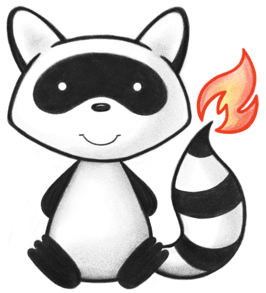
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.PositiveInt43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.CodeableReference; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.Enumerations; 018import org.hl7.fhir.r5.model.Task; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class Task43_50 { 050 051 public static org.hl7.fhir.r5.model.Task convertTask(org.hl7.fhir.r4b.model.Task src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r5.model.Task tgt = new org.hl7.fhir.r5.model.Task(); 055 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 058 if (src.hasInstantiatesCanonical()) 059 tgt.setInstantiatesCanonicalElement(Canonical43_50.convertCanonical(src.getInstantiatesCanonicalElement())); 060 if (src.hasInstantiatesUri()) 061 tgt.setInstantiatesUriElement(Uri43_50.convertUri(src.getInstantiatesUriElement())); 062 for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 063 if (src.hasGroupIdentifier()) 064 tgt.setGroupIdentifier(Identifier43_50.convertIdentifier(src.getGroupIdentifier())); 065 for (org.hl7.fhir.r4b.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 066 if (src.hasStatus()) 067 tgt.setStatusElement(convertTaskStatus(src.getStatusElement())); 068 if (src.hasStatusReason()) 069 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(src.getStatusReason())); 070 if (src.hasBusinessStatus()) 071 tgt.setBusinessStatus(CodeableConcept43_50.convertCodeableConcept(src.getBusinessStatus())); 072 if (src.hasIntent()) 073 tgt.setIntentElement(convertTaskIntent(src.getIntentElement())); 074 if (src.hasPriority()) 075 tgt.setPriorityElement(convertTaskPriority(src.getPriorityElement())); 076 if (src.hasCode()) 077 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 078 if (src.hasDescription()) 079 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 080 if (src.hasFocus()) 081 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 082 if (src.hasFor()) 083 tgt.setFor(Reference43_50.convertReference(src.getFor())); 084 if (src.hasEncounter()) 085 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 086 if (src.hasExecutionPeriod()) 087 tgt.setExecutionPeriod(Period43_50.convertPeriod(src.getExecutionPeriod())); 088 if (src.hasAuthoredOn()) 089 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 090 if (src.hasLastModified()) 091 tgt.setLastModifiedElement(DateTime43_50.convertDateTime(src.getLastModifiedElement())); 092 if (src.hasRequester()) 093 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 094 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getPerformerType()) 095 tgt.addRequestedPerformer(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 096 if (src.hasOwner()) 097 tgt.setOwner(Reference43_50.convertReference(src.getOwner())); 098 if (src.hasLocation()) 099 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 100 if (src.hasReasonCode()) 101 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(src.getReasonCode())); 102 if (src.hasReasonReference()) 103 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(src.getReasonReference())); 104 for (org.hl7.fhir.r4b.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 105 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 106 for (org.hl7.fhir.r4b.model.Reference t : src.getRelevantHistory()) 107 tgt.addRelevantHistory(Reference43_50.convertReference(t)); 108 if (src.hasRestriction()) 109 tgt.setRestriction(convertTaskRestrictionComponent(src.getRestriction())); 110 for (org.hl7.fhir.r4b.model.Task.ParameterComponent t : src.getInput()) tgt.addInput(convertParameterComponent(t)); 111 for (org.hl7.fhir.r4b.model.Task.TaskOutputComponent t : src.getOutput()) 112 tgt.addOutput(convertTaskOutputComponent(t)); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.r4b.model.Task convertTask(org.hl7.fhir.r5.model.Task src) throws FHIRException { 117 if (src == null) 118 return null; 119 org.hl7.fhir.r4b.model.Task tgt = new org.hl7.fhir.r4b.model.Task(); 120 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 121 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 122 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 123 if (src.hasInstantiatesCanonical()) 124 tgt.setInstantiatesCanonicalElement(Canonical43_50.convertCanonical(src.getInstantiatesCanonicalElement())); 125 if (src.hasInstantiatesUri()) 126 tgt.setInstantiatesUriElement(Uri43_50.convertUri(src.getInstantiatesUriElement())); 127 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 128 if (src.hasGroupIdentifier()) 129 tgt.setGroupIdentifier(Identifier43_50.convertIdentifier(src.getGroupIdentifier())); 130 for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 131 if (src.hasStatus()) 132 