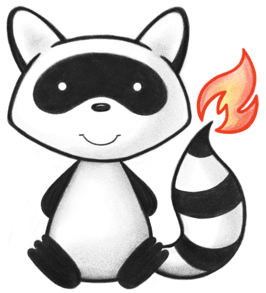
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Decimal43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4b.model.Reference; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.TestReport; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class TestReport43_50 { 045 046 public static org.hl7.fhir.r5.model.TestReport convertTestReport(org.hl7.fhir.r4b.model.TestReport src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.TestReport tgt = new org.hl7.fhir.r5.model.TestReport(); 050 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 051 if (src.hasIdentifier()) 052 tgt.setIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 053 if (src.hasName()) 054 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertTestReportStatus(src.getStatusElement())); 057 if (src.hasTestScript()) 058 tgt.setTestScript(src.getTestScript().getReference()); 059 if (src.hasResult()) 060 tgt.setResultElement(convertTestReportResult(src.getResultElement())); 061 if (src.hasScore()) 062 tgt.setScoreElement(Decimal43_50.convertDecimal(src.getScoreElement())); 063 if (src.hasTester()) 064 tgt.setTesterElement(String43_50.convertString(src.getTesterElement())); 065 if (src.hasIssued()) 066 tgt.setIssuedElement(DateTime43_50.convertDateTime(src.getIssuedElement())); 067 for (org.hl7.fhir.r4b.model.TestReport.TestReportParticipantComponent t : src.getParticipant()) 068 tgt.addParticipant(convertTestReportParticipantComponent(t)); 069 if (src.hasSetup()) 070 tgt.setSetup(convertTestReportSetupComponent(src.getSetup())); 071 for (org.hl7.fhir.r4b.model.TestReport.TestReportTestComponent t : src.getTest()) 072 tgt.addTest(convertTestReportTestComponent(t)); 073 if (src.hasTeardown()) 074 tgt.setTeardown(convertTestReportTeardownComponent(src.getTeardown())); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4b.model.TestReport convertTestReport(org.hl7.fhir.r5.model.TestReport src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4b.model.TestReport tgt = new org.hl7.fhir.r4b.model.TestReport(); 082 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 083 if (src.hasIdentifier()) 084 tgt.setIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 085 if (src.hasName()) 086 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 087 if (src.hasStatus()) 088 tgt.setStatusElement(convertTestReportStatus(src.getStatusElement())); 089 if (src.hasTestScript()) 090 tgt.setTestScript(new Reference().setReference(src.getTestScript())); 091 if (src.hasResult()) 092 tgt.setResultElement(convertTestReportResult(src.getResultElement())); 093 if (src.hasScore()) 094 tgt.setScoreElement(Decimal43_50.convertDecimal(src.getScoreElement())); 095 if (src.hasTester()) 096 tgt.setTesterElement(String43_50.convertString(src.getTesterElement())); 097 if (src.hasIssued()) 098 tgt.setIssuedElement(DateTime43_50.convertDateTime(src.getIssuedElement())); 099 for (org.hl7.fhir.r5.model.TestReport.TestReportParticipantComponent t : src.getParticipant()) 100 tgt.addParticipant(convertTestReportParticipantComponent(t)); 101 if (src.hasSetup()) 102 tgt.setSetup(convertTestReportSetupComponent(src.getSetup())); 103 for (org.hl7.fhir.r5.model.TestReport.TestReportTestComponent t : src.getTest()) 104 tgt.addTest(convertTestReportTestComponent(t)); 105 if (src.hasTeardown()) 106 tgt.setTeardown(convertTestReportTeardownComponent(src.getTeardown())); 107 return tgt; 108 } 109 110 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportStatus> convertTestReportStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportStatus> src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 Enumeration<TestReport.TestReportStatus> tgt = new Enumeration<>(new TestReport.TestReportStatusEnumFactory()); 114 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 115 if (src.getValue() == null) { 116 tgt.setValue(null); 117 } else { 118 switch (src.getValue()) { 119 case COMPLETED: 120 tgt.setValue(TestReport.TestReportStatus.COMPLETED); 121 break; 122 case INPROGRESS: 123 tgt.setValue(TestReport.TestReportStatus.INPROGRESS); 124 break; 125 case WAITING: 126 tgt.setValue(TestReport.TestReportStatus.WAITING); 127 break; 128 case STOPPED: 129 tgt.setValue(TestReport.TestReportStatus.STOPPED); 130 break; 131 case ENTEREDINERROR: 132 tgt.setValue(TestReport.TestReportStatus.ENTEREDINERROR); 133 break; 134 default: 135 tgt.setValue(TestReport.TestReportStatus.NULL); 136 break; 137 } 138 } 139 return tgt; 140 } 141 142 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportStatus> convertTestReportStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportStatus> src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestReport.TestReportStatusEnumFactory()); 