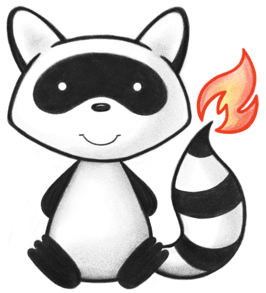
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileNotFoundException; 006import java.io.IOException; 007import java.util.HashSet; 008import java.util.Set; 009 010import org.hl7.fhir.convertors.factory.VersionConvertorFactory_40_50; 011import org.hl7.fhir.exceptions.FHIRFormatError; 012import org.hl7.fhir.utilities.FileUtilities; 013import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 014import org.hl7.fhir.utilities.json.model.JsonObject; 015import org.hl7.fhir.utilities.json.parser.JsonParser; 016 017@SuppressWarnings("checkstyle:systemout") 018public class ExamplesPackageBuilder { 019 020 public static void main(String[] args) throws FHIRFormatError, FileNotFoundException, IOException { 021 new ExamplesPackageBuilder().process(args[0]); 022 023 } 024 025 private void process(String source) throws FHIRFormatError, FileNotFoundException, IOException { 026 Set<String> set = new HashSet<>(); 027 for (File f : ManagedFileAccess.file(source).listFiles()) { 028 if (f.getName().endsWith(".json")) { 029 JsonObject obj = JsonParser.parseObject(ManagedFileAccess.inStream(f)); 030 if (obj.has("resourceType") && obj.has("id")) { 031 String type = obj.asString("resourceType"); 032 String id = obj.asString("id"); 033 byte[] content = FileUtilities.fileToBytes(f); 034 if (type.equals("ConceptMap")) { 035 System.out.println("convert "+f.getName()); 036 content = r5ToR4B(content); 037 FileUtilities.bytesToFile(content, f); 038 } 039// FileUtilities.bytesToFile(content, Utilities.path(dest2, type+"-"+id+".json")); 040// if (!set.contains(type+"/"+id)) { 041// set.add(type+"/"+id); 042// pck.addFile(Category.RESOURCE, type+"-"+id+".json", content); 043// } 044 } 045 } 046 } 047// pck.finish(); 048// 049 } 050 051 private byte[] r5ToR4B(byte[] content) throws FHIRFormatError, IOException { 052 try { 053 org.hl7.fhir.r5.model.Resource r5 = new org.hl7.fhir.r5.formats.JsonParser().parse(content); 054 org.hl7.fhir.r4.model.Resource r4 = VersionConvertorFactory_40_50.convertResource(r5); 055 return new org.hl7.fhir.r4.formats.JsonParser().composeBytes(r4); 056 } catch (Exception e) { 057 System.out.println(" .. failed: "+e.getMessage()); 058 return content; 059 } 060 } 061 062}