tgt.setStatusElement(convertTaskStatus(src.getStatusElement())); 133 if (src.hasStatusReason()) 134 tgt.setStatusReason(CodeableConcept43_50.convertCodeableReferenceToCodeableConcept(src.getStatusReason())); 135 if (src.hasBusinessStatus()) 136 tgt.setBusinessStatus(CodeableConcept43_50.convertCodeableConcept(src.getBusinessStatus())); 137 if (src.hasIntent()) 138 tgt.setIntentElement(convertTaskIntent(src.getIntentElement())); 139 if (src.hasPriority()) 140 tgt.setPriorityElement(convertTaskPriority(src.getPriorityElement())); 141 if (src.hasCode()) 142 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 143 if (src.hasDescription()) 144 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 145 if (src.hasFocus()) 146 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 147 if (src.hasFor()) 148 tgt.setFor(Reference43_50.convertReference(src.getFor())); 149 if (src.hasEncounter()) 150 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 151 if (src.hasExecutionPeriod()) 152 tgt.setExecutionPeriod(Period43_50.convertPeriod(src.getExecutionPeriod())); 153 if (src.hasAuthoredOn()) 154 tgt.setAuthoredOnElement(DateTime43_50.convertDateTime(src.getAuthoredOnElement())); 155 if (src.hasLastModified()) 156 tgt.setLastModifiedElement(DateTime43_50.convertDateTime(src.getLastModifiedElement())); 157 if (src.hasRequester()) 158 tgt.setRequester(Reference43_50.convertReference(src.getRequester())); 159 for (org.hl7.fhir.r5.model.CodeableReference t : src.getRequestedPerformer()) 160 tgt.addPerformerType(CodeableConcept43_50.convertCodeableReferenceToCodeableConcept(t)); 161 if (src.hasOwner()) 162 tgt.setOwner(Reference43_50.convertReference(src.getOwner())); 163 if (src.hasLocation()) 164 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 165 for (CodeableReference t : src.getReason()) { 166 if (t.hasConcept()) 167 tgt.setReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 168 else if (t.hasReference()) 169 tgt.setReasonReference(Reference43_50.convertReference(t.getReference())); 170 } 171 for (org.hl7.fhir.r5.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference43_50.convertReference(t)); 172 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 173 for (org.hl7.fhir.r5.model.Reference t : src.getRelevantHistory()) 174 tgt.addRelevantHistory(Reference43_50.convertReference(t)); 175 if (src.hasRestriction()) 176 tgt.setRestriction(convertTaskRestrictionComponent(src.getRestriction())); 177 for (org.hl7.fhir.r5.model.Task.TaskInputComponent t : src.getInput()) tgt.addInput(convertParameterComponent(t)); 178 for (org.hl7.fhir.r5.model.Task.TaskOutputComponent t : src.getOutput()) 179 tgt.addOutput(convertTaskOutputComponent(t)); 180 return tgt; 181 } 182 183 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Task.TaskStatus> convertTaskStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskStatus> src) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 Enumeration<Task.TaskStatus> tgt = new Enumeration<>(new Task.TaskStatusEnumFactory()); 187 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 188 if (src.getValue() == null) { 189 tgt.setValue(null); 190 } else { 191 switch (src.getValue()) { 192 case DRAFT: 193 tgt.setValue(Task.TaskStatus.DRAFT); 194 break; 195 case REQUESTED: 196 tgt.setValue(Task.TaskStatus.REQUESTED); 197 break; 198 case RECEIVED: 199 tgt.setValue(Task.TaskStatus.RECEIVED); 200 break; 201 case ACCEPTED: 202 tgt.setValue(Task.TaskStatus.ACCEPTED); 203 break; 204 case REJECTED: 205 tgt.setValue(Task.TaskStatus.REJECTED); 206 break; 207 case READY: 208 tgt.setValue(Task.TaskStatus.READY); 209 break; 210 case CANCELLED: 211 tgt.setValue(Task.TaskStatus.CANCELLED); 212 break; 213 case INPROGRESS: 214 tgt.setValue(Task.TaskStatus.INPROGRESS); 215 break; 216 case ONHOLD: 217 tgt.setValue(Task.TaskStatus.ONHOLD); 218 break; 219 case FAILED: 220 tgt.setValue(Task.TaskStatus.FAILED); 221 break; 222 case COMPLETED: 223 tgt.setValue(Task.TaskStatus.COMPLETED); 224 break; 225 case ENTEREDINERROR: 226 tgt.setValue(Task.TaskStatus.ENTEREDINERROR); 227 break; 228 default: 229 tgt.setValue(Task.TaskStatus.NULL); 230 break; 231 } 232 } 233 return tgt; 234 } 235 236 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskStatus> convertTaskStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Task.TaskStatus> src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Task.TaskStatusEnumFactory()); 240 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 241 if (src.getValue() == null) { 242 tgt.setValue(null); 243 } else { 244 switch (src.getValue()) { 245 case DRAFT: 246 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.DRAFT); 247 break; 248 case REQUESTED: 249 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.REQUESTED); 