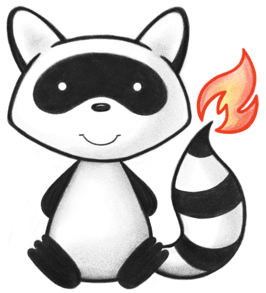
Package ca.uhn.fhir.jpa.dao.data
Interface IResourceTableDao
- All Superinterfaces:
org.springframework.data.repository.CrudRepository<ResourceTable,
,JpaPid> IForcedIdQueries
,IHapiFhirJpaRepository
,org.springframework.data.jpa.repository.JpaRepository<ResourceTable,
,JpaPid> org.springframework.data.repository.ListCrudRepository<ResourceTable,
,JpaPid> org.springframework.data.repository.ListPagingAndSortingRepository<ResourceTable,
,JpaPid> org.springframework.data.repository.PagingAndSortingRepository<ResourceTable,
,JpaPid> org.springframework.data.repository.query.QueryByExampleExecutor<ResourceTable>
,org.springframework.data.repository.Repository<ResourceTable,
JpaPid>
@Transactional(propagation=MANDATORY)
public interface IResourceTableDao
extends org.springframework.data.jpa.repository.JpaRepository<ResourceTable,JpaPid>, IHapiFhirJpaRepository, IForcedIdQueries
-
Method Summary
Modifier and TypeMethodDescriptionvoid
deleteByPid
(JpaPid theId) findAllByIdAndLoadForcedIds
(List<JpaPid> thePids) default Optional
<ResourceTable> Deprecated.findByTypeAndFhirId
(String theResourceName, String theFhirId) findCurrentVersionByPid
(JpaPid thePid) org.springframework.data.domain.Slice
<JpaPid> findIdsOfDeletedResources
(org.springframework.data.domain.Pageable thePageable) org.springframework.data.domain.Slice
<JpaPid> findIdsOfDeletedResourcesOfType
(org.springframework.data.domain.Pageable thePageable, Long theResourceId, String theResourceName) org.springframework.data.domain.Slice
<JpaPid> findIdsOfDeletedResourcesOfType
(org.springframework.data.domain.Pageable thePageable, String theResourceName) org.springframework.data.domain.Slice
<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromNewest
(org.springframework.data.domain.Pageable thePage, Date theLow, Date theHigh) org.springframework.data.domain.Slice
<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest
(org.springframework.data.domain.Pageable thePage, String theResourceType, Date theLow, Date theHigh) org.springframework.data.domain.Slice
<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest
(org.springframework.data.domain.Pageable thePage, Date theLow, Date theHigh) Collection
<Object[]> findLookupFieldsByResourcePid
(List<Long> thePids) This method returns a Collection where each row is an element in the collection.Collection
<Object[]> findLookupFieldsByResourcePidInPartitionIds
(List<Long> thePids, Collection<Integer> thePartitionId) This method returns a Collection where each row is an element in the collection.Collection
<Object[]> findLookupFieldsByResourcePidInPartitionIdsOrNullPartition
(List<Long> thePids, Collection<Integer> thePartitionId) This method returns a Collection where each row is an element in the collection.Collection
<Object[]> This method returns a Collection where each row is an element in the collection.Collection
<Object[]> Collection
<Object[]> This query will return rows with the following values: Id (JpaPid), FhirId, ResourceType (Patient, etc), version (long) Order matters!readByPartitionId
(int thePartitionId, Long theResourceId) readByPartitionIdNull
(Long theResourceId) readByPartitionIds
(Collection<Integer> thrValues, Long theResourceId) readByPartitionIdsOrNull
(Collection<Integer> thrValues, Long theResourceId) streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldest
(Date theLow, Date theHigh) streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForDefaultPartition
(Date theLow, Date theHigh) streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForPartitionIds
(Date theLow, Date theHigh, List<Integer> theRequestPartitionIds) void
updateIndexStatus
(JpaPid theId, EntityIndexStatusEnum theIndexStatus) void
updateLastUpdated
(JpaPid theId, Date theUpdated) Methods inherited from interface org.springframework.data.repository.CrudRepository
count, delete, deleteAll, deleteAll, deleteAllById, deleteById, existsById, findById, save
Methods inherited from interface ca.uhn.fhir.jpa.dao.data.custom.IForcedIdQueries
findAndResolveByForcedIdWithNoType, findAndResolveByForcedIdWithNoTypeIncludeDeleted, findAndResolveByForcedIdWithNoTypeInPartition, findAndResolveByForcedIdWithNoTypeInPartitionIdOrNullPartitionId, findAndResolveByForcedIdWithNoTypeInPartitionNull
Methods inherited from interface org.springframework.data.jpa.repository.JpaRepository
deleteAllByIdInBatch, deleteAllInBatch, deleteAllInBatch, deleteInBatch, findAll, findAll, flush, getById, getOne, getReferenceById, saveAllAndFlush, saveAndFlush
Methods inherited from interface org.springframework.data.repository.ListCrudRepository
