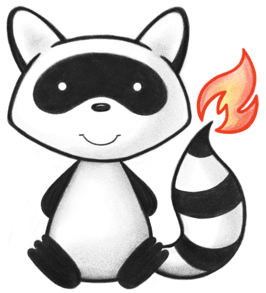
001package org.hl7.fhir.convertors.factory; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.utilities.Utilities; 007 008public final class VersionConvertorFactory_14_30 extends VersionConvertorFactory { 009 010 public static org.hl7.fhir.dstu3.model.Resource convertResource(org.hl7.fhir.dstu2016may.model.Resource src) throws FHIRException { 011 return convertResource(src, new BaseAdvisor_14_30()); 012 } 013 014 public static org.hl7.fhir.dstu3.model.Resource convertResource(org.hl7.fhir.dstu2016may.model.Resource src, BaseAdvisor_14_30 advisor) throws FHIRException { 015 cleanInputs(src, advisor); 016 return src != null ? new VersionConvertor_14_30(advisor).convertResource(src) : null; 017 } 018 019 public static org.hl7.fhir.dstu2016may.model.Resource convertResource(org.hl7.fhir.dstu3.model.Resource src) throws FHIRException { 020 return convertResource(src, new BaseAdvisor_14_30()); 021 } 022 023 public static org.hl7.fhir.dstu2016may.model.Resource convertResource(org.hl7.fhir.dstu3.model.Resource src, BaseAdvisor_14_30 advisor) throws FHIRException { 024 cleanInputs(src, advisor); 025 return src != null ? new VersionConvertor_14_30(advisor).convertResource(src) : null; 026 } 027 028 public static org.hl7.fhir.dstu3.model.Type convertType(org.hl7.fhir.dstu2016may.model.Type src) throws FHIRException { 029 return convertType(src, new BaseAdvisor_14_30()); 030 } 031 032 public static org.hl7.fhir.dstu3.model.Type convertType(org.hl7.fhir.dstu2016may.model.Type src, BaseAdvisor_14_30 advisor) throws FHIRException { 033 cleanInputs(src, advisor); 034 return src != null ? new VersionConvertor_14_30(advisor).convertType(src) : null; 035 } 036 037 public static org.hl7.fhir.dstu2016may.model.Type convertType(org.hl7.fhir.dstu3.model.Type src) throws FHIRException { 038 return convertType(src, new BaseAdvisor_14_30()); 039 } 040 041 public static org.hl7.fhir.dstu2016may.model.Type convertType(org.hl7.fhir.dstu3.model.Type src, BaseAdvisor_14_30 advisor) throws FHIRException { 042 cleanInputs(src, advisor); 043 return src != null ? new VersionConvertor_14_30(advisor).convertType(src) : null; 044 } 045 046 public static boolean convertsResource(String rt) { 047 return Utilities.existsInList(rt, "Parameters", "Bundle", "CodeSystem", "CompartmentDefinition", "ConceptMap", "CapabilityStatement", "DataElement", "ImplementationGuide", "NamingSystem", "OperationDefinition", "OperationOutcome", "Questionnaire", "QuestionnaireResponse", "SearchParameter", "StructureDefinition", "TestScript", "ValueSet"); 048 } 049}