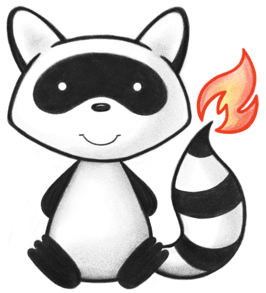
001package org.hl7.fhir.convertors.misc.utg; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.io.IOException; 007import java.net.URISyntaxException; 008import java.text.ParseException; 009import java.util.ArrayList; 010import java.util.Date; 011import java.util.HashMap; 012import java.util.List; 013import java.util.Map; 014 015import org.hl7.fhir.convertors.factory.VersionConvertorFactory_10_50; 016import org.hl7.fhir.convertors.factory.VersionConvertorFactory_30_50; 017import org.hl7.fhir.convertors.factory.VersionConvertorFactory_40_50; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r5.formats.IParser.OutputStyle; 020import org.hl7.fhir.r5.formats.JsonParser; 021import org.hl7.fhir.r5.formats.XmlParser; 022import org.hl7.fhir.r5.model.Base; 023import org.hl7.fhir.r5.model.Bundle; 024import org.hl7.fhir.r5.model.CanonicalResource; 025import org.hl7.fhir.r5.model.CodeSystem; 026import org.hl7.fhir.r5.model.Provenance; 027import org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent; 028import org.hl7.fhir.r5.model.Resource; 029import org.hl7.fhir.r5.model.ValueSet; 030import org.hl7.fhir.r5.utils.ToolingExtensions; 031import org.hl7.fhir.utilities.Utilities; 032import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 033import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager; 034import org.hl7.fhir.utilities.npm.NpmPackage; 035import org.hl7.fhir.utilities.npm.NpmPackage.PackageResourceInformation; 036 037import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 038 039@SuppressWarnings("checkstyle:systemout") 040public class UTGVersionSorter { 041 042 private final Date runTime = new Date(); 043 private FilesystemPackageCacheManager pcm; 044 045 public static void main(String[] args) throws FHIRException, IOException, ParseException, URISyntaxException { 046 new UTGVersionSorter().execute("C:\\work\\org.hl7.fhir.igs\\UTG\\input\\sourceOfTruth"); 047 } 048 049 private void execute(String source) throws IOException { 050 List<CanonicalResourceAnalysis> list = new ArrayList<>(); 051 System.out.println("Loading UTG"); 052 loadFromSource(list, ManagedFileAccess.file(source)); 053 054 Map<String, CanonicalResource> r2 = loadPackageR2("hl7.fhir.r2.core"); 055 Map<String, CanonicalResource> r3 = loadPackageR3("hl7.fhir.r3.core"); 056 Map<String, CanonicalResource> r4 = loadPackageR4("hl7.fhir.r4.core"); 057 058 System.out.println("Processing"); 059 for (CanonicalResourceAnalysis cr : list) { 060 cr.analyse(r2, r3, r4); 061 } 062 063 Bundle b = (Bundle) new JsonParser().parse(ManagedFileAccess.inStream("C:\\work\\org.hl7.fhir.igs\\UTG\\input\\sourceOfTruth\\history\\utgrel1hx-1-0-6.json")); 064 065 System.out.println("Summary"); 066 for (CanonicalResourceAnalysis cr : list) { 067 System.out.println(cr.summary()); 068 Provenance p = new Provenance(); 069 b.addEntry().setResource(p).setFullUrl("http://terminology.hl7.org/fhir/Provenance/fhir-1.0.51-" + cr.getId()); 070 p.setId("hx-fhir-1.0.51-" + cr.getId()); 071 p.addTarget().setReference(cr.fhirType() + "/" + cr.getId()); 072 p.getOccurredPeriod().setEnd(runTime, TemporalPrecisionEnum.DAY); 073 p.setRecorded(runTime); 074 p.addAuthorization().getConcept().setText("Reset Version after migration to UTG").addCoding("http://terminology.hl7.org/CodeSystem/v3-ActReason", "METAMGT", null); 075 p.getActivity().addCoding("http://terminology.hl7.org/CodeSystem/v3-DataOperation", "UPDATE", null); 076 ProvenanceAgentComponent pa = p.addAgent(); 