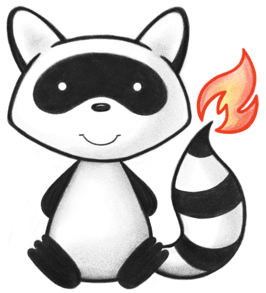
001/* 002 * #%L 003 * HAPI FHIR JAX-RS Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jaxrs.server; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.api.BundleInclusionRule; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.jaxrs.server.interceptor.JaxRsExceptionInterceptor; 026import ca.uhn.fhir.jaxrs.server.interceptor.JaxRsResponseException; 027import ca.uhn.fhir.jaxrs.server.util.JaxRsRequest; 028import ca.uhn.fhir.rest.api.Constants; 029import ca.uhn.fhir.rest.api.PreferReturnEnum; 030import ca.uhn.fhir.rest.api.RequestTypeEnum; 031import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 032import ca.uhn.fhir.rest.api.server.IRestfulServer; 033import ca.uhn.fhir.rest.server.IPagingProvider; 034import ca.uhn.fhir.rest.server.PageProvider; 035import ca.uhn.fhir.rest.server.method.PageMethodBinding; 036import jakarta.interceptor.Interceptors; 037import jakarta.ws.rs.GET; 038import jakarta.ws.rs.Produces; 039import jakarta.ws.rs.QueryParam; 040import jakarta.ws.rs.core.MediaType; 041import jakarta.ws.rs.core.Response; 042 043import java.io.IOException; 044 045/** 046 * Base class for a provider to provide the <code>[baseUrl]?_getpages=foo</code> request, which is a request to the 047 * server to retrieve the next page of a set of paged results. 048 */ 049@Produces({MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML, MediaType.TEXT_PLAIN}) 050@Interceptors(JaxRsExceptionInterceptor.class) 051public abstract class AbstractJaxRsPageProvider extends AbstractJaxRsProvider implements IRestfulServer<JaxRsRequest> { 052 053 private PageMethodBinding myBinding; 054 055 /** 056 * The default constructor. 057 */ 058 protected AbstractJaxRsPageProvider() { 059 try { 060 myBinding = new PageMethodBinding(getFhirContext(), PageProvider.class.getMethod("getPage")); 061 } catch (Exception e) { 062 throw new ca.uhn.fhir.context.ConfigurationException(Msg.code(1983), e); 063 } 064 } 065 066 /** 067 * Provides the ability to set the {@link FhirContext} instance. 068 * @param ctx the {@link FhirContext} instance. 069 */ 070 protected AbstractJaxRsPageProvider(FhirContext ctx) { 071 super(ctx); 072 try { 073 myBinding = new PageMethodBinding(getFhirContext(), PageProvider.class.getMethod("getPage")); 074 } catch (Exception e) { 075 throw new ca.uhn.fhir.context.ConfigurationException(Msg.code(1984), e); 076 } 077 } 078 079 @Override 080 public String getBaseForRequest() { 081 try { 082 return getUriInfo().getBaseUri().toURL().toExternalForm(); 083 } catch (Exception e) { 084 // cannot happen 085 return null; 086 } 087 } 088 089 /** 090 * This method implements the "getpages" action 091 */ 092 @GET 093 public Response getPages(@QueryParam(Constants.PARAM_PAGINGACTION) String thePageId) throws IOException { 094 JaxRsRequest theRequest = 095 getRequest(RequestTypeEnum.GET, RestOperationTypeEnum.GET_PAGE).build(); 096 try { 097 return (Response) myBinding.invokeServer(this, theRequest); 098 } catch (JaxRsResponseException theException) { 099 return new JaxRsExceptionInterceptor().convertExceptionIntoResponse(theRequest, theException); 100 } 101 } 102 103 /** 104 * Default: no paging provider 105 */ 106 @Override 107 public IPagingProvider getPagingProvider() { 108 return null; 109 } 110 111 /** 112 * Default: BundleInclusionRule.BASED_ON_INCLUDES 113 */ 114 @Override 115 public BundleInclusionRule getBundleInclusionRule() { 116 return BundleInclusionRule.BASED_ON_INCLUDES; 117 } 118 119 @Override 120 public PreferReturnEnum getDefaultPreferReturn() { 121 return PreferReturnEnum.REPRESENTATION; 122 } 123}