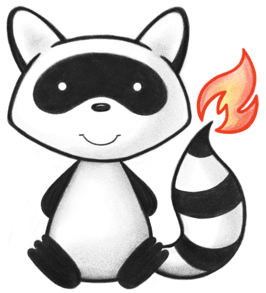
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext40_50; 005import org.hl7.fhir.convertors.context.ConversionContext43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Integer43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 019import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 020import org.hl7.fhir.exceptions.FHIRException; 021import org.hl7.fhir.r5.model.Enumeration; 022import org.hl7.fhir.r5.model.Enumerations; 023import org.hl7.fhir.r5.model.ValueSet; 024 025/* 026 Copyright (c) 2011+, HL7, Inc. 027 All rights reserved. 028 029 Redistribution and use in source and binary forms, with or without modification, 030 are permitted provided that the following conditions are met: 031 032 * Redistributions of source code must retain the above copyright notice, this 033 list of conditions and the following disclaimer. 034 * Redistributions in binary form must reproduce the above copyright notice, 035 this list of conditions and the following disclaimer in the documentation 036 and/or other materials provided with the distribution. 037 * Neither the name of HL7 nor the names of its contributors may be used to 038 endorse or promote products derived from this software without specific 039 prior written permission. 040 041 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 042 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 043 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 044 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 045 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 046 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 047 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 048 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 049 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 050 POSSIBILITY OF SUCH DAMAGE. 051 052*/ 053// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 054public class ValueSet43_50 { 055 056 public static org.hl7.fhir.r5.model.ValueSet convertValueSet(org.hl7.fhir.r4b.model.ValueSet src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.r5.model.ValueSet tgt = new org.hl7.fhir.r5.model.ValueSet(); 060 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 061 if (src.hasUrl()) 062 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 063 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 064 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 065 if (src.hasVersion()) 066 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 067 if (src.hasName()) 068 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 069 if (src.hasTitle()) 070 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 071 if (src.hasStatus()) 072 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 073 if (src.hasExperimental()) 074 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 075 if (src.hasDate()) 076 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 077 if (src.hasPublisher()) 078 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 079 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 080 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 081 if (src.hasDescription()) 082 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 083 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 084 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 085 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 086 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 087 if (src.hasImmutable()) 088 tgt.setImmutableElement(Boolean43_50.convertBoolean(src.getImmutableElement())); 089 if (src.hasPurpose()) 090 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 091 if (src.hasCopyright()) 092 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 093 if (src.hasCompose()) 094 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 095 if (src.hasExpansion()) 096 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.r4b.model.ValueSet convertValueSet(org.hl7.fhir.r5.model.ValueSet src) throws FHIRException { 101 if (src == null) 102 return null; 103 org.hl7.fhir.r4b.model.ValueSet tgt = new org.hl7.fhir.r4b.model.ValueSet(); 104 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 105 if (src.hasUrl()) 106 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 107 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 108 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 109 if (src.hasVersion()) 110 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 111 if (src.hasName()) 112 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 113 if (src.hasTitle()) 114 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 115 if (src.hasStatus()) 116 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 117 if (src.hasExperimental()) 118 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 119 if (src.hasDate()) 120 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 121 if (src.hasPublisher()) 122 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 123 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 124 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 125 if (src.hasDescription()) 126 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 127 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 128 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 129 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 130 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 131 if (src.hasImmutable()) 132 tgt.setImmutableElement(Boolean43_50.convertBoolean(src.getImmutableElement())); 133 if (src.hasPurpose()) 134 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 135 if (src.hasCopyright()) 136 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 137 if (src.hasCompose()) 138 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 139 if (src.hasExpansion()) 140 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r4b.