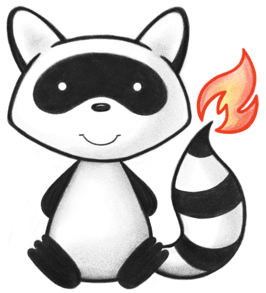
Package ca.uhn.fhir.jpa.dao.data
Interface IResourceHistoryTableDao
- All Superinterfaces:
org.springframework.data.repository.CrudRepository<ResourceHistoryTable,
,ResourceHistoryTablePk> IHapiFhirJpaRepository
,org.springframework.data.jpa.repository.JpaRepository<ResourceHistoryTable,
,ResourceHistoryTablePk> org.springframework.data.repository.ListCrudRepository<ResourceHistoryTable,
,ResourceHistoryTablePk> org.springframework.data.repository.ListPagingAndSortingRepository<ResourceHistoryTable,
,ResourceHistoryTablePk> org.springframework.data.repository.PagingAndSortingRepository<ResourceHistoryTable,
,ResourceHistoryTablePk> org.springframework.data.repository.query.QueryByExampleExecutor<ResourceHistoryTable>
,org.springframework.data.repository.Repository<ResourceHistoryTable,
ResourceHistoryTablePk>
public interface IResourceHistoryTableDao
extends org.springframework.data.jpa.repository.JpaRepository<ResourceHistoryTable,ResourceHistoryTablePk>, IHapiFhirJpaRepository
-
Method Summary
Modifier and TypeMethodDescriptionvoid
org.springframework.data.domain.Slice
<ResourceHistoryTable> findAllVersionsExceptSpecificForResourcePid
(org.springframework.data.domain.Pageable thePage, JpaPidFk theId, Long theDontWantVersion) This is really only intended for unit tests - There can be many versions of resources in the real world, use a pageable query for real uses.findCurrentVersionsByResourcePidsAndFetchResourceTable
(List<JpaPidFk> theVersionlessPids) findForIdAndVersion
(JpaPidFk theId, long theVersion) org.springframework.data.domain.Slice
<ResourceHistoryTablePk> findForResourceId
(org.springframework.data.domain.Pageable thePage, JpaPidFk theId, Long theDontWantVersion) org.springframework.data.domain.Slice
<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResourceId
(org.springframework.data.domain.Pageable thePage, JpaPid theResourceId) org.springframework.data.domain.Slice
<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResources
(org.springframework.data.domain.Pageable thePage) org.springframework.data.domain.Slice
<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResources
(org.springframework.data.domain.Pageable thePage, String theResourceName) void
updateNonInlinedContents
(byte[] theText, ResourceHistoryTablePk thePid) This method is only for use in unit tests - It is used to move the stored resource body contents from the newRES_TEXT_VC
column to the legacyRES_TEXT
column, which is where data may have been stored by versions of HAPI FHIR prior to 7.0.0void
updateVersion
(JpaPidFk theId, long theOldVersion, long theNewVersion) Methods inherited from interface org.springframework.data.repository.CrudRepository
count, delete, deleteAll, deleteAll, deleteAllById, deleteById, existsById, findById, save
Methods inherited from interface org.springframework.data.jpa.repository.JpaRepository
deleteAllByIdInBatch, deleteAllInBatch, deleteAllInBatch, deleteInBatch, findAll, findAll, flush, getById, getOne, getReferenceById, saveAllAndFlush, saveAndFlush
Methods inherited from interface org.springframework.data.repository.ListCrudRepository
findAll, findAllById, saveAll
Methods inherited from interface org.springframework.data.repository.ListPagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.PagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.query.QueryByExampleExecutor
count, exists, findAll, findBy, findOne
-
Method Details
-
findAllVersionsForResourceIdInOrder
