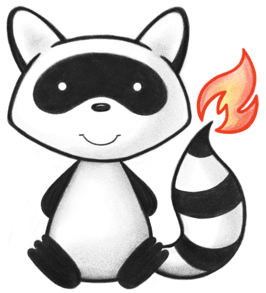
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Decimal43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Integer43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations; 016import org.hl7.fhir.r5.model.VisionPrescription; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class VisionPrescription43_50 { 048 049 public static org.hl7.fhir.r5.model.VisionPrescription convertVisionPrescription(org.hl7.fhir.r4b.model.VisionPrescription src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.VisionPrescription tgt = new org.hl7.fhir.r5.model.VisionPrescription(); 053 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertVisionStatus(src.getStatusElement())); 058 if (src.hasCreated()) 059 tgt.setCreatedElement(DateTime43_50.convertDateTime(src.getCreatedElement())); 060 if (src.hasPatient()) 061 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 062 if (src.hasEncounter()) 063 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 064 if (src.hasDateWritten()) 065 tgt.setDateWrittenElement(DateTime43_50.convertDateTime(src.getDateWrittenElement())); 066 if (src.hasPrescriber()) 067 tgt.setPrescriber(Reference43_50.convertReference(src.getPrescriber())); 068 for (org.hl7.fhir.r4b.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent t : src.getLensSpecification()) 069 tgt.addLensSpecification(convertVisionPrescriptionLensSpecificationComponent(t)); 070 return tgt; 071 } 072 073 public static org.hl7.fhir.r4b.model.VisionPrescription convertVisionPrescription(org.hl7.fhir.r5.model.VisionPrescription src) throws FHIRException { 074 if (src == null) 075 return null; 076 org.hl7.fhir.r4b.model.VisionPrescription tgt = new org.hl7.fhir.r4b.model.VisionPrescription(); 077 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 078 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 079 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 080 if (src.hasStatus()) 081 tgt.setStatusElement(convertVisionStatus(src.getStatusElement())); 082 if (src.hasCreated()) 083 tgt.setCreatedElement(DateTime43_50.convertDateTime(src.getCreatedElement())); 084 if (src.hasPatient()) 085 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 086 if (src.hasEncounter()) 087 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 088 if (src.hasDateWritten()) 089 tgt.setDateWrittenElement(DateTime43_50.convertDateTime(src.getDateWrittenElement())); 090 if (src.hasPrescriber()) 091 tgt.setPrescriber(Reference43_50.convertReference(src.getPrescriber())); 092 for (org.hl7.fhir.r5.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent t : src.getLensSpecification()) 093 tgt.addLensSpecification(convertVisionPrescriptionLensSpecificationComponent(t)); 094 return tgt; 095 } 096 097 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> convertVisionStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> src) throws FHIRException { 098 if (src == null || src.isEmpty()) 099 return null; 100 Enumeration<Enumerations.FinancialResourceStatusCodes> tgt = new Enumeration<>(new Enumerations.FinancialResourceStatusCodesEnumFactory()); 101 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 102 if (src.getValue() == null) { 103 tgt.setValue(null); 104 } else { 105 switch (src.getValue()) { 106 case ACTIVE: 107 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ACTIVE); 108 break; 109 case CANCELLED: 110 tgt.setValue(Enumerations.FinancialResourceStatusCodes.CANCELLED); 111 break; 112 case DRAFT: 113 tgt.setValue(Enumerations.FinancialResourceStatusCodes.DRAFT); 114 break; 115 case ENTEREDINERROR: 116 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ENTEREDINERROR); 117 break; 118 default: 119 tgt.setValue(Enumerations.FinancialResourceStatusCodes.NULL); 120 break; 121 } 122 } 123 return tgt; 124 } 125 126 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> convertVisionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> src) throws FHIRException { 127 if (src == null || src.isEmpty()) 128 return null; 129 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodesEnumFactory()); 130 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 131 if (src.getValue() == null) { 132 tgt.setValue(null); 133 } else { 134 switch (src.getValue()) { 135 case ACTIVE: 136 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.ACTIVE); 137 break; 138 case CANCELLED: 139 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.CANCELLED); 140 break; 141 case DRAFT: 142 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.DRAFT); 143 break; 144 case ENTEREDINERROR: 145 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.ENTEREDINERROR); 146 break; 147 default: 148 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.NULL); 149 break; 150 } 151 } 152 return tgt; 153 } 154 155 public static org.hl7.fhir.r5.