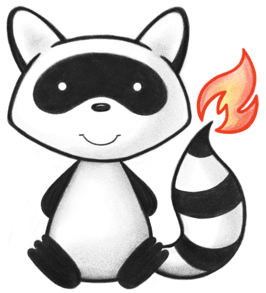
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Id43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Integer43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r5.model.CanonicalType; 021import org.hl7.fhir.r5.model.Enumeration; 022import org.hl7.fhir.r5.model.TestScript; 023import org.hl7.fhir.r5.model.TestScript.TestScriptScopeComponent; 024 025/* 026 Copyright (c) 2011+, HL7, Inc. 027 All rights reserved. 028 029 Redistribution and use in source and binary forms, with or without modification, 030 are permitted provided that the following conditions are met: 031 032 * Redistributions of source code must retain the above copyright notice, this 033 list of conditions and the following disclaimer. 034 * Redistributions in binary form must reproduce the above copyright notice, 035 this list of conditions and the following disclaimer in the documentation 036 and/or other materials provided with the distribution. 037 * Neither the name of HL7 nor the names of its contributors may be used to 038 endorse or promote products derived from this software without specific 039 prior written permission. 040 041 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 042 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 043 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 044 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 045 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 046 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 047 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 048 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 049 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 050 POSSIBILITY OF SUCH DAMAGE. 051 052*/ 053// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 054public class TestScript43_50 { 055 056 public static org.hl7.fhir.r5.model.TestScript convertTestScript(org.hl7.fhir.r4b.model.TestScript src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.r5.model.TestScript tgt = new org.hl7.fhir.r5.model.TestScript(); 060 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 061 for (org.hl7.fhir.r4b.model.Extension ext : src.getExtensionsByUrl("http://hl7.org/fhir/5.0/StructureDefinition/extension-TestScript.scope")) { 062 // the advisor will get this ignored. 063 TestScriptScopeComponent scope = tgt.addScope(); 064 scope.setArtifact(ext.getExtensionString("artifact")); 065 org.hl7.fhir.r4b.model.Extension se = ext.getExtensionByUrl("conformance"); 066 if (se != null) { 067 scope.setConformance(CodeableConcept43_50.convertCodeableConcept((org.hl7.fhir.r4b.model.CodeableConcept) se.getValue())); 068 } 069 se = ext.getExtensionByUrl("phase"); 070 if (se != null) { 071 scope.setPhase(CodeableConcept43_50.convertCodeableConcept((org.hl7.fhir.r4b.model.CodeableConcept) se.getValue())); 072 } 073 } 074 if (src.hasUrl()) 075 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 076 if (src.hasIdentifier()) 077 tgt.addIdentifier(Identifier43_50.convertIdentifier(src.getIdentifierFirstRep())); 078 if (src.hasVersion()) 079 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 080 if (src.hasName()) 081 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 082 if (src.hasTitle()) 083 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 084 if (src.hasStatus()) 085 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 086 if (src.hasExperimental()) 087 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 088 if (src.hasDate()) 089 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 090 if (src.hasPublisher()) 091 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 092 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 093 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 094 if (src.hasDescription()) 095 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 096 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 097 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 098 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 099 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 100 if (src.hasPurpose()) 101 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 102 if (src.hasCopyright()) 103 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 104 for (org.hl7.fhir.r4b.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 105 tgt.addOrigin(convertTestScriptOriginComponent(t)); 106 for (org.hl7.fhir.r4b.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 107 tgt.addDestination(convertTestScriptDestinationComponent(t)); 108 if (src.hasMetadata()) 109 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 110 for (org.hl7.fhir.r4b.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 111 tgt.addFixture(convertTestScriptFixtureComponent(t)); 112 for (org.hl7.fhir.r4b.model.Reference t : src.getProfile()) tgt.getProfile().add(Reference43_50.convertReferenceToCanonical(t)); 113 for (org.hl7.fhir.r4b.