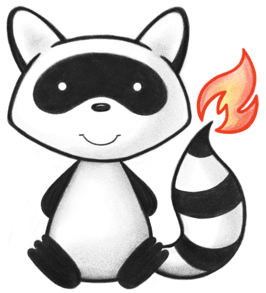
001package org.hl7.fhir.convertors.loaders; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.io.OutputStream; 006 007import org.hl7.fhir.convertors.factory.VersionConvertorFactory_10_50; 008import org.hl7.fhir.convertors.factory.VersionConvertorFactory_14_50; 009import org.hl7.fhir.convertors.factory.VersionConvertorFactory_30_50; 010import org.hl7.fhir.convertors.factory.VersionConvertorFactory_40_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.exceptions.FHIRFormatError; 013import org.hl7.fhir.r5.formats.IParser.OutputStyle; 014import org.hl7.fhir.r5.model.Resource; 015import org.hl7.fhir.utilities.Utilities; 016import org.hl7.fhir.utilities.VersionUtilities; 017 018public class XVersionLoader { 019 020 public static Resource loadXml(String version, InputStream stream) throws FHIRFormatError, IOException { 021 if (Utilities.noString(version)) { 022 return new org.hl7.fhir.r5.formats.XmlParser().parse(stream); 023 } 024 switch (VersionUtilities.getMajMin(version)) { 025 case "1.0": 026 return VersionConvertorFactory_10_50.convertResource(new org.hl7.fhir.dstu2.formats.XmlParser().parse(stream)); 027 case "1.4": 028 return VersionConvertorFactory_14_50.convertResource(new org.hl7.fhir.dstu2016may.formats.XmlParser().parse(stream)); 029 case "3.0": 030 return VersionConvertorFactory_30_50.convertResource(new org.hl7.fhir.dstu3.formats.XmlParser().parse(stream)); 031 case "4.0": 032 return VersionConvertorFactory_40_50.convertResource(new org.hl7.fhir.r4.formats.XmlParser().parse(stream)); 033 case "5.0": 034 return new org.hl7.fhir.r5.formats.XmlParser().parse(stream); 035 } 036 throw new FHIRException("Unknown version " + version + " loading resource"); 037 } 038 039 public static Resource loadJson(String version, InputStream stream) throws FHIRException, IOException { 040 if (Utilities.noString(version)) { 041 return new org.hl7.fhir.r5.formats.JsonParser().parse(stream); 042 } 043 switch (VersionUtilities.getMajMin(version)) { 044 case "1.0": 045 return VersionConvertorFactory_10_50.convertResource(new org.hl7.fhir.dstu2.formats.JsonParser().parse(stream)); 046 case "1.4": 047 return VersionConvertorFactory_14_50.convertResource(new org.hl7.fhir.dstu2016may.formats.JsonParser().parse(stream)); 048 case "3.0": 049 return VersionConvertorFactory_30_50.convertResource(new org.hl7.fhir.dstu3.formats.JsonParser().parse(stream)); 050 case "4.0": 051 return VersionConvertorFactory_40_50.convertResource(new org.hl7.fhir.r4.formats.JsonParser().parse(stream)); 052 case "5.0": 053 return new org.hl7.fhir.r5.formats.JsonParser().parse(stream); 054 } 055 throw new FHIRException("Unknown version " + version + " loading resource"); 056 } 057 058 public static void saveXml(String version, Resource resource, OutputStream stream) throws FHIRFormatError, IOException { 059 if (Utilities.noString(version)) { 060 new org.hl7.fhir.r5.formats.XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(stream, resource); 061 } 062 switch (VersionUtilities.getMajMin(version)) { 063 case "1.0": 064 new org.hl7.fhir.dstu2.formats.XmlParser().compose(stream, VersionConvertorFactory_10_50.convertResource(resource), true); 065 return; 066 case "1.4": 067 new org.hl7.fhir.dstu2016may.formats.XmlParser(true, true).compose(stream, VersionConvertorFactory_14_50.convertResource(resource), true); 068 return; 069 case "3.0": 070 new org.hl7.fhir.dstu3.formats.XmlParser().compose(stream, VersionConvertorFactory_30_50.convertResource(resource), true); 071 return; 072 case "4.0": 073 new org.hl7.fhir.r4.formats.XmlParser().compose(stream, VersionConvertorFactory_40_50.convertResource(resource), true); 074 return; 075 case "5.0": 076 new org.hl7.fhir.r5.formats.XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(stream, resource); 077 return; 078 } 079 throw new FHIRException("Unknown version " + version + " loading resource"); 080 } 081 082 public static void saveJson(String version, Resource resource, OutputStream stream) throws FHIRException, IOException { 083 if (Utilities.noString(version)) { 084 new org.hl7.fhir.r5.formats.JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(stream, resource); 085 } 086 switch (VersionUtilities.getMajMin(version)) { 087 case "1.0": 088 new org.hl7.fhir.dstu2.formats.JsonParser().setOutputStyle(org.hl7.fhir.dstu2.formats.IParser.OutputStyle.PRETTY).compose(stream, VersionConvertorFactory_10_50.convertResource(resource)); 089 return; 090 case "1.4": 091 new org.hl7.fhir.dstu2016may.formats.JsonParser().setOutputStyle(org.hl7.fhir.dstu2016may.formats.IParser.OutputStyle.PRETTY).compose(stream, VersionConvertorFactory_14_50.convertResource(resource)); 092 return; 093 case "3.0": 094 new org.hl7.fhir.dstu3.formats.JsonParser().setOutputStyle(org.hl7.fhir.dstu3.formats.IParser.OutputStyle.PRETTY).compose(stream, VersionConvertorFactory_30_50.convertResource(resource)); 095 return; 096 case "4.0": 097 new org.hl7.fhir.r4.formats.JsonParser().setOutputStyle(org.hl7.fhir.r4.formats.IParser.OutputStyle.PRETTY).compose(stream, VersionConvertorFactory_40_50.convertResource(resource)); 098 return; 099 case "5.0": 100 new org.hl7.fhir.r5.formats.JsonParser().setOutputStyle(org.hl7.fhir.r5.formats.IParser.OutputStyle.PRETTY).compose(stream, resource); 101 return; 102 } 103 throw new FHIRException("Unknown version " + version + " loading resource"); 104 } 105 106}