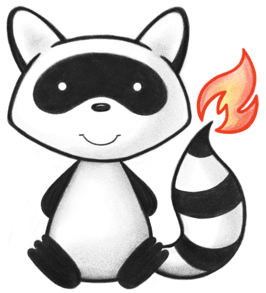
001/* 002 * #%L 003 * HAPI FHIR JAX-RS Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jaxrs.client; 021 022import ca.uhn.fhir.rest.client.api.IHttpResponse; 023import ca.uhn.fhir.rest.client.impl.BaseHttpResponse; 024import ca.uhn.fhir.util.StopWatch; 025import jakarta.ws.rs.core.MediaType; 026import jakarta.ws.rs.core.Response; 027 028import java.io.ByteArrayInputStream; 029import java.io.IOException; 030import java.io.InputStream; 031import java.io.Reader; 032import java.io.StringReader; 033import java.util.List; 034import java.util.Map; 035import java.util.Map.Entry; 036import java.util.concurrent.ConcurrentHashMap; 037 038/** 039 * A Http Response based on JaxRs. This is an adapter around the class {@link jakarta.ws.rs.core.Response Response} 040 * @author Peter Van Houte | peter.vanhoute@agfa.com | Agfa Healthcare 041 */ 042public class JaxRsHttpResponse extends BaseHttpResponse implements IHttpResponse { 043 044 private boolean myBufferedEntity = false; 045 private final Response myResponse; 046 047 public JaxRsHttpResponse(Response theResponse, StopWatch theResponseStopWatch) { 048 super(theResponseStopWatch); 049 this.myResponse = theResponse; 050 } 051 052 @Override 053 public void bufferEntity() throws IOException { 054 if (!myBufferedEntity && myResponse.hasEntity()) { 055 myBufferedEntity = true; 056 myResponse.bufferEntity(); 057 } else { 058 myResponse.bufferEntity(); 059 } 060 } 061 062 @Override 063 public void close() { 064 // automatically done by jax-rs 065 } 066 067 @Override 068 public Reader createReader() { 069 if (!myBufferedEntity && !myResponse.hasEntity()) { 070 return new StringReader(""); 071 } else { 072 return new StringReader(myResponse.readEntity(String.class)); 073 } 074 } 075 076 @Override 077 public Map<String, List<String>> getAllHeaders() { 078 Map<String, List<String>> theHeaders = new ConcurrentHashMap<String, List<String>>(); 079 for (Entry<String, List<String>> iterable_element : 080 myResponse.getStringHeaders().entrySet()) { 081 theHeaders.put(iterable_element.getKey().toLowerCase(), iterable_element.getValue()); 082 } 083 return theHeaders; 084 } 085 086 @Override 087 public String getMimeType() { 088 MediaType mediaType = myResponse.getMediaType(); 089 if (mediaType == null) { 090 return null; 091 } 092 // Keep only type and subtype and do not include the parameters such as charset 093 return new MediaType(mediaType.getType(), mediaType.getSubtype()).toString(); 094 } 095 096 @Override 097 public Response getResponse() { 098 return myResponse; 099 } 100 101 @Override 102 public int getStatus() { 103 return myResponse.getStatus(); 104 } 105 106 @Override 107 public String getStatusInfo() { 108 return myResponse.getStatusInfo().getReasonPhrase(); 109 } 110 111 @Override 112 public InputStream readEntity() { 113 if (!myBufferedEntity && !myResponse.hasEntity()) { 114 return new ByteArrayInputStream(new byte[0]); 115 } else { 116 return new ByteArrayInputStream(myResponse.readEntity(byte[].class)); 117 } 118 } 119 120 @Override 121 public List<String> getHeaders(String theName) { 122 List<String> retVal = myResponse.getStringHeaders().get(theName); 123 return retVal; 124 } 125}