146 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 147 if (src.getValue() == null) { 148 tgt.setValue(null); 149 } else { 150 switch (src.getValue()) { 151 case COMPLETED: 152 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.COMPLETED); 153 break; 154 case INPROGRESS: 155 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.INPROGRESS); 156 break; 157 case WAITING: 158 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.WAITING); 159 break; 160 case STOPPED: 161 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.STOPPED); 162 break; 163 case ENTEREDINERROR: 164 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.ENTEREDINERROR); 165 break; 166 default: 167 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportStatus.NULL); 168 break; 169 } 170 } 171 return tgt; 172 } 173 174 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportResult> convertTestReportResult(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportResult> src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 Enumeration<TestReport.TestReportResult> tgt = new Enumeration<>(new TestReport.TestReportResultEnumFactory()); 178 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 179 if (src.getValue() == null) { 180 tgt.setValue(null); 181 } else { 182 switch (src.getValue()) { 183 case PASS: 184 tgt.setValue(TestReport.TestReportResult.PASS); 185 break; 186 case FAIL: 187 tgt.setValue(TestReport.TestReportResult.FAIL); 188 break; 189 case PENDING: 190 tgt.setValue(TestReport.TestReportResult.PENDING); 191 break; 192 default: 193 tgt.setValue(TestReport.TestReportResult.NULL); 194 break; 195 } 196 } 197 return tgt; 198 } 199 200 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportResult> convertTestReportResult(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportResult> src) throws FHIRException { 201 if (src == null || src.isEmpty()) 202 return null; 203 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportResult> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestReport.TestReportResultEnumFactory()); 204 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 205 if (src.getValue() == null) { 206 tgt.setValue(null); 207 } else { 208 switch (src.getValue()) { 209 case PASS: 210 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportResult.PASS); 211 break; 212 case FAIL: 213 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportResult.FAIL); 214 break; 215 case PENDING: 216 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportResult.PENDING); 217 break; 218 default: 219 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportResult.NULL); 220 break; 221 } 222 } 223 return tgt; 224 } 225 226 public static org.hl7.fhir.r5.model.TestReport.TestReportParticipantComponent convertTestReportParticipantComponent(org.hl7.fhir.r4b.model.TestReport.TestReportParticipantComponent src) throws FHIRException { 227 if (src == null) 228 return null; 229 org.hl7.fhir.r5.model.TestReport.TestReportParticipantComponent tgt = new org.hl7.fhir.r5.model.TestReport.TestReportParticipantComponent(); 230 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 231 if (src.hasType()) 232 tgt.setTypeElement(convertTestReportParticipantType(src.getTypeElement())); 233 if (src.hasUri()) 234 tgt.setUriElement(Uri43_50.convertUri(src.getUriElement())); 235 if (src.hasDisplay()) 236 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 237 return tgt; 238 } 239 240 public static org.hl7.fhir.r4b.model.TestReport.TestReportParticipantComponent convertTestReportParticipantComponent(org.hl7.fhir.r5.model.TestReport.TestReportParticipantComponent src) throws FHIRException { 241 if (src == null) 242 return null; 243 org.hl7.fhir.r4b.model.TestReport.TestReportParticipantComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TestReportParticipantComponent(); 244 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 245 if (src.hasType()) 246 tgt.setTypeElement(convertTestReportParticipantType(src.getTypeElement())); 247 if (src.hasUri()) 248 tgt.setUriElement(Uri43_50.convertUri(src.getUriElement())); 249 if (src.hasDisplay()) 250 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 251 return tgt; 252 } 253 254 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportParticipantType> convertTestReportParticipantType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType> src) throws FHIRException { 255 if (src == null || src.isEmpty()) 256 return null; 257 Enumeration<TestReport.TestReportParticipantType> tgt = new Enumeration<>(new TestReport.TestReportParticipantTypeEnumFactory()); 258 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 259 if (src.getValue() == null) { 