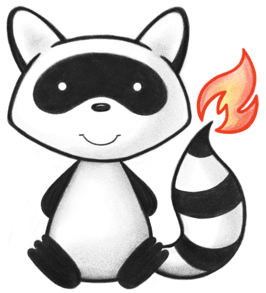
001package org.hl7.fhir.convertors.txClient; 002 003import java.net.URISyntaxException; 004import java.util.EnumSet; 005import java.util.HashMap; 006import java.util.Map; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035 */ 036 037 038import org.hl7.fhir.convertors.conv10_50.resources10_50.TerminologyCapabilities10_50; 039import org.hl7.fhir.convertors.factory.VersionConvertorFactory_10_50; 040import org.hl7.fhir.convertors.factory.VersionConvertorFactory_40_50; 041import org.hl7.fhir.dstu2.model.Resource; 042import org.hl7.fhir.dstu2.utils.client.FHIRToolingClient; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.r5.model.Bundle; 045import org.hl7.fhir.r5.model.CanonicalResource; 046import org.hl7.fhir.r5.model.CapabilityStatement; 047import org.hl7.fhir.r5.model.Parameters; 048import org.hl7.fhir.r5.model.TerminologyCapabilities; 049import org.hl7.fhir.r5.model.ValueSet; 050import org.hl7.fhir.r5.model.Parameters.ParametersParameterComponent; 051import org.hl7.fhir.r5.terminologies.client.ITerminologyClient; 052import org.hl7.fhir.r5.utils.client.network.ClientHeaders; 053import org.hl7.fhir.utilities.FhirPublication; 054import org.hl7.fhir.utilities.ToolingClientLogger; 055import org.hl7.fhir.utilities.Utilities; 056 057public class TerminologyClientR2 implements ITerminologyClient { 058 059 private final FHIRToolingClient client; // todo: use the R2 client 060 private String id; 061 062 public EnumSet<FhirPublication> supportableVersions() { 063 return EnumSet.of(FhirPublication.DSTU2); 064 } 065 066 public void setAllowedVersions(EnumSet<FhirPublication> versions) { 067 boolean found = false; 068 for (FhirPublication version : versions) { 069 if (version == FhirPublication.DSTU2) { 070 found = true; 071 } else { 072 throw new Error("Unsupported version for DSTU2 client: "+version.toCode()); 073 } 074 } 075 if (!found) { 076 throw new Error("DSTU2 client only supports DSTU2"); 077 } 078 } 079 080 public EnumSet<FhirPublication> getAllowedVersions() { 081 return EnumSet.of(FhirPublication.DSTU2); 082 } 083 084 public FhirPublication getActualVersion() { 085 return FhirPublication.DSTU2; 086 } 087 088 public TerminologyClientR2(String id, String address, String userAgent) throws URISyntaxException { 089 client = new FHIRToolingClient(address, userAgent); 090 this.client.setVersionInMimeTypes(true); 091 this.id = id; 092 } 093 094 @Override 095 public String getId() { 096 return id; 097 } 098 099 @Override 100 public TerminologyCapabilities getTerminologyCapabilities() throws FHIRException { 101 return TerminologyCapabilities10_50.convertTerminologyCapabilities(client.getTerminologyCapabilities()); 102 } 103 104 @Override 105 public String getAddress() { 106 return client.getAddress(); 107 } 108 109 @Override 110 public ValueSet expandValueset(ValueSet vs, Parameters p) throws FHIRException { 111 org.hl7.fhir.dstu2.model.ValueSet vs2 = (org.hl7.fhir.dstu2.model.ValueSet) VersionConvertorFactory_10_50.convertResource(vs); 112 org.hl7.fhir.dstu2.model.Parameters p2 = (org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(p); 113 vs2 = client.expandValueset(vs2, p2); 114 return (ValueSet) VersionConvertorFactory_10_50.convertResource(vs2); 115 } 116 117 @Override 118 public Parameters validateCS(Parameters pin) throws FHIRException { 119 org.hl7.fhir.dstu2.model.Parameters p2 = (org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(pin); 120 p2 = client.operateType(org.hl7.fhir.dstu2.model.ValueSet.class, "validate-code", p2); 121 return (Parameters) VersionConvertorFactory_10_50.convertResource(p2); 122 } 123 124 @Override 125 public Parameters subsumes(Parameters pin) throws FHIRException { 126 org.hl7.fhir.dstu2.model.Parameters p2 = (org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(pin); 127 p2 = client.operateType(org.hl7.fhir.dstu2.model.ValueSet.class, "subsumes", p2); 128 return (Parameters) VersionConvertorFactory_10_50.convertResource(p2); 129 } 130 131 @Override 132 public Parameters validateVS(Parameters pin) throws FHIRException { 133 org.hl7.fhir.dstu2.model.Parameters p2 = (org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(pin); 134 p2 = client.operateType(org.hl7.fhir.dstu2.model.ValueSet.class, "validate-code", p2); 