161 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 162 return tgt; 163 } 164 165 public static org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r4b.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 166 if (src == null) 167 return null; 168 org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent(); 169 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 170 if (src.hasQuantity()) 171 tgt.setQuantity(Ratio43_50.convertRatio(src.getQuantity())); 172 if (src.hasSubstance()) 173 tgt.setSubstance(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubstance())); 174 return tgt; 175 } 176 177 public static org.hl7.fhir.r4b.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 178 if (src == null) 179 return null; 180 org.hl7.fhir.r4b.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r4b.model.Substance.SubstanceIngredientComponent(); 181 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 182 if (src.hasQuantity()) 183 tgt.setQuantity(Ratio43_50.convertRatio(src.getQuantity())); 184 if (src.hasSubstance()) 185 tgt.setSubstance(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubstance())); 186 return tgt; 187 } 188}