findAll, findAllById, saveAll
Methods inherited from interface org.springframework.data.repository.ListPagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.PagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.query.QueryByExampleExecutor
count, exists, findAll, findBy, findOne
-
Method Details
-
findIdsOfDeletedResources
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myDeleted IS NOT NULL") org.springframework.data.domain.Slice<JpaPid> findIdsOfDeletedResources(org.springframework.data.domain.Pageable thePageable) -
findIdsOfDeletedResourcesOfType
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myResourceType = :restype AND t.myDeleted IS NOT NULL") org.springframework.data.domain.Slice<JpaPid> findIdsOfDeletedResourcesOfType(org.springframework.data.domain.Pageable thePageable, @Param("restype") String theResourceName) -
findIdsOfDeletedResourcesOfType
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myPid.myId = :resid AND t.myResourceType = :restype AND t.myDeleted IS NOT NULL") org.springframework.data.domain.Slice<JpaPid> findIdsOfDeletedResourcesOfType(org.springframework.data.domain.Pageable thePageable, @Param("resid") Long theResourceId, @Param("restype") String theResourceName) -
getResourceCounts
@Query("SELECT t.myResourceType as type, COUNT(t.myResourceType) as count FROM ResourceTable t GROUP BY t.myResourceType") List<Map<?,?>> getResourceCounts() -
findIdsOfResourcesWithinUpdatedRangeOrderedFromNewest
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high ORDER BY t.myUpdated DESC") org.springframework.data.domain.Slice<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromNewest(org.springframework.data.domain.Pageable thePage, @Param("low") Date theLow, @Param("high") Date theHigh) -
findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high ORDER BY t.myUpdated ASC") org.springframework.data.domain.Slice<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest(org.springframework.data.domain.Pageable thePage, @Param("low") Date theLow, @Param("high") Date theHigh) -
streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldest
@Query("SELECT t.myPid, t.myResourceType, t.myUpdated FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high ORDER BY t.myUpdated ASC") Stream<Object[]> streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldest(@Param("low") Date theLow, @Param("high") Date theHigh) -
streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForPartitionIds
@Query("SELECT t.myPid, t.myResourceType, t.myUpdated FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high AND t.myPartitionIdValue IN (:partition_ids) ORDER BY t.myUpdated ASC") Stream<Object[]> streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForPartitionIds(@Param("low") Date theLow, @Param("high") Date theHigh, @Param("partition_ids") List<Integer> theRequestPartitionIds) -
streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForDefaultPartition
@Query("SELECT t.myPid, t.myResourceType, t.myUpdated FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high ORDER BY t.myUpdated ASC") Stream<Object[]> streamIdsTypesAndUpdateTimesOfResourcesWithinUpdatedRangeOrderedFromOldestForDefaultPartition(@Param("low") Date theLow, @Param("high") Date theHigh) -
findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest
@Query("SELECT t.myPid FROM ResourceTable t WHERE t.myUpdated >= :low AND t.myUpdated <= :high AND t.myResourceType = :restype ORDER BY t.myUpdated ASC") org.springframework.data.domain.Slice<JpaPid> findIdsOfResourcesWithinUpdatedRangeOrderedFromOldest(org.springframework.data.domain.Pageable thePage, @Param("restype") String theResourceType, @Param("low") Date theLow, @Param("high") Date theHigh) -
updateIndexStatus
@Modifying @Query("UPDATE ResourceTable t SET t.myIndexStatus = :status WHERE t.myPid = :id") void updateIndexStatus(@Param("id") JpaPid theId, @Param("status") EntityIndexStatusEnum theIndexStatus) -
updateLastUpdated
@Modifying @Query("UPDATE ResourceTable t SET t.myUpdated = :updated WHERE t.myPid = :id") void updateLastUpdated(@Param("id") JpaPid theId, @Param("updated") Date theUpdated) -
deleteByPid
@Modifying @Query("DELETE FROM ResourceTable t WHERE t.myPid = :pid") void deleteByPid(@Param("pid") JpaPid theId) -
findLookupFieldsByResourcePid
@Query("SELECT t.myResourceType, t.myPid.myId, t.myDeleted, t.myPartitionIdValue, t.myPartitionDateValue FROM ResourceTable t WHERE t.myPid.myId IN (:pid)") Collection<Object[]> findLookupFieldsByResourcePid(@Param("pid") List<Long> thePids) This method returns a Collection where each row is an element in the collection. Each element in the collection is an object array, where the order matters (the array represents columns returned by the query). Be careful if you change this query in any way. -
