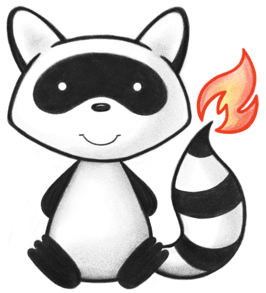
Package ca.uhn.fhir.jpa.dao.data
Interface IBatch2WorkChunkRepository
- All Superinterfaces:
org.springframework.data.repository.CrudRepository<Batch2WorkChunkEntity,
,String> IHapiFhirJpaRepository
,org.springframework.data.jpa.repository.JpaRepository<Batch2WorkChunkEntity,
,String> org.springframework.data.repository.ListCrudRepository<Batch2WorkChunkEntity,
,String> org.springframework.data.repository.ListPagingAndSortingRepository<Batch2WorkChunkEntity,
,String> org.springframework.data.repository.PagingAndSortingRepository<Batch2WorkChunkEntity,
,String> org.springframework.data.repository.query.QueryByExampleExecutor<Batch2WorkChunkEntity>
,org.springframework.data.repository.Repository<Batch2WorkChunkEntity,
String>
public interface IBatch2WorkChunkRepository
extends org.springframework.data.jpa.repository.JpaRepository<Batch2WorkChunkEntity,String>, IHapiFhirJpaRepository
-
Method Summary
Modifier and TypeMethodDescriptionint
deleteAllForInstance
(String theInstanceId) fetchAllChunkIdsForStepWithStatus
(String theInstanceId, String theStepId, WorkChunkStatusEnum theStatus) fetchChunks
(org.springframework.data.domain.Pageable thePageRequest, String theInstanceId) fetchChunksForStep
(String theInstanceId, String theTargetStepId) fetchChunksNoData
(org.springframework.data.domain.Pageable thePageRequest, String theInstanceId) A projection query to avoid fetching the CLOB over the wire.fetchWorkChunkStatusForInstance
(String theInstanceId) getDistinctStatusesForStep
(String theInstanceId, String theStepId) void
updateAllChunksForInstanceStatusClearDataAndSetError
(List<String> theChunkIds, Date theEndTime, WorkChunkStatusEnum theInProgress, String theError) int
updateAllChunksForStepWithStatus
(String theInstanceId, String theStepId, List<WorkChunkStatusEnum> theOldStatuses, WorkChunkStatusEnum theNewStatus) int
updateChunkForTooManyErrors
(WorkChunkStatusEnum theStatus, String theChunkId, int theMaxErrorCount, int theMaxErrorSize) Updates the workchunk error count and error message for WorkChunks that have failed after multiple retries.int
updateChunkStatus
(String theChunkId, WorkChunkStatusEnum theOldStatus, WorkChunkStatusEnum theNewStatus) void
updateChunkStatusAndClearDataForEndSuccess
(String theChunkId, Date theEndTime, int theRecordsProcessed, int theErrorRetries, WorkChunkStatusEnum theInProgress, String theWarningMessage) int
updateChunkStatusAndIncrementErrorCountForEndError
(String theChunkId, Date theEndTime, String theErrorMessage, WorkChunkStatusEnum theInProgress) int
updateChunkStatusForStart
(String theChunkId, Date theStartedTime, WorkChunkStatusEnum theInProgress, Collection<WorkChunkStatusEnum> theStartStatuses) int
updateWorkChunkNextPollTime
(String theChunkId, WorkChunkStatusEnum theStatus, Set<WorkChunkStatusEnum> theInitialStates, Date theNextPollTime) int
updateWorkChunksForPollWaiting
(String theInstanceId, Date theTime, Set<WorkChunkStatusEnum> theInitialStates, WorkChunkStatusEnum theNewStatus) Methods inherited from interface org.springframework.data.repository.CrudRepository
count, delete, deleteAll, deleteAll, deleteAllById, deleteById, existsById, findById, save
Methods inherited from interface org.springframework.data.jpa.repository.JpaRepository
deleteAllByIdInBatch, deleteAllInBatch, deleteAllInBatch, deleteInBatch, findAll, findAll, flush, getById, getOne, getReferenceById, saveAllAndFlush, saveAndFlush
Methods inherited from interface org.springframework.data.repository.ListCrudRepository
findAll, findAllById, saveAll