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 145 if (src == null) 146 return null; 147 org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent(); 148 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 149 if (src.hasLockedDate()) 150 tgt.setLockedDateElement(Date43_50.convertDate(src.getLockedDateElement())); 151 if (src.hasInactive()) 152 tgt.setInactiveElement(Boolean43_50.convertBoolean(src.getInactiveElement())); 153 for (org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent t : src.getInclude()) 154 tgt.addInclude(convertConceptSetComponent(t)); 155 for (org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent t : src.getExclude()) 156 tgt.addExclude(convertConceptSetComponent(t)); 157 return tgt; 158 } 159 160 public static org.hl7.fhir.r4b.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 161 if (src == null) 162 return null; 163 org.hl7.fhir.r4b.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ValueSetComposeComponent(); 164 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 165 if (src.hasLockedDate()) 166 tgt.setLockedDateElement(Date43_50.convertDate(src.getLockedDateElement())); 167 if (src.hasInactive()) 168 tgt.setInactiveElement(Boolean43_50.convertBoolean(src.getInactiveElement())); 169 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getInclude()) 170 tgt.addInclude(convertConceptSetComponent(t)); 171 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getExclude()) 172 tgt.addExclude(convertConceptSetComponent(t)); 173 return tgt; 174 } 175 176 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent src) throws FHIRException { 177 if (src == null) 178 return null; 179 org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent(); 180 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 181 if (src.hasSystem()) 182 tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 183 if (src.hasVersion()) 184 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 185 for (org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 186 tgt.addConcept(convertConceptReferenceComponent(t)); 187 for (org.hl7.fhir.r4b.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 188 tgt.addFilter(convertConceptSetFilterComponent(t)); 189 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getValueSet()) 190 tgt.getValueSet().add(Canonical43_50.convertCanonical(t)); 191 return tgt; 192 } 193 194 public static org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent src) throws FHIRException { 195 if (src == null) 196 return null; 197 org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ConceptSetComponent(); 198 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 199 if (src.hasSystem()) 200 tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 201 if (src.hasVersion()) 202 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 203 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 204 tgt.addConcept(convertConceptReferenceComponent(t)); 205 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 206 tgt.addFilter(convertConceptSetFilterComponent(t)); 207 for (org.hl7.fhir.r5.model.CanonicalType t : src.getValueSet()) 208 tgt.getValueSet().add(Canonical43_50.convertCanonical(t)); 209 return tgt; 210 } 211 212 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 213 if (src == null) 214 return null; 215 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent(); 216 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 217 if (src.hasCode()) 218 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 219 if (src.hasDisplay()) 220 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 221 for (org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 222 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 223 return tgt; 224 } 225 226 public static org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 227 if (src == null) 228 return null; 229 org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceComponent(); 230 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 231 if (src.hasCode()) 232 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 233 if (src.hasDisplay()) 234 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 235 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 236 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 237 return tgt; 238 } 239 240 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 241 if (src == null) 242 return null; 243 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent(); 244 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 245 if (src.hasLanguage()) 246 tgt.setLanguageElement(Code43_50.convertCode(src.getLanguageElement())); 247 if (src.hasUse()) 248 tgt.setUse(Coding43_50.convertCoding(src.getUse())); 249 if (src.hasValue()) 250 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 251 return tgt; 252 } 253 254 public static org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 255 if (src == null) 256 return null; 257 org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent(); 258 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 259 if (src.hasLanguage()) 260 tgt.setLanguageElement(Code43_50.convertCode(src.getLanguageElement())); 261 if (src.hasUse()) 262 tgt.setUse(Coding43_50.convertCoding(src.getUse())); 263 if (src.hasValue()) 264 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 265 return tgt; 266 } 267 268 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r4b.