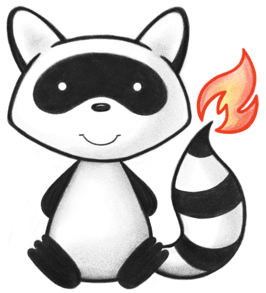
001package org.hl7.fhir.convertors.misc; 002 003import java.io.ByteArrayInputStream; 004import java.io.File; 005import java.io.FileOutputStream; 006import java.io.IOException; 007import java.text.ParseException; 008import java.text.SimpleDateFormat; 009import java.util.List; 010 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.context.ContextUtilities; 013import org.hl7.fhir.r5.formats.IParser.OutputStyle; 014import org.hl7.fhir.r5.formats.JsonParser; 015import org.hl7.fhir.r5.model.Enumerations.PublicationStatus; 016import org.hl7.fhir.r5.model.ValueSet; 017import org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent; 018import org.hl7.fhir.utilities.CSVReader; 019import org.hl7.fhir.utilities.FileUtilities; 020import org.hl7.fhir.utilities.Utilities; 021import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 022 023@SuppressWarnings("checkstyle:systemout") 024public class PhinVadsImporter extends OIDBasedValueSetImporter { 025 026 public PhinVadsImporter() throws FHIRException, IOException { 027 super(); 028 init(); 029 } 030 031 public static void main(String[] args) throws FHIRException, IOException, ParseException { 032// new PhinVadsImporter().importValueSet(FileUtilities.fileToBytes("C:\\work\\org.hl7.fhir\\packages\\us.cdc.phinvads-source\\source\\PHVS_BirthDefectsLateralityatDiagnosis_HL7_V1.txt")); 033 PhinVadsImporter self = new PhinVadsImporter(); 034 self.process(args[0], args[1]); 035 } 036 037 private void process(String source, String dest) throws IOException { 038 for (File f : ManagedFileAccess.file(source).listFiles()) { 039 try { 040 System.out.println("Process " + f.getName()); 041 ValueSet vs = importValueSet(FileUtilities.fileToBytes(f)); 042 if (vs.getId() != null) { 043 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(Utilities.path(dest, "ValueSet-" + vs.getId() + ".json")), vs); 044 } 045 } catch (Exception e) { 046 e.printStackTrace(); 047 } 048 } 049 } 050 051 private ValueSet importValueSet(byte[] source) throws FHIRException, IOException, ParseException { 052 // first thing do is split into 2 053 List<byte[]> parts = Utilities.splitBytes(source, "\r\n\r\n".getBytes()); 054 if (parts.size() < 2) { 055 FileUtilities.bytesToFile(source, Utilities.path("[tmp]", "phinvads.txt")); 056 throw new FHIRException("Unable to parse phinvads value set: " + parts.size() + " parts found"); 057 } 058 CSVReader rdr = new CSVReader(new ByteArrayInputStream(parts.get(0))); 059 rdr.setDelimiter('\t'); 060 rdr.setMultiline(true); 061 rdr.readHeaders(); 062 rdr.line(); 063 064 ValueSet vs = new ValueSet(); 065 vs.setId(rdr.cell("Value Set OID")); 066 vs.setUrl("http://phinvads.cdc.gov/fhir/ValueSet/" + vs.getId()); 067 vs.getMeta().setSource("https://phinvads.cdc.gov/vads/ViewValueSet.action?oid=" + vs.getId()); 068 vs.setVersion(rdr.cell("Value Set Version")); 069 vs.setTitle(rdr.cell("Value Set Name")); 070 vs.setName(rdr.cell("Value Set Code")); 071 vs.setDescription(rdr.cell("Value Set Definition")); 072 if ("Published".equals(rdr.cell("Value Set Status"))) { 073 vs.setStatus(PublicationStatus.ACTIVE); 074 } else { 075 vs.setStatus(PublicationStatus.DRAFT); 076 } 077 if (rdr.has("VS Last Updated Date")) { 078 vs.setDate(new SimpleDateFormat("mm/dd/yyyy").parse(rdr.cell("VS Last Updated Date"))); 079 } 080 081 rdr = new CSVReader(new ByteArrayInputStream(parts.get(parts.size() - 1))); 082 rdr.setMultiline(true); 083 rdr.setDelimiter('\t'); 084 rdr.readHeaders(); 085 while (rdr.line()) { 086 String code = rdr.cell("Concept Code"); 087 String display = rdr.cell("Preferred Concept Name"); 088 String csoid = rdr.cell("Code System OID"); 089 String csver = rdr.cell("Code System Version"); 090 String url = new ContextUtilities(context).oid2Uri(csoid); 091 if (url == null) { 092 url = "urn:oid:" + csoid; 093 } 094 csver = fixVersionforSystem(url, csver); 095 ConceptSetComponent inc = getInclude(vs, url, csver); 096 inc.addConcept().setCode(code).setDisplay(display); 097 } 098 return vs; 099 } 100 101 102}