077 pa.getType().addCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author", null); 078 pa.getWho().setDisplay("Grahame Grieve"); 079 pa = p.addAgent(); 080 pa.getType().addCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "custodian", null); 081 pa.getWho().setDisplay("Vocabulary WG"); 082 CanonicalResource res = cr.resource; 083 res.setVersion(cr.recommendation); 084 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(cr.filename), res); 085 } 086 System.out.println(); 087 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream("C:\\work\\org.hl7.fhir.igs\\UTG\\input\\sourceOfTruth\\history\\utgrel1hx-1-0-6.json"), b); 088 System.out.println("Done"); 089 } 090 091 private Map<String, CanonicalResource> loadPackageR2(String id) throws IOException { 092 Map<String, CanonicalResource> res = new HashMap<>(); 093 if (pcm == null) { 094 pcm = new FilesystemPackageCacheManager.Builder().build(); 095 } 096 System.out.println("Load " + id); 097 NpmPackage npm = pcm.loadPackage(id); 098 for (PackageResourceInformation p : npm.listIndexedResources("CodeSystem", "ValueSet")) { 099 CanonicalResource r = (CanonicalResource) VersionConvertorFactory_10_50.convertResource(new org.hl7.fhir.dstu2.formats.JsonParser().parse(npm.load(p))); 100 res.put(r.getUrl(), r); 101 } 102 return res; 103 } 104 105 private Map<String, CanonicalResource> loadPackageR3(String id) throws IOException { 106 Map<String, CanonicalResource> res = new HashMap<>(); 107 if (pcm == null) { 108 pcm = new FilesystemPackageCacheManager.Builder().build(); 109 } 110 System.out.println("Load " + id); 111 NpmPackage npm = pcm.loadPackage(id); 112 for (PackageResourceInformation p : npm.listIndexedResources("CodeSystem", "ValueSet")) { 113 CanonicalResource r = (CanonicalResource) VersionConvertorFactory_30_50.convertResource(new org.hl7.fhir.dstu3.formats.JsonParser().parse(npm.load(p))); 114 res.put(r.getUrl(), r); 115 } 116 return res; 117 } 118 119 private Map<String, CanonicalResource> loadPackageR4(String id) throws IOException { 120 Map<String, CanonicalResource> res = new HashMap<>(); 121 if (pcm == null) { 122 pcm = new FilesystemPackageCacheManager.Builder().build(); 123 } 124 System.out.println("Load " + id); 125 NpmPackage npm = pcm.loadPackage(id); 126 for (PackageResourceInformation p : npm.listIndexedResources("CodeSystem", "ValueSet")) { 127 CanonicalResource r = (CanonicalResource) VersionConvertorFactory_40_50.convertResource(new org.hl7.fhir.r4.formats.JsonParser().parse(npm.load(p))); 128 res.put(r.getUrl(), r); 129 } 130 return res; 131 } 132 133 private void loadFromSource(List<CanonicalResourceAnalysis> list, File source) { 134 for (File f : source.listFiles()) { 135 if (f.isDirectory()) { 136 loadFromSource(list, f); 137 } else if (f.getName().endsWith(".xml")) { 138 try { 139 Resource r = new XmlParser().parse(ManagedFileAccess.inStream(f)); 140 if (r instanceof CanonicalResource) { 141 CanonicalResource cr = (CanonicalResource) r; 142 cr.setWebPath(f.getAbsolutePath()); 143 if (cr.hasVersion() && cr.getVersion().startsWith("4.") && !Utilities.existsInList(cr.getId(), "v3-DataOperation", "v3-KnowledgeSubjectObservationValue")) { 144 list.add(new CanonicalResourceAnalysis(cr, f.getAbsolutePath())); 145 } 146 } 147 } catch (Exception e) { 148 System.out.println(f.getAbsolutePath() + " not a resource? " + e.getMessage()); 149 } 150 } 151 } 152 153 } 154 155 public class CanonicalResourceAnalysis { 156 157 private final CanonicalResource resource; 158 private