Methods inherited from interface org.springframework.data.repository.ListPagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.PagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.query.QueryByExampleExecutor
count, exists, findAll, findBy, findOne
-
Method Details
-
fetchChunks
@Query("SELECT e FROM Batch2WorkChunkEntity e WHERE e.myInstanceId = :instanceId ORDER BY e.mySequence ASC, e.myId ASC") List<Batch2WorkChunkEntity> fetchChunks(org.springframework.data.domain.Pageable thePageRequest, @Param("instanceId") String theInstanceId) -
fetchChunksNoData
@Query("SELECT new Batch2WorkChunkEntity(e.myId, e.mySequence, e.myJobDefinitionId, e.myJobDefinitionVersion, e.myInstanceId, e.myTargetStepId, e.myStatus,e.myCreateTime, e.myStartTime, e.myUpdateTime, e.myEndTime,e.myErrorMessage, e.myErrorCount, e.myRecordsProcessed, e.myWarningMessage,e.myNextPollTime, e.myPollAttempts) FROM Batch2WorkChunkEntity e WHERE e.myInstanceId = :instanceId ORDER BY e.mySequence ASC, e.myId ASC") List<Batch2WorkChunkEntity> fetchChunksNoData(org.springframework.data.domain.Pageable thePageRequest, @Param("instanceId") String theInstanceId) A projection query to avoid fetching the CLOB over the wire. Otherwise, the same as fetchChunks. -
getDistinctStatusesForStep
@Query("SELECT DISTINCT e.myStatus from Batch2WorkChunkEntity e where e.myInstanceId = :instanceId AND e.myTargetStepId = :stepId") Set<WorkChunkStatusEnum> getDistinctStatusesForStep(@Param("instanceId") String theInstanceId, @Param("stepId") String theStepId) -
fetchChunksForStep
@Query("SELECT e FROM Batch2WorkChunkEntity e WHERE e.myInstanceId = :instanceId AND e.myTargetStepId = :targetStepId ORDER BY e.mySequence ASC") Stream<Batch2WorkChunkEntity> fetchChunksForStep(@Param("instanceId") String theInstanceId, @Param("targetStepId") String theTargetStepId) -
updateChunkStatusAndClearDataForEndSuccess
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myEndTime = :et, e.myRecordsProcessed = :rp, e.myErrorCount = e.myErrorCount + :errorRetries, e.mySerializedData = null, e.mySerializedDataVc = null, e.myWarningMessage = :warningMessage WHERE e.myId = :id") void updateChunkStatusAndClearDataForEndSuccess(@Param("id") String theChunkId, @Param("et") Date theEndTime, @Param("rp") int theRecordsProcessed, @Param("errorRetries") int theErrorRetries, @Param("status") WorkChunkStatusEnum theInProgress, @Param("warningMessage") String theWarningMessage) -
updateWorkChunkNextPollTime
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myNextPollTime = :nextPollTime, e.myPollAttempts = COALESCE(e.myPollAttempts, 0) + 1 WHERE e.myId = :id AND e.myStatus IN(:states)") int updateWorkChunkNextPollTime(@Param("id") String theChunkId, @Param("status") WorkChunkStatusEnum theStatus, @Param("states") Set<WorkChunkStatusEnum> theInitialStates, @Param("nextPollTime") Date theNextPollTime) -
updateWorkChunksForPollWaiting
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myNextPollTime = null WHERE e.myInstanceId = :instanceId AND e.myStatus IN(:states) AND e.myNextPollTime <= :pollTime") int updateWorkChunksForPollWaiting(@Param("instanceId") String theInstanceId, @Param("pollTime") Date theTime, @Param("states") Set<WorkChunkStatusEnum> theInitialStates, @Param("status") WorkChunkStatusEnum theNewStatus) -
updateAllChunksForInstanceStatusClearDataAndSetError
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myEndTime = :et, e.mySerializedData = null, e.mySerializedDataVc = null, e.myErrorMessage = :em WHERE e.myId IN(:ids)") void updateAllChunksForInstanceStatusClearDataAndSetError(@Param("ids") List<String> theChunkIds, @Param("et") Date theEndTime, @Param("status") WorkChunkStatusEnum theInProgress, @Param("em") String theError) -
