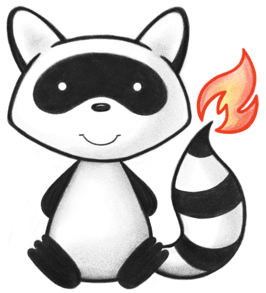
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileNotFoundException; 006import java.io.IOException; 007import java.io.InputStream; 008import java.io.InputStreamReader; 009 010import org.hl7.fhir.utilities.FileUtilities; 011import org.hl7.fhir.utilities.Utilities; 012import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 013 014public class BOMRemover { 015 016 public static void main(String[] args) throws FileNotFoundException, IOException { 017 new BOMRemover().execute(ManagedFileAccess.file(args[0])); 018 019 } 020 021 private void execute(File f) throws FileNotFoundException, IOException { 022 if (f.isDirectory()) { 023 for (File file : f.listFiles()) { 024 execute(file); 025 } 026 } else if (f.getName().endsWith(".java")) { 027 String src = fileToString(f); 028 String s = Utilities.stripBOM(src); 029 if (!s.equals(src)) { 030 System.out.println("Remove BOM from "+f.getAbsolutePath()); 031 FileUtilities.stringToFile(s, f); 032 } 033 } 034 } 035 036 037 public static String fileToString(File f) throws FileNotFoundException, IOException { 038 return streamToString(new FileInputStream(f)); 039 } 040 041 public static String streamToString(InputStream input) throws IOException { 042 InputStreamReader sr = new InputStreamReader(input, "UTF-8"); 043 StringBuilder b = new StringBuilder(); 044 //while (sr.ready()) { Commented out by Claude Nanjo (1/14/2014) - sr.ready() always returns false - please remove if change does not impact other areas of codebase 045 int i = -1; 046 while((i = sr.read()) > -1) { 047 char c = (char) i; 048 b.append(c); 049 } 050 sr.close(); 051 052 return b.toString(); 053 } 054 055}