250 break; 251 case RECEIVED: 252 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.RECEIVED); 253 break; 254 case ACCEPTED: 255 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.ACCEPTED); 256 break; 257 case REJECTED: 258 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.REJECTED); 259 break; 260 case READY: 261 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.READY); 262 break; 263 case CANCELLED: 264 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.CANCELLED); 265 break; 266 case INPROGRESS: 267 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.INPROGRESS); 268 break; 269 case ONHOLD: 270 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.ONHOLD); 271 break; 272 case FAILED: 273 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.FAILED); 274 break; 275 case COMPLETED: 276 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.COMPLETED); 277 break; 278 case ENTEREDINERROR: 279 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.ENTEREDINERROR); 280 break; 281 default: 282 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskStatus.NULL); 283 break; 284 } 285 } 286 return tgt; 287 } 288 289 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Task.TaskIntent> convertTaskIntent(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskIntent> src) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 Enumeration<Task.TaskIntent> tgt = new Enumeration<>(new Task.TaskIntentEnumFactory()); 293 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 294 if (src.getValue() == null) { 295 tgt.setValue(null); 296 } else { 297 switch (src.getValue()) { 298 case UNKNOWN: 299 tgt.setValue(Task.TaskIntent.UNKNOWN); 300 break; 301 case PROPOSAL: 302 tgt.setValue(Task.TaskIntent.PROPOSAL); 303 break; 304 case PLAN: 305 tgt.setValue(Task.TaskIntent.PLAN); 306 break; 307 case ORDER: 308 tgt.setValue(Task.TaskIntent.ORDER); 309 break; 310 case ORIGINALORDER: 311 tgt.setValue(Task.TaskIntent.ORIGINALORDER); 312 break; 313 case REFLEXORDER: 314 tgt.setValue(Task.TaskIntent.REFLEXORDER); 315 break; 316 case FILLERORDER: 317 tgt.setValue(Task.TaskIntent.FILLERORDER); 318 break; 319 case INSTANCEORDER: 320 tgt.setValue(Task.TaskIntent.INSTANCEORDER); 321 break; 322 case OPTION: 323 tgt.setValue(Task.TaskIntent.OPTION); 324 break; 325 default: 326 tgt.setValue(Task.TaskIntent.NULL); 327 break; 328 } 329 } 330 return tgt; 331 } 332 333 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskIntent> convertTaskIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Task.TaskIntent> src) throws FHIRException { 334 if (src == null || src.isEmpty()) 335 return null; 336 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Task.TaskIntent> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Task.TaskIntentEnumFactory()); 337 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 338 if (src.getValue() == null) { 339 tgt.setValue(null); 340 } else { 341 switch (src.getValue()) { 342 case UNKNOWN: 343 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.UNKNOWN); 344 break; 345 case PROPOSAL: 346 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.PROPOSAL); 347 break; 348 case PLAN: 349 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.PLAN); 350 break; 351 case ORDER: 352 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.ORDER); 353 break; 354 case ORIGINALORDER: 355 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.ORIGINALORDER); 356 break; 357 case REFLEXORDER: 358 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.REFLEXORDER); 359 break; 360 case FILLERORDER: 361 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.FILLERORDER); 362 break; 363 case INSTANCEORDER: 364 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.INSTANCEORDER); 365 break; 366 case OPTION: 367 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.OPTION); 368 break; 369 default: 370 tgt.setValue(org.hl7.fhir.r4b.model.Task.TaskIntent.NULL); 371 break; 372 } 373 } 374 return tgt; 375 } 376 377 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertTaskPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 378 if (src == null || src.isEmpty()) 379 return null; 380 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 381 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 382 if (src.getValue() == null) { 383 tgt.setValue(null); 384 } else { 385 switch (src.getValue()) { 386 case ROUTINE: 387 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 388 break; 389 case URGENT: 390 tgt.setValue(Enumerations.RequestPriority.URGENT); 391 break; 392 case ASAP: 393 tgt.setValue(Enumerations.RequestPriority.ASAP); 394 break; 395 case STAT: 396 tgt.setValue(Enumerations.RequestPriority.STAT); 397 break; 398 default: 