updateChunkStatusAndIncrementErrorCountForEndError
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myEndTime = :et, e.myErrorMessage = :em, e.myErrorCount = e.myErrorCount + 1 WHERE e.myId = :id") int updateChunkStatusAndIncrementErrorCountForEndError(@Param("id") String theChunkId, @Param("et") Date theEndTime, @Param("em") String theErrorMessage, @Param("status") WorkChunkStatusEnum theInProgress) -
updateChunkForTooManyErrors
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :failed, e.myErrorMessage = LEFT(CONCAT(\'Too many errors: \', CAST(e.myErrorCount as string), \'. Last err msg \', e.myErrorMessage), :maxErrorSize) WHERE e.myId = :chunkId and e.myErrorCount > :maxCount") int updateChunkForTooManyErrors(@Param("failed") WorkChunkStatusEnum theStatus, @Param("chunkId") String theChunkId, @Param("maxCount") int theMaxErrorCount, @Param("maxErrorSize") int theMaxErrorSize) Updates the workchunk error count and error message for WorkChunks that have failed after multiple retries.- Parameters:
theStatus
- - the new status of the workchunktheChunkId
- - the id of the workchunk to updatetheMaxErrorCount
- - maximum error count (# of errors allowed for retry)theMaxErrorSize
- - max error size (maximum number of characters)- Returns:
- - the number of updated chunks (should be 1)
-
updateChunkStatusForStart
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :status, e.myStartTime = :st WHERE e.myId = :id AND e.myStatus IN :startStatuses") int updateChunkStatusForStart(@Param("id") String theChunkId, @Param("st") Date theStartedTime, @Param("status") WorkChunkStatusEnum theInProgress, @Param("startStatuses") Collection<WorkChunkStatusEnum> theStartStatuses) -
updateChunkStatus
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :newStatus WHERE e.myId = :id AND e.myStatus = :oldStatus") int updateChunkStatus(@Param("id") String theChunkId, @Param("oldStatus") WorkChunkStatusEnum theOldStatus, @Param("newStatus") WorkChunkStatusEnum theNewStatus) -
updateAllChunksForStepWithStatus
@Modifying @Query("UPDATE Batch2WorkChunkEntity e SET e.myStatus = :newStatus WHERE e.myInstanceId = :instanceId AND e.myTargetStepId = :stepId AND e.myStatus IN ( :oldStatuses )") int updateAllChunksForStepWithStatus(@Param("instanceId") String theInstanceId, @Param("stepId") String theStepId, @Param("oldStatuses") List<WorkChunkStatusEnum> theOldStatuses, @Param("newStatus") WorkChunkStatusEnum theNewStatus) -
deleteAllForInstance
@Modifying @Query("DELETE FROM Batch2WorkChunkEntity e WHERE e.myInstanceId = :instanceId") int deleteAllForInstance(@Param("instanceId") String theInstanceId) -
fetchAllChunkIdsForStepWithStatus
@Query("SELECT e.myId from Batch2WorkChunkEntity e where e.myInstanceId = :instanceId AND e.myTargetStepId = :stepId AND e.myStatus = :status") List<String> fetchAllChunkIdsForStepWithStatus(@Param("instanceId") String theInstanceId, @Param("stepId") String theStepId, @Param("status") WorkChunkStatusEnum theStatus) -
fetchWorkChunkStatusForInstance
@Query("SELECT new ca.uhn.fhir.batch2.model.BatchWorkChunkStatusDTO(e.myTargetStepId, e.myStatus, min(e.myStartTime), max(e.myEndTime), avg(cast((e.myEndTime - e.myStartTime) as long)), count(*)) FROM Batch2WorkChunkEntity e WHERE e.myInstanceId=:instanceId GROUP BY e.myTargetStepId, e.myStatus") List<BatchWorkChunkStatusDTO> fetchWorkChunkStatusForInstance(@Param("instanceId") String theInstanceId)
-