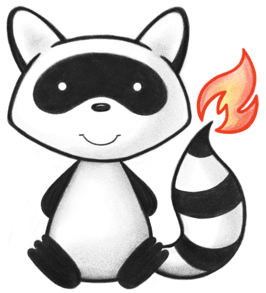
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.IOException; 005import java.util.ArrayList; 006import java.util.List; 007 008import org.hl7.fhir.utilities.FileUtilities; 009import org.hl7.fhir.utilities.Utilities; 010import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 011import org.hl7.fhir.utilities.json.model.JsonObject; 012import org.hl7.fhir.utilities.json.parser.JsonParser; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041 */ 042 043public class PackageMaintainer { 044 045 046 private static final String PATH = "C:\\work\\org.hl7.fhir\\packages\\core"; 047 048 public static void main(String[] args) throws IOException { 049 new PackageMaintainer().check("r4"); 050 new PackageMaintainer().check("r2"); 051 new PackageMaintainer().check("r3"); 052 new PackageMaintainer().check("r2b"); 053 } 054 055 private void check(String ver) throws IOException { 056 System.out.println("Check " + ver); 057 List<String> allIds = listResources(Utilities.path(PATH, "hl7.fhir." + ver + ".examples", "package")); 058 List<String> coreIds = listResources(Utilities.path(PATH, "hl7.fhir." + ver + ".core", "package")); 059 for (String s : coreIds) { 060 if (!allIds.contains(s)) { 061 System.out.println("Core contains " + s + " but allIds doesn't"); 062 } 063 } 064 for (String s : allIds) { 065 if (!coreIds.contains(s)) { 066 String c = s.substring(0, s.indexOf("-")); 067 if (Utilities.existsInList(c, "CodeSystem", "ValueSet", "ConceptMap", "StructureDefinition", "StructureMap", "NamingSystem", "SearchParameter", "OperationDefinition", "CapabilityStatement", "Conformance")) 068 System.out.println("Examples contains " + s + " but core doesn't"); 069 } 070 } 071 strip(ManagedFileAccess.file(Utilities.path(PATH, "hl7.fhir." + ver + ".core", "package"))); 072 strip(ManagedFileAccess.file(Utilities.path(PATH, "hl7.fhir." + ver + ".expansions", "package"))); 073 if (!ver.equals("r2b")) 074 strip(ManagedFileAccess.file(Utilities.path(PATH, "hl7.fhir." + ver + ".elements", "package"))); 075 } 076 077 078 private List<String> listResources(String dir) throws IOException { 079 File folder = ManagedFileAccess.file(dir); 080 List<String> res = new ArrayList<>(); 081 for (String fn : folder.list()) { 082 if (fn.endsWith(".json") && fn.contains("-")) { 083 String s = fn; 084 s = s.substring(0, s.indexOf(".")); 085 res.add(s); 086 } 087 } 088 return res; 089 } 090 091 private void strip(File folder) throws IOException { 092 for (File f : folder.listFiles()) { 093 if (f.isDirectory()) 094 strip(f); 095 else if (f.getName().endsWith(".json")) { 096 JsonObject json = JsonParser.parseObject(f); 097 if (json.has("resourceType") && json.has("text")) { 098 json.remove("text"); 099 String src = JsonParser.compose(json); 100 FileUtilities.stringToFile(src, f.getAbsolutePath()); 101 } 102 } 103 } 104 } 105 106}