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent convertVisionPrescriptionLensSpecificationComponent(org.hl7.fhir.r4b.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r5.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent tgt = new org.hl7.fhir.r5.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent(); 159 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 160 if (src.hasProduct()) 161 tgt.setProduct(CodeableConcept43_50.convertCodeableConcept(src.getProduct())); 162 if (src.hasEye()) 163 tgt.setEyeElement(convertVisionEyes(src.getEyeElement())); 164 if (src.hasSphere()) 165 tgt.setSphereElement(Decimal43_50.convertDecimal(src.getSphereElement())); 166 if (src.hasCylinder()) 167 tgt.setCylinderElement(Decimal43_50.convertDecimal(src.getCylinderElement())); 168 if (src.hasAxis()) 169 tgt.setAxisElement(Integer43_50.convertInteger(src.getAxisElement())); 170 for (org.hl7.fhir.r4b.model.VisionPrescription.PrismComponent t : src.getPrism()) 171 tgt.addPrism(convertPrismComponent(t)); 172 if (src.hasAdd()) 173 tgt.setAddElement(Decimal43_50.convertDecimal(src.getAddElement())); 174 if (src.hasPower()) 175 tgt.setPowerElement(Decimal43_50.convertDecimal(src.getPowerElement())); 176 if (src.hasBackCurve()) 177 tgt.setBackCurveElement(Decimal43_50.convertDecimal(src.getBackCurveElement())); 178 if (src.hasDiameter()) 179 tgt.setDiameterElement(Decimal43_50.convertDecimal(src.getDiameterElement())); 180 if (src.hasDuration()) 181 tgt.setDuration(SimpleQuantity43_50.convertSimpleQuantity(src.getDuration())); 182 if (src.hasColor()) 183 tgt.setColorElement(String43_50.convertString(src.getColorElement())); 184 if (src.hasBrand()) 185 tgt.setBrandElement(String43_50.convertString(src.getBrandElement())); 186 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 187 return tgt; 188 } 189 190 public static org.hl7.fhir.r4b.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent convertVisionPrescriptionLensSpecificationComponent(org.hl7.fhir.r5.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent src) throws FHIRException { 191 if (src == null) 192 return null; 193 org.hl7.fhir.r4b.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent tgt = new org.hl7.fhir.r4b.model.VisionPrescription.VisionPrescriptionLensSpecificationComponent(); 194 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 195 if (src.hasProduct()) 196 tgt.setProduct(CodeableConcept43_50.convertCodeableConcept(src.getProduct())); 197 if (src.hasEye()) 198 tgt.setEyeElement(convertVisionEyes(src.getEyeElement())); 199 if (src.hasSphere()) 200 tgt.setSphereElement(Decimal43_50.convertDecimal(src.getSphereElement())); 201 if (src.hasCylinder()) 202 tgt.setCylinderElement(Decimal43_50.convertDecimal(src.getCylinderElement())); 203 if (src.hasAxis()) 204 tgt.setAxisElement(Integer43_50.convertInteger(src.getAxisElement())); 205 for (org.hl7.fhir.r5.model.VisionPrescription.PrismComponent t : src.getPrism()) 206 tgt.addPrism(convertPrismComponent(t)); 207 if (src.hasAdd()) 208 tgt.setAddElement(Decimal43_50.convertDecimal(src.getAddElement())); 209 if (src.hasPower()) 210 tgt.setPowerElement(Decimal43_50.convertDecimal(src.getPowerElement())); 211 if (src.hasBackCurve()) 212 tgt.setBackCurveElement(Decimal43_50.convertDecimal(src.getBackCurveElement())); 213 if (src.hasDiameter()) 214 tgt.setDiameterElement(Decimal43_50.convertDecimal(src.getDiameterElement())); 215 if (src.hasDuration()) 216 tgt.setDuration(SimpleQuantity43_50.convertSimpleQuantity(src.getDuration())); 217 if (src.hasColor()) 218 tgt.setColorElement(String43_50.convertString(src.getColorElement())); 219 if (src.hasBrand()) 220 tgt.setBrandElement(String43_50.convertString(src.getBrandElement())); 221 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 222 return tgt; 223 } 224 225 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VisionPrescription.VisionEyes> convertVisionEyes(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes> src) throws FHIRException { 226 if (src == null || src.isEmpty()) 227 return null; 228 Enumeration<VisionPrescription.VisionEyes> tgt = new Enumeration<>(new VisionPrescription.VisionEyesEnumFactory()); 229 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 230 if (src.getValue() == null) { 231 tgt.setValue(null); 232 } else { 233 switch (src.getValue()) { 234 case RIGHT: 235 tgt.setValue(VisionPrescription.VisionEyes.RIGHT); 236 break; 237 case LEFT: 238 tgt.setValue(VisionPrescription.VisionEyes.LEFT); 239 break; 240 default: 241 tgt.setValue(VisionPrescription.VisionEyes.NULL); 242 break; 243 } 244 } 245 return tgt; 246 } 247 248 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes> convertVisionEyes(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VisionPrescription.VisionEyes> src) throws FHIRException { 249 if (src == null || src.isEmpty()) 250 return null; 251 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.VisionPrescription.VisionEyesEnumFactory()); 252 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 253 if (src.getValue() == null) { 254 tgt.setValue(null); 255 } else { 256 switch (src.getValue()) { 257 case RIGHT: 258 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes.RIGHT); 259 break; 260 case LEFT: 261 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes.LEFT); 262 break; 263 default: 264 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionEyes.NULL); 265 break; 266 } 267 } 268 return tgt; 269 } 270 271 public static org.hl7.fhir.r5.model.VisionPrescription.PrismComponent convertPrismComponent(org.hl7.fhir.r4b.model.VisionPrescription.PrismComponent src) throws FHIRException { 272 if (src == null) 273 return null; 274 org.hl7.fhir.r5.model.VisionPrescription.PrismComponent tgt = new org.hl7.fhir.r5.model.VisionPrescription.PrismComponent(); 275 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 276 if (src.hasAmount()) 277 tgt.setAmountElement(Decimal43_50.convertDecimal(src.getAmountElement())); 278 if (src.hasBase()) 279 tgt.setBaseElement(convertVisionBase(src.getBaseElement())); 280 return tgt; 281 } 282 283 public static org.hl7.fhir.r4b.model.VisionPrescription.PrismComponent convertPrismComponent(org.hl7.fhir.r5.model.VisionPrescription.PrismComponent src) throws FHIRException { 284 if (src == null) 285 return null; 286 org.hl7.fhir.r4b.model.VisionPrescription.PrismComponent tgt = new org.hl7.fhir.r4b.model.VisionPrescription.PrismComponent(); 287 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 288 if (src.hasAmount()) 289 tgt.setAmountElement(Decimal43_50.convertDecimal(src.getAmountElement())); 290 if (src.hasBase()) 291 tgt.setBaseElement(convertVisionBase(src.getBaseElement())); 292 return tgt; 293 } 294 295 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VisionPrescription.VisionBase> convertVisionBase(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionBase> src) throws FHIRException { 296 if (src == null || src.isEmpty()) 297 return null; 298 Enumeration<VisionPrescription.VisionBase> tgt = new Enumeration<>(new VisionPrescription.VisionBaseEnumFactory()); 299 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 300 if (src.getValue() == null) { 301 tgt.setValue(null); 302 } else { 303 switch (src.getValue()) { 304 case UP: 305 tgt.setValue(VisionPrescription.VisionBase.UP); 306 break; 307 case DOWN: 308 tgt.setValue(VisionPrescription.VisionBase.DOWN); 309 break; 310 case IN: 311 tgt.setValue(VisionPrescription.VisionBase.IN); 312 break; 313 case OUT: 314 tgt.setValue(VisionPrescription.VisionBase.OUT); 315 break; 316 default: 317 tgt.setValue(VisionPrescription.VisionBase.NULL); 318 break; 319 } 320 } 321 return tgt; 322 } 323 324 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionBase> convertVisionBase(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VisionPrescription.VisionBase> src) throws FHIRException { 325 if (src == null || src.isEmpty()) 326 return null; 327 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.VisionPrescription.VisionBase> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.VisionPrescription.VisionBaseEnumFactory()); 328 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 329 if (src.getValue() == null) { 330 tgt.setValue(null); 331 } else { 332 switch (src.getValue()) { 333 case UP: 334 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionBase.UP); 335 break; 336 case DOWN: 337 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionBase.DOWN); 338 break; 339 case IN: 340 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionBase.IN); 341 break; 342 case OUT: 343 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionBase.OUT); 344 break; 345 default: 346 tgt.setValue(org.hl7.fhir.r4b.model.VisionPrescription.VisionBase.NULL); 347 break; 348 } 349 } 350 return tgt; 351 } 352}