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 114 tgt.addVariable(convertTestScriptVariableComponent(t)); 115 if (src.hasSetup()) 116 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 117 for (org.hl7.fhir.r4b.model.TestScript.TestScriptTestComponent t : src.getTest()) 118 tgt.addTest(convertTestScriptTestComponent(t)); 119 if (src.hasTeardown()) 120 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 121 return tgt; 122 } 123 124 public static org.hl7.fhir.r4b.model.TestScript convertTestScript(org.hl7.fhir.r5.model.TestScript src) throws FHIRException { 125 if (src == null) 126 return null; 127 org.hl7.fhir.r4b.model.TestScript tgt = new org.hl7.fhir.r4b.model.TestScript(); 128 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 129 if (src.hasUrl()) 130 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 131 if (src.hasIdentifier()) 132 tgt.addIdentifier(Identifier43_50.convertIdentifier(src.getIdentifierFirstRep())); 133 if (src.hasVersion()) 134 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 135 if (src.hasName()) 136 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 137 if (src.hasTitle()) 138 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 139 if (src.hasStatus()) 140 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 141 if (src.hasExperimental()) 142 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 143 if (src.hasDate()) 144 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 145 if (src.hasPublisher()) 146 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 147 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 148 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 149 if (src.hasDescription()) 150 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 151 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 152 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 153 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 154 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 155 if (src.hasPurpose()) 156 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 157 if (src.hasCopyright()) 158 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 159 for (org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 160 tgt.addOrigin(convertTestScriptOriginComponent(t)); 161 for (org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 162 tgt.addDestination(convertTestScriptDestinationComponent(t)); 163 if (src.hasMetadata()) 164 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 165 for (org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 166 tgt.addFixture(convertTestScriptFixtureComponent(t)); 167 for (CanonicalType t : src.getProfile()) tgt.addProfile(Reference43_50.convertCanonicalToReference(t)); 168 for (org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 169 tgt.addVariable(convertTestScriptVariableComponent(t)); 170 if (src.hasSetup()) 171 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 172 for (org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent t : src.getTest()) 173 tgt.addTest(convertTestScriptTestComponent(t)); 174 if (src.hasTeardown()) 175 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 176 for (TestScriptScopeComponent scope : src.getScope()) { 177 org.hl7.fhir.r4b.model.Extension ext = tgt.addExtension(); 178 ext.setUrl("http://hl7.org/fhir/5.0/StructureDefinition/extension-TestScript.scope"); 179 if (scope.hasArtifact()) { 180 org.hl7.fhir.r4b.model.Extension se = ext.addExtension(); 181 se.setUrl("artifact"); 182 se.setValue(Canonical43_50.convertCanonical(scope.getArtifactElement())); 183 } 184 if (scope.hasConformance()) { 185 org.hl7.fhir.r4b.model.Extension se = ext.addExtension(); 186 se.setUrl("conformance"); 187 se.setValue(CodeableConcept43_50.convertCodeableConcept(scope.getConformance())); 188 } 189 if (scope.hasPhase()) { 190 org.hl7.fhir.r4b.model.Extension se = ext.addExtension(); 191 se.setUrl("phase"); 192 se.setValue(CodeableConcept43_50.convertCodeableConcept(scope.getPhase())); 193 } 194 } 195 return tgt; 196 } 197 198 public static org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 199 if (src == null) 200 return null; 201 org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent(); 202 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 203 if (src.hasIndex()) 204 tgt.setIndexElement(Integer43_50.convertInteger(src.getIndexElement())); 205 if (src.hasProfile()) 206 tgt.setProfile(Coding43_50.convertCoding(src.getProfile())); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r4b.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 211 if (src == null) 212 return null; 213 org.hl7.fhir.r4b.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptOriginComponent(); 214 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 215 if (src.hasIndex()) 216 tgt.setIndexElement(Integer43_50.convertInteger(src.getIndexElement())); 217 if (src.hasProfile()) 218 tgt.setProfile(Coding43_50.convertCoding(src.getProfile())); 219 return tgt; 220 } 221 222 public static org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 223 if (src == null) 224 return null; 225 org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent(); 226 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 227 if (src.hasIndex()) 228 tgt.setIndexElement(Integer43_50.convertInteger(src.getIndexElement())); 229 if (src.hasProfile()) 230 tgt.setProfile(Coding43_50.convertCoding(src.getProfile())); 231 return tgt; 232 } 233 234 public static org.hl7.fhir.r4b.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 235 if (src == null) 236 return null; 237 org.hl7.fhir.r4b.