final String filename; 159 private CanonicalResource r2; 160 private CanonicalResource r3; 161 private CanonicalResource r4; 162 private String fmm; 163 private boolean normative; 164 private String recommendation; 165 166 public CanonicalResourceAnalysis(CanonicalResource cr, String filename) { 167 this.resource = cr; 168 this.filename = filename; 169 } 170 171 public String summary() { 172// return "Relevant: "+resource.getUrl()+" [r2: "+r2Ver+"/"+r2Fmm+"]"+" [r3: "+r3Ver+"/"+r3Fmm+"]"+" [r4: "+r4Ver+"/"+r4Fmm+"/"+r4Normative+"] ---> "+recommendation; 173 return resource.getUrl() + " ---> " + recommendation + " in " + filename; 174 } 175 176 public void analyse(Map<String, CanonicalResource> r2l, Map<String, CanonicalResource> r3l, Map<String, CanonicalResource> r4l) { 177 r2 = findMatch(r2l); 178 r3 = findMatch(r3l); 179 r4 = findMatch(r4l); 180 181 fmm = r4 != null ? ToolingExtensions.readStringExtension(r4, ToolingExtensions.EXT_FMM_LEVEL) : null; 182 normative = (r4 != null) && ToolingExtensions.readStringExtension(r4, ToolingExtensions.EXT_NORMATIVE_VERSION) != null; 183 if (normative) { 184 recommendation = "1.0.0"; 185 } else if (Utilities.existsInList(fmm, "3", "4", "5")) { 186 recommendation = "0.5.0"; 187 } else { 188 int i = 1; 189 if (r2 != null && r3 != null && !match(r2, r3, r2l, r3l)) { 190 i++; 191 } 192 if (r3 != null && r4 != null && !match(r3, r4, r3l, r4l)) { 193 i++; 194 } 195 recommendation = "0." + i + ".0"; 196 } 197 } 198 199 private boolean match(CanonicalResource l, CanonicalResource r, Map<String, CanonicalResource> ll, Map<String, CanonicalResource> rl) { 200 if (l instanceof CodeSystem && r instanceof CodeSystem) { 201 return matchCS((CodeSystem) l, (CodeSystem) r); 202 } else if (l instanceof ValueSet && r instanceof ValueSet) { 203 return matchVS((ValueSet) l, (ValueSet) r, ll, rl); 204 } else { 205 return false; 206 } 207 } 208 209 private boolean matchVS(ValueSet l, ValueSet r, Map<String, CanonicalResource> ll, Map<String, CanonicalResource> rl) { 210 if (l.getCompose().getInclude().size() == 1 && l.getCompose().getExclude().isEmpty() && l.getCompose().getIncludeFirstRep().hasSystem() && !l.getCompose().getIncludeFirstRep().hasConcept() && !l.getCompose().getIncludeFirstRep().hasFilter() && 211 r.getCompose().getInclude().size() == 1 && r.getCompose().getExclude().isEmpty() && r.getCompose().getIncludeFirstRep().hasSystem() && !r.getCompose().getIncludeFirstRep().hasConcept() && !r.getCompose().getIncludeFirstRep().hasFilter()) { 212 CodeSystem lc = (CodeSystem) ll.get(l.getCompose().getIncludeFirstRep().getSystem()); 213 CodeSystem rc = (CodeSystem) rl.get(l.getCompose().getIncludeFirstRep().getSystem()); 214 if (lc != null && rc != null) { 215 return matchCS(lc, rc); 216 } 217 } 218 return false; 219 } 220 221 private boolean matchCS(CodeSystem l, CodeSystem r) { 222 return Base.compareDeep(l.getConcept(), r.getConcept(), false); 223 } 224 225 public CanonicalResource findMatch(Map<String, CanonicalResource> r2) { 226 CanonicalResource r = r2.get(resource.getUrl()); 227 if (r == null) { 228 r = r2.get(resource.getUrl().replaceAll("http://terminology.hl7.org/", "http://hl7.org/fhir/")); 229 } 230 if (r == null) { 231 r = r2.get(resource.getUrl().replaceAll("http://terminology.hl7.org/CodeSystem", "http://hl7.org/fhir/")); 232 } 233 return r; 234 } 235 236 public String getId() { 237 return resource.getId(); 238 } 239 240 public String fhirType() { 241 return resource.fhirType(); 242 } 243 244 } 245 246 247}