@Query("SELECT t FROM ResourceHistoryTable t WHERE t.myResourcePid = :resId ORDER BY t.myResourceVersion ASC") List<ResourceHistoryTable> findAllVersionsForResourceIdInOrder(@Param("resId") JpaPidFk theId) This is really only intended for unit tests - There can be many versions of resources in the real world, use a pageable query for real uses. -
findForIdAndVersion
@Query("SELECT t FROM ResourceHistoryTable t WHERE t.myResourcePid = :id AND t.myResourceVersion = :version") ResourceHistoryTable findForIdAndVersion(@Param("id") JpaPidFk theId, @Param("version") long theVersion) -
findForResourceId
@Query("SELECT t.myId FROM ResourceHistoryTable t WHERE t.myResourcePid = :resId AND t.myResourceVersion <> :dontWantVersion") org.springframework.data.domain.Slice<ResourceHistoryTablePk> findForResourceId(org.springframework.data.domain.Pageable thePage, @Param("resId") JpaPidFk theId, @Param("dontWantVersion") Long theDontWantVersion) -
findAllVersionsExceptSpecificForResourcePid
@Query("SELECT t FROM ResourceHistoryTable t WHERE t.myResourcePid = :resId AND t.myResourceVersion <> :dontWantVersion") org.springframework.data.domain.Slice<ResourceHistoryTable> findAllVersionsExceptSpecificForResourcePid(org.springframework.data.domain.Pageable thePage, @Param("resId") JpaPidFk theId, @Param("dontWantVersion") Long theDontWantVersion) -
findIdsOfPreviousVersionsOfResourceId
@Query("SELECT v.myId FROM ResourceHistoryTable v LEFT OUTER JOIN ResourceTable t ON (v.myResourceTable = t) WHERE v.myResourceVersion <> t.myVersion AND t.myPid = :resId") org.springframework.data.domain.Slice<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResourceId(org.springframework.data.domain.Pageable thePage, @Param("resId") JpaPid theResourceId) -
findIdsOfPreviousVersionsOfResources
@Query("SELECT v.myId FROM ResourceHistoryTable v LEFT OUTER JOIN ResourceTable t ON (v.myResourceTable = t) WHERE v.myResourceVersion <> t.myVersion AND t.myResourceType = :restype") org.springframework.data.domain.Slice<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResources(org.springframework.data.domain.Pageable thePage, @Param("restype") String theResourceName) -
findIdsOfPreviousVersionsOfResources
@Query("SELECT v.myId FROM ResourceHistoryTable v LEFT OUTER JOIN ResourceTable t ON (v.myResourceTable = t) WHERE v.myResourceVersion <> t.myVersion") org.springframework.data.domain.Slice<ResourceHistoryTablePk> findIdsOfPreviousVersionsOfResources(org.springframework.data.domain.Pageable thePage) -
updateVersion
@Modifying @Query("UPDATE ResourceHistoryTable r SET r.myResourceVersion = :newVersion WHERE r.myResourcePid = :id AND r.myResourceVersion = :oldVersion") void updateVersion(@Param("id") JpaPidFk theId, @Param("oldVersion") long theOldVersion, @Param("newVersion") long theNewVersion) -
deleteByPid
@Modifying @Query("DELETE FROM ResourceHistoryTable t WHERE t.myId = :pid") void deleteByPid(@Param("pid") ResourceHistoryTablePk theId) -
updateNonInlinedContents
@Modifying @Query("UPDATE ResourceHistoryTable r SET r.myResourceTextVc = null, r.myResource = :text, r.myEncoding = \'JSONC\' WHERE r.myId = :pid") void updateNonInlinedContents(@Param("text") byte[] theText, @Param("pid") ResourceHistoryTablePk thePid) This method is only for use in unit tests - It is used to move the stored resource body contents from the newRES_TEXT_VC
column to the legacyRES_TEXT
column, which is where data may have been stored by versions of HAPI FHIR prior to 7.0.0- Since:
- 7.0.0
-
findCurrentVersionsByResourcePidsAndFetchResourceTable
@Query("SELECT v FROM ResourceHistoryTable v JOIN FETCH v.myResourceTable t WHERE v.myResourcePid IN (:pids) AND t.myVersion = v.myResourceVersion") List<ResourceHistoryTable> findCurrentVersionsByResourcePidsAndFetchResourceTable(@Param("pids") List<JpaPidFk> theVersionlessPids)
-