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptDestinationComponent(); 238 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 239 if (src.hasIndex()) 240 tgt.setIndexElement(Integer43_50.convertInteger(src.getIndexElement())); 241 if (src.hasProfile()) 242 tgt.setProfile(Coding43_50.convertCoding(src.getProfile())); 243 return tgt; 244 } 245 246 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 247 if (src == null) 248 return null; 249 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent(); 250 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 251 for (org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 252 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 253 for (org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 254 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 255 return tgt; 256 } 257 258 public static org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 259 if (src == null) 260 return null; 261 org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataComponent(); 262 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 263 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 264 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 265 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 266 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 267 return tgt; 268 } 269 270 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 271 if (src == null) 272 return null; 273 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent(); 274 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 275 if (src.hasUrl()) 276 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 277 if (src.hasDescription()) 278 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 279 return tgt; 280 } 281 282 public static org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 283 if (src == null) 284 return null; 285 org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataLinkComponent(); 286 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 287 if (src.hasUrl()) 288 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 289 if (src.hasDescription()) 290 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 291 return tgt; 292 } 293 294 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 295 if (src == null) 296 return null; 297 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent(); 298 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 299 if (src.hasRequired()) 300 tgt.setRequiredElement(Boolean43_50.convertBoolean(src.getRequiredElement())); 301 if (src.hasValidated()) 302 tgt.setValidatedElement(Boolean43_50.convertBoolean(src.getValidatedElement())); 303 if (src.hasDescription()) 304 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 305 for (org.hl7.fhir.r4b.model.IntegerType t : src.getOrigin()) tgt.getOrigin().add(Integer43_50.convertInteger(t)); 306 if (src.hasDestination()) 307 tgt.setDestinationElement(Integer43_50.convertInteger(src.getDestinationElement())); 308 for (org.hl7.fhir.r4b.model.UriType t : src.getLink()) tgt.getLink().add(Uri43_50.convertUri(t)); 309 if (src.hasCapabilities()) 310 tgt.setCapabilitiesElement(Canonical43_50.convertCanonical(src.getCapabilitiesElement())); 311 return tgt; 312 } 313 314 public static org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 315 if (src == null) 316 return null; 317 org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptMetadataCapabilityComponent(); 318 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 319 if (src.hasRequired()) 320 tgt.setRequiredElement(Boolean43_50.convertBoolean(src.getRequiredElement())); 321 if (src.hasValidated()) 322 tgt.setValidatedElement(Boolean43_50.convertBoolean(src.getValidatedElement())); 323 if (src.hasDescription()) 324 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 325 for (org.hl7.fhir.r5.model.IntegerType t : src.getOrigin()) tgt.getOrigin().add(Integer43_50.convertInteger(t)); 326 if (src.hasDestination()) 327 tgt.setDestinationElement(Integer43_50.convertInteger(src.getDestinationElement())); 328 for (org.hl7.fhir.r5.model.UriType t : src.getLink()) tgt.getLink().add(Uri43_50.convertUri(t)); 329 if (src.hasCapabilities()) 330 tgt.setCapabilitiesElement(Canonical43_50.convertCanonical(src.getCapabilitiesElement())); 331 return tgt; 332 } 333 334 public static org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 335 if (src == null) 336 return null; 337 org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent(); 338 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 339 if (src.hasAutocreate()) 340 tgt.setAutocreateElement(Boolean43_50.convertBoolean(src.getAutocreateElement())); 341 if (src.hasAutodelete()) 342 tgt.setAutodeleteElement(Boolean43_50.convertBoolean(src.getAutodeleteElement())); 343 if (src.hasResource()) 344 tgt.setResource(Reference43_50.convertReference(src.getResource())); 345 return tgt; 346 } 347 348 public static org.hl7.fhir.r4b.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 349 if (src == null) 350 return null; 351 org.hl7.fhir.r4b.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptFixtureComponent(); 352 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 353 if (src.hasAutocreate()) 354 tgt.setAutocreateElement(Boolean43_50.convertBoolean(src.getAutocreateElement())); 355 if (src.hasAutodelete()) 356 tgt.setAutodeleteElement(Boolean43_50.convertBoolean(src.getAutodeleteElement())); 357 if (src.hasResource()) 358 tgt.setResource(Reference43_50.convertReference(src.getResource())); 359 return tgt; 360 } 361 362 public static org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 363 if (src == null) 364 return null; 365 org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent(); 366 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 367 if (src.hasName()) 368 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 369 if (src.hasDefaultValue()) 370 tgt.setDefaultValueElement(String43_50.convertString(src.getDefaultValueElement())); 371 if (src.hasDescription()) 372 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 373 if (src.hasExpression()) 374 tgt.setExpressionElement(String43_50.convertString(src.getExpressionElement())); 375 if (src.hasHeaderField()) 376 tgt.setHeaderFieldElement(String43_50.convertString(src.getHeaderFieldElement())); 377 if (src.hasHint()) 378 tgt.setHintElement(String43_50.convertString(src.getHintElement())); 379 if (src.hasPath()) 380 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 381 if (src.hasSourceId()) 382 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 383 return tgt; 384 } 385 386 public static org.hl7.fhir.r4b.