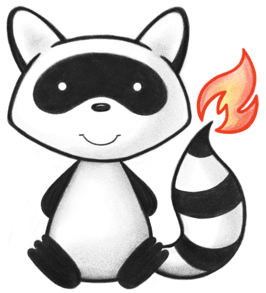
001package org.hl7.fhir.convertors.misc; 002 003 004 005 006 007import java.io.FileInputStream; 008import java.io.FileOutputStream; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038 039import org.fhir.ucum.UcumEssenceService; 040import org.hl7.fhir.convertors.misc.ccda.CCDAConverter; 041import org.hl7.fhir.dstu3.context.SimpleWorkerContext; 042import org.hl7.fhir.dstu3.formats.IParser; 043import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 044import org.hl7.fhir.dstu3.formats.JsonParser; 045import org.hl7.fhir.dstu3.formats.XmlParser; 046import org.hl7.fhir.dstu3.model.Bundle; 047import org.hl7.fhir.utilities.Utilities; 048import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 049 050public class Test { 051 public final static String DEF_TS_SERVER = "http://fhir-dev.healthintersections.com.au/open"; 052 public final static String DEV_TS_SERVER = "http://local.fhir.org:8080/open"; 053 054 055 public static final String DEF_PATH = "c:\\work\\org.hl7.fhir\\build\\implementations\\java\\org.hl7.fhir.convertors\\samples\\"; 056 public static final String UCUM_PATH = "c:\\work\\org.hl7.fhir\\build\\implementations\\java\\org.hl7.fhir.convertors\\samples\\ucum-essence.xml"; 057 public static final String SRC_PATH = "c:\\work\\org.hl7.fhir\\build\\publish\\"; 058 059 public static void main(String[] args) { 060 try { 061 CCDAConverter c = new CCDAConverter(new UcumEssenceService(UCUM_PATH), SimpleWorkerContext.fromPack(Utilities.path(SRC_PATH, "validation.zip"))); 062 Bundle a = c.convert(ManagedFileAccess.inStream(DEF_PATH + "ccda.xml")); 063 String fx = DEF_PATH + "output.xml"; 064 IParser x = new XmlParser().setOutputStyle(OutputStyle.PRETTY); 065 x.compose(ManagedFileAccess.outStream(fx), a); 066 String fj = DEF_PATH + "output.json"; 067 IParser j = new JsonParser().setOutputStyle(OutputStyle.PRETTY); 068 j.compose(ManagedFileAccess.outStream(fj), a); 069 System.out.println("done. save as " + fx + " and " + fj); 070 } catch (Exception e) { 071 e.printStackTrace(); 072 } 073 074 075 } 076 077}