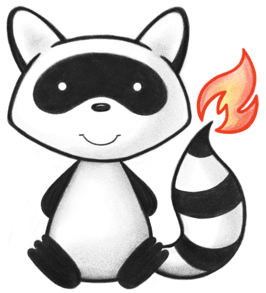
001package org.hl7.fhir.convertors.factory; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_40_50; 004import org.hl7.fhir.convertors.conv40_50.VersionConvertor_40_50; 005import org.hl7.fhir.exceptions.FHIRException; 006 007public final class VersionConvertorFactory_40_50 extends VersionConvertorFactory { 008 009 public static org.hl7.fhir.r5.model.Resource convertResource(org.hl7.fhir.r4.model.Resource src) throws FHIRException { 010 return convertResource(src, new BaseAdvisor_40_50()); 011 } 012 013 public static org.hl7.fhir.r5.model.Resource convertResource(org.hl7.fhir.r4.model.Resource src, BaseAdvisor_40_50 advisor) throws FHIRException { 014 cleanInputs(src, advisor); 015 return src != null ? new VersionConvertor_40_50(advisor).convertResource(src) : null; 016 } 017 018 public static org.hl7.fhir.r4.model.Resource convertResource(org.hl7.fhir.r5.model.Resource src) throws FHIRException { 019 return convertResource(src, new BaseAdvisor_40_50()); 020 } 021 022 public static org.hl7.fhir.r4.model.Resource convertResource(org.hl7.fhir.r5.model.Resource src, BaseAdvisor_40_50 advisor) throws FHIRException { 023 cleanInputs(src, advisor); 024 return src != null ? new VersionConvertor_40_50(advisor).convertResource(src) : null; 025 } 026 027 public static org.hl7.fhir.r5.model.DataType convertType(org.hl7.fhir.r4.model.Type src) throws FHIRException { 028 return convertType(src, new BaseAdvisor_40_50()); 029 } 030 031 public static org.hl7.fhir.r5.model.DataType convertType(org.hl7.fhir.r4.model.Type src, BaseAdvisor_40_50 advisor) throws FHIRException { 032 cleanInputs(src, advisor); 033 return src != null ? new VersionConvertor_40_50(advisor).convertType(src) : null; 034 } 035 036 public static org.hl7.fhir.r4.model.Type convertType(org.hl7.fhir.r5.model.DataType src) throws FHIRException { 037 return convertType(src, new BaseAdvisor_40_50()); 038 } 039 040 public static org.hl7.fhir.r4.model.Type convertType(org.hl7.fhir.r5.model.DataType src, BaseAdvisor_40_50 advisor) throws FHIRException { 041 cleanInputs(src, advisor); 042 return src != null ? new VersionConvertor_40_50(advisor).convertType(src) : null; 043 } 044}