260 tgt.setValue(null); 261 } else { 262 switch (src.getValue()) { 263 case TESTENGINE: 264 tgt.setValue(TestReport.TestReportParticipantType.TESTENGINE); 265 break; 266 case CLIENT: 267 tgt.setValue(TestReport.TestReportParticipantType.CLIENT); 268 break; 269 case SERVER: 270 tgt.setValue(TestReport.TestReportParticipantType.SERVER); 271 break; 272 default: 273 tgt.setValue(TestReport.TestReportParticipantType.NULL); 274 break; 275 } 276 } 277 return tgt; 278 } 279 280 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType> convertTestReportParticipantType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportParticipantType> src) throws FHIRException { 281 if (src == null || src.isEmpty()) 282 return null; 283 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestReport.TestReportParticipantTypeEnumFactory()); 284 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 285 if (src.getValue() == null) { 286 tgt.setValue(null); 287 } else { 288 switch (src.getValue()) { 289 case TESTENGINE: 290 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType.TESTENGINE); 291 break; 292 case CLIENT: 293 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType.CLIENT); 294 break; 295 case SERVER: 296 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType.SERVER); 297 break; 298 default: 299 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportParticipantType.NULL); 300 break; 301 } 302 } 303 return tgt; 304 } 305 306 public static org.hl7.fhir.r5.model.TestReport.TestReportSetupComponent convertTestReportSetupComponent(org.hl7.fhir.r4b.model.TestReport.TestReportSetupComponent src) throws FHIRException { 307 if (src == null) 308 return null; 309 org.hl7.fhir.r5.model.TestReport.TestReportSetupComponent tgt = new org.hl7.fhir.r5.model.TestReport.TestReportSetupComponent(); 310 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 311 for (org.hl7.fhir.r4b.model.TestReport.SetupActionComponent t : src.getAction()) 312 tgt.addAction(convertSetupActionComponent(t)); 313 return tgt; 314 } 315 316 public static org.hl7.fhir.r4b.model.TestReport.TestReportSetupComponent convertTestReportSetupComponent(org.hl7.fhir.r5.model.TestReport.TestReportSetupComponent src) throws FHIRException { 317 if (src == null) 318 return null; 319 org.hl7.fhir.r4b.model.TestReport.TestReportSetupComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TestReportSetupComponent(); 320 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 321 for (org.hl7.fhir.r5.model.TestReport.SetupActionComponent t : src.getAction()) 322 tgt.addAction(convertSetupActionComponent(t)); 323 return tgt; 324 } 325 326 public static org.hl7.fhir.r5.model.TestReport.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r4b.model.TestReport.SetupActionComponent src) throws FHIRException { 327 if (src == null) 328 return null; 329 org.hl7.fhir.r5.model.TestReport.SetupActionComponent tgt = new org.hl7.fhir.r5.model.TestReport.SetupActionComponent(); 330 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 331 if (src.hasOperation()) 332 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 333 if (src.hasAssert()) 334 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 335 return tgt; 336 } 337 338 public static org.hl7.fhir.r4b.model.TestReport.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r5.model.TestReport.SetupActionComponent src) throws FHIRException { 339 if (src == null) 340 return null; 341 org.hl7.fhir.r4b.model.TestReport.SetupActionComponent tgt = new org.hl7.fhir.r4b.model.TestReport.SetupActionComponent(); 342 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 343 if (src.hasOperation()) 344 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 345 if (src.hasAssert()) 346 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 347 return tgt; 348 } 349 350 public static org.hl7.fhir.r5.model.TestReport.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r4b.model.TestReport.SetupActionOperationComponent src) throws FHIRException { 351 if (src == null) 352 return null; 353 org.hl7.fhir.r5.model.TestReport.SetupActionOperationComponent tgt = new org.hl7.fhir.r5.model.TestReport.SetupActionOperationComponent(); 354 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 355 if (src.hasResult()) 356 tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 357 if (src.hasMessage()) 358 tgt.setMessageElement(MarkDown43_50.convertMarkdown(src.getMessageElement())); 359 if (src.hasDetail()) 360 tgt.setDetailElement(Uri43_50.convertUri(src.getDetailElement())); 361 return tgt; 362 } 363 364 public static org.hl7.fhir.r4b.model.TestReport.