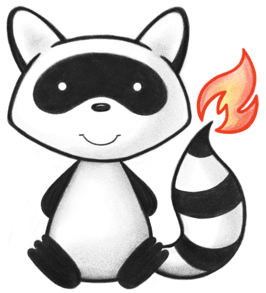
001package org.hl7.fhir.convertors.misc; 002 003import java.io.FileInputStream; 004import java.io.FileOutputStream; 005import java.io.IOException; 006 007/* 008 Copyright (c) 2011+, HL7, Inc. 009 All rights reserved. 010 011 Redistribution and use in source and binary forms, with or without modification, 012 are permitted provided that the following conditions are met: 013 014 * Redistributions of source code must retain the above copyright notice, this 015 list of conditions and the following disclaimer. 016 * Redistributions in binary form must reproduce the above copyright notice, 017 this list of conditions and the following disclaimer in the documentation 018 and/or other materials provided with the distribution. 019 * Neither the name of HL7 nor the names of its contributors may be used to 020 endorse or promote products derived from this software without specific 021 prior written permission. 022 023 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 024 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 025 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 026 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 027 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 028 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 030 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 031 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 032 POSSIBILITY OF SUCH DAMAGE. 033 034 */ 035 036 037import org.hl7.fhir.dstu2.formats.IParser.OutputStyle; 038import org.hl7.fhir.dstu2.formats.JsonParser; 039import org.hl7.fhir.dstu2.formats.XmlParser; 040import org.hl7.fhir.dstu2.model.Bundle; 041import org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent; 042import org.hl7.fhir.dstu2.model.Resource; 043import org.hl7.fhir.dstu2.model.ValueSet; 044import org.hl7.fhir.exceptions.FHIRFormatError; 045import org.hl7.fhir.utilities.Utilities; 046import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 047 048public class GenValueSetExpansionConvertor { 049 050 public static void main(String[] args) throws FHIRFormatError, IOException { 051 String src = args[0]; 052 String tgt = args[1]; 053 Bundle bundle = (Bundle) new XmlParser().parse(ManagedFileAccess.inStream(src)); 054 for (BundleEntryComponent be : bundle.getEntry()) { 055 Resource res = be.getResource(); 056 if (res != null) { 057 String id = res.getId(); 058 if (Utilities.noString(id)) 059 id = tail(((ValueSet) res).getUrl()); 060 String dst = Utilities.path(tgt, res.fhirType() + "-" + id + ".json"); 061 System.out.println(dst); 062 new JsonParser().setOutputStyle(OutputStyle.NORMAL).compose(ManagedFileAccess.outStream(dst), res); 063 } 064 } 065 } 066 067 private static String tail(String url) { 068 return url.substring(url.lastIndexOf("/") + 1); 069 } 070 071}