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 387 if (src == null) 388 return null; 389 org.hl7.fhir.r4b.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptVariableComponent(); 390 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 391 if (src.hasName()) 392 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 393 if (src.hasDefaultValue()) 394 tgt.setDefaultValueElement(String43_50.convertString(src.getDefaultValueElement())); 395 if (src.hasDescription()) 396 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 397 if (src.hasExpression()) 398 tgt.setExpressionElement(String43_50.convertString(src.getExpressionElement())); 399 if (src.hasHeaderField()) 400 tgt.setHeaderFieldElement(String43_50.convertString(src.getHeaderFieldElement())); 401 if (src.hasHint()) 402 tgt.setHintElement(String43_50.convertString(src.getHintElement())); 403 if (src.hasPath()) 404 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 405 if (src.hasSourceId()) 406 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 407 return tgt; 408 } 409 410 public static org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 411 if (src == null) 412 return null; 413 org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent(); 414 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 415 for (org.hl7.fhir.r4b.model.TestScript.SetupActionComponent t : src.getAction()) 416 tgt.addAction(convertSetupActionComponent(t)); 417 return tgt; 418 } 419 420 public static org.hl7.fhir.r4b.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 421 if (src == null) 422 return null; 423 org.hl7.fhir.r4b.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptSetupComponent(); 424 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 425 for (org.hl7.fhir.r5.model.TestScript.SetupActionComponent t : src.getAction()) 426 tgt.addAction(convertSetupActionComponent(t)); 427 return tgt; 428 } 429 430 public static org.hl7.fhir.r5.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r4b.model.TestScript.SetupActionComponent src) throws FHIRException { 431 if (src == null) 432 return null; 433 org.hl7.fhir.r5.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionComponent(); 434 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 435 if (src.hasOperation()) 436 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 437 if (src.hasAssert()) 438 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 439 return tgt; 440 } 441 442 public static org.hl7.fhir.r4b.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r5.model.TestScript.SetupActionComponent src) throws FHIRException { 443 if (src == null) 444 return null; 445 org.hl7.fhir.r4b.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r4b.model.TestScript.SetupActionComponent(); 446 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 447 if (src.hasOperation()) 448 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 449 if (src.hasAssert()) 450 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 451 return tgt; 452 } 453 454 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r4b.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 455 if (src == null) 456 return null; 457 org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent(); 458 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 459 if (src.hasType()) 460 tgt.setType(Coding43_50.convertCoding(src.getType())); 461 if (src.hasResource()) 462 tgt.setResource(src.getResource().toCode()); 463 if (src.hasLabel()) 464 tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 465 if (src.hasDescription()) 466 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 467 if (src.hasAccept()) 468 tgt.setAcceptElement(Code43_50.convertCode(src.getAcceptElement())); 469 if (src.hasContentType()) 470 tgt.setContentTypeElement(Code43_50.convertCode(src.getContentTypeElement())); 471 if (src.hasDestination()) 472 tgt.setDestinationElement(Integer43_50.convertInteger(src.getDestinationElement())); 473 if (src.hasEncodeRequestUrl()) 474 tgt.setEncodeRequestUrlElement(Boolean43_50.convertBoolean(src.getEncodeRequestUrlElement())); 475 if (src.hasMethod()) 476 tgt.setMethodElement(convertTestScriptRequestMethodCode(src.getMethodElement())); 477 if (src.hasOrigin()) 478 tgt.setOriginElement(Integer43_50.convertInteger(src.getOriginElement())); 479 if (src.hasParams()) 480 tgt.setParamsElement(String43_50.convertString(src.getParamsElement())); 481 for (org.hl7.fhir.r4b.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 482 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 483 if (src.hasRequestId()) 484 tgt.setRequestIdElement(Id43_50.convertId(src.getRequestIdElement())); 485 if (src.hasResponseId()) 486 tgt.setResponseIdElement(Id43_50.convertId(src.getResponseIdElement())); 487 if (src.hasSourceId()) 488 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 489 if (src.hasTargetId()) 490 tgt.setTargetIdElement(Id43_50.convertId(src.getTargetIdElement())); 491 if (src.hasUrl()) 492 tgt.setUrlElement(String43_50.convertString(src.getUrlElement())); 493 return tgt; 494 } 495 496 public static org.hl7.fhir.r4b.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 497 if (src == null) 498 return null; 499 org.hl7.fhir.r4b.