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r5.model.TestReport.SetupActionOperationComponent src) throws FHIRException { 365 if (src == null) 366 return null; 367 org.hl7.fhir.r4b.model.TestReport.SetupActionOperationComponent tgt = new org.hl7.fhir.r4b.model.TestReport.SetupActionOperationComponent(); 368 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 369 if (src.hasResult()) 370 tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 371 if (src.hasMessage()) 372 tgt.setMessageElement(MarkDown43_50.convertMarkdown(src.getMessageElement())); 373 if (src.hasDetail()) 374 tgt.setDetailElement(Uri43_50.convertUri(src.getDetailElement())); 375 return tgt; 376 } 377 378 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportActionResult> convertTestReportActionResult(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportActionResult> src) throws FHIRException { 379 if (src == null || src.isEmpty()) 380 return null; 381 Enumeration<TestReport.TestReportActionResult> tgt = new Enumeration<>(new TestReport.TestReportActionResultEnumFactory()); 382 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 383 if (src.getValue() == null) { 384 tgt.setValue(null); 385 } else { 386 switch (src.getValue()) { 387 case PASS: 388 tgt.setValue(TestReport.TestReportActionResult.PASS); 389 break; 390 case SKIP: 391 tgt.setValue(TestReport.TestReportActionResult.SKIP); 392 break; 393 case FAIL: 394 tgt.setValue(TestReport.TestReportActionResult.FAIL); 395 break; 396 case WARNING: 397 tgt.setValue(TestReport.TestReportActionResult.WARNING); 398 break; 399 case ERROR: 400 tgt.setValue(TestReport.TestReportActionResult.ERROR); 401 break; 402 default: 403 tgt.setValue(TestReport.TestReportActionResult.NULL); 404 break; 405 } 406 } 407 return tgt; 408 } 409 410 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportActionResult> convertTestReportActionResult(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestReport.TestReportActionResult> src) throws FHIRException { 411 if (src == null || src.isEmpty()) 412 return null; 413 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestReport.TestReportActionResult> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestReport.TestReportActionResultEnumFactory()); 414 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 415 if (src.getValue() == null) { 416 tgt.setValue(null); 417 } else { 418 switch (src.getValue()) { 419 case PASS: 420 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.PASS); 421 break; 422 case SKIP: 423 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.SKIP); 424 break; 425 case FAIL: 426 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.FAIL); 427 break; 428 case WARNING: 429 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.WARNING); 430 break; 431 case ERROR: 432 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.ERROR); 433 break; 434 default: 435 tgt.setValue(org.hl7.fhir.r4b.model.TestReport.TestReportActionResult.NULL); 436 break; 437 } 438 } 439 return tgt; 440 } 441 442 public static org.hl7.fhir.r5.model.TestReport.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r4b.model.TestReport.SetupActionAssertComponent src) throws FHIRException { 443 if (src == null) 444 return null; 445 org.hl7.fhir.r5.model.TestReport.SetupActionAssertComponent tgt = new org.hl7.fhir.r5.model.TestReport.SetupActionAssertComponent(); 446 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 447 if (src.hasResult()) 448 tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 449 if (src.hasMessage()) 450 tgt.setMessageElement(MarkDown43_50.convertMarkdown(src.getMessageElement())); 451 if (src.hasDetail()) 452 tgt.setDetailElement(String43_50.convertString(src.getDetailElement())); 453 return tgt; 454 } 455 456 public static org.hl7.fhir.r4b.model.TestReport.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r5.model.TestReport.SetupActionAssertComponent src) throws FHIRException { 457 if (src == null) 458 return null; 459 org.hl7.fhir.r4b.model.TestReport.SetupActionAssertComponent tgt = new org.hl7.fhir.r4b.model.TestReport.SetupActionAssertComponent(); 460 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 461 if (src.hasResult()) 462 tgt.setResultElement(convertTestReportActionResult(src.getResultElement())); 463 if (src.hasMessage()) 464 tgt.setMessageElement(MarkDown43_50.convertMarkdown(src.getMessageElement())); 465 if (src.hasDetail()) 466 tgt.setDetailElement(String43_50.convertString(src.getDetailElement())); 467 return tgt; 468 } 469 470 public static org.hl7.fhir.r5.model.TestReport.TestReportTestComponent convertTestReportTestComponent(org.hl7.fhir.r4b.model.TestReport.TestReportTestComponent src) throws FHIRException { 471 if (src == null) 472 return null; 473 org.hl7.fhir.r5.model.TestReport.TestReportTestComponent