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 269 if (src == null) 270 return null; 271 org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent(); 272 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 273 if (src.hasProperty()) 274 tgt.setPropertyElement(Code43_50.convertCode(src.getPropertyElement())); 275 if (src.hasOp()) 276 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 277 if (src.hasValue()) 278 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 279 return tgt; 280 } 281 282 public static org.hl7.fhir.r4b.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 283 if (src == null) 284 return null; 285 org.hl7.fhir.r4b.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ConceptSetFilterComponent(); 286 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 287 if (src.hasProperty()) 288 tgt.setPropertyElement(Code43_50.convertCode(src.getPropertyElement())); 289 if (src.hasOp()) 290 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 291 if (src.hasValue()) 292 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 293 return tgt; 294 } 295 296 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> src) throws FHIRException { 297 if (src == null || src.isEmpty()) 298 return null; 299 Enumeration<Enumerations.FilterOperator> tgt = new Enumeration<>(new Enumerations.FilterOperatorEnumFactory()); 300 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 301 if (src.getValue() == null) { 302 tgt.setValue(null); 303 } else { 304 switch (src.getValue()) { 305 case EQUAL: 306 tgt.setValue(Enumerations.FilterOperator.EQUAL); 307 break; 308 case ISA: 309 tgt.setValue(Enumerations.FilterOperator.ISA); 310 break; 311 case DESCENDENTOF: 312 tgt.setValue(Enumerations.FilterOperator.DESCENDENTOF); 313 break; 314 case ISNOTA: 315 tgt.setValue(Enumerations.FilterOperator.ISNOTA); 316 break; 317 case REGEX: 318 tgt.setValue(Enumerations.FilterOperator.REGEX); 319 break; 320 case IN: 321 tgt.setValue(Enumerations.FilterOperator.IN); 322 break; 323 case NOTIN: 324 tgt.setValue(Enumerations.FilterOperator.NOTIN); 325 break; 326 case GENERALIZES: 327 tgt.setValue(Enumerations.FilterOperator.GENERALIZES); 328 break; 329 case EXISTS: 330 tgt.setValue(Enumerations.FilterOperator.EXISTS); 331 break; 332 default: 333 tgt.setValue(Enumerations.FilterOperator.NULL); 334 break; 335 } 336 } 337 return tgt; 338 } 339 340 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> src) throws FHIRException { 341 if (src == null || src.isEmpty()) 342 return null; 343 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.FilterOperatorEnumFactory()); 344 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 345 if (src.getValue() == null) { 346 tgt.setValue(null); 347 } else { 348 switch (src.getValue()) { 349 case EQUAL: 350 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.EQUAL); 351 break; 352 case ISA: 353 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.ISA); 354 break; 355 case DESCENDENTOF: 356 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.DESCENDENTOF); 357 break; 358 case ISNOTA: 359 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.ISNOTA); 360 break; 361 case REGEX: 362 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.REGEX); 363 break; 364 case IN: 365 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.IN); 366 break; 367 case NOTIN: 368 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.NOTIN); 369 break; 370 case GENERALIZES: 371 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.GENERALIZES); 372 break; 373 case EXISTS: 374 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.EXISTS); 375 break; 376 default: 377 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.NULL); 378 break; 379 } 380 } 381 return tgt; 382 } 383 384 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 385 if (src == null) 386 return null; 387 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent(); 388 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt, VersionConvertorConstants.EXT_VS_EXP_PROP); 389 if (src.hasIdentifier()) 390 tgt.setIdentifierElement(Uri43_50.convertUri(src.getIdentifierElement())); 391 if (src.hasTimestamp()) 392 tgt.setTimestampElement(DateTime43_50.convertDateTime(src.getTimestampElement())); 393 if (src.hasTotal()) 394 tgt.setTotalElement(Integer43_50.convertInteger(src.getTotalElement())); 395 if (src.hasOffset()) 396 tgt.setOffsetElement(Integer43_50.convertInteger(src.getOffsetElement())); 397 for (org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 398 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 399 for (org.hl7.fhir.r4b.model.Extension t : src.getExtension()) { 400 if (VersionConvertorConstants.EXT_VS_EXP_PROP.equals(t.getUrl())) { 401 tgt.addProperty().setCode(t.getExtensionString("code")).setUri(t.getExtensionString("uri")); 402 } 403 } 404 for (org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 405 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 406 return tgt; 407 } 408 409 public static org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 410 if (src == null) 411 return null; 412 