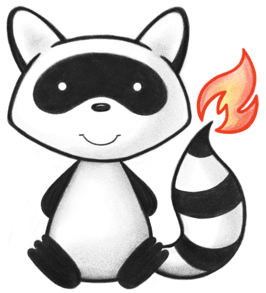
001package org.hl7.fhir.convertors.loaders.loaderR5; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.ArrayList; 006import java.util.List; 007import java.util.UUID; 008 009/* 010 Copyright (c) 2011+, HL7, Inc. 011 All rights reserved. 012 013 Redistribution and use in source and binary forms, with or without modification, 014 are permitted provided that the following conditions are met: 015 016 * Redistributions of source code must retain the above copyright notice, this 017 list of conditions and the following disclaimer. 018 * Redistributions in binary form must reproduce the above copyright notice, 019 this list of conditions and the following disclaimer in the documentation 020 and/or other materials provided with the distribution. 021 * Neither the name of HL7 nor the names of its contributors may be used to 022 endorse or promote products derived from this software without specific 023 prior written permission. 024 025 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 026 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 027 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 028 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 029 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 030 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 031 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 032 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 033 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 034 POSSIBILITY OF SUCH DAMAGE. 035 036 */ 037 038 039import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_14_50; 040import org.hl7.fhir.convertors.factory.VersionConvertorFactory_14_50; 041import org.hl7.fhir.convertors.txClient.TerminologyClientFactory; 042import org.hl7.fhir.dstu2016may.formats.JsonParser; 043import org.hl7.fhir.dstu2016may.formats.XmlParser; 044import org.hl7.fhir.dstu2016may.model.Resource; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.r5.model.Bundle; 047import org.hl7.fhir.r5.model.Bundle.BundleEntryComponent; 048import org.hl7.fhir.r5.model.Bundle.BundleType; 049import org.hl7.fhir.r5.model.CanonicalResource; 050import org.hl7.fhir.r5.model.CodeSystem; 051import org.hl7.fhir.r5.model.StructureDefinition; 052import org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind; 053import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 054 055public class R2016MayToR5Loader extends BaseLoaderR5 { 056 057 private final BaseAdvisor_14_50 advisor = new BaseAdvisor_14_50(); 058 059 public R2016MayToR5Loader(List<String> types, ILoaderKnowledgeProviderR5 lkp) { 060 super(types, lkp); 061 } 062 063 @Override 064 public Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException { 065 Resource r2016may = null; 066 if (isJson) 067 r2016may = new JsonParser().parse(stream); 068 else 069 r2016may = new XmlParser().parse(stream); 070 org.hl7.fhir.r5.model.Resource r5 = VersionConvertorFactory_14_50.convertResource(r2016may, advisor); 071 072 Bundle b; 073 if (r5 instanceof Bundle) 074 b = (Bundle) r5; 075 else { 076 b = new Bundle(); 077 b.setId(UUID.randomUUID().toString().toLowerCase()); 078 b.setType(BundleType.COLLECTION); 079 b.addEntry().setResource(r5).setFullUrl(r5 instanceof CanonicalResource ? ((CanonicalResource) r5).getUrl() : null); 080 } 081 082 for (CodeSystem cs : advisor.getCslist()) { 083 BundleEntryComponent be = b.addEntry(); 084 be.setFullUrl(cs.getUrl()); 085 be.setResource(cs); 086 } 087 if (killPrimitives) { 088 List<BundleEntryComponent> remove = new ArrayList<BundleEntryComponent>(); 089 for (BundleEntryComponent be : b.getEntry()) { 090 if (be.hasResource() && be.getResource() instanceof StructureDefinition) { 091 StructureDefinition sd = (StructureDefinition) be.getResource(); 092 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 093 remove.add(be); 094 } 095 } 096 b.getEntry().removeAll(remove); 097 } 098 if (patchUrls) { 099 for (BundleEntryComponent be : b.getEntry()) { 100 if (be.hasResource()) { 101 doPatchUrls(be.getResource()); 102 } 103 } 104 } 105 return b; 106 } 107 108 @Override 109 public org.hl7.fhir.r5.model.Resource loadResource(InputStream stream, boolean isJson) throws FHIRException, IOException { 110 Resource r2016may = null; 111 if (isJson) 112 r2016may = new JsonParser().parse(stream); 113 else 114 r2016may = new XmlParser().parse(stream); 115 org.hl7.fhir.r5.model.Resource r5 = VersionConvertorFactory_14_50.convertResource(r2016may); 116 setPath(r5); 117 118 if (!advisor.getCslist().isEmpty()) { 119 throw new FHIRException("Error: Cannot have included code systems"); 120 } 121 if (killPrimitives) { 122 throw new FHIRException("Cannot kill primitives when using deferred loading"); 123 } 124 if (patchUrls) { 125 doPatchUrls(r5); 126 } 127 return r5; 128 } 129 130 131 @Override 132 public List<CodeSystem> getCodeSystems() { 133 return new ArrayList<>(); 134 } 135 136 @Override 137 protected String versionString() { 138 return "4.3"; 139 } 140 141 @Override 142 public ITerminologyClientFactory txFactory() { 143 return new TerminologyClientFactory(versionString()); 144 } 145 146}