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r4b.model.TestScript.SetupActionOperationComponent(); 500 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 501 if (src.hasType()) 502 tgt.setType(Coding43_50.convertCoding(src.getType())); 503 if (src.hasResource()) 504 tgt.getResourceElement().setValueAsString(src.getResource()); 505 if (src.hasLabel()) 506 tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 507 if (src.hasDescription()) 508 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 509 if (src.hasAccept()) 510 tgt.setAcceptElement(Code43_50.convertCode(src.getAcceptElement())); 511 if (src.hasContentType()) 512 tgt.setContentTypeElement(Code43_50.convertCode(src.getContentTypeElement())); 513 if (src.hasDestination()) 514 tgt.setDestinationElement(Integer43_50.convertInteger(src.getDestinationElement())); 515 if (src.hasEncodeRequestUrl()) 516 tgt.setEncodeRequestUrlElement(Boolean43_50.convertBoolean(src.getEncodeRequestUrlElement())); 517 if (src.hasMethod()) 518 tgt.setMethodElement(convertTestScriptRequestMethodCode(src.getMethodElement())); 519 if (src.hasOrigin()) 520 tgt.setOriginElement(Integer43_50.convertInteger(src.getOriginElement())); 521 if (src.hasParams()) 522 tgt.setParamsElement(String43_50.convertString(src.getParamsElement())); 523 for (org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 524 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 525 if (src.hasRequestId()) 526 tgt.setRequestIdElement(Id43_50.convertId(src.getRequestIdElement())); 527 if (src.hasResponseId()) 528 tgt.setResponseIdElement(Id43_50.convertId(src.getResponseIdElement())); 529 if (src.hasSourceId()) 530 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 531 if (src.hasTargetId()) 532 tgt.setTargetIdElement(Id43_50.convertId(src.getTargetIdElement())); 533 if (src.hasUrl()) 534 tgt.setUrlElement(String43_50.convertString(src.getUrlElement())); 535 return tgt; 536 } 537 538 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 539 if (src == null || src.isEmpty()) 540 return null; 541 Enumeration<TestScript.TestScriptRequestMethodCode> tgt = new Enumeration<>(new TestScript.TestScriptRequestMethodCodeEnumFactory()); 542 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 543 if (src.getValue() == null) { 544 tgt.setValue(null); 545 } else { 546 switch (src.getValue()) { 547 case DELETE: 548 tgt.setValue(TestScript.TestScriptRequestMethodCode.DELETE); 549 break; 550 case GET: 551 tgt.setValue(TestScript.TestScriptRequestMethodCode.GET); 552 break; 553 case OPTIONS: 554 tgt.setValue(TestScript.TestScriptRequestMethodCode.OPTIONS); 555 break; 556 case PATCH: 557 tgt.setValue(TestScript.TestScriptRequestMethodCode.PATCH); 558 break; 559 case POST: 560 tgt.setValue(TestScript.TestScriptRequestMethodCode.POST); 561 break; 562 case PUT: 563 tgt.setValue(TestScript.TestScriptRequestMethodCode.PUT); 564 break; 565 case HEAD: 566 tgt.setValue(TestScript.TestScriptRequestMethodCode.HEAD); 567 break; 568 default: 569 tgt.setValue(TestScript.TestScriptRequestMethodCode.NULL); 570 break; 571 } 572 } 573 return tgt; 574 } 575 576 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 577 if (src == null || src.isEmpty()) 578 return null; 579 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCodeEnumFactory()); 580 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 581 if (src.getValue() == null) { 582 tgt.setValue(null); 583 } else { 584 switch (src.getValue()) { 585 case DELETE: 586 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.DELETE); 587 break; 588 case GET: 589 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.GET); 590 break; 591 case OPTIONS: 592 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.OPTIONS); 593 break; 594 case PATCH: 595 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.PATCH); 596 break; 597 case POST: 598 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.POST); 599 break; 600 case PUT: 601 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.PUT); 602 break; 603 case HEAD: 604 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.HEAD); 605 break; 606 default: 607 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.TestScriptRequestMethodCode.NULL); 608 break; 609 } 610 } 611 return tgt; 612 } 613 614 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r4b.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 615 if (src == null) 616 return null; 617 org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent(); 618 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 619 if (src.hasField()) 620 tgt.setFieldElement(String43_50.convertString(src.getFieldElement())); 621 if (src.hasValue()) 622 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 623 return tgt; 624 } 625 626 public static org.hl7.fhir.r4b.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 627 if (src == null) 628 return null; 629 org.hl7.fhir.r4b.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r4b.model.TestScript.SetupActionOperationRequestHeaderComponent(); 630 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 631 if (src.hasField()) 632 tgt.setFieldElement(String43_50.convertString(src.getFieldElement())); 633 if (src.hasValue()) 634 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 635 return tgt; 636 } 637 638 public static org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r4b.