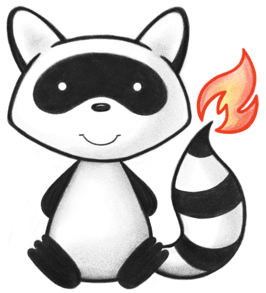
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.io.IOException; 007 008import org.hl7.fhir.exceptions.FHIRFormatError; 009import org.hl7.fhir.utilities.FileUtilities; 010import org.hl7.fhir.utilities.Utilities; 011import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 012import org.hl7.fhir.utilities.json.model.JsonObject; 013import org.hl7.fhir.utilities.json.parser.JsonParser; 014import org.hl7.fhir.utilities.npm.NpmPackage; 015 016@SuppressWarnings("checkstyle:systemout") 017public class CorePackageTools { 018 019 public static void main(String[] args) throws FHIRFormatError, IOException { 020 if ("-xml".equals(args[0])) { 021 new CorePackageTools().buildXml(args[1], args[2], args[3]); 022 } 023 if ("-pack".equals(args[0])) { 024 new CorePackageTools().buildPackage(args[1], args[2]); 025 } 026 } 027 028 private void buildPackage(String path, String output) throws IOException { 029 NpmPackage npm = NpmPackage.fromFolder(path); 030 npm.loadAllFiles(); 031 npm.save(ManagedFileAccess.outStream(output)); 032 033 } 034 035 private void buildXml(String json, String xml, String version) throws FHIRFormatError, IOException { 036 for (File f : ManagedFileAccess.file(Utilities.path(json, "package")).listFiles()) { 037 if (f.getName().endsWith(".json")) { 038 JsonObject j = JsonParser.parseObject(f); 039 if (j.has("resourceType")) { 040 if ("1.4".equals(version)) { 041 String n = f.getName(); 042 System.out.println(n); 043 String xn = FileUtilities.changeFileExt(n, ".xml"); 044 org.hl7.fhir.dstu2016may.model.Resource r = new org.hl7.fhir.dstu2016may.formats.JsonParser().parse(ManagedFileAccess.inStream(f)); 045 new org.hl7.fhir.dstu2016may.formats.XmlParser().setOutputStyle(org.hl7.fhir.dstu2016may.formats.IParser.OutputStyle.NORMAL).compose(ManagedFileAccess.outStream(Utilities.path(xml, "package", xn)), r); 046 } 047 } 048 } 049 } 050 } 051}