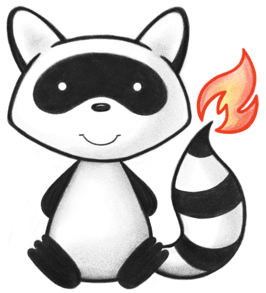
001package org.hl7.fhir.convertors.txClient; 002 003import java.net.URISyntaxException; 004 005import org.hl7.fhir.r5.terminologies.client.ITerminologyClient; 006import org.hl7.fhir.r5.terminologies.client.TerminologyClientManager.ITerminologyClientFactory; 007import org.hl7.fhir.r5.terminologies.client.TerminologyClientR5; 008import org.hl7.fhir.utilities.FhirPublication; 009import org.hl7.fhir.utilities.ToolingClientLogger; 010import org.hl7.fhir.utilities.Utilities; 011import org.hl7.fhir.utilities.VersionUtilities; 012 013public class TerminologyClientFactory implements ITerminologyClientFactory { 014 015 private String v; 016 017 public TerminologyClientFactory(FhirPublication v) { 018 super(); 019 this.v = v == null ? null : v.toCode(); 020 } 021 022 public TerminologyClientFactory(String version) { 023 super(); 024 this.v = version; 025 } 026 027 @Override 028 public ITerminologyClient makeClient(String id, String url, String userAgent, ToolingClientLogger logger) throws URISyntaxException { 029 if (v == null) 030 return new TerminologyClientR5(id, checkEndsWith("/r4", url), userAgent).setLogger(logger); 031 v = VersionUtilities.getMajMin(v); 032 if (VersionUtilities.isR2Ver(v)) { 033 return new TerminologyClientR2(id, checkEndsWith("/r2", url), userAgent).setLogger(logger); 034 } 035 if (VersionUtilities.isR2BVer(v)) { 036 return new TerminologyClientR3(id, checkEndsWith("/r3", url), userAgent).setLogger(logger); // r3 is the least worst match 037 } 038 if (VersionUtilities.isR3Ver(v)) { 039 return new TerminologyClientR3(id, checkEndsWith("/r3", url), userAgent).setLogger(logger); // r3 is the least worst match 040 } 041 if (VersionUtilities.isR4Ver(v)) { 042 return new TerminologyClientR4(id, checkEndsWith("/r4", url), userAgent).setLogger(logger); 043 } 044 if (VersionUtilities.isR4BVer(v)) { 045 return new TerminologyClientR4(id, checkEndsWith("/r4", url), userAgent).setLogger(logger); 046 } 047 if (VersionUtilities.isR5Plus(v)) { 048 return new TerminologyClientR5(id, checkEndsWith("/r5", url), userAgent).setLogger(logger); // r4 for now, since the terminology is currently the same 049 } 050 throw new Error("The version " + v + " is not currently supported"); 051 } 052 053 private String checkEndsWith(String term, String url) { 054 if (url.endsWith(term)) 055 return url; 056 if (Utilities.isTxFhirOrgServer(url)) { 057 return Utilities.pathURL(url, term); 058 } 059 return url; 060 } 061 062 @Override 063 public String getVersion() { 064 return v; 065 } 066 067}