tgt = new org.hl7.fhir.r5.model.TestReport.TestReportTestComponent(); 474 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 475 if (src.hasName()) 476 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 477 if (src.hasDescription()) 478 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 479 for (org.hl7.fhir.r4b.model.TestReport.TestActionComponent t : src.getAction()) 480 tgt.addAction(convertTestActionComponent(t)); 481 return tgt; 482 } 483 484 public static org.hl7.fhir.r4b.model.TestReport.TestReportTestComponent convertTestReportTestComponent(org.hl7.fhir.r5.model.TestReport.TestReportTestComponent src) throws FHIRException { 485 if (src == null) 486 return null; 487 org.hl7.fhir.r4b.model.TestReport.TestReportTestComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TestReportTestComponent(); 488 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 489 if (src.hasName()) 490 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 491 if (src.hasDescription()) 492 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 493 for (org.hl7.fhir.r5.model.TestReport.TestActionComponent t : src.getAction()) 494 tgt.addAction(convertTestActionComponent(t)); 495 return tgt; 496 } 497 498 public static org.hl7.fhir.r5.model.TestReport.TestActionComponent convertTestActionComponent(org.hl7.fhir.r4b.model.TestReport.TestActionComponent src) throws FHIRException { 499 if (src == null) 500 return null; 501 org.hl7.fhir.r5.model.TestReport.TestActionComponent tgt = new org.hl7.fhir.r5.model.TestReport.TestActionComponent(); 502 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 503 if (src.hasOperation()) 504 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 505 if (src.hasAssert()) 506 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 507 return tgt; 508 } 509 510 public static org.hl7.fhir.r4b.model.TestReport.TestActionComponent convertTestActionComponent(org.hl7.fhir.r5.model.TestReport.TestActionComponent src) throws FHIRException { 511 if (src == null) 512 return null; 513 org.hl7.fhir.r4b.model.TestReport.TestActionComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TestActionComponent(); 514 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 515 if (src.hasOperation()) 516 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 517 if (src.hasAssert()) 518 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 519 return tgt; 520 } 521 522 public static org.hl7.fhir.r5.model.TestReport.TestReportTeardownComponent convertTestReportTeardownComponent(org.hl7.fhir.r4b.model.TestReport.TestReportTeardownComponent src) throws FHIRException { 523 if (src == null) 524 return null; 525 org.hl7.fhir.r5.model.TestReport.TestReportTeardownComponent tgt = new org.hl7.fhir.r5.model.TestReport.TestReportTeardownComponent(); 526 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 527 for (org.hl7.fhir.r4b.model.TestReport.TeardownActionComponent t : src.getAction()) 528 tgt.addAction(convertTeardownActionComponent(t)); 529 return tgt; 530 } 531 532 public static org.hl7.fhir.r4b.model.TestReport.TestReportTeardownComponent convertTestReportTeardownComponent(org.hl7.fhir.r5.model.TestReport.TestReportTeardownComponent src) throws FHIRException { 533 if (src == null) 534 return null; 535 org.hl7.fhir.r4b.model.TestReport.TestReportTeardownComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TestReportTeardownComponent(); 536 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 537 for (org.hl7.fhir.r5.model.TestReport.TeardownActionComponent t : src.getAction()) 538 tgt.addAction(convertTeardownActionComponent(t)); 539 return tgt; 540 } 541 542 public static org.hl7.fhir.r5.model.TestReport.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r4b.model.TestReport.TeardownActionComponent src) throws FHIRException { 543 if (src == null) 544 return null; 545 org.hl7.fhir.r5.model.TestReport.TeardownActionComponent tgt = new org.hl7.fhir.r5.model.TestReport.TeardownActionComponent(); 546 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 547 if (src.hasOperation()) 548 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 549 return tgt; 550 } 551 552 public static org.hl7.fhir.r4b.model.TestReport.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r5.model.TestReport.TeardownActionComponent src) throws FHIRException { 553 if (src == null) 554 return null; 555 org.hl7.fhir.r4b.model.TestReport.TeardownActionComponent tgt = new org.hl7.fhir.r4b.model.TestReport.TeardownActionComponent(); 556 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 557 if (src.hasOperation()) 558 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 559 return tgt; 560 } 561}