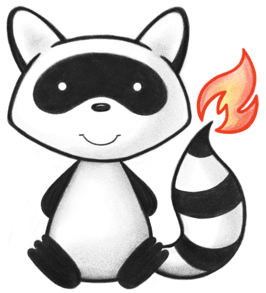
001package org.hl7.fhir.convertors.loaders.loaderR5; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.ArrayList; 006import java.util.List; 007import java.util.UUID; 008 009/* 010 Copyright (c) 2011+, HL7, Inc. 011 All rights reserved. 012 013 Redistribution and use in source and binary forms, with or without modification, 014 are permitted provided that the following conditions are met: 015 016 * Redistributions of source code must retain the above copyright notice, this 017 list of conditions and the following disclaimer. 018 * Redistributions in binary form must reproduce the above copyright notice, 019 this list of conditions and the following disclaimer in the documentation 020 and/or other materials provided with the distribution. 021 * Neither the name of HL7 nor the names of its contributors may be used to 022 endorse or promote products derived from this software without specific 023 prior written permission. 024 025 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 026 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 027 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 028 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 029 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 030 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 031 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 032 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 033 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 034 POSSIBILITY OF SUCH DAMAGE. 035 036 */ 037 038 039import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_40_50; 040import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_43_50; 041import org.hl7.fhir.convertors.factory.VersionConvertorFactory_40_50; 042import org.hl7.fhir.convertors.factory.VersionConvertorFactory_43_50; 043import org.hl7.fhir.convertors.txClient.TerminologyClientFactory; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.r4b.formats.JsonParser; 046import org.hl7.fhir.r4b.formats.XmlParser; 047import org.hl7.fhir.r4b.model.Resource; 048import org.hl7.fhir.r5.conformance.StructureDefinitionHacker; 049import org.hl7.fhir.r5.context.IContextResourceLoader; 050import org.hl7.fhir.r5.model.Bundle; 051import org.hl7.fhir.r5.model.Bundle.BundleEntryComponent; 052import org.hl7.fhir.r5.model.Bundle.BundleType; 053import org.hl7.fhir.r5.model.CanonicalResource; 054import org.hl7.fhir.r5.model.CodeSystem; 055import org.hl7.fhir.r5.model.StructureDefinition; 056import org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind; 057import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 058import org.hl7.fhir.utilities.VersionUtilities; 059 060public class R4BToR5Loader extends BaseLoaderR5 implements IContextResourceLoader { 061 062 private final BaseAdvisor_43_50 advisor = new BaseAdvisor_43_50(); 063 private String version; 064 065 public R4BToR5Loader(List<String> types, ILoaderKnowledgeProviderR5 lkp, String version) { // might be 4B 066 super(types, lkp); 067 this.version = version; 068 } 069 070 @Override 071 public Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException { 072 Resource r4 = null; 073 if (isJson) 074 r4 = new JsonParser().parse(stream); 075 else 076 r4 = new XmlParser().parse(stream); 077 org.hl7.fhir.r5.model.Resource r5 = VersionConvertorFactory_43_50.convertResource(r4, advisor); 078 079 Bundle b; 080 if (r5 instanceof Bundle) 081 b = (Bundle) r5; 082 else { 083 b = new Bundle(); 084 b.setId(UUID.randomUUID().toString().toLowerCase()); 085 b.setType(BundleType.COLLECTION); 086 b.addEntry().setResource(r5).setFullUrl(r5 instanceof CanonicalResource ? ((CanonicalResource) r5).getUrl() : null); 087 } 088 for (CodeSystem cs : advisor.getCslist()) { 089 BundleEntryComponent be = b.addEntry(); 090 be.setFullUrl(cs.getUrl()); 091 be.setResource(cs); 092 } 093 if (killPrimitives) { 094 List<BundleEntryComponent> remove = new ArrayList<BundleEntryComponent>(); 095 for (BundleEntryComponent be : b.getEntry()) { 096 if (be.hasResource() && be.getResource() instanceof StructureDefinition) { 097 StructureDefinition sd = (StructureDefinition) be.getResource(); 098 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 099 remove.add(be); 100 } 101 } 102 b.getEntry().removeAll(remove); 103 } 104 if (patchUrls) { 105 for (BundleEntryComponent be : b.getEntry()) { 106 if (be.hasResource()) { 107 doPatchUrls(be.getResource()); 108 } 109 } 110 } 111 return b; 112 } 113 114 @Override 115 public org.hl7.fhir.r5.model.Resource loadResource(InputStream stream, boolean isJson) throws FHIRException, IOException { 116 Resource r4 = null; 117 if (isJson) 118 r4 = new JsonParser().parse(stream); 119 else 120 r4 = new XmlParser().parse(stream); 121 org.hl7.fhir.r5.model.Resource r5 = VersionConvertorFactory_43_50.convertResource(r4); 122 setPath(r5); 123 124 if (!advisor.getCslist().isEmpty()) { 125 throw new FHIRException("Error: Cannot have included code systems"); 126 } 127 if (killPrimitives) { 128 throw new FHIRException("Cannot kill primitives when using deferred loading"); 129 } 130 if (r5 instanceof StructureDefinition) { 131 r5 = new StructureDefinitionHacker(version).fixSD((StructureDefinition) r5); 132 } 133 if (patchUrls) { 134 doPatchUrls(r5); 135 } 136 return r5; 137 } 138 139 @Override 140 public List<CodeSystem> getCodeSystems() { 141 return new ArrayList<>(); 142 } 143 144 @Override 145 protected String versionString() { 146 return "4.3"; 147 } 148 149 150 @Override 151 public ITerminologyClientFactory txFactory() { 152 return new TerminologyClientFactory(versionString()); 153 } 154 155}