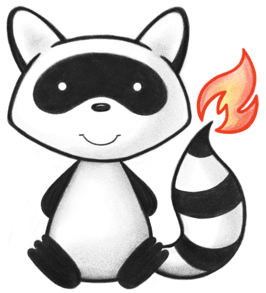
001package org.hl7.fhir.convertors.misc; 002 003import java.io.File; 004import java.io.IOException; 005import java.io.InputStream; 006 007import org.hl7.fhir.utilities.FileUtilities; 008import org.hl7.fhir.utilities.Utilities; 009import org.hl7.fhir.utilities.filesystem.DirectoryVisitor; 010import org.hl7.fhir.utilities.filesystem.DirectoryVisitor.IDirectoryVisitorImplementation; 011import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 012import org.hl7.fhir.utilities.npm.NpmPackage; 013 014public class SpecMapUnpacker { 015 016 public static void main(String[] args) throws IOException { 017 new SpecMapUnpacker().unpack(args[0]); 018 } 019 020 public class SpecMapScanner implements IDirectoryVisitorImplementation { 021 022 @Override 023 public boolean enterDirectory(File directory) throws IOException { 024 return true; 025 } 026 027 @Override 028 public boolean visitFile(File file) throws IOException { 029 System.out.println("Look at "+file.getAbsolutePath()); 030 try { 031 NpmPackage npm = NpmPackage.fromPackage(ManagedFileAccess.inStream(file)); 032 if (npm.hasFile("other", "spec.internals")) { 033 byte[] cnt = FileUtilities.streamToBytes(npm.load("other", "spec.internals")); 034 FileUtilities.bytesToFile(cnt, Utilities.path(FileUtilities.getDirectoryForFile(file.getAbsolutePath()), "page-map.json")); 035 System.out.println(" ...extracted"); 036 return true; 037 } else { 038 System.out.println(" ...not found"); 039 return false; 040 } 041 042 } catch (Exception e) { 043 System.out.println(" ...error: "+e.getMessage()); 044 return false; 045 } 046 } 047 048 } 049 050 private void unpack(String path) throws IOException { 051 System.out.println("Scanning "+path); 052 int count = DirectoryVisitor.visitDirectory(new SpecMapScanner(), path, "tgz"); 053 System.out.println("Done. "+count+" files extracted"); 054 } 055 056}