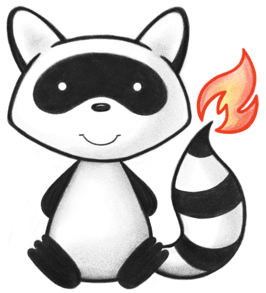
001package org.hl7.fhir.convertors.misc; 002 003import java.io.FileNotFoundException; 004import java.io.FileOutputStream; 005import java.io.IOException; 006 007import org.hl7.fhir.r5.formats.JsonParser; 008import org.hl7.fhir.r5.model.Resource; 009import org.hl7.fhir.r5.utils.ResourceMinifier; 010import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 011import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager; 012import org.hl7.fhir.utilities.npm.NpmPackage; 013import org.hl7.fhir.utilities.npm.NpmPackage.PackageResourceInformation; 014 015public class PackageMinifier { 016 017 public static void main(String[] args) throws IOException { 018 new PackageMinifier().process(args[0], args[1]); 019 } 020 021 private void process(String source, String target) throws FileNotFoundException, IOException { 022 FilesystemPackageCacheManager pcm = new FilesystemPackageCacheManager.Builder().build(); 023 NpmPackage src = pcm.loadPackage(source); 024 NpmPackage tgt = NpmPackage.empty(); 025 tgt.setNpm(src.getNpm().deepCopy()); 026 tgt.getNpm().set("name", tgt.getNpm().asString("name")+".min"); 027 028 ResourceMinifier min = new ResourceMinifier(); 029 for (PackageResourceInformation pri : src.listIndexedResources()) { 030 if (min.isMinified(pri.getResourceType())) { 031 Resource res = new JsonParser().parse(src.load(pri)); 032 if (min.minify(res)) { 033 tgt.addFile("package", res.fhirType()+"-"+res.getIdPart()+".json", new JsonParser().composeBytes(res), null); 034 } 035 } 036 } 037 tgt.save(ManagedFileAccess.outStream(target)); 038 } 039 040}