org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionComponent(); 413 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 414 if (src.hasIdentifier()) 415 tgt.setIdentifierElement(Uri43_50.convertUri(src.getIdentifierElement())); 416 if (src.hasTimestamp()) 417 tgt.setTimestampElement(DateTime43_50.convertDateTime(src.getTimestampElement())); 418 if (src.hasTotal()) 419 tgt.setTotalElement(Integer43_50.convertInteger(src.getTotalElement())); 420 if (src.hasOffset()) 421 tgt.setOffsetElement(Integer43_50.convertInteger(src.getOffsetElement())); 422 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 423 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 424 for (ValueSet.ValueSetExpansionPropertyComponent t : src.getProperty()) { 425 org.hl7.fhir.r4b.model.Extension ext = tgt.addExtension().setUrl(VersionConvertorConstants.EXT_VS_EXP_PROP); 426 ext.addExtension().setUrl("code").setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getCodeElement())); 427 ext.addExtension().setUrl("uri").setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getUriElement())); 428 } 429 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 430 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 431 return tgt; 432 } 433 434 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 435 if (src == null) 436 return null; 437 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent(); 438 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 439 if (src.hasName()) 440 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 441 if (src.hasValue()) 442 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 443 return tgt; 444 } 445 446 public static org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 447 if (src == null) 448 return null; 449 org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionParameterComponent(); 450 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 451 if (src.hasName()) 452 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 453 if (src.hasValue()) 454 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 455 return tgt; 456 } 457 458 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 459 if (src == null) 460 return null; 461 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent(); 462 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 463 if (src.hasSystem()) 464 tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 465 if (src.hasAbstract()) 466 tgt.setAbstractElement(Boolean43_50.convertBoolean(src.getAbstractElement())); 467 if (src.hasInactive()) 468 tgt.setInactiveElement(Boolean43_50.convertBoolean(src.getInactiveElement())); 469 if (src.hasVersion()) 470 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 471 if (src.hasCode()) 472 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 473 if (src.hasDisplay()) 474 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 475 for (org.hl7.fhir.r4b.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 476 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 477 for (org.hl7.fhir.r4b.model.Extension t : src.getExtension()) { 478 if (VersionConvertorConstants.EXT_EXP_VS_CONT_PROP.equals(t.getUrl())) { 479 ValueSet.ConceptPropertyComponent prop = tgt.addProperty(); 480 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(t, prop, "code", "value[x]"); 481 prop.setCode(t.getExtensionString("code")); 482 if (t.hasExtension("value")) { 483 prop.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getExtensionByUrl("value").getValue())); 484 } else if (t.hasExtension("value[x]")) { 485 prop.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getExtensionByUrl("value[x]").getValue())); 486 } 487 } 488 } 489 for (org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 490 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 491 return tgt; 492 } 493 494 public static org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 495 if (src == null) 496 return null; 497 org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r4b.model.ValueSet.ValueSetExpansionContainsComponent(); 498 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 499 if (src.hasSystem()) 500 tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 501 if (src.hasAbstract()) 502 tgt.setAbstractElement(Boolean43_50.convertBoolean(src.getAbstractElement())); 503 if (src.hasInactive()) 504 tgt.setInactiveElement(Boolean43_50.convertBoolean(src.getInactiveElement())); 505 if (src.hasVersion()) 506 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 507 if (src.hasCode()) 508 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 509 if (src.hasDisplay()) 510 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 511 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 512 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 513 for (org.hl7.fhir.r5.model.ValueSet.ConceptPropertyComponent t : src.getProperty()) { 514 org.hl7.fhir.r4b.model.Extension ext = tgt.addExtension().setUrl(VersionConvertorConstants.EXT_EXP_VS_CONT_PROP); 515 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(t, ext, "code", "value[x]"); 516 ext.addExtension().setUrl("code").setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getCodeElement())); 517 ext.addExtension().setUrl("value[x]").setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(t.getValue())); 518 } 519 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 520 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 521 return tgt; 522 } 523}