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 639 if (src == null) 640 return null; 641 org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent(); 642 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 643 if (src.hasLabel()) 644 tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 645 if (src.hasDescription()) 646 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 647 if (src.hasDirection()) 648 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 649 if (src.hasCompareToSourceId()) 650 tgt.setCompareToSourceIdElement(String43_50.convertString(src.getCompareToSourceIdElement())); 651 if (src.hasCompareToSourceExpression()) 652 tgt.setCompareToSourceExpressionElement(String43_50.convertString(src.getCompareToSourceExpressionElement())); 653 if (src.hasCompareToSourcePath()) 654 tgt.setCompareToSourcePathElement(String43_50.convertString(src.getCompareToSourcePathElement())); 655 if (src.hasContentType()) 656 tgt.setContentTypeElement(Code43_50.convertCode(src.getContentTypeElement())); 657 if (src.hasExpression()) 658 tgt.setExpressionElement(String43_50.convertString(src.getExpressionElement())); 659 if (src.hasHeaderField()) 660 tgt.setHeaderFieldElement(String43_50.convertString(src.getHeaderFieldElement())); 661 if (src.hasMinimumId()) 662 tgt.setMinimumIdElement(String43_50.convertString(src.getMinimumIdElement())); 663 if (src.hasNavigationLinks()) 664 tgt.setNavigationLinksElement(Boolean43_50.convertBoolean(src.getNavigationLinksElement())); 665 if (src.hasOperator()) 666 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 667 if (src.hasPath()) 668 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 669 if (src.hasRequestMethod()) 670 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 671 if (src.hasRequestURL()) 672 tgt.setRequestURLElement(String43_50.convertString(src.getRequestURLElement())); 673 if (src.hasResource()) 674 tgt.setResource(src.getResource().toCode()); 675 if (src.hasResponse()) 676 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 677 if (src.hasResponseCode()) 678 tgt.setResponseCodeElement(String43_50.convertString(src.getResponseCodeElement())); 679 if (src.hasSourceId()) 680 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 681 if (src.hasValidateProfileId()) 682 tgt.setValidateProfileIdElement(Id43_50.convertId(src.getValidateProfileIdElement())); 683 if (src.hasValue()) 684 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 685 if (src.hasWarningOnly()) 686 tgt.setWarningOnlyElement(Boolean43_50.convertBoolean(src.getWarningOnlyElement())); 687 return tgt; 688 } 689 690 public static org.hl7.fhir.r4b.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 691 if (src == null) 692 return null; 693 org.hl7.fhir.r4b.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r4b.model.TestScript.SetupActionAssertComponent(); 694 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 695 if (src.hasLabel()) 696 tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 697 if (src.hasDescription()) 698 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 699 if (src.hasDirection()) 700 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 701 if (src.hasCompareToSourceId()) 702 tgt.setCompareToSourceIdElement(String43_50.convertString(src.getCompareToSourceIdElement())); 703 if (src.hasCompareToSourceExpression()) 704 tgt.setCompareToSourceExpressionElement(String43_50.convertString(src.getCompareToSourceExpressionElement())); 705 if (src.hasCompareToSourcePath()) 706 tgt.setCompareToSourcePathElement(String43_50.convertString(src.getCompareToSourcePathElement())); 707 if (src.hasContentType()) 708 tgt.setContentTypeElement(Code43_50.convertCode(src.getContentTypeElement())); 709 if (src.hasExpression()) 710 tgt.setExpressionElement(String43_50.convertString(src.getExpressionElement())); 711 if (src.hasHeaderField()) 712 tgt.setHeaderFieldElement(String43_50.convertString(src.getHeaderFieldElement())); 713 if (src.hasMinimumId()) 714 tgt.setMinimumIdElement(String43_50.convertString(src.getMinimumIdElement())); 715 if (src.hasNavigationLinks()) 716 tgt.setNavigationLinksElement(Boolean43_50.convertBoolean(src.getNavigationLinksElement())); 717 if (src.hasOperator()) 718 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 719 if (src.hasPath()) 720 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 721 if (src.hasRequestMethod()) 722 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 723 if (src.hasRequestURL()) 724 tgt.setRequestURLElement(String43_50.convertString(src.getRequestURLElement())); 725 if (src.hasResource()) 726 tgt.getResourceElement().setValueAsString(src.getResource()); 727 if (src.hasResponse()) 728 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 729 if (src.hasResponseCode()) 730 tgt.setResponseCodeElement(String43_50.convertString(src.getResponseCodeElement())); 731 if (src.hasSourceId()) 732 tgt.setSourceIdElement(Id43_50.convertId(src.getSourceIdElement())); 733 if (src.hasValidateProfileId()) 734 tgt.setValidateProfileIdElement(Id43_50.convertId(src.getValidateProfileIdElement())); 735 if (src.hasValue()) 736 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 737 if (src.hasWarningOnly()) 738 tgt.setWarningOnlyElement(Boolean43_50.convertBoolean(src.getWarningOnlyElement())); 739 return tgt; 740 } 741 742 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType> src) throws FHIRException { 743 if (src == null || src.isEmpty()) 744 return null; 745 Enumeration<TestScript.AssertionDirectionType> tgt = new Enumeration<>(new TestScript.AssertionDirectionTypeEnumFactory()); 746 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 747 if (src.getValue() == null) { 748 tgt.setValue(null); 749 } else { 750 switch (src.getValue()) { 751 case RESPONSE: 752 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 753 break; 754 case REQUEST: 755 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 756 break; 757 default: 758 tgt.setValue(TestScript.AssertionDirectionType.NULL); 759 break; 760 } 761 } 762 return tgt; 763 } 764 765 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> src) throws FHIRException { 766 if (src == null || src.isEmpty()) 767 return null; 768 