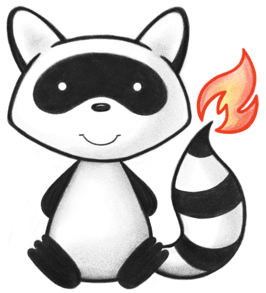
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.io.IOException; 007import java.util.Date; 008 009import javax.annotation.Nonnull; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038 */ 039 040 041import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_30; 042import org.hl7.fhir.convertors.factory.VersionConvertorFactory_10_30; 043import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 044import org.hl7.fhir.dstu3.model.Bundle; 045import org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent; 046import org.hl7.fhir.dstu3.model.Bundle.BundleType; 047import org.hl7.fhir.dstu3.model.CodeSystem; 048import org.hl7.fhir.dstu3.model.ValueSet; 049import org.hl7.fhir.exceptions.FHIRException; 050import org.hl7.fhir.utilities.FhirPublication; 051import org.hl7.fhir.utilities.Utilities; 052import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 053 054public class IGPackConverter102 extends BaseAdvisor_10_30 { 055 056 private final Bundle cslist = new Bundle(); 057 058 public static void main(String[] args) throws Exception { 059 new IGPackConverter102().process(); 060 } 061 062 private void process() throws IOException, FHIRException { 063 initCSList(); 064 for (String s : ManagedFileAccess.file(Utilities.path("[tmp]", "igpack2")).list()) { 065 if (s.endsWith(".xml") && !s.startsWith("z-") && !Utilities.existsInList(s, "expansions.xml", "v3-codesystems.xml", "v2-tables.xml")) { 066 System.out.println("process " + s); 067 org.hl7.fhir.dstu2.formats.XmlParser xp = new org.hl7.fhir.dstu2.formats.XmlParser(); 068 org.hl7.fhir.dstu2.model.Resource r10 = xp.parse(ManagedFileAccess.inStream(Utilities.path("[tmp]", "igpack2\\" + s))); 069 org.hl7.fhir.dstu3.model.Resource r17 = VersionConvertorFactory_10_30.convertResource(r10, this); 070 org.hl7.fhir.dstu3.formats.XmlParser xc = new org.hl7.fhir.dstu3.formats.XmlParser(); 071 xc.setOutputStyle(OutputStyle.PRETTY); 072 xc.compose(ManagedFileAccess.outStream(Utilities.path("[tmp]", "igpack2\\" + s)), r17); 073 } 074 } 075 System.out.println("save codesystems"); 076 org.hl7.fhir.dstu3.formats.XmlParser xc = new org.hl7.fhir.dstu3.formats.XmlParser(); 077 xc.setOutputStyle(OutputStyle.PRETTY); 078 xc.compose(ManagedFileAccess.outStream(Utilities.path("[tmp]", "igpack2\\codesystems.xml")), cslist); 079 System.out.println("done"); 080 } 081 082 private void initCSList() { 083 cslist.setId("codesystems"); 084 cslist.setType(BundleType.COLLECTION); 085 cslist.getMeta().setLastUpdated(new Date()); 086 } 087 088 @Override 089 public boolean ignoreEntry(@Nonnull BundleEntryComponent src, @Nonnull FhirPublication publication) { 090 return false; 091 } 092 093 094 @Override 095 public void handleCodeSystem(@Nonnull CodeSystem tgtcs, @Nonnull ValueSet vs) { 096 cslist.addEntry().setFullUrl(tgtcs.getUrl()).setResource(tgtcs); 097 } 098 099 @Override 100 public CodeSystem getCodeSystem(@Nonnull ValueSet src) { 101 return null; 102 } 103 104}