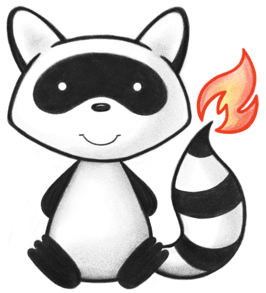
001package org.hl7.fhir.convertors.misc; 002 003import java.io.IOException; 004 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.context.IWorkerContext; 007import org.hl7.fhir.r5.context.SimpleWorkerContext; 008import org.hl7.fhir.r5.model.ValueSet; 009import org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent; 010import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager; 011import org.hl7.fhir.utilities.npm.NpmPackage; 012 013public class OIDBasedValueSetImporter { 014 015 protected IWorkerContext context; 016 017 protected void init() throws FHIRException, IOException { 018 FilesystemPackageCacheManager pcm = new FilesystemPackageCacheManager.Builder().build(); 019 NpmPackage npm = pcm.loadPackage("hl7.fhir.r5.core", "5.0.0"); 020 SimpleWorkerContext context = new SimpleWorkerContext.SimpleWorkerContextBuilder().withAllowLoadingDuplicates(true).fromPackage(npm); 021 context.loadFromPackage(pcm.loadPackage("hl7.terminology"), null); 022 this.context = context; 023 } 024 025 protected String fixVersionforSystem(String url, String csver) { 026 if ("http://snomed.info/sct".equals(url)) { 027 return "http://snomed.info/sct/731000124108/version/" + csver; 028 } 029 if ("http://loinc.org".equals(url)) { 030 return csver; 031 } 032 if ("http://www.nlm.nih.gov/research/umls/rxnorm".equals(url)) { 033 if (csver.length() == 8) { 034 return csver.substring(4, 6) + csver.substring(6, 8) + csver.substring(0, 4); 035 } else { 036 return csver; 037 } 038 039 } 040 return csver; 041 } 042 043 protected ConceptSetComponent getInclude(ValueSet vs, String url, String csver) { 044 for (ConceptSetComponent t : vs.getCompose().getInclude()) { 045 if (csver == null) { 046 if (t.getSystem().equals(url) && !t.hasVersion()) { 047 return t; 048 } 049 } else { 050 if (t.getSystem().equals(url) && t.hasVersion() && t.getVersion().equals(csver)) { 051 return t; 052 } 053 } 054 } 055 ConceptSetComponent c = vs.getCompose().addInclude(); 056 c.setSystem(url); 057 c.setVersion(csver); 058 return c; 059 } 060}