findLookupFieldsByResourcePidWithPartitionId
@Query("SELECT t.myResourceType, t.myPid.myId, t.myDeleted, t.myPartitionIdValue, t.myPartitionDateValue FROM ResourceTable t WHERE t.myPid IN (:pid)") Collection<Object[]> findLookupFieldsByResourcePidWithPartitionId(@Param("pid") List<JpaPid> thePids) -
findLookupFieldsByResourcePidInPartitionIds
@Query("SELECT t.myResourceType, t.myPid.myId, t.myDeleted, t.myPartitionIdValue, t.myPartitionDateValue FROM ResourceTable t WHERE t.myPid.myId IN (:pid) AND t.myPartitionIdValue IN :partition_id") Collection<Object[]> findLookupFieldsByResourcePidInPartitionIds(@Param("pid") List<Long> thePids, @Param("partition_id") Collection<Integer> thePartitionId) This method returns a Collection where each row is an element in the collection. Each element in the collection is an object array, where the order matters (the array represents columns returned by the query). Be careful if you change this query in any way. -
findLookupFieldsByResourcePidInPartitionIdsOrNullPartition
@Query("SELECT t.myResourceType, t.myPid.myId, t.myDeleted, t.myPartitionIdValue, t.myPartitionDateValue FROM ResourceTable t WHERE t.myPid.myId IN (:pid) AND (t.myPartitionIdValue IS NULL OR t.myPartitionIdValue IN :partition_id)") Collection<Object[]> findLookupFieldsByResourcePidInPartitionIdsOrNullPartition(@Param("pid") List<Long> thePids, @Param("partition_id") Collection<Integer> thePartitionId) This method returns a Collection where each row is an element in the collection. Each element in the collection is an object array, where the order matters (the array represents columns returned by the query). Be careful if you change this query in any way. -
findLookupFieldsByResourcePidInPartitionNull
@Query("SELECT t.myResourceType, t.myPid.myId, t.myDeleted, t.myPartitionIdValue, t.myPartitionDateValue FROM ResourceTable t WHERE t.myPid.myId IN (:pid) AND t.myPartitionIdValue IS NULL") Collection<Object[]> findLookupFieldsByResourcePidInPartitionNull(@Param("pid") List<Long> thePids) This method returns a Collection where each row is an element in the collection. Each element in the collection is an object array, where the order matters (the array represents columns returned by the query). Be careful if you change this query in any way. -
findCurrentVersionByPid
@Query("SELECT t.myVersion FROM ResourceTable t WHERE t.myPid = :pid") Long findCurrentVersionByPid(@Param("pid") JpaPid thePid) -
getResourceVersionsForPid
@Query("SELECT t.myPid, t.myResourceType, t.myFhirId, t.myVersion FROM ResourceTable t WHERE t.myPid IN ( :pid )") Collection<Object[]> getResourceVersionsForPid(@Param("pid") Collection<JpaPid> pid) This query will return rows with the following values: Id (JpaPid), FhirId, ResourceType (Patient, etc), version (long) Order matters!- Parameters:
pid
- - list of pids to get versions for
-
readByPartitionIdNull
@Query("SELECT t FROM ResourceTable t WHERE t.myPartitionIdValue IS NULL AND t.myPid.myId = :pid") Optional<ResourceTable> readByPartitionIdNull(@Param("pid") Long theResourceId) -
readByPartitionId
@Query("SELECT t FROM ResourceTable t WHERE t.myPartitionIdValue = :partitionId AND t.myPid.myId = :pid") Optional<ResourceTable> readByPartitionId(@Param("partitionId") int thePartitionId, @Param("pid") Long theResourceId) -
readByPartitionIdsOrNull
@Query("SELECT t FROM ResourceTable t WHERE (t.myPartitionIdValue IS NULL OR t.myPartitionIdValue IN (:partitionIds)) AND t.myPid.myId = :pid") Optional<ResourceTable> readByPartitionIdsOrNull(@Param("partitionIds") Collection<Integer> thrValues, @Param("pid") Long theResourceId) -
readByPartitionIds
@Query("SELECT t FROM ResourceTable t WHERE t.myPartitionIdValue IN (:partitionIds) AND t.myPid.myId = :pid") Optional<ResourceTable> readByPartitionIds(@Param("partitionIds") Collection<Integer> thrValues, @Param("pid") Long theResourceId) -
findAllByIdAndLoadForcedIds
@Query("SELECT t FROM ResourceTable t WHERE t.myPid IN :pids") List<ResourceTable> findAllByIdAndLoadForcedIds(@Param("pids") List<JpaPid> thePids) -
findByTypeAndFhirId
@Query("SELECT t FROM ResourceTable t where t.myResourceType = :restype and t.myFhirId = :fhirId") Optional<ResourceTable> findByTypeAndFhirId(@Param("restype") String theResourceName, @Param("fhirId") String theFhirId) -
findById
Deprecated.UseCrudRepository.findById(Object)
-
CrudRepository.findById(Object)