399 tgt.setValue(Enumerations.RequestPriority.NULL); 400 break; 401 } 402 } 403 return tgt; 404 } 405 406 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertTaskPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 407 if (src == null || src.isEmpty()) 408 return null; 409 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 410 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 411 if (src.getValue() == null) { 412 tgt.setValue(null); 413 } else { 414 switch (src.getValue()) { 415 case ROUTINE: 416 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 417 break; 418 case URGENT: 419 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 420 break; 421 case ASAP: 422 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 423 break; 424 case STAT: 425 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 426 break; 427 default: 428 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 429 break; 430 } 431 } 432 return tgt; 433 } 434 435 public static org.hl7.fhir.r5.model.Task.TaskRestrictionComponent convertTaskRestrictionComponent(org.hl7.fhir.r4b.model.Task.TaskRestrictionComponent src) throws FHIRException { 436 if (src == null) 437 return null; 438 org.hl7.fhir.r5.model.Task.TaskRestrictionComponent tgt = new org.hl7.fhir.r5.model.Task.TaskRestrictionComponent(); 439 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 440 if (src.hasRepetitions()) 441 tgt.setRepetitionsElement(PositiveInt43_50.convertPositiveInt(src.getRepetitionsElement())); 442 if (src.hasPeriod()) 443 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 444 for (org.hl7.fhir.r4b.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference43_50.convertReference(t)); 445 return tgt; 446 } 447 448 public static org.hl7.fhir.r4b.model.Task.TaskRestrictionComponent convertTaskRestrictionComponent(org.hl7.fhir.r5.model.Task.TaskRestrictionComponent src) throws FHIRException { 449 if (src == null) 450 return null; 451 org.hl7.fhir.r4b.model.Task.TaskRestrictionComponent tgt = new org.hl7.fhir.r4b.model.Task.TaskRestrictionComponent(); 452 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 453 if (src.hasRepetitions()) 454 tgt.setRepetitionsElement(PositiveInt43_50.convertPositiveInt(src.getRepetitionsElement())); 455 if (src.hasPeriod()) 456 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 457 for (org.hl7.fhir.r5.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference43_50.convertReference(t)); 458 return tgt; 459 } 460 461 public static org.hl7.fhir.r5.model.Task.TaskInputComponent convertParameterComponent(org.hl7.fhir.r4b.model.Task.ParameterComponent src) throws FHIRException { 462 if (src == null) 463 return null; 464 org.hl7.fhir.r5.model.Task.TaskInputComponent tgt = new org.hl7.fhir.r5.model.Task.TaskInputComponent(); 465 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 466 if (src.hasType()) 467 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 468 if (src.hasValue()) 469 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 470 return tgt; 471 } 472 473 public static org.hl7.fhir.r4b.model.Task.ParameterComponent convertParameterComponent(org.hl7.fhir.r5.model.Task.TaskInputComponent src) throws FHIRException { 474 if (src == null) 475 return null; 476 org.hl7.fhir.r4b.model.Task.ParameterComponent tgt = new org.hl7.fhir.r4b.model.Task.ParameterComponent(); 477 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 478 if (src.hasType()) 479 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 480 if (src.hasValue()) 481 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 482 return tgt; 483 } 484 485 public static org.hl7.fhir.r5.model.Task.TaskOutputComponent convertTaskOutputComponent(org.hl7.fhir.r4b.model.Task.TaskOutputComponent src) throws FHIRException { 486 if (src == null) 487 return null; 488 org.hl7.fhir.r5.model.Task.TaskOutputComponent tgt = new org.hl7.fhir.r5.model.Task.TaskOutputComponent(); 489 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 490 if (src.hasType()) 491 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 492 if (src.hasValue()) 493 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 494 return tgt; 495 } 496 497 public static org.hl7.fhir.r4b.model.Task.TaskOutputComponent convertTaskOutputComponent(org.hl7.fhir.r5.model.Task.TaskOutputComponent src) throws FHIRException { 498 if (src == null) 499 return null; 500 org.hl7.fhir.r4b.model.Task.TaskOutputComponent tgt = new org.hl7.fhir.r4b.model.Task.TaskOutputComponent(); 501 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 502 if (src.hasType()) 503 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 504 if (src.hasValue()) 505 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 506 return tgt; 507 } 508}