135 return (Parameters) VersionConvertorFactory_10_50.convertResource(p2); 136 } 137 138 @Override 139 public ITerminologyClient setTimeoutFactor(int i) { 140 client.setTimeoutFactor(i); 141 return this; 142 } 143 144 @Override 145 public ToolingClientLogger getLogger() { 146 return client.getLogger(); 147 } 148 149 @Override 150 public ITerminologyClient setLogger(ToolingClientLogger txLog) { 151 client.setLogger(txLog); 152 return this; 153 } 154 155 @Override 156 public ITerminologyClient setRetryCount(int retryCount) throws FHIRException { 157 client.setRetryCount(retryCount); 158 return this; 159 } 160 161 @Override 162 public CapabilityStatement getCapabilitiesStatementQuick() throws FHIRException { 163 return (CapabilityStatement) VersionConvertorFactory_10_50.convertResource(client.getConformanceStatementQuick()); 164 } 165 166 @Override 167 public Parameters lookupCode(Map<String, String> params) throws FHIRException { 168 return (Parameters) VersionConvertorFactory_10_50.convertResource(client.lookupCode(params)); 169 } 170 171 @Override 172 public Parameters lookupCode(Parameters params) throws FHIRException { 173 return (Parameters) VersionConvertorFactory_10_50.convertResource(client.lookupCode((org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(params))); 174 } 175 176 @Override 177 public int getRetryCount() throws FHIRException { 178 return client.getRetryCount(); 179 } 180 181 @Override 182 public Bundle validateBatch(Bundle batch) { 183 return (Bundle) VersionConvertorFactory_10_50.convertResource(client.transaction((org.hl7.fhir.dstu2.model.Bundle) VersionConvertorFactory_10_50.convertResource(batch))); 184 } 185 186 @Override 187 public CanonicalResource read(String type, String id) { 188 Class<Resource> t; 189 try { 190 t = (Class<Resource>) Class.forName("org.hl7.fhir.dstu2.model." + type);// todo: do we have to deal with any resource renaming? Use cases are limited... 191 } catch (ClassNotFoundException e) { 192 throw new FHIRException("Unable to fetch resources of type " + type + " in R2"); 193 } 194 org.hl7.fhir.dstu2.model.Resource r2 = client.read(t, id); 195 if (r2 == null) { 196 throw new FHIRException("Unable to fetch resource " + Utilities.pathURL(getAddress(), type, id)); 197 } 198 org.hl7.fhir.r5.model.Resource r5 = VersionConvertorFactory_10_50.convertResource(r2); 199 if (r5 == null) { 200 throw new FHIRException("Unable to convert resource " + Utilities.pathURL(getAddress(), type, id) + " to R5 (internal representation)"); 201 } 202 if (!(r5 instanceof CanonicalResource)) { 203 throw new FHIRException("Unable to convert resource " + Utilities.pathURL(getAddress(), type, id) + " to R5 canonical resource (internal representation)"); 204 } 205 return (CanonicalResource) r5; 206 } 207 208 @Override 209 public ClientHeaders getClientHeaders() { 210 return null; 211 } 212 213 @Override 214 public ITerminologyClient setClientHeaders(ClientHeaders clientHeaders) { 215 return null; 216 } 217 218 @Override 219 public ITerminologyClient setUserAgent(String userAgent) { 220 client.setUserAgent(userAgent); 221 return this; 222 } 223 224 @Override 225 public String getUserAgent() { 226 return client.getUserAgent(); 227 } 228 229 @Override 230 public String getServerVersion() { 231 return client.getServerVersion(); 232 } 233 234 @Override 235 public ITerminologyClient setAcceptLanguage(String lang) { 236 client.setAcceptLanguage(lang); 237 return this; 238 } 239 240 @Override 241 public ITerminologyClient setContentLanguage(String lang) { 242 client.setContentLanguage(lang); 243 return this; 244 } 245 246 @Override 247 public int getUseCount() { 248 return client.getUseCount(); 249 } 250 251 @Override 252 public Bundle search(String type, String criteria) { 253 org.hl7.fhir.dstu2.model.Bundle result = client.search(type, criteria); 254 return result == null ? null : (Bundle) VersionConvertorFactory_10_50.convertResource(result); 255 } 256 257 @Override 258 public Parameters translate(Parameters params) throws FHIRException { 259 return (Parameters) VersionConvertorFactory_10_50.convertResource(client.translate((org.hl7.fhir.dstu2.model.Parameters) VersionConvertorFactory_10_50.convertResource(params))); 260 } 261 262}