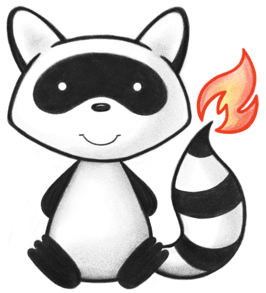
001package org.hl7.fhir.convertors.loaders.loaderR5; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.ArrayList; 006import java.util.List; 007import java.util.UUID; 008 009import org.hl7.fhir.convertors.txClient.TerminologyClientFactory; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038 */ 039 040 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.r5.formats.JsonParser; 043import org.hl7.fhir.r5.formats.XmlParser; 044import org.hl7.fhir.r5.model.Bundle; 045import org.hl7.fhir.r5.model.Bundle.BundleEntryComponent; 046import org.hl7.fhir.r5.model.Bundle.BundleType; 047import org.hl7.fhir.r5.model.CanonicalResource; 048import org.hl7.fhir.r5.model.CodeSystem; 049import org.hl7.fhir.r5.model.Resource; 050import org.hl7.fhir.r5.model.StructureDefinition; 051import org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind; 052import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 053 054public class R5ToR5Loader extends BaseLoaderR5 { 055 056 public R5ToR5Loader(List<String> types, ILoaderKnowledgeProviderR5 lkp) { 057 super(types, lkp); 058 } 059 060 @Override 061 public Bundle loadBundle(InputStream stream, boolean isJson) throws FHIRException, IOException { 062 Resource r5 = null; 063 if (isJson) 064 r5 = new JsonParser().parse(stream); 065 else 066 r5 = new XmlParser().parse(stream); 067 068 Bundle b; 069 if (r5 instanceof Bundle) 070 b = (Bundle) r5; 071 else { 072 b = new Bundle(); 073 b.setId(UUID.randomUUID().toString().toLowerCase()); 074 b.setType(BundleType.COLLECTION); 075 b.addEntry().setResource(r5).setFullUrl(r5 instanceof CanonicalResource ? ((CanonicalResource) r5).getUrl() : null); 076 } 077 if (killPrimitives) { 078 List<BundleEntryComponent> remove = new ArrayList<BundleEntryComponent>(); 079 for (BundleEntryComponent be : b.getEntry()) { 080 if (be.hasResource() && be.getResource() instanceof StructureDefinition) { 081 StructureDefinition sd = (StructureDefinition) be.getResource(); 082 if (sd.getKind() == StructureDefinitionKind.PRIMITIVETYPE) 083 remove.add(be); 084 } 085 } 086 b.getEntry().removeAll(remove); 087 } 088 if (patchUrls) { 089 for (BundleEntryComponent be : b.getEntry()) { 090 if (be.hasResource()) { 091 doPatchUrls(be.getResource()); 092 } 093 } 094 } 095 return b; 096 } 097 098 @Override 099 public Resource loadResource(InputStream stream, boolean isJson) throws FHIRException, IOException { 100 Resource r5 = null; 101 if (isJson) 102 r5 = new JsonParser().parse(stream); 103 else 104 r5 = new XmlParser().parse(stream); 105 setPath(r5); 106 107 if (killPrimitives) { 108 throw new FHIRException("Cannot kill primitives when using deferred loading"); 109 } 110 if (patchUrls) { 111 doPatchUrls(r5); 112 } 113 if (r5 instanceof StructureDefinition) { 114 StructureDefinition sd = (StructureDefinition) r5; 115 if ("http://hl7.org/fhir/StructureDefinition/Base".equals(sd.getUrl())) { 116 sd.getSnapshot().getElementFirstRep().getConstraint().clear(); 117 118 } 119 } 120 return r5; 121 } 122 123 @Override 124 public List<CodeSystem> getCodeSystems() { 125 return new ArrayList<>(); 126 } 127 128 @Override 129 protected String versionString() { 130 return "5.0"; 131 } 132 133 134 @Override 135 public ITerminologyClientFactory txFactory() { 136 return new TerminologyClientFactory(versionString()); 137 } 138 139}