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestScript.AssertionDirectionTypeEnumFactory()); 769 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 770 if (src.getValue() == null) { 771 tgt.setValue(null); 772 } else { 773 switch (src.getValue()) { 774 case RESPONSE: 775 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType.RESPONSE); 776 break; 777 case REQUEST: 778 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType.REQUEST); 779 break; 780 default: 781 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionDirectionType.NULL); 782 break; 783 } 784 } 785 return tgt; 786 } 787 788 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType> src) throws FHIRException { 789 if (src == null || src.isEmpty()) 790 return null; 791 Enumeration<TestScript.AssertionOperatorType> tgt = new Enumeration<>(new TestScript.AssertionOperatorTypeEnumFactory()); 792 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 793 if (src.getValue() == null) { 794 tgt.setValue(null); 795 } else { 796 switch (src.getValue()) { 797 case EQUALS: 798 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 799 break; 800 case NOTEQUALS: 801 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 802 break; 803 case IN: 804 tgt.setValue(TestScript.AssertionOperatorType.IN); 805 break; 806 case NOTIN: 807 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 808 break; 809 case GREATERTHAN: 810 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 811 break; 812 case LESSTHAN: 813 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 814 break; 815 case EMPTY: 816 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 817 break; 818 case NOTEMPTY: 819 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 820 break; 821 case CONTAINS: 822 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 823 break; 824 case NOTCONTAINS: 825 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 826 break; 827 case EVAL: 828 tgt.setValue(TestScript.AssertionOperatorType.EVAL); 829 break; 830 default: 831 tgt.setValue(TestScript.AssertionOperatorType.NULL); 832 break; 833 } 834 } 835 return tgt; 836 } 837 838 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> src) throws FHIRException { 839 if (src == null || src.isEmpty()) 840 return null; 841 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestScript.AssertionOperatorTypeEnumFactory()); 842 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 843 if (src.getValue() == null) { 844 tgt.setValue(null); 845 } else { 846 switch (src.getValue()) { 847 case EQUALS: 848 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.EQUALS); 849 break; 850 case NOTEQUALS: 851 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.NOTEQUALS); 852 break; 853 case IN: 854 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.IN); 855 break; 856 case NOTIN: 857 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.NOTIN); 858 break; 859 case GREATERTHAN: 860 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.GREATERTHAN); 861 break; 862 case LESSTHAN: 863 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.LESSTHAN); 864 break; 865 case EMPTY: 866 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.EMPTY); 867 break; 868 case NOTEMPTY: 869 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.NOTEMPTY); 870 break; 871 case CONTAINS: 872 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.CONTAINS); 873 break; 874 case NOTCONTAINS: 875 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.NOTCONTAINS); 876 break; 877 case EVAL: 878 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.EVAL); 879 break; 880 default: 881 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionOperatorType.NULL); 882 break; 883 } 884 } 885 return tgt; 886 } 887 888 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 889 if (src == null || src.isEmpty()) 890 return null; 891 Enumeration<TestScript.AssertionResponseTypes> tgt = new Enumeration<>(new TestScript.AssertionResponseTypesEnumFactory()); 892 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 893 if (src.getValue() == null) { 894 tgt.setValue(null); 895 } else { 896 switch (src.getValue()) { 897 case OKAY: 898 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 899 break; 900 case CREATED: 901 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 902 break; 903 case NOCONTENT: 904 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 905 break; 906 case NOTMODIFIED: 907 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 908 break; 909 case BAD: 910 tgt.setValue(TestScript.AssertionResponseTypes.BADREQUEST); 911 break; 912 case FORBIDDEN: 913 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 914 break; 915 case NOTFOUND: 916 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 917 break; 918 case METHODNOTALLOWED: 919 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 920 break; 921 case CONFLICT: 922 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 923 break; 924 case GONE: 925 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 926 break; 927 case PRECONDITIONFAILED: 928 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 929 break; 930 case UNPROCESSABLE: 931 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLECONTENT); 932 break; 933 default: 934 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 935 break; 936 } 937 } 938 return tgt; 939 } 940 941 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 942 if (src == null || src.isEmpty()) 943 return null; 944 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypesEnumFactory()); 945 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 946 if (src.getValue() == null) { 947 tgt.setValue(null); 948 } else { 949 switch (src.getValue()) { 950 case OKAY: 951 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.OKAY); 952 break; 953 case CREATED: 954 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.CREATED); 955 break; 956 case NOCONTENT: 957 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.NOCONTENT); 958 break; 959 case NOTMODIFIED: 960 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 961 break; 962 case BADREQUEST: 963 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.BAD); 964 break; 965 case FORBIDDEN: 966 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.FORBIDDEN); 967 break; 968 case NOTFOUND: 969 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.NOTFOUND); 970 break; 971 case METHODNOTALLOWED: 972 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 973 break; 974 case CONFLICT: 975 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.CONFLICT); 976 break; 977 case GONE: 978 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.GONE); 979 break; 980 case PRECONDITIONFAILED: 981 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 982 break; 983 case UNPROCESSABLECONTENT: 984 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 985 break; 986 default: 987 tgt.setValue(org.hl7.fhir.r4b.model.TestScript.AssertionResponseTypes.NULL); 988 break; 989 } 990 } 991 return tgt; 992 } 993 994 public static org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptTestComponent src) throws FHIRException { 995 if (src == null) 996 return null; 997 org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent(); 998 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 999 if (src.hasName()) 1000 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 1001 if (src.hasDescription()) 1002 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 1003 for (org.hl7.fhir.r4b.model.TestScript.TestActionComponent t : src.getAction()) 1004 tgt.addAction(convertTestActionComponent(t)); 1005 return tgt; 1006 } 1007 1008 public static org.hl7.fhir.r4b.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent src) throws FHIRException { 1009 if (src == null) 1010 return null; 1011 org.hl7.fhir.r4b.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptTestComponent(); 1012 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1013 if (src.hasName()) 1014 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 1015 if (src.hasDescription()) 1016 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 1017 for (org.hl7.fhir.r5.model.TestScript.TestActionComponent t : src.getAction()) 1018 tgt.addAction(convertTestActionComponent(t)); 1019 return tgt; 1020 } 1021 1022 public static org.hl7.fhir.r5.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.r4b.model.TestScript.TestActionComponent src) throws FHIRException { 1023 if (src == null) 1024 return null; 1025 org.hl7.fhir.r5.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestActionComponent(); 1026 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1027 if (src.hasOperation()) 1028 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 1029 if (src.hasAssert()) 1030 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 1031 return tgt; 1032 } 1033 1034 public static org.hl7.fhir.r4b.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.r5.model.TestScript.TestActionComponent src) throws FHIRException { 1035 if (src == null) 1036 return null; 1037 org.hl7.fhir.r4b.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestActionComponent(); 1038 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1039 if (src.hasOperation()) 1040 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 1041 if (src.hasAssert()) 1042 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 1043 return tgt; 1044 } 1045 1046 public static org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r4b.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 1047 if (src == null) 1048 return null; 1049 org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent(); 1050 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1051 for (org.hl7.fhir.r4b.model.TestScript.TeardownActionComponent t : src.getAction()) 1052 tgt.addAction(convertTeardownActionComponent(t)); 1053 return tgt; 1054 } 1055 1056 public static org.hl7.fhir.r4b.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 1057 if (src == null) 1058 return null; 1059 org.hl7.fhir.r4b.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TestScriptTeardownComponent(); 1060 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1061 for (org.hl7.fhir.r5.model.TestScript.TeardownActionComponent t : src.getAction()) 1062 tgt.addAction(convertTeardownActionComponent(t)); 1063 return tgt; 1064 } 1065 1066 public static org.hl7.fhir.r5.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r4b.model.TestScript.TeardownActionComponent src) throws FHIRException { 1067 if (src == null) 1068 return null; 1069 org.hl7.fhir.r5.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TeardownActionComponent(); 1070 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1071 if (src.hasOperation()) 1072 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 1073 return tgt; 1074 } 1075 1076 public static org.hl7.fhir.r4b.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r5.model.TestScript.TeardownActionComponent src) throws FHIRException { 1077 if (src == null) 1078 return null; 1079 org.hl7.fhir.r4b.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r4b.model.TestScript.TeardownActionComponent(); 1080 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 1081 if (src.hasOperation()) 1082 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 1083 return tgt; 1084 } 1085}