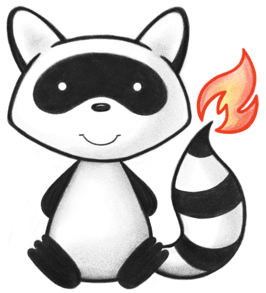
001package org.hl7.fhir.convertors.misc.utg; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileNotFoundException; 006import java.io.FileOutputStream; 007import java.io.IOException; 008import java.util.Calendar; 009import java.util.Date; 010 011import org.hl7.fhir.r4.formats.IParser; 012import org.hl7.fhir.r4.formats.IParser.OutputStyle; 013import org.hl7.fhir.r4.formats.JsonParser; 014import org.hl7.fhir.r4.formats.XmlParser; 015import org.hl7.fhir.r4.model.Bundle; 016import org.hl7.fhir.r4.model.Bundle.BundleType; 017import org.hl7.fhir.r4.model.CodeSystem; 018import org.hl7.fhir.r4.model.CodeSystem.CodeSystemContentMode; 019import org.hl7.fhir.r4.model.Period; 020import org.hl7.fhir.r4.model.Provenance; 021import org.hl7.fhir.r4.model.Provenance.ProvenanceAgentComponent; 022import org.hl7.fhir.r4.model.Resource; 023import org.hl7.fhir.utilities.Utilities; 024import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 025 026public class UTGCaseSensitivePopulator { 027 028 public static void main(String[] args) throws FileNotFoundException, IOException { 029 new UTGCaseSensitivePopulator().process(ManagedFileAccess.file(args[0])); 030 031 } 032 033 private void process(File root) throws FileNotFoundException, IOException { 034 Bundle bundle = new Bundle(); 035 bundle.setType(BundleType.COLLECTION); 036 bundle.setId("hxutg1-1-0-12"); 037 038 scanFolders(root, bundle); 039 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(Utilities.path(root.getAbsolutePath(), "input", "sourceOfTruth", "history", "utgrel1hx-1-0-12.json")), bundle); 040 System.out.println("Done"); 041 } 042 043 private void scanFolders(File folder, Bundle bundle) throws FileNotFoundException, IOException { 044 Calendar today = Calendar.getInstance(); 045 Date dt = today.getTime(); 046 today.set(Calendar.HOUR_OF_DAY, 0); 047 Date d = today.getTime(); 048 System.out.println("Scan "+folder.getAbsolutePath()); 049 050 for (File f : folder.listFiles()) { 051 if (f.isDirectory() && !f.getName().equals("retired")) { 052 scanFolders(f, bundle); 053 } else if (f.getName().endsWith(".xml")) { 054 processFile(f, bundle, new XmlParser(), d, dt); 055 } else if (f.getName().endsWith(".json")) { 056 processFile(f, bundle, new JsonParser(), d, dt); 057 } 058 } 059 060 } 061 062 private void processFile(File f, Bundle bundle, IParser parser, Date d, Date dt) { 063 try { 064 Resource r = parser.parse(ManagedFileAccess.inStream(f)); 065 if (r instanceof CodeSystem) { 066 if (processCodeSystem((CodeSystem) r, bundle, d, dt)) { 067 parser.setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), r); 068 } 069 } 070 } catch (Exception e) { 071 } 072 073 } 074 075 private boolean processCodeSystem(CodeSystem cs, Bundle bundle, Date d, Date dt) { 076 if (cs.hasCaseSensitive()) { 077 return false; 078 } 079 if (!cs.getUrl().startsWith("http://terminology.hl7.org") || cs.getContent() == CodeSystemContentMode.NOTPRESENT) { 080 return false; 081 } 082 cs.setCaseSensitive(true); 083 Provenance p = new Provenance(); 084 p.setId("cs-286-"+cs.getId()); 085 p.addTarget().setReference("CodeSystem/"+cs.getId()); 086 p.setOccurred(new Period()); 087 p.getOccurredPeriod().setEnd(d); 088 p.setRecorded(dt); 089 p.addReason().setText("Populate Missing caseSensitive property;UP-286").addCoding().setSystem("http://terminology.hl7.org/CodeSystem/v3-ActReason").setCode("METAMGT"); 090 p.getActivity().addCoding().setSystem("http://terminology.hl7.org/CodeSystem/v3-DataOperation").setCode("UPDATE"); 091 ProvenanceAgentComponent agent = p.addAgent(); 092 agent.getType().addCoding().setSystem("http://terminology.hl7.org/CodeSystem/provenance-participant-type").setCode("author"); 093 agent.getWho().setDisplay("Grahame Grieve"); 094 agent = p.addAgent(); 095 agent.getType().addCoding().setSystem("http://terminology.hl7.org/CodeSystem/provenance-participant-type").setCode("custodian"); 096 agent.getWho().setDisplay("Vocabulary WG"); 097 098 bundle.addEntry().setFullUrl("http://terminology.hl7.org/fhir/Provenance/cs-286-"+cs.getId()).setResource(p); 099 100 return true; 101 } 102 103}