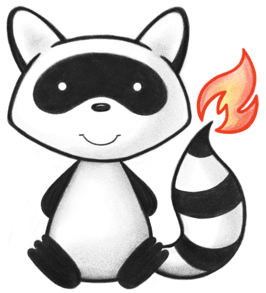
001package org.hl7.fhir.convertors.loaders.loaderR4; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.ArrayList; 006import java.util.List; 007import java.util.UUID; 008 009/* 010 Copyright (c) 2011+, HL7, Inc. 011 All rights reserved. 012 013 Redistribution and use in source and binary forms, with or without modification, 014 are permitted provided that the following conditions are met: 015 016 * Redistributions of source code must retain the above copyright notice, this 017 list of conditions and the following disclaimer. 018 * Redistributions in binary form must reproduce the above copyright notice, 019 this list of conditions and the following disclaimer in the documentation 020 and/or other materials provided with the distribution. 021 * Neither the name of HL7 nor the names of its contributors may be used to 022 endorse or promote products derived from this software without specific 023 prior written permission. 024 025 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 026 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 027 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 028 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 029 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 030 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 031 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 032 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 033 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 034 POSSIBILITY OF SUCH DAMAGE. 035 036 */ 037 038 039import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_40; 040import org.hl7.fhir.convertors.factory.VersionConvertorFactory_10_40; 041import org.hl7.fhir.dstu2.formats.JsonParser; 042import org.hl7.fhir.dstu2.formats.XmlParser; 043import org.hl7.fhir.dstu2.model.Resource; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.r4.model.Bundle; 046import org.hl7.fhir.r4.model.Bundle.BundleEntryComponent; 047import org.hl7.fhir.r4.model.Bundle.BundleType; 048import org.hl7.fhir.r4.model.MetadataResource; 049import org.hl7.fhir.r4.model.StructureDefinition; 050import org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind; 051import org.hl7.fhir.r5.utils.ToolingExtensions; 052import org.hl7.fhir.r4.model.UriType; 053 054public class R2ToR4Loader extends BaseLoaderR4 { 055 056 private final BaseAdvisor_10_40 advisor = new BaseAdvisor_10_40(); 057 058 public R2ToR4Loader() { 059 super(new String[0], new NullLoaderKnowledgeProviderR4()); 060 } 061 062 @Override 063 public Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException { 064 Resource r2 = null; 065 if (isJson) 066 r2 = new JsonParser().parse(stream); 067 else 068 r2 = new XmlParser().parse(stream); 069 org.hl7.fhir.r4.model.Resource r4 = VersionConvertorFactory_10_40.convertResource(r2, advisor); 070 Bundle b; 071 if (r4 instanceof Bundle) { 072 b = (Bundle) r4; 073 } else { 074 b = new Bundle(); 075 b.setId(UUID.randomUUID().toString().toLowerCase()); 076 b.setType(BundleType.COLLECTION); 077 b.addEntry().setResource(r4).setFullUrl(r4 instanceof MetadataResource ? ((MetadataResource) r4).getUrl() : null); 078 } 079 // Add any code systems defined as part of processing value sets to the end of the converted Bundle 080 advisor.getCslist().forEach(cs -> { 081 BundleEntryComponent be = b.addEntry(); 082 be.setFullUrl(cs.getUrl()); 083 be.setResource(cs); 084 }); 085 advisor.getCslist().clear(); 086 087 if (killPrimitives) { 088 List<BundleEntryComponent> remove = new ArrayList<BundleEntryComponent>(); 089 for (BundleEntryComponent be : b.getEntry()) { 090 if (be.hasResource() && be.getResource() instanceof StructureDefinition) { 091 StructureDefinition sd = (StructureDefinition) be.getResource(); 092 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 093 remove.add(be); 094 } 095 } 096 b.getEntry().removeAll(remove); 097 } 098 if (patchUrls) { 099 b.getEntry().stream() 100 .filter(be -> be.hasResource() && be.getResource() instanceof StructureDefinition) 101 .map(be -> (StructureDefinition) be.getResource()) 102 .forEach(sd -> { 103 sd.setUrl(sd.getUrl().replace(URL_BASE, URL_DSTU2)); 104 sd.addExtension().setUrl(ToolingExtensions.EXT_XML_NAMESPACE).setValue(new UriType(URL_BASE)); 105 }); 106 } 107 return b; 108 } 109}